70+ JavaScript Challenges: Data Structures & Algorithms
12h 29m 29s
English
Paid
I am known for my practical project-based courses. I created this course because, in addition to those practical skills, I want to give you more foundational problem solving skills and dive into algorithms by solving challenges and learn about and implement data structures like stacks, queues, trees and hash maps. We also learn about other computer science fundamentals like recursion and complexity. Pass those pesky interviews by taking this course.
Read more about the course
What you'll learn
- Take on challenges or follow along and learn by example
- Solve problems using iteration, recursion, high order array methods and more
- Learn about time & space complexity for more efficient code
- Learn about and implement data structures like stacks, queues, linked lists, trees and graphs
- Depth-first and breadth-first traversal of binary trees and graphs
- Sorting algorithms like bubble sort and merge sort
- Full sandbox environment with Jest testing
Watch Online 70+ JavaScript Challenges: Data Structures & Algorithms
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Welcome To The Course | 03:24 |
2 | Course Structure | 04:19 |
3 | DSA - What & Why | 06:56 |
4 | Tools & Basic Setup | 05:36 |
5 | Sandbox Files Setup & Explanation | 06:28 |
6 | Hello World (Starter Challenge) | 01:58 |
7 | Get Sum (Starter Challenge) | 02:03 |
8 | Calculator | 05:45 |
9 | Count Occurences | 07:44 |
10 | Find Max Number | 03:44 |
11 | Title Case | 05:25 |
12 | Reverse String | 07:20 |
13 | Palindrome | 14:27 |
14 | Count Vowels | 03:08 |
15 | Remove Duplicates | 05:31 |
16 | FizzBuzz Array | 05:42 |
17 | Array Intersection | 05:56 |
18 | Display Likes | 04:57 |
19 | Find Missing Number | 06:18 |
20 | Find Missing Letter | 07:43 |
21 | Are All Characters Unique | 07:14 |
22 | First Non-Repeating Character | 07:52 |
23 | Dice Game Simulation | 06:33 |
24 | Format Phone Number | 09:20 |
25 | Validate Email | 09:16 |
26 | Simple Examples | 13:32 |
27 | Sum Of Even Squares | 04:54 |
28 | Calculate Total Sales | 06:05 |
29 | Highest Scoring Word | 10:38 |
30 | Valid Anagrams | 07:12 |
31 | Hashtag Generator | 10:32 |
32 | Valid IPv4 | 04:28 |
33 | Analyze Car Milage | 07:46 |
34 | Password Validator | 06:19 |
35 | Find Missing Letter Refactor | 10:36 |
36 | Recursion Intro (Countdown) | 06:56 |
37 | Recursive Unwinding (Sum Up To) | 05:50 |
38 | Reverse String Using Recursion | 07:35 |
39 | Fibonacci Sequence | 10:41 |
40 | Factorial | 06:38 |
41 | Power | 05:49 |
42 | Array Sum | 05:54 |
43 | Number Range | 04:44 |
44 | Flatten Array | 04:01 |
45 | Permutations | 07:29 |
46 | What Is Time Complexity? | 05:31 |
47 | Big O Notation | 05:42 |
48 | Constant Time Complexity | 05:20 |
49 | Linear Time Complexity | 05:25 |
50 | Quadratic Time Complexity | 04:27 |
51 | Logarithmic Time Complexity | 04:57 |
52 | Space Complexity | 06:25 |
53 | Max Subarray - Quadratic | 08:21 |
54 | Sliding Window Technique | 04:10 |
55 | Max Subarray - Linear | 06:50 |
56 | Hash Table Intro | 03:54 |
57 | Maps | 09:52 |
58 | Word Frequency Counter | 05:36 |
59 | Phone Number Directory | 02:42 |
60 | Anagram Grouping | 05:39 |
61 | Sets | 05:29 |
62 | Symmetric Difference | 04:47 |
63 | Two Sum | 05:36 |
64 | Longest Consecutive | 06:27 |
65 | Custom Hash Table - Init, Set & Print | 18:44 |
66 | Custom Hash Table - Get, Remove & Has | 11:24 |
67 | Word Instance Counter With Custom Hash Table | 05:54 |
68 | Add getValues Method | 03:28 |
69 | Anagram Grouping With Custom Hash Table | 05:50 |
