The Complete Python Programming Course for Beginners
Python is the most popular programming language in the world. It's used by big companies like Google, Facebook, Dropbox, Reddit, Spotify, Quora, etc. Mathematicians, scientists, engineers and developers love it because of its simple and elegant syntax. It's the #1 language for AI and machine learning, and the ideal language to learn for beginners. Much easier than C++ or JavaScript! This course teaches you everything Python has to offer from the basics to more advanced topics.
Read more about the course
A perfect mix of theory and practice, packed with real-world examples, exercises and step-by-step solutions - free of "fluff" and lengthy description!
By the end of this course…
You’ll be able to:
- Write Python code with confidence
- Get ready to learn web development and machine learning with Python
- Build command-line utilities
- Automate boring, repetitive tasks
- Write clean code like a pro
What You'l Learn...
Along with all the programming fundamentals, you’ll learn how to...
- Use essential Python data structures
- Use classes and modules
- Apply object-oriented programming principles
- Work with exceptions
- Build web scraping tools
- Use 3rd-party Python packages and publish your own
- Work with files and directories
- Work with CSV, JSON, Excel spreadsheets, PDFs, ZIP files, etc
- Send emails and text messages
- Automate UI testing with Selenium
- Call backend APIs
- Basics of building web apps with Python and Django
- Use Python in machine learning and data science projects
- And much, much more!
Watch Online The Complete Python Programming Course for Beginners
# | Title | Duration |
---|---|---|
1 | 1- What is Python | 03:22 |
2 | 2- Installing Python | 01:53 |
3 | 3- Python Interpreter | 01:56 |
4 | 4- Code Editors | 01:20 |
5 | 5- Your First Python Program | 03:37 |
6 | 6- Python Extension | 02:53 |
7 | 7- Linting Python Code | 04:15 |
8 | 8- Formatting Python Code | 03:55 |
9 | 9- Running Python Code | 03:00 |
10 | 10- Python Implementations | 02:29 |
11 | 11- How Python Code is Executed | 02:47 |
12 | 12- Quiz | 01:38 |
13 | 1- Variables | 03:05 |
14 | 2- Variable Names | 03:04 |
15 | 3- Strings | 05:31 |
16 | 4- Escape Sequences | 02:41 |
17 | 5- Formatted Strings | 02:09 |
18 | 6- String Methods | 05:55 |
19 | 7- Numbers | 02:47 |
20 | 8- Working with Numbers | 02:10 |
21 | 9- Type Conversion | 05:05 |
22 | 10- Quiz | 02:44 |
23 | 1- Comparison Operators | 02:05 |
24 | 2- Conditional Statements | 04:10 |
25 | 3- Ternary Operator | 02:10 |
26 | 4- Logical Operators | 04:03 |
27 | 5- Short-circuit Evaluation | 02:07 |
28 | 6- Chaining Comparison Operators | 01:23 |
29 | 7- Quiz | 01:44 |
30 | 8- For Loops | 03:39 |
31 | 9- For..Else | 02:47 |
32 | 10- Nested Loops | 02:45 |
33 | 11- Iterables | 03:09 |
34 | 12- While Loops | 05:00 |
35 | 13- Infinite Loops | 01:38 |
36 | 14- Exercise | 02:06 |
37 | 1- Defining Functions | 02:25 |
38 | 2- Arguments | 02:21 |
39 | 3- Types of Functions | 04:03 |
40 | 4- Keyword Arguments | 02:01 |
41 | 5- Default Arguments | 01:36 |
