Nodejs Express - unit testing/integration tests with Jest
Learn how to write a full CRUD REST API with Nodejs, Express and Jest using the test-driven development method! TDD is becoming more and more of standard, and for good reasons! With TDD, you can feel more safe and secure that any changes you make are not breaking existing functionality in your applications. Manual tests cannot be sustainable in large applications, or even medium applications. Plus, they're incredibly tedious.
Read more about the course
Without TDD... well, you know the story. You try to add functionality while trying to touch the existing code as little as possible, because you don't want to break things unintentionally..
With TDD you won't be scared to make big refactors in your code to clean up messy code, you will know quickly if you've broken any existing functionality!
I'll show you step-by-step how to create a REST API with Express with unit tests and integration tests using the Jest test framework. There's going to be lots of opportunities for you to work on your own and do some exercises as well for optimal learning!
If you have any questions or suggestions to the course, feel free to message me anytime, I love interacting with my students!
- Students somewhat familiar with Nodejs who wants to learn how to make production-ready software with TDD
- Basic JavaScript ES6
- Some familiarity with Nodejs Express is a plus
What you'll learn:
- Learn how to make unit tests
- Learn how to make integration tests
- Learn how to create a Express REST API from scratch
- Learn how to build a Express REST API with test-driven development
- Learn how to mock Mongoose models in Nodejs with Jest
- Learn how to use Jest with TDD to build a REST API
- Learn how to use MongoDB with Mongoose together with Jest tests
- Learn how to write tests for error handling
Watch Online Nodejs Express - unit testing/integration tests with Jest
# | Title | Duration |
---|---|---|
1 | Initialising project | 01:19 |
2 | Hello World Express REST API in less than 5 mins! | 03:51 |
3 | CRUD Methods we are going to build the TDD-way in REST | 01:31 |
4 | Short intro to Jest + How to install Jest in our project | 00:40 |
5 | Our first test with Jest and Express REST API | 03:56 |
6 | Making our first test with Jest + Express REST API pass! | 01:17 |
7 | Make Jest run automatically on file changes | 01:22 |
8 | Defining a data model for our Todo items with Mongoose | 03:28 |
9 | Using jest.fn to mock our Mongoose model functions | 04:05 |
10 | Making our test with Jest mock model pass | 01:41 |
11 | How to configure Jest test environment | 01:29 |
12 | How to mock Request and Response objects in Express API | 03:23 |
13 | Writing test with mock Request object and making it pass! | 02:46 |
14 | Using beforeEach in Jest tests | 01:18 |
15 | Testing if response code is 201 and response is sent | 02:37 |
16 | Test if response is sending back JSON body | 04:48 |
17 | Creating our first integration test with Supertest and Jest | 06:36 |
18 | How to make our Express app ready for integration testing | 01:50 |
19 | Creating our Express Router | 03:16 |
20 | How to debug tests in Jest | 02:09 |
21 | Setting up Express JSON request body middleware | 01:01 |
22 | Fix for address already in use error | 01:05 |
23 | Making our controller function use async/await | 03:01 |
24 | Setting up our MongoDB database in the cloud! | 02:04 |
25 | Creating a MongoDB database user | 01:25 |
26 | Connect to MongoDB database with Mongoose | 03:18 |
27 | Modify our unit tests to use async/await | 03:30 |
28 | Creating a server.js for running our Express REST API manually | 02:09 |
29 | Manual Testing with Postman | 03:06 |
30 | Oops! We're missing error handling! | 02:56 |
31 | Unit tests for error handling | 03:17 |
32 | Making our error handling unit test PASS | 00:55 |
33 | Creating a integration test for error handling | 01:42 |
34 | Manual test for error handling | 01:15 |
35 | Making a test for errors returning JSON body | 00:48 |
36 | Create middleware to parse errors in JSON | 02:31 |
37 | Making all of our tests pass and final manual test! | 02:03 |
38 | Intro + first test exercise | 01:27 |
39 | Making first test for getTodos pass | 01:37 |
40 | Test if TodoModel.find is called | 03:23 |
41 | Test if response is being sent back correctly | 04:34 |
42 | Test for error handling | 03:48 |
43 | Integration test for GET /todos | 03:59 |
44 | Manual test with Postman | 00:57 |
45 | Intro + Getting first test done | 02:43 |
46 | Creating mock function TodoModel.findById and test for calls | 03:15 |
47 | Exercise! Make test for response and make test pass! | 01:44 |
48 | Solution to response testing with getById() | 04:20 |
49 | Error handling unit test | 02:54 |
50 | If TodoModel could not be found in database | 02:31 |
51 | Integration test for GET /todos/:todoId | 03:58 |
52 | 404 Integration test + final manual tests | 03:08 |
53 | Intro to PUT method and first test | 01:07 |
54 | Test if TodoModel.findByIdAndUpdate is called | 04:50 |
55 | Test if PUT response is sent back | 03:07 |
56 | Test HTTP PUT error handling | 02:18 |
57 | HTTP PUT 404 test | 02:10 |
58 | HTTP PUT Integration test | 03:59 |
59 | Using Jest.mock instead of multiple Jest.fn + Intro to final challenge! | 02:59 |
60 | Unit tests for HTTP Delete method | 08:15 |
61 | Integration test for HTTP Delete Method + manual test + you are awesome!! | 06:12 |
Similar courses to Nodejs Express - unit testing/integration tests with Jest
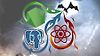
Master Full-Stack Web Development | Node, SQL, React, & Moreudemy
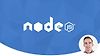
The Complete Node.js Developer Course (3rd Edition)udemy
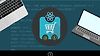
MERN eCommerce From ScratchudemyBrad Traversy
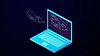
Full Stack Isomorphic JavaScript with Vue.js & Node.jsudemy
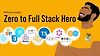
Zero to Full Stack Heropapareact.com
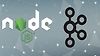
NodeJS Microservices: Breaking a Monolith to Microservicesudemy
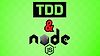