Understanding Node.js: Core Concepts
Welcome to the most comprehensive Node.js course on the internet! In this course, we're going to do a deep dive into Node.js itself without cluttering our minds with other tools and NPM packages and truly master this powerful technology.
This course is heavily focused on computer science topics and fundamentals that are crucial to understand for becoming a great back-end engineer. You can only properly understand Node.js and unlock its full power if you understand these other computer science topics. So that's why we will also learn these other vital topics so that you can truly master Node.js and take your back-end engineering skills to a whole new level.
We will also use all these vital concepts that we'll learn in practice by building various exciting projects just using Node.js.
This is an intense course for people who want to get to the top of the field and get to a level of driving innovation and making an impact within the industry instead of just scratching the surface and following a few software trends and tools.
Each section of the course is like its own mini-course, and by completing each section, you'll learn some essential Node.js, computer science and back-end engineering concepts that will help you not just if you want to use Node.js but throughout your whole career as a software engineer. These things will stay with you for years and decades to come.
Read more about the course
Let's do a quick walkthrough about what you will accomplish after completing some of the sections:
Understanding Buffers: Here, we will deeply understand buffers and how to work directly with binary data, which is essential for all the other sections.
Understanding File System: As a back-end developer, you'll work with files a lot, be it saving some data to disk, handling file uploads and many other examples, so it's essential to have a good understanding of them, which you'll gain after completing this section. We'll also learn how Node.js deals with files and master the "fs" module.
Understanding Streams: In this section, we're going to master Streams, which will allow us to develop highly-performant apps capable of handling terabytes of data with ease while having great memory usage. We'll build many mini-projects throughout the section, including an encryption-decryption app from scratch that could encrypt terabytes of data by directly modifying the binary data. This section lays the foundation for future sections where we'll utilize Streams heavily to create powerful and efficient network applications.
Understanding Networking: Node.js was primarily designed to create network applications, so it's of utmost importance that we gain a decent understanding of networking, which we will do after completing this section. Here's a list of items we'll learn in this section:
- What exactly a network is
- How the internet works
- Mac Addresses
- IPV4 & IPv6 Addresses
- TCP
- UDP
- DNS
- Fundamentals of deployment
We'll build 2 low-level apps using only Node.js, a chat and a file uploader app directly on top of TCP! And then, we'll deploy them to a Linux server in the most basic way without using unnecessary tools.
We'll see exactly what happens in our network card, every single 0s and 1s exchanged for a particular thing using Wireshark, and gain a much better understanding of networking and how most of the well-known protocols like HTTP, FTP, Email protocols, SSH, DNS and many others work. This section will broaden your horizons, and you'll realize that there are far more things that you can do with Node.js than just creating web servers.
Understanding HTTP: In this section, we'll utilize and combine all that we've learned from previous sections and finally deeply understand HTTP once and for all! We won't be learning how to use Express; instead, we will build something similar ourselves!
We'll start by understanding the most important HTTP concepts, such as connection types, client-server model, messages, requests, responses, HTTP Methods, status codes, mime types, necessary headers and so much more. And then emulate an HTTP protocol directly on top of TCP using the net module and see precisely every single 0s and 1s that get exchanged for an HTTP request and response interaction!
Once we understand the fundamentals of HTTP and the "http" module, we'll take things to the next level and start building our framework. And then, using our framework, we'll make a fully functional web application.
This section will take your web development skills to the next level. You'll have a much better understanding of how all these popular NPM packages, like Express, body-parser, Multer, cors, etc., that are built on top of the "http" module work. Well, you'll learn how to make them from scratch, just using them will not be that much of a problem!
Watch Online Understanding Node.js: Core Concepts
# | Title | Duration |
---|---|---|
1 | Course Overview | 29:44 |
2 | Setting Up - For Windows Users Only | 49:41 |
3 | Setting Up - For Mac Users Only | 59:57 |
4 | Setting Up - For Linux Users Only | 53:06 |
5 | Introduction to the Command Line | 40:47 |
6 | Learning NVM & Node.js Versions | 15:24 |
7 | Node.js Under the Hood | 01:36:02 |
8 | Understanding EventEmitter | 34:06 |
9 | Introdcution | 06:48 |
10 | Understanding Binary Numbers | 13:32 |
11 | Understanding Hexadecimal Numbers | 21:10 |
12 | Using a Programming Calculator | 11:49 |
13 | Understanding Character Encodings | 25:21 |
14 | The Concept of Buffers | 07:35 |
15 | Buffers in Action | 38:47 |
