Modern React with Redux [2023 Update]
Congratulations! You've found the most popular, most complete, and most up-to-date resource online for learning React and Redux!
Thousands of other engineers have learned React and Redux, and you can too. This course uses a time-tested, battle-proven method to make sure you understand exactly how React and Redux work, and will get you a new job working as a software engineer or help you build that app you've always been dreaming about.
Read more about the course
The difference between this course and all the others: you will understand the design patterns used by top companies to build massively popular web apps.
React is the most popular Javascript library of the last five years, and the job market is still hotter than ever. Companies large and small can't hire engineers who understand React and Redux fast enough, and salaries for engineers are at an all time high. It's a great time to learn React!
What will you build?
This course features hundreds of videos with dozens of custom diagrams to help you understand how React and Redux work. No prior experience with either is necessary. Through tireless, patient explanations and many interesting practical examples, you'll learn the fundamentals of building dynamic and live web apps using React.
Each topic included in this course is added incrementally, to make sure that you have a solid foundation of knowledge. You'll find plenty of discussion added in to help you understand exactly when and where to use each feature of React and Redux.
My guarantee to you: there is no other course online that teaches more features of React and Redux. This is the most comprehensive resource there is.
Below is a partial list of the topics you'll find in this course:
Master the fundamental features of React, including JSX, state, and props
From square one, understand how to build reusable components
Dive into the source code of Redux to understand how it works behind the scenes
Test your knowledge and hone your skills with numerous coding exercises
Integrate React with advanced browser features, even geolocation API's!
Use popular styling libraries to build beautiful apps
Master different techniques of deployment so you can show off the apps you build!
See different methods of building UI's through composition of components
Besides just React and Redux, you'll pick up countless other tidbits of knowledge, including ES2015 syntax, popular design patterns, even the clearest explanation of the keyword 'this' in Javascript that you'll ever hear.
This is the course I wanted to take when I first learned React: complete, up-to-date, and clear.
- A Mac or Windows Computer
- Programmers looking to learn React
- Developers who want to grow out of just using jQuery
- Engineers who have researched React but have had trouble mastering some concepts
What you'll learn:
- Build amazing single page applications with React JS and Redux
- Master fundamental concepts behind structuring Redux applications
- Realize the power of building composable components
- Be the engineer who explains how Redux works to everyone else, because you know the fundamentals so well
- Become fluent in the toolchain supporting React, including NPM, Webpack, Babel, and ES6/ES2015 Javascript syntax
Watch Online Modern React with Redux [2023 Update]
# | Title | Duration |
---|---|---|
1 | How to Get Help | 01:02 |
2 | Let's Build an App! | 07:57 |
3 | Critical Questions | 05:50 |
4 | A Few More Critical Questions | 08:52 |
5 | Node Setup | 01:59 |
6 | Creating a React Project | 02:39 |
7 | What is Create React App? | 05:51 |
8 | Showing Basic Content | 06:42 |
9 | What is JSX? | 05:49 |
10 | Printing JavaScript Variables in JSX | 04:30 |
11 | Shorthand JS Expressions | 01:52 |
12 | Exercise Overview | 00:43 |
13 | Exercise Solution | 02:06 |
14 | Typical Component Layouts | 01:59 |
15 | Customizing Elements with Props | 07:37 |
16 | Converting HTML to JSX | 05:08 |
