LeetCode In Python: 50 Algorithms Coding Interview Questions
In this course, you'll have a detailed, step by step explanation of 50 hand-picked LeetCode questions where you'll learn about the most popular techniques and problems used in the coding interview, This is the course I wish I had when I was doing my interviews.
Read more about the course
- Getting ready for your software engineering coding interview? This is the place for you.
Want to learn about the most popular techniques, patterns, data structures and algorithms used in those difficult interviews? This is the place for you.
Want a step by step explanation of 50 of the most popular interview questions in the industry? This is the place for you.
What is LeetCode?
LeetCode is essentially a huge repository of real interview questions asked by the most popular tech companies ( Google, Amazon, Facebook, Microsoft and more ).
The problem with LeetCode is also its advantage, IT'S HUGE, so huge in fact that interviewers from the most popular companies often directly ask questions they find on LeetCode, So it's hard to navigate through the huge amount of problems to find those that really matter, this is what this course is for.
I spent countless hours on LeetCode and I'm telling you that you don't have to do the same and still be able to get a job at a major tech company.
In this course, I compiled 50 of the most important and the most popular interview questions asked by these major companies and I explain them, in a true STEP BY STEP fashion to help you understand exactly how to solve these types of questions.
The problems are handpicked to ensure complete coverage of the most popular techniques, data structures, and algorithms used in interviews so you can generalize the patterns you learn here on other problems.
Each problem gets 2 videos :
Explanation video: we do a detailed explanation of the problems and its solution, this video will be longer because we will do a step by step explanation for the problems.
Coding video: where we code the solution discussed in the explanation video together.
We will use basic python for this course to code our solutions, previous knowledge in python is preferred but NOT required for the coding part of the course. The problems are categorized for easier navigation and will be regularly updated with more popular and interesting problems.
Some of the stuff this course will cover are :
Arrays and Strings.
Searching.
Dynamic Programming.
Backtracking ( With step by step visualization ).
Trees and Graphs.
Data structures Like Stacks, Queues, Maps, Linked Lists, and more.
- Basic Knowledge of Fundamental data structures and algorithms is preferred
- Basic Knowledge of python is prefered
Who this course is for:
- Developers eager to pass the coding interview at huge companies like Google, Facebook, Microsoft, Amazon, etc.
- People who want to develop their problem solving skills.
- Developers getting ready for their interviews.
- Students getting ready for their internship interviews.
What you'll learn:
- How to solve some of the most popular interview questions asked by major tech companies
- Popular problems patterns
- Breaking down the coding interview problems
- Algorithms and Data structures
- Arrays, Stacks, Queues, Maps and Linked Lists
- Dynamic Programming
- Backtracking
- Search techniques
- Math techniques
- Trees and Graphs
Watch Online LeetCode In Python: 50 Algorithms Coding Interview Questions
# | Title | Duration |
---|---|---|
1 | Introduction | 01:36 |
2 | How to use this course | 01:56 |
3 | Big O Introduction | 04:24 |
4 | Big O Exercises | 06:17 |
