The Modern React 18 Bootcamp - A Complete Developer Guide
20h 35m 57s
English
Paid
In this course we will take you from a React 18 novice to a job ready engineer. This course is loaded with practical projects and examples so that you can truly understand and utilize React 18 in great depth!
We will be building five projects, each one getting more and more complex. We will end this course by building an Netflix clone with features like payments and user authentication. By the end of this course, you should have multiple practical example to show off your knowledge!
Read more about the course
Here are a list of thing you will learn in this course:
- The difference between pure Vanilla JS and React 18
- How to utilize the all the different React hooks
- Conditional rendering and mapping through a list of elements
- Fetching data from an external API and handling the success, loading and error states
- Handling user authentication
- Building a Postgres database to handle complex relations
- Utilizing TypeScript for bug free code
- All the important ways to handle state (useState, useContext, useReducer, Redux Toolkit)
- Handling subscriptions payments with Stripe
- Scroll based pagination with the Observer Intersection API
- Optimizing performance by preventing unnecessary re-renders
Watch Online The Modern React 18 Bootcamp - A Complete Developer Guide
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | A Little Setup | 02:56 |
2 | What is React | 04:55 |
3 | Building an App With Vanilla JS | 14:16 |
4 | Discussing the Annoyances We Faced | 02:19 |
5 | Building the Same App With ReactJS | 18:29 |
6 | Creating a React App | 12:32 |
7 | Exploring the Files and Folders | 09:52 |
8 | State and Event Handling | 08:51 |
9 | Project Intro | 02:59 |
10 | Class vs ClassName | 07:40 |
11 | Building the JSX Structure | 07:53 |
12 | Listing Out Everything We Need to Work On | 02:42 |
13 | Two Way Binding | 10:26 |
14 | Defining an Array State | 11:52 |
15 | Iterating Through the List to Render Elements | 05:26 |
16 | The Key Prop | 04:22 |
17 | Computing the Quantity | 05:14 |
18 | Conditionally Render JSX Elements | 04:09 |
19 | Deleting an Item | 06:24 |
20 | Two Way Binding a List Item | 07:38 |
21 | The useEffect Hook | 10:10 |
22 | Project Introduction | 07:28 |
23 | Building the JSX Structure | 10:07 |
24 | Two Way Binding | 08:24 |
25 | A Cleaner and DRYer Way of Two Way Binding | 07:13 |
26 | Input Validation and Conditional Styling | 08:21 |
27 | Storing Store List and Map Through List to Render Elements | 12:38 |
28 | Installing Dependencies | 07:14 |
29 | Computing a New State | 07:29 |
30 | Project Introduction | 06:58 |
31 | Building the JSX Structure for the Home Page | 12:55 |
32 | Dividing Our JSX into Components | 10:32 |
33 | Passing Props to Components | 10:51 |
34 | Understanding Routing | 09:46 |
35 | How React Renders Content to the Browser (CSR vs SSR) | 12:07 |
36 | Installing react-router-dom | 05:05 |
37 | Defining Our Routing Rules | 05:38 |
38 | Adding Another Page to Our App | 03:23 |
39 | Nested Routes for Common Elements | 08:27 |
40 | Link Based Navigation | 07:14 |
41 | Defining Dynamic Routes | 05:32 |
42 | The useParams Hook for Extracting the Params | 08:00 |
43 | The useNavigate Hook for Programmatic Navigation | 05:56 |
44 | Where Will the Data Come From | 07:01 |
45 | Fetching Data From an API With Our React App | 06:22 |
46 | Iterating Through the Fetched Data | 08:08 |