42 | 6- xargs | 04:16 |
43 | 7- xxargs | 02:21 |
44 | 8- Scope | 05:10 |
45 | 9- Debugging | 06:51 |
46 | 10- VSCode Coding Tricks - Windows | 02:22 |
47 | 11- VSCode Coding Tricks - Mac | 01:50 |
48 | 12- Exercise | 01:30 |
49 | 13- Solution | 04:42 |
50 | 1- Lists | 03:55 |
51 | 2- Accessing Items | 03:14 |
52 | 3- List Unpacking | 03:52 |
53 | 4- Looping over Lists | 02:55 |
54 | 5- Adding or Removing Items | 02:57 |
55 | 6- Finding Items | 01:29 |
56 | 7- Sorting Lists | 04:36 |
57 | 8- Lambda Functions | 01:50 |
58 | 9- Map Function | 03:26 |
59 | 10- Filter Function | 02:06 |
60 | 11- List Comprehensions | 03:11 |
61 | 12- Zip Function | 01:50 |
62 | 13- Stacks | 04:25 |
63 | 14- Queues | 02:51 |
64 | 15- Tuples | 04:03 |
65 | 16- Swapping Variables | 02:38 |
66 | 17- Arrays | 03:12 |
67 | 18- Sets | 04:04 |
68 | 19- Dictionaries | 05:25 |
69 | 20- Dictionary Comprehensions | 03:20 |
70 | 21- Generator Expressions | 03:52 |
71 | 22- Unpacking Operator | 04:06 |
72 | 23- Exercise | 06:22 |
73 | 1- Exceptions | 02:17 |
74 | 2- Handling Exceptions | 04:11 |
75 | 3- Handling Different Exceptions | 03:06 |
76 | 4- Cleaning Up | 01:58 |
77 | 5- The With Statement | 03:08 |
78 | 6- Raising Exceptions | 03:22 |
79 | 7- Cost of Raising Exceptions | 04:42 |
80 | 1- Classes | 02:36 |
81 | 2- Creating Classes | 03:46 |
82 | 3- Constructors | 04:38 |
83 | 4- Class vs Instance Attributes | 03:59 |
84 | 5- Class vs Instance Methods | 04:06 |
85 | 6- Magic Methods | 03:14 |
86 | 7- Comparing Objects | 03:12 |
87 | 8- Performing Arithmetic Operations | 01:32 |
88 | 9- Making Custom Containers | 06:56 |
89 | 10- Private Members | 03:41 |
90 | 11- Properties | 07:31 |
91 | 12- Inheritance | 04:24 |
92 | 13- The Object Class | 02:24 |
93 | 14- Method Overriding | 03:15 |
94 | 15- Multi-level Inheritance | 02:43 |
95 | 16- Multiple Inheritance | 03:23 |
96 | 17- A Good Example of Inheritance | 04:32 |
97 | 18- Abstract Base Classes | 04:51 |
98 | 19- Polymorphism | 03:57 |
99 | 20- Duck Typing | 02:51 |
100 | 21- Extending Built-in Types | 02:27 |
101 | 22- Data Classes | 04:37 |
102 | 1- Creating Modules | 04:17 |
103 | 2- Compiled Python Files | 02:20 |
104 | 3- Module Search Path | 01:36 |
105 | 4- Packages | 02:28 |
106 | 5- Sub-packages | 01:02 |
107 | 6- Intra-package References | 01:37 |
108 | 7- The dir Function | 01:40 |
109 | 8- Executing Modules as Scripts | 02:56 |
110 | 1- Python Standard Library | 00:52 |
111 | 2- Working With Paths | 04:49 |
112 | 3- Working with Directories | 04:15 |
113 | 4- Working with Files | 04:00 |
114 | 5- Working with Zip Files | 03:16 |
115 | 6- Working with CSV Files | 04:51 |
116 | 7- Working with JSON Files | 03:59 |
117 | 8- Working with a SQLite Database | 09:11 |
118 | 9- Working with Timestamps | 02:25 |
119 | 10- Working with DateTimes | 05:06 |
120 | 11- Working with Time Deltas | 02:42 |
121 | 12- Generating Random Values | 04:10 |
122 | 13- Opening the Browser | 01:13 |
123 | 14- Sending Emails | 06:49 |
124 | 15- Templates | 04:54 |
125 | 16- Command-line Arguments | 01:55 |