5 | Formalizing Big O | 09:20 |
6 | Big O Simplification | 05:33 |
7 | Space complexity | 04:51 |
8 | Logarithms | 06:30 |
9 | Binary Search Algorithm | 08:05 |
10 | Binary Search Implementation | 05:46 |
11 | Sliding Window Technique | 10:29 |
12 | Sliding Window Implementation | 05:04 |
13 | Explanation - Move Zeroes - Easy #283 | 09:09 |
14 | Code - Move Zeroes - Easy #283 | 02:29 |
15 | [Custom Input - NEW] - Move Zeroes | 02:34 |
16 | Explanation - Boats to Save People - Medium #881 | 11:59 |
17 | Code - Boats to Save People - Medium #881 | 03:59 |
18 | [Custom Input - NEW] - Boats to save people | 05:40 |
19 | Explanation - Valid Mountain Array - Easy #941 | 10:21 |
20 | Code - Valid Mountain Array - Easy #941 | 03:50 |
21 | [Custom Input - NEW] - Valid Mountain Array | 02:33 |
22 | Explanation - Container With Most Water - Medium #11 | 11:40 |
23 | Code - Container With Most Water - Medium #11 | 05:00 |
24 | [Custom Input - NEW] - Container with most water | 04:30 |
25 | Explanation - Longest Substring Without Repeating Characters - Medium #3 | 12:01 |
26 | Code - Longest Substring Without Repeating Characters - Medium #3 | 02:51 |
27 | [Custom Input - NEW] - Longest Substring Without repeating characters | 01:39 |
28 | Explanation - Find First and Last Position of Element in Sorted Array-Medium #34 | 17:35 |
29 | Code - Find First and Last Position of Element in Sorted Array - Medium #34 | 09:24 |
30 | [Custom Input - NEW] - Find first and last position of element in sorted array | 03:03 |
31 | Explanation - First Bad Version - Easy #278 | 07:26 |
32 | Code - First Bad Version - Easy #278 | 03:34 |
33 | [Custom Input - NEW] - First Bad Version - Easy #278 | 05:55 |
34 | Explanation - Missing Number - Easy #268 | 08:38 |
35 | Code - Missing Number - Easy #268 | 01:48 |
36 | [Custom Input - NEW] - Missing Number - Easy #268 | 01:34 |
37 | Explanation - Count Primes - Easy #204 | 05:00 |
38 | Code - Count Primes - Easy #204 | 03:44 |
39 | [Custom Input - NEW] - Count Primes - Easy #204 | 01:29 |
40 | Explanation - Single Number - Easy #136 | 05:05 |
41 | Code - Single Number - Easy #136 | 00:55 |
42 | [Custom Input - NEW] - Single Number - Easy#136 | 01:00 |
43 | Explanation - Robot Return to Origin - Easy #657 | 03:08 |
44 | Code - Robot Return to Origin - Easy #657 | 01:54 |
45 | [Custom Input - NEW] - Robot Return to Origin - Easy #657 | 02:03 |
46 | Explanation - Add Binary - Easy #67 | 06:14 |
47 | Code - Add Binary - Easy #67 | 05:19 |
48 | [Custom Input - NEW] - Add Binary - Easy #67 | 02:24 |
49 | What are hash tables ? | 05:33 |
50 | Collision handling | 07:06 |
51 | Collision handling techniques comparison | 03:48 |
52 | Explanation - Two Sum - Easy #1 | 06:51 |
53 | Code - Two Sum - Easy #1 | 02:26 |
54 | [Custom Input] - Two Sum - Easy #1 | 02:13 |
55 | Explanation - Contains Duplicate - Easy #217 | 05:00 |
56 | Code - Contains Duplicate - Easy #217 | 02:06 |
57 | [Custom Input] - Contains Duplicate - Easy #217 | 01:43 |
58 | Explanation - Majority Element - Easy #169 | 03:41 |
59 | Code - Majority Element - Easy #169 | 01:54 |
60 | [Custom Input] - Majority Element - Easy #169 | 03:23 |
61 | Explanation - Group Anagrams - Medium #49 | 06:06 |
62 | Code - Group Anagrams - Medium #49 | 03:44 |
63 | [Custom Input] - Group Anagrams - Medium #49 | 03:16 |
64 | Explanation - 4Sum II - Medium #454 | 09:03 |
65 | Code - 4Sum II - Medium #454 | 04:36 |
66 | [Custom Input] - 4Sum II - Medium #454 | 05:47 |
67 | Explanation - LRU Cache - Medium #146 | 14:35 |
68 | Code - LRU Cache - Medium #146 | 04:49 |
