Build Web Apps with React & Firebase
React is a hugely popular front-end library and React developers are always in hight demand in the web dev job market. In this course you'll learn how to use React from the ground-up to create dynamic & interactive websites, and by the time you finish you'll be in a great position to succeed in a job as a React developer. You'll also have 4 full React projects under your belt too, which you can customize and use in your portfolio!
Read more about the course
Throughout the course you'll learn exactly what React is and why it's such a popular choice to make interactive & dynamic websites. You'll learn how to set up a React website from scratch, how to create React components, how to use state to manage component data & how to work with interactive events such as click events & form submissions.
You'll also get hands-on practise with the React Router (which is used in React to create website with "multiple pages") and you'll see how these are actually known as Single Page Applications (or SPA's for short).
We'll dive into React Hooks such as useState, useEffect, useParams & useHistory and use them to help us create 4 full React projects from scratch - a memory game, a recipe website, a finance tracker & a project management application.
You'll also learn some more avanced topics such as the React Context API to handle global state & reducers (including the useReducer hook) to help manage more complex state.
Once you've mastered React, we'll take our websites to the next level by integrating them with Firebase - a backend as a service. You'll learn how to add services such as a real-time database & authentication into your React sites as well as how to allow end-users to upload files from their computers with the help of Firebase Storage. Finally, I'll teach you how to build & deploy your React sites to the web using Firebase Hosting.
By the end of the course you'll have a solid understanding of React & be able to make your own production-ready websites!
Watch Online Build Web Apps with React & Firebase
# | Title | Duration |
---|---|---|
1 | Welcome to the Course | 02:57 |
2 | React at a Glance | 04:05 |
3 | What You Should Already Know | 01:19 |
4 | Environment Setup | 03:08 |
5 | Using the Course Files | 01:38 |
6 | Using React with a CDN | 05:08 |
7 | Making a React Component | 04:40 |
8 | JSX & Templates | 03:51 |
9 | Template Expressions & Variables | 04:46 |
10 | Click Events & Event Handlers | 06:08 |
11 | Making a React Site (create-react-app) | 03:57 |
12 | Project Structure Walkthrough | 07:24 |
13 | Running the Application | 04:36 |
14 | Using Images | 03:34 |
15 | Using Stylesheets | 03:19 |
16 | Why We Need State | 07:14 |
17 | Using the useState Hook | 06:52 |
18 | How State & Rendering Works | 04:51 |
19 | Outputting Lists | 08:19 |
20 | Using the Previous State | 09:37 |
21 | Conditional Templates | 06:54 |
22 | useState Limitations | 01:36 |
23 | Using Mutliple Components | 01:39 |
24 | Creating a Title Component | 05:31 |
25 | Intro to Props | 05:52 |
26 | React Fragments | 03:48 |
27 | Children Prop (Making a Modal Component) | 07:46 |
28 | Functions as Props | 04:56 |
29 | CHALLENGE - Showing the Modal | 02:29 |
30 | Portals | 03:51 |
31 | CHALLENGE - Reusable Event List Component | 06:54 |
32 | Class Components Overview | 02:47 |
33 | Using Global Stylesheets | 03:10 |
34 | Component Stylesheets | 06:38 |
35 | Using Inline Styles | 03:21 |
36 | Dynamic Inline Styles | 02:37 |
37 | Conditional CSS Classes | 02:47 |
38 | CSS Modules | 06:23 |
39 | Forms & Labels in React | 07:16 |
40 | The onChange Event | 06:17 |
41 | Controlled Inputs | 05:36 |
42 | Submitting Forms (onSubmit) | 06:21 |
43 | Adding Events to the Event List | 06:36 |
44 | Using the useRef Hook | 06:33 |
45 | Select Boxes | 05:22 |
46 | New Project & JSON Server | 07:01 |
47 | Why We Need useEffect | 08:19 |
