Angular Architecture. How to Build Scalable Web Applications
The main goal of the course is to learn how to make scalable applications that will be easy to maintain, and on which you can conveniently work as a team. You will see that a correctly chosen architecture allows you to concentrate on the tasks of business logic and saves time if you are developing a large project. If your application has become confusing and hard to maintain, or if you know the elements of Angular, but you are not sure how they can be combined in the best way, this course is for you.
More
We will use strong TypeScript data-models, NgRx to store data, Firebase as a backend, SCSS and BEM-methodology for styles, Angular Material as a core of Shared controls. And of course RxJS to make all these things work together.
The course is designed for students who have already had [already have] experience in developing with Angular, at least at the level of creating Tour of Heroes from the official site and familiarization with the Angular documentation.
During this course, we will create the CourseApp application, which is a platform for posting resumes and jobs.
The final application will be quite large, because it is impossible to show complex architecture on a simple application. But each section is accompanied by ready-made code that you can use.
Watch Online Angular Architecture. How to Build Scalable Web Applications
# | Title | Duration |
---|---|---|
1 | Course Preview | 04:26 |
2 | How to work with the course | 00:55 |
3 | Installation | 01:34 |
4 | THEORY: General Structure of Web Applications | 03:49 |
5 | THEORY: Border between Angular and Typescript | 02:28 |
6 | Preview | 00:21 |
7 | Default Settings | 01:52 |
8 | Tsconfig | 01:51 |
9 | Environments | 04:56 |
10 | Packages | 00:37 |
11 | THEORY: Firebase | 01:50 |
12 | Firebase Control Panel | 01:20 |
13 | Firebase config | 00:48 |
14 | Adding Firebase in Module and Component | 01:42 |
15 | Firestore | 02:41 |
16 | Speed Optimization | 01:04 |
17 | Removing Default page | 00:25 |
18 | THEORY: Styles | 01:25 |
19 | Tools | 01:03 |
20 | THEORY: SCSS | 01:34 |
21 | THEORY: BEM | 03:20 |
22 | THEORY: Component Styles | 00:45 |
23 | THEORY: Styles Structure | 01:19 |
24 | Styles Folder | 06:45 |
25 | Header Component | 02:02 |
26 | Demo Section | 05:41 |
27 | Ending | 00:20 |
28 | THEORY: Review basic Angular Elements | 04:41 |
29 | THEORY: Division into Modules and Elements Grouping | 05:27 |
30 | THEORY: When does a Component become a Module? | 02:41 |
31 | Intro | 01:01 |
32 | THEORY: Shared | 03:35 |
33 | Angular Material Folders | 00:13 |
34 | Shared Folders | 01:35 |
35 | THEORY: Moving Components to Shared | 03:32 |
36 | Button | 03:04 |
37 | THEORY: Form Controls | 03:02 |
38 | Input | 06:38 |
39 | THEORY: Validation | 00:44 |
40 | Form-field | 08:05 |
41 | Form-field Validators | 04:18 |
42 | Password | 05:45 |
43 | THEORY: Frontend Models | 01:20 |
44 | Frontend Models | 02:12 |
45 | THEORY: Why use a Shared Component if you already have a Material Component? | 01:25 |
46 | Select | 07:17 |
47 | Checkboxes | 06:09 |
48 | Radios | 03:21 |
49 | Date | 07:05 |
50 | DateRange | 06:12 |
51 | Autocomplete Preview | 00:15 |
52 | Autocomplete | 10:14 |
53 | Form Buttons | 03:37 |
54 | Demo Actions Section | 01:00 |
55 | Spinner | 03:42 |
56 | THEORY: Services | 02:32 |
57 | Notification | 06:29 |
58 | Ending | 00:51 |
59 | Preview | 00:30 |
60 | THEORY: Data Models | 03:28 |
61 | Deleting Test DB | 00:18 |
62 | Backend Models | 04:35 |
63 | Firebase Collections | 02:25 |
64 | Ending | 00:25 |
65 | Form Items | 00:33 |
66 | THEORY: NgRx | 04:18 |
67 | NgRx DevTools | 00:27 |
68 | Basic Files | 01:15 |
69 | Models | 00:54 |
70 | Actions and Reducer | 04:02 |
71 | THEORY: RxJS | 06:25 |
72 | Effects | 06:09 |
73 | Root Registration | 00:41 |
74 | Selectors | 02:03 |
75 | Store App Module | 02:26 |
76 | Redux Tool | 00:19 |
77 | Flags | 00:39 |
78 | Assets | 01:15 |
79 | Countries | 02:42 |
80 | Ending | 00:26 |
81 | Preview | 00:31 |
82 | Enable Firebase Auth | 00:31 |
83 | User Store: Structure | 00:33 |
84 | User Store: Models | 00:49 |
85 | User Store: Actions | 01:39 |
86 | User Store: Reducer | 01:33 |
87 | Effects: SignUp | 03:16 |
88 | Effects: SignIn | 02:05 |
89 | Effects: SignOut | 00:35 |
90 | Selectors | 01:25 |