17 | Applying Styling in JSX | 06:14 |
18 | Exercise Solution | 02:06 |
19 | Extracting Components | 04:27 |
20 | Module Systems Overview | 16:07 |
21 | Project Overview | 05:38 |
22 | Creating Core Components | 05:01 |
23 | Introducing the Props System | 04:52 |
24 | Picturing the Movement of Data | 06:07 |
25 | Adding Props | 03:05 |
26 | Using Argument Destructuring | 04:58 |
27 | Exercise Solution | 01:32 |
28 | The React Developer Tools | 02:38 |
29 | The Most Common Props Mistake | 04:44 |
30 | Including Images | 08:10 |
31 | Handling Image Accessibility | 06:40 |
32 | Review on how CSS Works | 06:11 |
33 | Adding CSS Libraries with NPM | 05:58 |
34 | A Big Pile of HTML! | 09:39 |
35 | Last Bit of Styling | 05:46 |
36 | App Overview | 02:53 |
37 | Initial App Setup | 05:10 |
38 | Introducing the Event System | 03:16 |
39 | Events in Detail | 08:12 |
40 | Variations on Event Handlers | 06:43 |
41 | Exercise Solution | 01:16 |
42 | Introducing the State System | 03:44 |
43 | More on State | 08:44 |
44 | Understanding the Re-Rendering Process | 08:11 |
45 | Why Array Destructuring? | 08:43 |
46 | Back to the App | 03:34 |
47 | Picking a Random Element | 07:12 |
48 | List Building in React | 09:47 |
49 | Loading and Showing SVGs | 05:51 |
50 | Increasing Image Size | 05:52 |
51 | Adding Custom CSS | 06:22 |
52 | Finalizing Styling | 05:59 |
53 | App Wrapup and Review | 08:53 |
54 | App Overview | 02:25 |
55 | Project Setup | 05:54 |
56 | The Path Forward | 04:07 |
57 | Overview of HTTP Requests | 11:17 |
58 | Understanding the API | 06:08 |
59 | Making an HTTP Request | 10:37 |
60 | [Optional] Using Async:Await | 02:40 |
61 | Data Fetching Cleanup | 03:21 |
62 | Thinking About Data Flow | 08:43 |
63 | Child to Parent Communication | 06:08 |
64 | Implementing Child to Parent Communication | 07:30 |
65 | Handling Form Submission | 11:33 |
66 | Handling Input Elements | 09:22 |
67 | [Optional] OK But Why? | 14:22 |
68 | Exercise Solution | 02:36 |
69 | Running the Search | 04:07 |
70 | Reminder on Async:Await | 03:01 |
71 | Communicating the List of Images Down | 12:32 |
72 | Building a List of Images | 05:04 |
73 | Handling List Updates | 09:11 |
74 | Notes on Keys | 09:23 |
75 | Displaying Images | 01:49 |
76 | A Touch of Styling | 04:27 |
77 | App Wrapup | 03:42 |
78 | App Overview | 03:21 |
79 | Initial Setup | 05:57 |
80 | State Location | 05:15 |
81 | Reminder on Event Handlers | 04:52 |
82 | Receiving New Titles | 08:56 |
83 | Adding Styling | 02:13 |
84 | Updating State | 10:26 |
85 | Don't Mutate That State! | 04:27 |
86 | [Optional] Adding Elements to the Start or End | 01:25 |
87 | [Optional] Exercise Solution | 02:10 |
88 | [Optional] Inserting Elements | 04:44 |
89 | [Optional] Exercise Solution | 02:10 |
90 | [Optional] Removing Elements | 07:05 |
91 | [Optional] Exercise Solution | 02:10 |
92 | [Optional] Modifying Elements | 06:06 |
93 | [Super Optional] Why the Special Syntax? | 07:11 |
94 | [Optional] Exercise Solution | 02:27 |
95 | [Optional] Adding, Changing, or Removing Object Properties | 03:59 |
96 | Adding a Book, For Real! | 01:50 |
97 | Generating Random ID's | 02:06 |
98 | Displaying the List | 05:36 |
99 | Deleting Records | 06:37 |
100 | Toggling Form Display | 06:32 |
101 | Default Form Values | 07:07 |
102 | Updating the Title | 08:45 |
103 | Closing the Form on Submit | 05:07 |
104 | A Better Solution! | 04:31 |
105 | Collapsing Two Handlers into One | 03:19 |
106 | Adding Images | 07:59 |
107 | Adding Data Persistence | 07:49 |
108 | Server Setup | 03:45 |
109 | What Just Happened? | 04:37 |
110 | How the API Works | 05:21 |
111 | Introducing the REST Client | 05:07 |
112 | Using the REST Client | 06:59 |
113 | Creating a New Record | 06:57 |
114 | Fetching a List of Records | 04:26 |
115 | Introducing useEffect | 02:52 |
116 | useEffect in Action | 06:25 |
117 | More on useEffect | 09:53 |
118 | Updating a Record | 03:19 |
119 | Thinking About Updates | 05:28 |
