React & TypeScript - The Practical Guide
TypeScript is an amazing technology that helps developers write better code with less errors - simply because it let's you catch & fix type-related errors whilst writing the code (instead of when testing the application).
But using TypeScript with React can sometimes be tricky. Especially when building more complex, dynamic components, defining the right types can be challenging.
That's why I built this course!
Read more about the course
Because this course will teach you how to use TypeScript with React - and, of course, the course will introduce you to all the core concepts & patterns you need to work with components, state, side effects & more in a type-safe way!
This course will:
- Teach you WHY using TypeScript in your React projects might be a good idea
- Introduce you to the key TypeScript concepts you'll need - when working with React & in general
- Get you started with using TypeScript with React - for components, state & more
- Explore more advanced, complex patterns & examples
- Help you with building dynamic or even polymorphic components in a type-safe way
- Teach you how to use TypeScript with React's Context API
- Explore how you can enhance code used with useReducer() with help of TypeScript
- Cover data fetching & useEffect() with TypeScript
- Use the popular Redux library in a type-safe way
- Build or improve multiple demo projects so that can apply your knowledge
By the end of the course, you'll be able to use TypeScript in your own (future) React projects and write better, more type-safe code.
Watch Online React & TypeScript - The Practical Guide
# | Title | Duration |
---|---|---|
1 | Welcome To The Course! | 01:27 |
2 | Why React & TypeScript? | 01:57 |
3 | About The Course & Course Content | 01:47 |
4 | How To Get The Most Out Of The Course | 03:00 |
5 | Creating & Using React + TypeScript Projects | 01:01 |
6 | Module Introduction | 01:14 |
7 | TypeScript Setup & Using TypeScript | 03:02 |
8 | Working with Types: Type Inference & Explicit Type Annotations | 07:47 |
9 | Basic Primitive Types | 01:08 |
10 | Invoking The TypeScript Compiler | 03:14 |
11 | Combining Types Union Types (Alternative Types) | 02:22 |
12 | Working with Object Types | 05:40 |
13 | Working with Array Types | 04:00 |
14 | Adding Types to Functions - Parameter & Return Value Types | 05:05 |
15 | Defining Function Types | 03:29 |
16 | Creating Custom Types / Type Aliases | 03:58 |
17 | Defining Object Types with Interfaces | 03:07 |
18 | Interfaces vs Custom Types | 04:25 |
19 | Being Specific With Literal Types | 04:22 |
20 | Merging Types | 03:06 |
21 | Adding Type Guards | 03:43 |
22 | Making Sense Of Generic Types | 12:21 |
23 | Summary | 00:36 |
24 | Module Introduction | 01:14 |
25 | Creating a React + TypeScript Project | 06:32 |
26 | Understanding the Role of tsconfig.json | 05:05 |
27 | Building a First Component & Facing a Missing Type | 05:48 |
28 | Defining Component Props Types | 04:06 |
29 | Storing Props Types as a Custom Type or Interface | 02:05 |
30 | Defining a Type for Props with "children" | 07:03 |
31 | Another Way Of Typing Components | 03:19 |
32 | Exercise: Creating a Header Component | 06:12 |
33 | Using useState() and TypeScript | 06:40 |
34 | Working with State & Outputting State-based Values | 05:50 |
35 | Another Exercise & Reusing Types Across Files | 07:32 |
36 | Passing Functions as Values - In A Type-Safe Way | 10:08 |
37 | Handling & Typing Events | 08:01 |
38 | Working with Generic Event Types | 04:25 |
39 | Using useRef() with TypeScript | 08:51 |
40 | Handling User Input In A Type-Safe Way | 05:09 |
41 | Summary | 01:43 |
42 | Module Introduction | 01:32 |
43 | Building a More Dynamic & Flexible Component | 11:09 |
44 | Problem: Flexible Components With Required Prop Combinations | 06:20 |
45 | Solution: Building Components with Discriminated Unions | 06:07 |
46 | Onwards To A New Project | 01:15 |
47 | Building a Basic Wrapper Component | 04:57 |
48 | Building Better Wrapper Components with ComponentPropsWithoutRef | 07:26 |
49 | Building a Wrapper Component That Renders Different Elements | 07:06 |
50 | Working with Type Predicates & Facing TypeScript Limitations | 10:52 |
51 | Building a Basic Polymorphic Component | 05:27 |
52 | Building a Better Polymorphic Component with Generics | 10:07 |
53 | Using forwardRef with TypeScript | 07:33 |
54 | Building Another Wrapper Component (Custom Form Component) | 03:46 |
55 | Sharing Logic with "unknown" & Type Casting | 10:11 |
56 | Exposing Component APIs with useImperativeHandle (with TypeScript) | 09:59 |
57 | Summary | 00:58 |
58 | The Starting Project | 01:40 |
59 | Module Introduction | 01:00 |
60 | Creating a Context & Fitting Types | 07:08 |
61 | Creating a Type-Safe Provider Component | 04:41 |
62 | Accessing Context Type-Safe With A Custom Hook | 06:34 |
63 | Getting Started with useReducer() & TypeScript | 03:16 |
64 | A Basic Reducer Function & A Basic Action Type | 06:29 |
65 | Changing State via the Reducer Function | 02:44 |
66 | Using Better Action Types | 05:48 |
67 | Wiring Everything Up & Finishing the App | 07:27 |
68 | Module Introduction | 00:47 |
69 | Creating a First Side Effect | 04:46 |
70 | Using useEffect() with TypeScript | 04:32 |
71 | Managing An Interval With Refs & The Effect Cleanup Function | 08:54 |
72 | useEffect() & Its Dependencies | 05:46 |
73 | Onwards to Data Fetching! | 02:11 |
74 | Building a Utility "get" Function with TypeScript | 07:01 |
75 | Fetching & Transforming Data | 13:28 |
76 | Handling Loading & Error States | 08:15 |
77 | The Starting Project | 02:59 |
78 | Module Introduction | 01:05 |
79 | Redux Setup | 01:19 |
80 | Creating a Redux Store & A First Slice | 05:18 |
81 | Setting a State Type | 02:41 |
82 | A First Reducer & Controlling the Action Payload Type | 03:29 |
83 | Adding Logic To The Reducer | 06:18 |
84 | Providing the Redux Store | 02:35 |
85 | Dispatching Actions & Adjusting the useDispatch Hook | 08:26 |
86 | Creating a Type-Safe useSelector Hook | 05:16 |
87 | Selecting & Transforming Redux Store Data | 03:37 |
88 | Finishing Touches & Summary | 09:01 |
89 | Your Task | 02:04 |
Similar courses to React & TypeScript - The Practical Guide
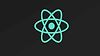
Starting with React & Redux: Build modern apps (2nd edition)udemy
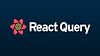
React Query - Essentials (v2)Tanner Linsley
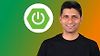
Master Spring Boot 3 & Spring Framework 6 with Javaudemy
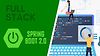
Full Stack Spring Boot & Reactamigoscode (Nelson Djalo)
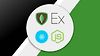
MERN 2024 Edition - MongoDB, Express, React and NodeJSudemy
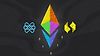
NFT Marketplace in React, Typescript & Solidity - Full Guideudemy
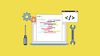