69 | [Custom Input] - LRU Cache - Medium #146 | 04:10 |
70 | Explanation - Minimum Window Substring - Hard #76 | 20:09 |
71 | Code - Minimum Window Substring - Hard #76 | 10:20 |
72 | What are Linked Lists ? | 07:30 |
73 | Singly linked list creation implementation | 05:02 |
74 | Singly linked list insertion implementation | 07:32 |
75 | Singly linked list deletion implementation | 04:29 |
76 | Doubly Linked Lists | 02:59 |
77 | Doubly linked lists creation implementation | 08:45 |
78 | Doubly linked lists insertion implementation | 10:23 |
79 | Doubly linked list deletion implementation | 08:47 |
80 | Explanation - Merge Two Sorted Lists - Easy #21 | 07:11 |
81 | Code - Merge Two Sorted Lists - Easy #21 | 05:22 |
82 | [Custom Input - NEW] - Merge Two Sorted Lists - Easy #21 | 06:02 |
83 | Explanation - Linked List Cycle - Easy #141 | 04:51 |
84 | Code - Linked List Cycle - Easy #141 | 01:55 |
85 | [Custom Input - NEW] - Linked List Cycle - Easy #141 | 04:41 |
86 | Explanation - Reverse Linked List - Easy #206 | 08:30 |
87 | Code - Reverse Linked List - Easy #206 | 02:10 |
88 | [Custom Input - NEW] - Reverse Linked List - Easy #206 | 04:12 |
89 | Explanation - Add Two Numbers - Medium #2 | 09:53 |
90 | Code - Add Two Numbers - Medium #2 | 04:49 |
91 | [Custom Input - NEW] - Add Two Numbers - Medium #2 | 06:41 |
92 | Explanation - Remove Nth Node From End of List - Medium #19 | 07:28 |
93 | Code - Remove Nth Node From End of List - Medium #19 | 02:36 |
94 | [Custom Input - NEW] - Remove Nth Node From End of List - Medium #19 | 04:36 |
95 | Explanation - Odd Even Linked List - Medium #328 | 07:38 |
96 | Code - Odd Even Linked List - Medium #328 | 04:04 |
97 | [Custom Input - NEW] - Odd Even Linked List - Medium #328 | 05:33 |
98 | Explanation - Merge K Sorted Lists - Hard #23 | 09:26 |
99 | Code - Merge K Sorted Lists - Hard #23 | 08:02 |
100 | Explanation - Subsets - Medium #78 | 22:25 |
101 | Code - Subsets - Medium #78 | 03:03 |
102 | Explanation - Letter Combinations of a Phone Number - Medium #17 | 20:01 |
103 | Code - Letter Combinations of a Phone Number - Medium #17 | 06:02 |
104 | Explanation - Word Search - Medium #79 | 28:24 |
105 | Code - Word Search - Medium #79 | 09:09 |
106 | Explanation - Combination Sum - Medium #39 | 29:00 |
107 | Code - Combination Sum - Medium #39 | 03:51 |
108 | Explanation - Palindrome Partitioning - Medium #131 | 11:59 |
109 | Code - Palindrome Partitioning - Medium #131 | 05:29 |
110 | What are stacks ? | 04:03 |
111 | Stacks Implementation | 02:43 |
112 | What are Queues | 03:28 |
113 | Queues Implementation | 02:17 |
114 | What are Graphs ? | 04:05 |
115 | Directed Graph Implementation - Adjacency List | 07:57 |
116 | Directed Graph Implementation - Adjacency Matrix | 10:14 |
117 | Undirected Graph Implementation - Adjacency List | 07:29 |
118 | Undirected Graph Implementation - Adjacency Matrix | 06:12 |
119 | Depth First Search (DFS) | 08:57 |
120 | DFS implementation | 08:09 |
121 | Breadth First Search (BFS) | 08:35 |
122 | BFS Implementation | 07:55 |
123 | Dijkstra's Algorithm | 11:13 |
124 | Explanation - network delay time - Medium #743 | 08:13 |
125 | Code - network delay time - Medium #743 | 08:55 |
126 | What are trees ? | 11:40 |
127 | Trees Implementation | 03:33 |
128 | In-order Traversal Technique | 04:23 |
129 | In-order traversal implementation | 04:48 |
130 | Pre-order Traversal Technique | 04:05 |
131 | Pre-order traversal implementation | 03:20 |
132 | Post-order Traversal Technique | 03:54 |
133 | Post-order traversal implementation | 03:20 |
134 | What is a binary search tree (BST) | 06:44 |
