Python 3: Deep Dive (Part 3 - Hash Maps)
This course is an in-depth look at Python dictionaries. Dictionaries are ubiquitous in Python. Classes are essentially dictionaries, modules are dictionaries, namespaces are dictionaries, sets are dictionaries and many more.
Read more about the course
In this course we'll take an in-depth look at:
associative arrays and how they can be implemented using hash maps
hash functions and how we can leverage them for our own custom classes
Python dictionaries and sets and the various operations we can perform with them
specialized dictionary structures such as OrderedDict and how it relates to the built-in Python3.6+ dict
Python's implementation of multi-sets, the Counter class
the ChainMap class
how to create custom dictionaries by inheriting from the UserDict class
how to serialize and deserialize dictionaries to JSON
the use of schemas in custom JSON deserialization
a brief introduction to some useful libraries such as JSONSchema, Marshmallow, PyYaml and Serpy
Please note that this is a relatively advanced Python course, and a strong knowledge of some topics in Python is required.
Beyond the basics of Python (loops, conditional statements, exception handling, built-in data types, creating classes, etc), you should also have an in-depth understanding of the following topics:
functions and functional programming (recursion, *args, **kwargs, zip, map, sorted, any, all, etc)
lambdas, closures and decorators (including standard decorators such as @singledispatch, @wraps, etc)
iterables, iterators, generators and context managers
named tuples
variable scopes and namespaces (globals, locals, etc)
For this course you will also need to install some 3rd party libraries, so you need to be comfortable with doing this using the tool of your choice (e.g. pip, conda, etc)
Finally, most of the code in this course is illustrated using the freely available Jupyter Notebooks, so you will need that as well.
- This is an advanced course, so a solid Python foundation is necessary
- Jupyter Notebooks
- functional programming (zip, map, sorted, any, all, etc)
- lambdas, closures and decorators
- built-in decorators such as @lru_cache, @singledispatch and @wraps
- iterables, iterators, generators and context managers
- variable scopes and namespaces (globals, locals, etc)
- ability to install 3rd party libraries (e.g. pip install)
- Python developers who want a deeper understanding of Python dictionaries and related topics
What you'll learn:
- Associative Arrays
- Hash Tables and Hash Functions
- Python's implementation of hash tables
- Dictionaries and Sets
- Defining hash functions for our custom classes and why that is useful
- Creating customized dictionaries using the UserDict class
- defaultdict
- OrderedDict and Python3.6+ equivalences
- Counter (multi-sets)
- ChainMap
- Serialization and Deserialization
- JSON serialization/deserialization
- Intro to JSONSchema, Marshmallow, PyYaml and Serpy 3rd party libraries
Watch Online Python 3: Deep Dive (Part 3 - Hash Maps)
# | Title | Duration |
---|---|---|
1 | Course Overview | 12:57 |
2 | Prerequisites | 07:23 |
3 | Introduction | 03:25 |
4 | Associative Arrays | 05:41 |
5 | Hash Maps | 24:13 |
6 | Python Dictionaries | 09:39 |
7 | Python's hash() Function | 08:04 |
8 | Introduction | 01:14 |
9 | Creating Dictionaries - Lecture | 13:10 |
10 | Creating Dictionaries - Coding | 24:02 |
11 | Common Operations - Lecture | 08:12 |
12 | Common Operations - Coding | 32:44 |