70 | What Is A Stack? | 02:25 |
71 | Stack Implementation | 10:32 |
72 | Reverse String Using A Stack | 04:05 |
73 | Balanced Parenthesis | 04:29 |
74 | What Is A Queue? | 02:20 |
75 | Queue Implementation | 07:24 |
76 | Reverse String Using A Queue | 03:40 |
77 | Palindrome Using A Queue & Stack | 07:49 |
78 | What Is A Linked List? | 04:09 |
79 | Linked List Implementation | 14:18 |
80 | Reverse String Using A Linked List | 03:55 |
81 | Fast & Slow Pointers | 05:52 |
82 | Find Middle | 07:13 |
83 | What Is A Doubly Linked List? | 02:48 |
84 | Doubly Linked List Implementation - Part 1 | 14:28 |
85 | Doubly Linked List implementation - Part 2 | 10:18 |
86 | Find Pair Sum | 04:11 |
87 | What Is A Tree / Binary Tree? | 06:28 |
88 | Tree Node Class | 05:00 |
89 | Depth-First Traversal | 13:04 |
90 | Depth-First Traversal With Recursion | 04:18 |
91 | Breadth-First Traversal | 08:56 |
92 | Maximum Depth | 06:53 |
93 | What Is A Binary Search Tree? | 08:27 |
94 | Binary Search Tree Implementation - Part 1 | 10:51 |
95 | Binary Search Tree Implementation - Part 2 | 14:24 |
96 | Validate BST | 11:23 |
97 | What Is A Graph? | 03:42 |
98 | Adjacency Matrix & Adjacency List | 04:30 |
99 | Graph Implementation | 11:24 |
100 | Graph Traversal Visualization | 05:52 |
101 | Graph Depth-First Traversal | 09:56 |
102 | Graph Breadth-First Traversal | 06:17 |
103 | What Are Sorting Algorithms? | 02:48 |
104 | Bubble Sort Algorithm | 02:47 |
105 | Bubble Sort Implementation | 08:01 |
106 | Insertion Sort Algorithm | 02:44 |
107 | Insertion Sort Implementation | 06:25 |
108 | Selection Sort Algorithm | 03:29 |
109 | Selection Sort Implementation | 06:44 |
110 | Merge Sort Algorithm | 02:00 |
111 | Merge Sort Implementation | 08:27 |
112 | Quick Sort Algorithm | 02:51 |
113 | Quick Sort Implmentation | 05:27 |
114 | Wrap Up | 01:37 |
Similar courses to 70+ JavaScript Challenges: Data Structures & Algorithms
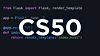
CS50's Web Programming with Python and JavaScriptHarvardX (Harvard University)
Category: JavaScript, Python
Duration 14 hours 3 minutes 25 seconds
Course
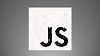
JavaScript: Understanding the Weird PartsudemyAnthony Alicea
Category: JavaScript
Duration 12 hours 10 minutes 48 seconds
Course
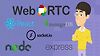
Discord Clone - Learn MERN Stack with WebRTC and SocketIOudemy
Category: JavaScript, React.js, Node.js, MongoDB, Socket.IO, WebRTC
Duration 19 hours 29 minutes 29 seconds
Course
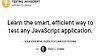
Testing JavaScript with Kent C. DoddsKent C. Dodds
Category: JavaScript, Cypress, Other (QA)
Duration 14 hours 11 minutes 26 seconds
Course
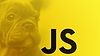
Learn JavaScript: Full-Stack from Scratchudemy
Category: JavaScript, MongoDB
Duration 27 hours 6 minutes 45 seconds
Course
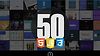
50 Projects In 50 Days - HTML, CSS & JavaScriptudemyBrad Traversy
Category: JavaScript, HTML, CSS
Duration 18 hours 13 minutes 45 seconds
Course
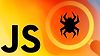
Mastering JavaScript Unit Testingcodewithmosh (Mosh Hamedani)
Category: JavaScript
Duration 3 hours 51 minutes 31 seconds
Course
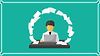
JavaScript & LeetCode | The Ultimate Interview Bootcampudemy
Category: JavaScript, Preparing for an interview
Duration 4 hours 49 minutes 58 seconds
Course
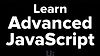
Advanced JavaScriptui.dev (ex. Tyler McGinnis)
Category: JavaScript
Duration 3 hours 5 minutes 51 seconds
Course