120 | Deleting a Record | 01:19 |
121 | Introducing Context | 09:13 |
122 | Context in Action | 06:16 |
123 | Changing Context Values | 06:52 |
124 | More on Changing Context | 08:47 |
125 | Application vs Component State | 11:52 |
126 | Refactoring to Use Context | 04:39 |
127 | Refactoring the App | 05:33 |
128 | Reminder on Sharing with Context | 06:13 |
129 | Props and Context Together | 03:09 |
130 | Last Bit of Refactoring | 05:57 |
131 | A Small Taste of Reusable Hooks | 09:54 |
132 | Return to useEffect | 08:07 |
133 | Understanding the Issue | 07:18 |
134 | Applying the Fix | 06:24 |
135 | ESLint is Good, but be Careful! | 10:58 |
136 | Stable References with useCallback | 13:09 |
137 | Fixing Bugs with useCallback | 02:09 |
138 | useEffect Cleanup Functions | 05:34 |
139 | The Purpose of Cleanup Functions | 05:38 |
140 | Project Overview | 04:26 |
141 | Project Setup | 01:55 |
142 | Some Button Theory | 08:20 |
143 | Underlying Elements | 03:49 |
144 | The Children Prop | 04:22 |
145 | Props Design | 06:22 |
146 | Validating Props with PropTypes | 08:30 |
147 | PropTypes in Action | 06:53 |
148 | Introducing TailwindCSS | 06:38 |
149 | Installing Tailwind | 04:51 |
150 | How to use Tailwind | 06:36 |
151 | Review on Styling | 10:23 |
152 | The ClassNames Library | 11:29 |
153 | Building Some Variations | 05:56 |
154 | Finalizing the Variations | 06:08 |
155 | Using Icons in React Projects | 09:50 |
156 | Issues with Event Handlers | 07:17 |
157 | Passing Props Through | 06:08 |
158 | Handling the Special ClassName Case | 05:18 |
159 | Project Organization | 10:44 |
160 | Refactoring with Organization | 05:57 |
161 | Component Overview | 04:49 |
162 | Component Setup | 03:43 |
163 | Reminder on Building Lists | 04:05 |
164 | State Design Process Overview | 29:31 |
165 | Finding the Expanded Item | 04:10 |
166 | Conditional Rendering | 06:26 |
167 | Inline Event Handlers | 10:01 |
168 | Variation on Event Handlers | 08:22 |
169 | Conditional Icon Rendering | 02:15 |
170 | Displaying Icons | 01:46 |
171 | Adding Styling | 03:49 |
172 | Toggling Panel Collapse | 02:08 |
173 | [Optional] Delayed State Updates | 08:35 |
174 | [Optional] Functional State Updates | 11:44 |
175 | Exercise Solution | 01:27 |
176 | Component Overview | 01:09 |
177 | Designing the Props | 05:50 |
178 | Component Creation | 04:15 |
179 | [Optional] More State Design | 20:12 |
180 | Finally... Implementation! | 06:46 |
181 | Reminder on Event Handlers in Maps | 04:48 |
182 | Dropdown as a Controlled Component | 04:46 |
183 | Controlled Component Implementation | 05:37 |
184 | Existence Check Helper | 04:31 |
185 | Community Convention with Props Names | 06:35 |
186 | Exercise Solution | 01:38 |
187 | Adding Styling | 05:34 |
188 | The Panel Component | 07:08 |
189 | Creating the Reusable Panel | 06:00 |
190 | A Challenging Extra Feature | 05:20 |
191 | Document-Wide Click Handlers | 02:47 |
192 | Event Capture and Bubbling | 06:15 |
193 | Putting it All Together | 02:21 |
194 | Why a Capture Phase Handler? | 14:35 |
195 | Reminder on the useEffect Function | 03:53 |
196 | Reminder on useEffect Cleanup | 05:47 |
197 | Issues with Element References | 02:57 |
198 | useRef in Action | 05:06 |
199 | Checking Click Location | 03:15 |
200 | Traditional Browser Navigation | 09:34 |
201 | Theory of Navigation in React | 07:57 |
202 | Extracting the DropdownPage | 02:44 |
203 | Answering Critical Questions | 05:34 |
204 | The PushState Function | 02:57 |
205 | Handling Link Clicks | 02:29 |
206 | Handling Back:Forward Buttons | 06:24 |
207 | Navigation Context | 04:06 |
208 | Listening to Forward and Back Clicks | 06:20 |
209 | Programmatic Navigation | 05:37 |
210 | A Link Component | 05:26 |
211 | A Route Component | 05:50 |
212 | Handling Control and Command Keys | 02:53 |
213 | Link Styling | 01:19 |
214 | Custom Navigation Hook | 02:56 |
215 | Adding a Sidebar Component | 08:23 |
216 | Highlighting the Active Link | 07:26 |