48 | Fetching Data with useEffect | 07:49 |
49 | The useEffect Dependency Array | 09:25 |
50 | useCallback for Function Dependencies | 08:30 |
51 | Creating a Custom Fetch Hook | 14:04 |
52 | Adding a Loading/Pending State | 04:25 |
53 | Handling Errors | 08:43 |
54 | Why We Need a Cleanup Function | 04:40 |
55 | Aborting Fetch Requests | 05:45 |
56 | useEffect Gotcha - Infinite Loops | 06:16 |
57 | Project Preview & Setup | 05:43 |
58 | Setting up & Shuffling Cards | 08:06 |
59 | Creating a Card Grid | 04:19 |
60 | CHALLENGE - Creating a Card Component | 04:11 |
61 | Making Card Choices | 05:57 |
62 | CHALLENGE - Comparing Choices | 07:15 |
63 | Adding a 'matched' Property to Cards | 05:58 |
64 | Flipping Cards | 08:58 |
65 | Flipping Animation (CSS) | 02:20 |
66 | Making Cards "disabled" | 05:04 |
67 | Finishing Touches | 03:52 |
68 | Multi-Page React Sites | 05:40 |
69 | React Router Setup | 06:59 |
70 | Switch & Exact Match | 06:03 |
71 | Links & Nav Links | 07:15 |
72 | Fetching Data | 07:25 |
73 | Route Parameters | 06:36 |
74 | The useParams Hook | 06:51 |
75 | Programmatic Redirects | 05:41 |
76 | Redirect Component | 02:29 |
77 | Query Parameters | 05:23 |
78 | Project Preview & Setup | 06:39 |
79 | Router & Pages Setup | 06:19 |
80 | Making a Navbar Component | 04:54 |
81 | Fetching Data | 04:41 |
82 | Recipe List Component | 09:46 |
83 | CHALLENGE - Fetching a Single Recipe | 05:18 |
84 | Recipe Details Template | 05:04 |
85 | Making a "Create Recipe" Form | 08:41 |
86 | Adding Multiple Ingredients | 12:35 |
87 | Making a POST Request | 12:34 |
88 | CHALLENGE - Redirecting the User | 03:43 |
89 | Making a Search Bar Component | 07:13 |
90 | Search Results Page | 08:17 |
91 | Finishing Touches | 03:22 |
92 | Prop Drilling | 02:36 |
93 | What is the Context API? | 02:55 |
94 | Creating a Context & Provider | 07:35 |
95 | Accessing Context Values | 03:40 |
96 | Creating a Custom Context Hook | 05:49 |
97 | Reducers & useReducer | 13:58 |
98 | Making a Color Theme Selector | 10:37 |
99 | Light & Dark Mode Selector | 12:23 |
100 | Styling Light & Dark Modes | 06:41 |
101 | What is Firebase? | 03:32 |
102 | Firestore Databases | 05:04 |
103 | Connecting to Firebase | 07:11 |
104 | Fetching a Firestore Collection | 11:48 |
105 | Fetching a Firestore Document | 09:38 |
106 | Adding Firestore Data | 05:53 |
107 | Deleting Firestore Data | 05:36 |
108 | Real-Time Collection Data | 07:48 |
109 | Updating Documents | 04:41 |
110 | Real-Time Document Data | 04:31 |
111 | Project Preview & Setup | 03:18 |
112 | CHALLENGE - Creating Pages & Routes | 05:17 |
113 | Creating a Navbar | 04:44 |
114 | Making the Login Form | 07:20 |
115 | CHALLENGE - Making the Signup Form | 04:55 |
116 | Firebase Setup | 03:49 |
117 | Firebase Authentication Setup | 04:03 |
118 | Creating a Signup Hook | 11:15 |
119 | Using the Signup Hook | 09:10 |
120 | Creating an Auth Context | 11:40 |
121 | Dispatching a Login Action | 06:08 |
122 | Creating a Logout Hook | 06:32 |
123 | Using the Logout Hook | 04:49 |
124 | Adding Cleanup Functions | 08:15 |
125 | Creating a Login Hook | 04:49 |
126 | Using the Login Hook | 03:58 |
127 | Conditionall Showing User Content | 08:07 |
128 | Firebase Auth State Changes | 09:29 |
129 | Waiting Until Auth is Ready | 04:23 |
130 | Route Guarding | 06:01 |
131 | Making a Transaction Form | 12:15 |
132 | Creating a useFirestore Hook | 13:03 |
133 | Adding Firestore Documents | 10:58 |
134 | Firestore Timestamps | 03:42 |
135 | Using the useFirestore Hook | 09:37 |
136 | Creating a useCollection Hook | 11:20 |
137 | Listing Transactions | 07:55 |
138 | Firestore Queries | 10:30 |
139 | Ordering Firestore Queries | 06:09 |
140 | Deleting Transactions | 08:36 |
141 | What Are Firestore Rules? | 04:57 |
142 | The Firebase CLI | 05:44 |
143 | Securing Collection Data | 06:16 |
144 | Deploying Firestore Rules | 03:48 |
145 | Building a React App | 02:11 |