135 | Binary Search tree creation and insertion implementation | 10:46 |
136 | Binary search tree searching Implementation | 06:31 |
137 | BInary search tree deletion implementation | 07:10 |
138 | Explanation - Symmetric Trees - Easy #101 | 08:30 |
139 | Code - Symmetric Trees - Easy #101 | 02:45 |
140 | Explanation - Maximum Depth of Binary Tree - Easy #104 | 12:04 |
141 | Code - Maximum Depth of Binary Tree - Easy #104 | 02:15 |
142 | Explanation - Path Sum - Easy #112 | 06:31 |
143 | Code - Path Sum - Easy #112 | 02:36 |
144 | Explanation- Lowest Common Ancestor of a Binary Tree - Medium #236 | 14:46 |
145 | Code- Lowest Common Ancestor of a Binary Tree - Medium #236 | 03:05 |
146 | Explanation - Kth Smallest Element in a BST - Medium #230 | 10:30 |
147 | Code - Kth Smallest Element in a BST - Medium #230 | 01:57 |
148 | Explanation - Serialize and Deserialize Binary Tree - hard #297 | 11:01 |
149 | Code - Serialize and Deserialize Binary Tree - hard #297 | 05:20 |
150 | Explanation - Binary Tree Maximum Path Sum - Hard #124 | 23:45 |
151 | Code - Binary Tree Maximum Path Sum - Hard #124 | 03:28 |
152 | Explanation - Min Stack - Easy #155 | 06:07 |
153 | Code - Min Stack - Easy #155 | 02:28 |
154 | Explanation - Valid Parenthesis - Easy #20 | 09:19 |
155 | Code - Valid Parenthesis - Easy #20 | 04:19 |
156 | Explanation - Binary Tree Level Order Traversal - Medium #102 | 10:54 |
157 | Code - Binary Tree Level Order Traversal - Medium #102 | 03:23 |
158 | Explanation - Binary Tree Zigzag Level Order Traversal - Medium #103 | 14:44 |
159 | Code - Binary Tree Zigzag Level Order Traversal - Medium #103 | 04:06 |
160 | Explanation - Binary Tree Postorder Traversal - Medium #145 | 10:28 |
161 | Code - Binary Tree Postorder Traversal - Medium #145 | 02:14 |
162 | What is Dynamic Programming ? | 06:52 |
163 | Explanation - House Robber - Easy #198 | 06:44 |
164 | Code - House Robber - Easy #198 | 03:57 |
165 | Explanation - Best Time to Buy and Sell Stock - Easy #121 | 08:35 |
166 | Code - Best Time to Buy and Sell Stock - Easy #121 | 02:13 |
167 | Explanation - Climbing Stairs - Easy #70 | 04:52 |
168 | Code - Climbing Stairs - Easy #70 | 03:05 |
169 | Explanation - Coin Change - Medium #322 | 13:04 |
170 | Code - Coin Change - Medium #322 | 04:04 |
171 | Explanation - Unique Paths - Medium #62 | 08:42 |
172 | Code - Unique Paths - Medium #62 | 03:18 |
173 | Explanation - Longest Palindromic Substring - Medium #5 | 22:44 |
174 | Code - Longest Palindromic Substring - Medium #5 | 04:25 |
175 | Explanation - Trapping Rain Water - Hard #42 | 21:55 |
176 | Code - Trapping Rain Water - Hard #42 | 04:22 |
Similar courses to LeetCode In Python: 50 Algorithms Coding Interview Questions
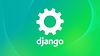
The Ultimate Django Series: Part 1codewithmosh (Mosh Hamedani)
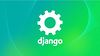
The Ultimate Django Series: Part 2codewithmosh (Mosh Hamedani)
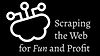
Scraping the Web for Fun and ProfitJakob Greenfeld
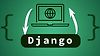
Python Django - The Practical GuideAcademind Pro
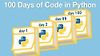
#100DaysOfCode with Python courseTalkpython
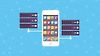
REST APIs with Flask and Pythonudemy
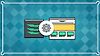
Build a Backend REST API with Python & Django - Advancedudemy
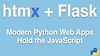
HTMX + Flask: Modern Python Web Apps, Hold the JavaScriptTalkpython
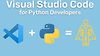
Visual Studio Code for Python DevelopersTalkpython
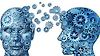