47 | Encapsulating the Fetch Logic in a Custom Hook | 05:33 |
48 | Handling a Loading State | 07:07 |
49 | Handling an Error State | 04:22 |
50 | Querying for Data by Keyword | 12:01 |
51 | A Quick Fix | 02:34 |
52 | Another Custom Hook | 07:41 |
53 | Traversing and Rendering the Data | 13:26 |
54 | The Children Prop | 06:42 |
55 | Building the Recipe Info Component | 08:12 |
56 | Potentials Issues With the State We Defined | 03:34 |
57 | The useReducer Hook | 14:19 |
58 | Adding Query Params | 09:47 |
59 | Handling Error State with an Error Message | 06:16 |
60 | A 404 Error Page | 05:24 |
61 | More Nested Pages | 06:28 |
62 | Passing Data to an Outlet With useOutletContext | 07:03 |
63 | Wrapping Things Up | 03:55 |
64 | Deploying the Application | 07:07 |
65 | Viewing Our Deployment | 01:30 |
66 | React Has Many Hooks | 03:23 |
67 | The useRef Hook | 01:45 |
68 | Building a Mini Project With useState | 09:45 |
69 | Improving Performance With useRef | 07:55 |
70 | Referencing Elements with useRef | 08:42 |
71 | The useMemo Hook | 13:14 |
72 | Memo and Props | 07:43 |
73 | The useCallback Hook | 15:01 |
74 | An Intro to TypeScript | 03:48 |
75 | Creating a TypeScript React App | 04:32 |
76 | A Quick TypeScript Lesson | 16:09 |
77 | Exploring the Starter Code | 05:23 |
78 | State with TypeScript | 07:03 |
79 | Props with TypeScript | 07:34 |
80 | Params with TypeScript | 15:41 |
81 | Installing Packages with TypeScript | 02:51 |
82 | Major Project Introduction | 08:45 |
83 | Creating a TypeScript React App | 02:31 |
84 | Tailwind Integration | 08:08 |
85 | Building the NavBar | 12:00 |
86 | Building the Home Page | 11:45 |
87 | Building the Login Page | 09:39 |
88 | Building the Plans Page | 14:57 |
89 | Building the Browse Page | 24:23 |
90 | Building the Watch Page | 07:50 |
91 | Implementing Page Navigation | 09:16 |
92 | How Will We Get the Data? | 06:46 |
93 | Building an Express Server | 09:21 |
94 | Create a Movies List GET Endpoint | 03:21 |
95 | useReducer with TypeScript | 15:47 |
96 | Fetching the Data From the Hook | 08:51 |
97 | Consuming the Data in Our Browse Page | 06:27 |
98 | Fixing the Card Styles | 01:09 |
99 | Conditionally Adding a NavBar Background | 04:21 |
100 | Navigating to the Watch Page | 02:41 |
101 | Creating a Movie GET Endpoint | 02:57 |
102 | Fetching and Rendering the Data From React | 08:53 |
103 | Introduction to Pagination | 03:49 |
104 | Types of Pagination | 05:17 |
105 | Offsets and Limits | 04:35 |
106 | Returning Paginated Data From the Server | 03:05 |
107 | Intersection Obersever API to Detecting the Visibility of an Element | 13:13 |
108 | Appending the Data Instead of Replacing | 06:14 |
109 | Adding a Loading State | 07:28 |
110 | Prevent Refetching When Client Has All the Data | 08:14 |
111 | Introduction to Database Solutions | 05:54 |
112 | Spinning Up a Postgres Database | 04:37 |
113 | Raw SQL vs ORM | 06:12 |
114 | Defining Our Schema & Connecting to the DB | 11:18 |
115 | Writing and Executing a Seed Script | 10:16 |
116 | Refactoring Our Endpoints to Fetch Data With Prisma | 09:12 |
117 | Introduction to Authentication | 04:52 |
118 | Refactoring Common Endpoints to Separate Files | 07:32 |
119 | Validating the User Inputs | 14:46 |
120 | Validating That the User Doesn't Already Exist | 04:13 |
121 | Three Different Ways to Store a Password | 06:21 |
122 | Hashing and Saving the Password | 05:39 |