217 | Navigation Wrapup | 01:55 |
218 | Modal Component Overview | 04:22 |
219 | Toggling Visibility | 05:50 |
220 | At First Glance, Easy! | 03:06 |
221 | We're Lucky it Works At All! | 09:27 |
222 | Fixing the Modal with Portals | 07:10 |
223 | Closing the Modal | 02:05 |
224 | Customizing the Modal | 05:04 |
225 | Additional Styling | 01:45 |
226 | One Small Bug | 01:54 |
227 | Modal Wrapup | 05:49 |
228 | Creating a Reusable table | 03:35 |
229 | Communicating Data to the Table | 03:04 |
230 | Reminder on Table HTML Structure | 04:26 |
231 | Building the Rows | 02:53 |
232 | Better Styling | 03:39 |
233 | Done! But It's Not Reusable | 05:11 |
234 | Here's the Idea | 02:30 |
235 | Dynamic Table Headers | 04:47 |
236 | Rendering Individual Cells | 05:19 |
237 | Fixed Cell Values | 05:01 |
238 | Nested Maps | 04:36 |
239 | Fixing the Color | 00:58 |
240 | Adding a Key Function | 04:13 |
241 | Adding Sorting to the Table | 06:39 |
242 | Reminder on Sorting in JavaScript | 06:25 |
243 | Sorting Strings | 02:47 |
244 | Sorting Objects | 03:58 |
245 | Object Sort Implementation | 07:39 |
246 | Reversing Sort Order | 04:27 |
247 | Optional Sorting | 04:11 |
248 | A Small Extra Feature | 03:15 |
249 | Customizing Header Cells | 03:50 |
250 | React Fragments | 05:59 |
251 | The Big Reveal | 10:40 |
252 | Adding SortableTable | 11:33 |
253 | Watching for Header Cell Clicks | 01:44 |
254 | Quick State Design | 06:58 |
255 | Adding Sort State | 05:25 |
256 | Yessssss, It Worked! | 11:01 |
257 | Determining Icon Set | 05:09 |
258 | Styling Header Cells | 03:38 |
259 | Resetting Sort Order | 02:56 |
260 | Table Wrapup | 03:54 |
261 | Exploring Code Reuse | 03:40 |
262 | Revisiting Custom Hooks | 03:11 |
263 | Creating the Demo Component | 04:47 |
264 | Custom Hook Creation | 03:17 |
265 | Hook Creation Process in Depth | 09:43 |
266 | Making a Reusable Sorting Hook | 11:10 |
267 | App Overview | 03:04 |
268 | Adding the Form | 04:27 |
269 | More on the Form | 06:59 |
270 | useReducer in Action | 09:48 |
271 | Rules of Reducer Functions | 09:23 |
272 | Understanding Action Objects | 10:47 |
273 | Constant Action Types | 06:36 |
274 | Refactoring to a Switch | 05:35 |
275 | Adding New State Updates | 06:21 |
276 | A Few Design Considerations Around Reducers | 09:52 |
277 | Introducing Immer | 05:00 |
278 | Immer in Action | 03:22 |
279 | Into the World of Redux | 05:24 |
280 | Redux vs Redux Toolkit | 09:18 |
281 | App Overview | 03:04 |
282 | The Path Forward | 03:33 |
283 | Implementation Time! | 04:42 |
284 | Understanding the Store | 09:22 |
285 | The Store's Initial State | 03:33 |
286 | Understanding Slices | 07:51 |
287 | Understanding Action Creators | 06:34 |
288 | Connecting React to Redux | 04:25 |
289 | Updating State from a Component | 08:26 |
290 | Accessing State in a Component | 06:35 |
291 | Removing Content | 07:13 |
292 | Practice Updating State! | 06:56 |
293 | Practice Accessing State! | 02:03 |
294 | Even More State Updating! | 04:30 |
295 | Resetting State | 06:26 |
296 | Multiple State Updates | 04:48 |
297 | Understanding Action Flow | 06:46 |
298 | Watching for Other Actions | 05:12 |
299 | Getting an Action Creator's Type | 03:08 |
300 | Manual Action Creation | 09:32 |
301 | File and Folder Structure | 08:42 |
302 | Refactoring the Project Structure | 11:08 |
303 | Project Overview | 04:11 |
304 | Adding Component Boilerplate | 06:09 |
305 | Thinking About Derived State | 07:55 |
306 | Thinking About Redux Design | 05:18 |
307 | Adding the Form Slice | 05:35 |
308 | Maintaining a Collection with a Slice | 09:41 |
309 | Creating the Store | 04:34 |
310 | Form Values to Update State | 08:45 |
311 | Receiving the Cost | 05:18 |
312 | Dispatching During the Form Submission | 04:32 |
313 | Awkward Double Keys | 07:19 |
314 | Listing the Records | 03:26 |
315 | Deleting Records | 02:17 |
316 | Adding Styling | 01:29 |
317 | Form Reset on Submission | 03:22 |
318 | Reminder on ExtraReducers | 02:24 |