126 | 17- Running External Programs | 08:07 |
127 | 1- Pypi | 01:50 |
128 | 2- Pip | 06:24 |
129 | 3- Virtual Environments | 04:05 |
130 | 4- Pipenv | 03:41 |
131 | 5- Virtual Environments in VSCode | 03:50 |
132 | 6- Pipfile | 04:49 |
133 | 7- Managing Dependencies | 03:29 |
134 | 8- Publishing Packages | 08:24 |
135 | 9- Docstrings | 05:49 |
136 | 10- Pydoc | 04:07 |
137 | 1- Introduction | 01:42 |
138 | 2- What are APIs | 02:37 |
139 | 3- Yelp API | 02:52 |
140 | 4- Searching for Businesses | 09:55 |
141 | 5- Hiding API Key | 02:06 |
142 | 6- Sending Text Messages | 06:03 |
143 | 7- Web Scraping | 09:07 |
144 | 8- Browser Automation | 11:29 |
145 | 9- Working with PDFs | 06:19 |
146 | 10- Working with Excel Spreadsheets | 09:53 |
147 | 11- Command Query Separation Principle | 04:40 |
148 | 12- NumPy | 09:07 |
149 | 1- Introduction | 01:44 |
150 | 2- Your First Django Project | 04:12 |
151 | 3- Your First App | 03:42 |
152 | 4- Views | 08:00 |
153 | 5- Models | 04:58 |
154 | 6- Migrations | 08:01 |
155 | 7- Changing the Models | 05:39 |
156 | 8- Admin | 04:30 |
157 | 9- Customizing the Admin | 06:56 |
158 | 10- Database Abstraction API | 03:53 |
159 | 11- Templates | 10:24 |
160 | 12- Adding Bootstrap | 04:20 |
161 | 13- Customizing the Layout | 02:25 |
162 | 14- Sharing a Template Across Multiple Apps | 03:49 |
163 | 15- Url Parameters | 04:38 |
164 | 16- Getting a Single Object | 03:49 |
165 | 17- Raising 404 Errors | 03:52 |
166 | 18- Referencing Urls | 03:48 |
167 | 19- Creating APIs | 09:27 |
168 | 20- Adding the Homepage | 04:28 |
169 | 21- Getting Ready to Deploy | 09:45 |
170 | 22- Deployment | 08:00 |
171 | 1- What is Machine Learning | 01:59 |
172 | 2- Machine Learning in Action | 02:48 |
173 | 3- Libraries and Tools | 04:55 |
174 | 4- Importing a Data Set | 06:22 |
175 | 5- Jupyter Shortcuts | 05:27 |
176 | 6- A Real Machine Learning Problem | 03:18 |
177 | 7- Preparing the Data | 03:06 |
178 | 8- Learning and Predicting | 04:05 |
179 | 9- Calculating the Accuracy | 06:22 |
180 | 10- Persisting Models | 03:15 |
181 | 11- Visualizing a Decision Tree | 06:27 |
Similar courses to The Complete Python Programming Course for Beginners
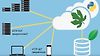
Eve: Building RESTful APIs with MongoDB and FlaskTalkpython
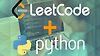
Python & LeetCode | The Ultimate Interview BootCampkaeducation.com
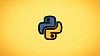
Python on the Backendudemy
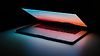
2022 Python for Machine Learning & Data Science Masterclassudemy
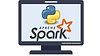
Spark and Python for Big Data with PySparkudemy
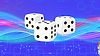
Statistics Bootcamp (with Python): Zero to Masteryzerotomastery.io
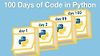
#100DaysOfCode with Python courseTalkpython
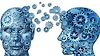
Machine Learning A-Z : Become Kaggle Masterudemy
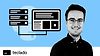
Web Developer Bootcamp with Flask and Python in 2022udemy
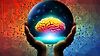