123 | Saving the User | 02:34 |
124 | Forming and Returning a JSON Web Token | 08:32 |
125 | The Sign In Endpoint | 09:39 |
126 | The Me Endpoint | 09:04 |
127 | Back to the Client | 01:33 |
128 | Fixing the Form Card | 06:43 |
129 | The React Hook Form Library | 02:13 |
130 | Setting Up the Form With the useForm Hook | 07:06 |
131 | Managing State and Two Way Binding | 08:14 |
132 | Validating the Form Input Values | 09:24 |
133 | Handling Error States | 06:14 |
134 | Bring Things Together | 02:32 |
135 | Creating a useAuth Hook | 06:08 |
136 | Making the HTTP Requests | 07:06 |
137 | Handling Auth Errors | 08:55 |
138 | Navigating to the Browse Page Upon Successful Authentication | 03:36 |
139 | Storing the JWT in the Browser's Cookie | 05:42 |
140 | Introduction to Redux Toolkit | 12:38 |
141 | Defining Our Global State | 06:24 |
142 | Persisting the Authentication State | 10:48 |
143 | Defining Private Routes | 07:14 |
144 | Adding the Logout Logic | 04:44 |
145 | Time to Make Some Money | 02:53 |
146 | Adding Products to Stripe | 02:56 |
147 | Connecting Our Server to Stripe | 08:08 |
148 | Defining the Products Endpoint | 05:04 |
149 | The usePlans Hook | 12:38 |
150 | Rendering the Plans | 12:22 |
151 | Defining a Session Endpoint | 11:34 |
152 | Purchasing a Subscription From the Client | 12:34 |
153 | Walking Through All User Flows | 04:37 |
154 | Show the Plans Page Only if You Are Authenticated | 01:36 |
155 | A Check Auth Middleware | 11:49 |
156 | Creating a My Subscription Endpoint | 08:26 |
157 | Redirect Users that Have Plans to the Manage Plans Page | 14:16 |
158 | Manage Page Redirection | 04:47 |
159 | Add Permission Logic to the Movie List Endpoint | 11:05 |
160 | Redirect User to the Plan Page if They Don't Have a Subscription | 04:34 |
161 | Premium Plan Permissions | 09:36 |
Similar courses to The Modern React 18 Bootcamp - A Complete Developer Guide
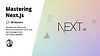
Mastering Next.js - 50+ Lesson Video Course on React and Nextmasteringnuxt.com
Category: React.js, Next.js
Duration 5 hours 9 minutes 45 seconds
Course
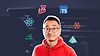
Mastering Maintainable Reactudemy
Category: React.js
Duration 7 hours 8 minutes 52 seconds
Course
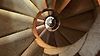
Testing React with Jest and Testing Libraryudemy
Category: React.js
Duration 7 hours 41 minutes 32 seconds
Course
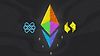
NFT Marketplace in React, Typescript & Solidity - Full Guideudemy
Category: TypeScript, React.js, Decentralized Applications (dApps) / 'Web 3'
Duration 16 hours 20 minutes 55 seconds
Course
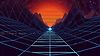
MERN STACK 2022 - Build Ultimate CMS (WordPress Clone)udemy
Category: React.js, Node.js, MongoDB
Duration 34 hours 4 minutes 17 seconds
Course
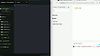
Add React Storybook to a Projectegghead
Category: React.js
Duration 3 minutes 36 seconds
Course
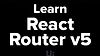
React Router v5ui.dev (ex. Tyler McGinnis)
Category: React.js
Duration 3 hours 38 minutes 49 seconds
Course
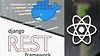
React and Django: A Practical Guide with Dockerudemy
Category: React.js, Docker, Django
Duration 6 hours 50 minutes 20 seconds
Course
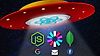
MERN Stack Web Development with Ultimate Authenticationudemy
Category: React.js, Node.js, MongoDB
Duration 9 hours 24 minutes 59 seconds
Course