146 | Deploying to Firebase | 03:10 |
147 | Updating the Site & Re-Deploying | 01:58 |
148 | Rolling Back Deployments | 01:22 |
149 | Project Preview & Setup | 06:26 |
150 | Firebase Setup | 06:42 |
151 | Firebase Init (Rules, Hosting & Storage) | 02:41 |
152 | Re-using Firebase Hooks & Auth Context | 05:54 |
153 | Router & Pages Setup | 06:54 |
154 | Navbar Component | 07:05 |
155 | Sidebar Component | 11:59 |
156 | Creating a Signup Page | 07:22 |
157 | Handling File Inputs | 13:47 |
158 | Firebase Storage Setup | 02:41 |
159 | Uploading Profile Images | 10:48 |
160 | Signing a User Up | 04:37 |
161 | Creating User Documents | 10:36 |
162 | Logging Users Out | 06:24 |
163 | Making a Login Page | 04:17 |
164 | CHALLENGE - Logging Users In | 05:07 |
165 | Redirects & Route Guards | 09:00 |
166 | CHALLENGE - Conditional Navbar Links | 03:45 |
167 | User Avatar Component | 07:07 |
168 | Fetching Users | 08:46 |
169 | Showing Users Online | 04:15 |
170 | Making the "Create Project" Form | 09:00 |
171 | Using React-Select | 05:32 |
172 | Assigning Users | 11:29 |
173 | Setting Form Errors | 07:28 |
174 | Creating a Project Object | 11:23 |
175 | CHALLENGE - Saving New Projects | 06:06 |
176 | Fetching Projects | 07:44 |
177 | Making a Project List / Grid | 09:39 |
178 | Making a useDocument Hook | 07:28 |
179 | Project Details Page (fetching a project) | 09:08 |
180 | Project Summary Component | 08:05 |
181 | Making a Comments Form | 10:13 |
182 | Updating Firestore Documents | 07:12 |
183 | Adding Comments | 04:47 |
184 | Listing Comments | 06:58 |
185 | Completing / Deleting Projects | 09:33 |
186 | Making a Filter (part 1) | 10:02 |
187 | Making a Filter (part 2) | 05:00 |
188 | Making a Filter (part 3) | 10:55 |
189 | Adding Firestore Rules | 11:21 |
190 | Final Touches | 03:48 |
191 | Deploying the App | 04:21 |
192 | Intro & Starter Project | 09:13 |
193 | Firebase Config File | 04:34 |
194 | Getting Documents | 08:16 |
195 | Real-Time Collection Data | 08:40 |
196 | Adding Data | 03:34 |
197 | Deleting Data | 03:20 |
198 | Setting Up Firebase Auth | 01:09 |
199 | Signing Users Up | 06:54 |
200 | Logging Users Out | 03:18 |
201 | Logging Users In | 04:08 |
202 | Adding Auth Context | 07:31 |
203 | Dispatching Actions | 06:15 |
204 | Route Guards & Redirects | 04:55 |
205 | Assigning Users to Books | 04:32 |
206 | Firestore Queries | 06:21 |
207 | Firebase Further Reading | 01:30 |
208 | Introduction to React Router 6 | 13:59 |
209 | The Route Component | 07:41 |
210 | Redirects & useNavigate | 07:54 |
211 | Nested Routes | 10:38 |
212 | Refactoring the Recipe Site | 09:35 |
213 | Refactoring the Project Management Site | 12:37 |
214 | Extra JavaScript Lessons | 00:38 |
215 | Destructuring | 07:01 |
216 | Import & Exports | 05:05 |
217 | Filter & Map Methods | 06:23 |
218 | Spread Syntax | 02:14 |
219 | Template Strings | 02:11 |
220 | Arrow Functions | 02:47 |
221 | Fetch API and Promises | 05:27 |
222 | Async & Await | 03:02 |
Similar courses to Build Web Apps with React & Firebase
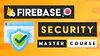
Firebase Security Coursefireship.io
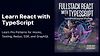
Fullstack React with Typescriptfullstack.io
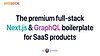
Bedrock: Jumpstart your next SaaS productMax Stoiber (@mxstbr)
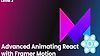
Advanced Animating React with Framer Motionleveluptutorials
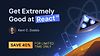
Learn React 19 with Epic React v2Kent C. Dodds
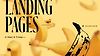
Build fancy landing pages with React and ThreejsPaul Henschel (@0xca0a)
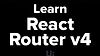
React Router v4ui.dev (ex. Tyler McGinnis)
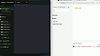
Add React Storybook to a Projectegghead
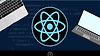
React Front To Back 2022udemyBrad Traversy
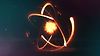