91 | Auth Forms Intro | 00:08 |
92 | Auth Page Structure | 02:25 |
93 | Header Links | 00:12 |
94 | App Routing | 00:26 |
95 | Registration: Form Validation | 03:58 |
96 | Registration: Template | 03:05 |
97 | Registration: Loading | 00:55 |
98 | Registration: Dispatch | 00:44 |
99 | Registration: test | 00:27 |
100 | Registration: Store Data | 00:33 |
101 | Registration: Firebase Redirect | 00:29 |
102 | Email Confirm | 01:11 |
103 | Init User Intro | 00:20 |
104 | Store: Init | 03:29 |
105 | Sign Out | 02:39 |
106 | Log In | 03:27 |
107 | Ending | 00:31 |
108 | Intro | 00:19 |
109 | Structure | 02:35 |
110 | Markup and Styles | 01:00 |
111 | Preview | 00:26 |
112 | Intro | 00:46 |
113 | Structure | 01:35 |
114 | Directive | 00:58 |
115 | Directive + Demo | 02:08 |
116 | Template | 01:15 |
117 | Dropzone Directive | 02:04 |
118 | OnDrop | 02:27 |
119 | Select and Dropzone Test | 01:30 |
120 | Upload Component | 06:13 |
121 | Firebase Storage | 01:07 |
122 | FileSize Pipe | 01:05 |
123 | URL Paths to Demo | 02:05 |
124 | Cropper | 05:00 |
125 | Ending | 00:18 |
126 | Intro | 01:06 |
127 | THEORY: Profile | 03:37 |
128 | Structure | 02:16 |
129 | Profile Link | 00:54 |
130 | Form Markup | 00:17 |
131 | THEORY: Stepper | 02:09 |
132 | Stepper [part 1] | 01:29 |
133 | Stepper [part 2] | 02:21 |
134 | Stepper [part 3] | 01:05 |
135 | Stepper [part 4] | 01:16 |
136 | Stepper [part 5] | 01:40 |
137 | Stepper [part 6] | 01:04 |
138 | Stepper [part 7] | 07:45 |
139 | Dictionaries Intro | 00:38 |
140 | Dictionaries Import | 01:00 |
141 | THEORY: Form Models | 01:44 |
142 | THEORY: Form Tasks | 02:11 |
143 | Personal Modules | 00:39 |
144 | Personal | 07:05 |
145 | UserPhoto Module | 03:07 |
146 | Professional [part 1] | 01:26 |
147 | Professional [part 2] | 02:08 |
148 | Professional Roles | 00:52 |
149 | Recruiter Form | 03:16 |
150 | Employee Form | 03:33 |
151 | Experiences | 06:52 |
152 | Utils Control | 01:14 |
153 | Employee Controls | 04:42 |
154 | Store: User Create and Update | 05:13 |
155 | Init Form Store | 01:13 |
156 | Store: Form | 02:41 |
157 | Resolver | 02:07 |
158 | Mapper [part 1] | 01:38 |
159 | Mapper [part 2] | 05:53 |
160 | Mapper [part 3] | 03:55 |
161 | Form Testing | 02:23 |
162 | Firebase Testing | 00:26 |
163 | Form Finishing | 01:45 |
164 | Store: Display | 04:08 |
165 | Display [part 1] | 04:29 |
166 | Display [part 2] | 03:07 |
167 | Testing in Redux Tool | 01:22 |
168 | Firebase Rules | 00:31 |
169 | Dictionaries Request | 01:39 |
170 | Ending | 00:45 |
171 | Intro | 00:12 |
172 | Structure | 01:11 |
173 | Store | 05:35 |
174 | Root Module | 00:23 |
175 | Employees Component | 00:48 |
176 | Employee Component | 02:02 |
177 | Demo | 00:52 |
178 | Intro | 00:27 |
179 | Structure | 01:02 |
180 | Store Models and Actions | 02:09 |
181 | @angular/entity Intro | 00:44 |
182 | Reducer | 02:16 |
183 | Effects | 02:20 |
184 | Selectors | 01:50 |
185 | Jobs Component | 00:32 |
186 | Adding Nested Components | 00:57 |
187 | Job Component | 01:34 |
188 | Form Module | 04:28 |
189 | isEditable | 01:30 |
190 | THEORY: Guards | 02:18 |
191 | Demo | 02:30 |
192 | Structure | 01:08 |
193 | Auth and Unauth Guards | 02:08 |
194 | Role Guard | 01:58 |
195 | Demo Guards | 03:13 |
196 | Application Guards | 02:02 |
197 | Dev/Prod Building | 01:42 |
198 | Firebase Hosting | 02:51 |
199 | Redirect | 01:11 |
200 | Congratulations! | 00:14 |
Similar courses to Angular Architecture. How to Build Scalable Web Applications
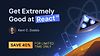
Learn React 19 with Epic React v2Kent C. Dodds
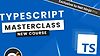
TypeScript MasterclassNet Ninja
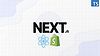
Next JS & Typescript with Shopify Integration - Full Guideudemy
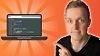
Build Fullstack Trello clone: WebSocket, Socket IOudemy
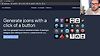
Building an AI Icon Generator using the T3 Stack (Next.js, Prisma, TailwindCSS, Typescript, Dall-E API)Web Dev Cody
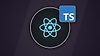
React & TypeScript - The Practical GuideAcademind Pro
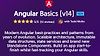
Angular Basics (v15)ultimatecourses.com
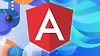
Complete Angular Developer in 2023 Zero to Masteryzerotomastery.io
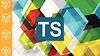