319 | Adding a Searching Input | 05:37 |
320 | Derived State in useSelector | 05:08 |
321 | Total Car Cost | 06:23 |
322 | Highlighting Existing Cars | 06:31 |
323 | App Overview | 08:37 |
324 | Adding a Few Dependencies | 02:46 |
325 | Initial App Boilerplate | 02:10 |
326 | API Server Setup | 02:52 |
327 | Adding a Few Components | 03:14 |
328 | Creating the Redux Store | 05:35 |
329 | Thinking About Data Structuring | 09:20 |
330 | Reminder on Request Conventions | 01:56 |
331 | Data Fetching Techniques | 02:38 |
332 | Adding State for Data Loading | 06:53 |
333 | Understanding Async Thunks | 04:15 |
334 | Steps for Adding a Thunk | 07:47 |
335 | More on Adding Thunks | 13:18 |
336 | Wrapping up the Thunk | 05:24 |
337 | Using Loading State | 04:31 |
338 | Adding a Pause for Testing | 03:28 |
339 | Adding a Skeleton Loader | 05:40 |
340 | Animations with TailwindCSS | 12:03 |
341 | Rendering the List of Users | 03:17 |
342 | Creating New Users | 12:08 |
343 | Unexpected Loading State | 06:04 |
344 | Strategies for Fine-Grained Loading State | 07:36 |
345 | Local Fine-Grained Loading State | 10:08 |
346 | More on Loading State | 02:56 |
347 | Handling Errors with User Creation | 05:04 |
348 | Creating a Reusable Thunk Hook | 11:35 |
349 | Creating a Fetch-Aware Button Component | 05:43 |
350 | Better Skeleton Display | 03:42 |
351 | A Thunk to Delete a User | 04:57 |
352 | Updating the Slice | 04:29 |
353 | Refactoring the Component | 03:33 |
354 | Deleting the User | 04:47 |
355 | Fixing a Delete Error | 05:14 |
356 | Album Feature Overview | 04:49 |
357 | Additional Components | 07:22 |
358 | Adding the ExpandablePanel | 04:52 |
359 | Wrapping Up the ExpandablePanel | 04:01 |
360 | Adding the Albums Listing | 02:44 |
361 | [Optional] Getting Caught Up | 02:48 |
362 | Introducing Redux Toolkit Query | 08:01 |
363 | Creating a RTK Query API | 06:21 |
364 | Creating an Endpoint | 14:02 |
365 | Using the Generated Hook | 11:02 |
366 | A Few Immediate Notes | 05:57 |
367 | Rendering the List | 04:26 |
368 | Changing Data with Mutations | 08:43 |
369 | Differences Between Queries and Mutations | 03:14 |
370 | Options for Refetching Data | 06:19 |
371 | Request De-Duplication | 03:51 |
372 | Some Internals of Redux Toolkit Query | 06:15 |
373 | Refetching with Tags | 07:34 |
374 | Fine-Grained Tag Validation | 09:14 |
375 | Styling Fixups | 03:21 |
376 | Adding a Pause for Testing | 03:22 |
377 | Implementing Delete Endpoints | 05:35 |
378 | Refactoring the List | 04:47 |
379 | Remove Implementation | 07:43 |
380 | Easy Tag Invalidation | 05:59 |
381 | Getting Clever with Cache Tags | 06:25 |
382 | More Clever Tag Implementation | 04:48 |
383 | Photos Feature Overview | 04:12 |
384 | Lots of Photos Setup! | 06:02 |
385 | Adding the Endpoints | 12:39 |
386 | Creating the Photo | 04:38 |
387 | Showing the List of Photos | 05:15 |
388 | Adding Mouse-Over Deletes | 06:20 |
389 | Adding Automatic Data Refetching | 08:36 |
Similar courses to Modern React with Redux [2023 Update]
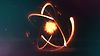
Understanding React | Don’t Imitate UnderstandAnthony Alicea
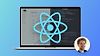
The Ultimate React Course 2024: React, Redux & Moreudemy
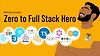
Zero to Full Stack Heropapareact.com
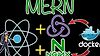
MERN Invoice Web App with Docker,NGINX and ReduxToolkitudemy
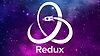
The Ultimate Redux Coursecodewithmosh (Mosh Hamedani)
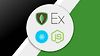
MERN 2024 Edition - MongoDB, Express, React and NodeJSudemy
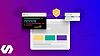
Microservices with Node JS and ReactudemyStephen Grider
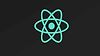
Starting with React & Redux: Build modern apps (2nd edition)udemy
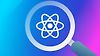
Testing React Apps with React Testing Librarycodewithmosh (Mosh Hamedani)
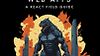