13 | Dictionary Views - Lecture | 10:42 |
14 | Dictionary Views - Coding | 30:13 |
15 | Updating, Merging, and Copying - Lecture | 12:21 |
16 | Updating, Merging, and Copying - Coding | 33:56 |
17 | Custom Classes and Hashing - Lecture | 20:26 |
18 | Custom Classes and Hashing - Coding | 33:55 |
19 | Exercises | 06:22 |
20 | Solution 1 | 04:35 |
21 | Solution 2 | 02:05 |
22 | Solution 3 | 07:15 |
23 | Solution 4 | 05:23 |
24 | Introduction | 01:56 |
25 | Basic Set Theory | 12:36 |
26 | Python Sets | 08:59 |
27 | Creating Sets - Lecture | 03:41 |
28 | Creating Sets - Coding | 14:46 |
29 | Common Operations - Lecture | 03:13 |
30 | Common Operations - Coding | 15:18 |
31 | Set Operations - Lecture | 08:01 |
32 | Set Operations - Coding | 20:31 |
33 | Update Operations - Lecture | 06:09 |
34 | Update Operations - Coding | 21:08 |
35 | Copying Sets - Lecture | 01:14 |
36 | Copying Sets - Coding | 08:11 |
37 | Frozen Sets - Lecture | 06:55 |
38 | Frozen Sets - Coding | 26:38 |
39 | Dictionary Views - Lecture | 09:28 |
40 | Dictionary Views - Coding | 27:59 |
41 | Project 1 - Goals | 05:31 |
42 | Project 1 - Solution | 49:38 |
43 | Introduction | 04:30 |
44 | Pickling - Lecture | 05:58 |
45 | Pickling - Coding | 28:48 |
46 | JSON Serialization - Lecture | 07:23 |
47 | JSON Serialization - Coding | 22:30 |
48 | Custom JSON Encoding - Lecture | 02:31 |
49 | Custom JSON Encoding - Coding | 37:37 |
50 | Using JSONEncoder - Lecture | 11:37 |
51 | Using JSONEncoder - Coding | 34:01 |
52 | Custom JSON Decoding - Lecture | 16:36 |
53 | Custom JSON Decoding - Coding | 51:59 |
54 | Using JSONDecoder - Lecture | 01:30 |
55 | Using JSONDecoder - Coding | 41:01 |
56 | JSON Schema | 26:07 |
57 | Marshmallow | 33:37 |
58 | PyYaml | 18:17 |
59 | Serpy | 08:57 |
60 | Exercises | 03:49 |
61 | Solution 1 | 15:22 |
62 | Solution 2 | 21:02 |
63 | Solution 3 | 17:05 |
64 | Introduction | 02:03 |
65 | DefaultDict - Lecture | 06:34 |
66 | DefaultDict - Coding | 30:47 |
67 | OrderedDict - Lecture | 03:41 |
68 | OrderedDict - Coding | 24:23 |
69 | OrderedDict and Python 3.6 Dicts | 31:45 |
70 | Counter - Lecture | 05:02 |
71 | Counter - Coding | 43:45 |
72 | ChainMap - Lecture | 11:09 |
73 | ChainMap - Coding | 22:45 |
74 | UserDict - Lecture | 05:35 |
75 | UserDict - Coding | 25:39 |
76 | Exercises | 05:35 |
77 | Solution 1 | 05:53 |
78 | Solution 2 | 04:38 |
79 | Solution 3 | 14:30 |
Similar courses to Python 3: Deep Dive (Part 3 - Hash Maps)
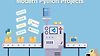
Modern Python ProjectsTalkpython
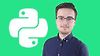
The Complete Python Course | Learn Python by Doingudemy
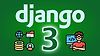
Django 3 - Full Stack Websites with Python Web Developmentudemy
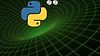
Python 3: Deep Dive (Part 2 - Iteration, Generators)udemy
![[Full Stack] Airbnb Clone Coding](https://cdn.courseflix.net/courses/100x56/full-stack-airbnb-clone-coding.jpg?d=1748875200021)
[Full Stack] Airbnb Clone CodingNomad Coders
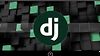
Python Django Dev To DeploymentudemyBrad Traversy
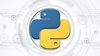
Complete Python Developer in 2023: Zero to Masteryudemyzerotomastery.io
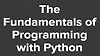
The Fundamentals of Programming with Pythontechwithtim.net (Tim Ruscica)
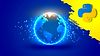
Python for Business Data Analytics & Intelligencezerotomastery.io
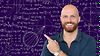