16 | Allocating Huge Buffers | 18:15 |
17 | Fastest Way of Allocating Buffers | 20:59 |
18 | Reading the Node.js Docs | 14:02 |
19 | Outro | 02:09 |
20 | Introduction | 11:19 |
21 | What Exactly Is a File? | 07:33 |
22 | How Node.js Deals with Files | 04:07 |
23 | Three Different Ways of Doing the Same Thing | 14:43 |
24 | Watching the Command File for Changes | 13:33 |
25 | Reading the Content of the Command File | 18:41 |
26 | Cleaning Up the Code using EventEmitter | 04:23 |
27 | Making Sense Out of the Read Data | 03:54 |
28 | Implementing the Create File Command | 15:37 |
29 | Defining the Rest of the Commands | 15:22 |
30 | Solution - Implementing the deleteFile Function | 11:08 |
31 | Solution - Implementing the renameFile Function | 03:39 |
32 | Solution - Implementing the addToFile Function | 11:08 |
33 | Outro | 01:08 |
34 | Introduction | 04:52 |
35 | Benchmarking Writing a Million Times to a File | 26:26 |
36 | Using Streams Naively in Our Solution | 07:58 |
37 | So What Exactly Are Streams? | 08:59 |
38 | Understanding Different Types of Streams | 17:53 |
39 | Fixing the Memory Issue in Our Program | 36:55 |
40 | Reading Writable Streams Node.js Docs and Recap | 09:49 |
41 | Readable Streams in Action | 21:36 |
42 | Selectively Writing Our Data from the Readable Stream | 09:18 |
43 | Understanding the Splitting Issue | 16:57 |
44 | Implementing the Solution and Resolving the Splitting Issue | 16:28 |
45 | Reading Node.js Docs and Recap | 08:04 |
46 | Building Our Own Streaming Solution Using Buffers | 23:31 |
47 | Understanding Piping | 25:43 |
48 | Implementing Our Own Writable Stream | 42:29 |
49 | Implementing Our Own Readable Stream | 20:03 |
50 | Understanding Duplex and Transform Streams | 17:27 |
51 | Creating an Encryption/Decryption Application | 39:04 |
52 | Final Notes | 26:05 |
53 | Introduction | 12:00 |
54 | Understanding MAC Addresses and Switches | 15:03 |
55 | Understanding Routers and How the Internet Works | 15:17 |
56 | Understanding Networking Layers | 16:30 |
57 | Creating a Simple TCP Application in Node.js | 24:44 |
58 | Understanding the Transport Layer - TCP/UDP | 13:03 |
59 | A Networking Scenario in Action | 25:20 |
60 | Understanding Port Numbers | 12:03 |
61 | Creating a Chat Application PART 1 | 29:47 |
62 | Creating a Chat Application PART 2 | 34:19 |
63 | Improving the UI of our Chat App | 21:35 |
64 | Identifying Users in the Chat App | 18:47 |
65 | Notifying Everyone When Somebody Joins/Leaves the Chat | 04:10 |
66 | Some Final Notes About the Chat App | 15:27 |
67 | Deploying our Chat App to AWS | 34:32 |
68 | Understanding IPv4 Addresses | 40:10 |
69 | Understanding DNS | 31:37 |
70 | Understanding IPv6 Addresses | 26:45 |
71 | Creating an Uploader Application | 26:03 |
72 | Taking Care of Backpressures in the Uploader App | 33:16 |
73 | Getting the File Names Dynamically in the Uploader App | 20:44 |
74 | Displaying the Upload Progress in Our Uploader App | 16:03 |
75 | Deploying our Uploader App | 20:06 |
76 | Understanding UDP and the Dgram Module | 32:38 |
77 | Outro | 01:31 |
78 | Introduction | 10:39 |
79 | The Idea of HTTP | 07:01 |
80 | Creating a Simple HTTP Server in Node | 15:10 |
81 | Understanding HTTP Messages, Requests & Responses | 12:52 |
82 | Understanding Connection Types | 16:14 |
83 | Building our Client and Sending a Request | 21:11 |
84 | Sending a Response to the Client | 12:44 |
85 | Learning Postman & Testing Our Server with It | 25:36 |
86 | Reading Some Docs | 28:35 |
87 | Sending an HTTP Request Directly on Top of TCP! PART 1 | 20:06 |
88 | Sending an HTTP Request Directly on Top of TCP! PART 2 | 22:06 |
89 | Emulating our HTTP Server Using the Net Module | 11:20 |
90 | Understanding Media Types (MIME Types) | 16:52 |
91 | Understanding HTTP Methods | 21:37 |
92 | Understanding HTTP status codes | 15:05 |
93 | Creating a Simple Web Server in Node | 18:23 |
94 | Serving a CSS and JavaScript File | 22:06 |
95 | Adding Some JSON Routes | 07:55 |
96 | Adding a File Upload Route | 13:02 |
97 | Creating our Own Little Mini-Express Framework! | 23:19 |
98 | Making our Framework More Robust | 15:05 |
99 | Getting Ready for Our Next Project | 11:26 |
100 | Using Our Framework to Create a Web App | 16:16 |
101 | Adding the Login Route | 21:16 |
102 | Understanding HTTP Proxies | 18:20 |
103 | HTTP is Stateless | 12:58 |
104 | Understanding Cookies | 13:08 |
105 | Recognizing Users for Future Requests after Logging In | 18:16 |
106 | Sending the User’s Info in Profile | 09:15 |
107 | Enhancing Our Framework with Middleware Support | 23:05 |
108 | Defining the Middleware Functions | 18:09 |
109 | Implementing the Create Post, Update User, and Logout Routes | 25:03 |
110 | Outro | 02:54 |
Similar courses to Understanding Node.js: Core Concepts
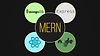
React, NodeJS, Express & MongoDB - The MERN Fullstack Guideudemy
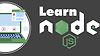
Learn Nodewesbos
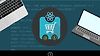
MERN eCommerce From ScratchudemyBrad Traversy
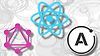
Full-Stack React with GraphQL and Apollo Boostudemy
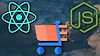
Build a Shopping Cart AppReed Barger
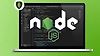
Node.js, Express, MongoDB Bootcamp 2020 - with Real Projectsudemy
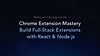
Chrome Extension Mastery: Build Full-Stack Extensions with React & Node.jsRyan Fitzgerald
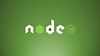
Learn and Understand NodeJSudemy
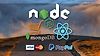