The No-BS Solution for Enterprise-Ready Next.js Applications
Next.js is one of the most popular frameworks for building web applications. It is fast, flexible, and underpins some of the largest websites on the internet.
However, when it comes to creating a production-ready application, setting up a project in Next.js can become quite a challenge.
With the introduction of App Router and React Server Components, there have been significant changes in how applications are built and structured. You will also need to make decisions regarding styling approaches, data loading strategies, testing, and CI/CD pipeline. This can be quite complex.
More
Here are just some of the challenges that developers face with Next.js:
Routing Uncertainty:
Which router to choose - App Router or the deprecated pages router? What trade-offs need to be considered?
Complexities with Components:
When should you use client-side components versus React Server Components? How to optimally handle context and data exchange?
Performance Issues:
Why does your application seem slow? How can you optimize data loading and rendering?
Authentication:
How to safely manage user authentication and sessions?
Deployment:
How to ensure reliable and secure deployment of applications on Next.js with minimal downtime and errors?
Scalability:
How to prepare your Next.js application for increased user load?
Working with Next.js shouldn't be confusing. Whether you're a beginner or an experienced developer looking to master the latest updates, Pro NextJS will teach you everything you need to confidently create web applications.
This series of self-guided master classes contains deep knowledge, real-life examples, and practical advice to help you understand all the nuances of working with Next.js.
What the Course Includes
Introduction to Next.js
In the first master class, we will touch on all the most important aspects of working with Next.js. Instead of a typical "To Do List" application, you will create a full-fledged application similar to ChatGPT. Here's what we will cover:
- Project Setup and Deployment: Configuring a Next.js application with TypeScript, ESLint, and Tailwind CSS, along with continuous deployment on Vercel.
- Routing and Layouts: Using App Router to create routes and layouts that affect data loading and navigation.
- Authentication: Implementing user authentication and session management.
- AI Streaming Responses: Building a dynamic chat with connection to the OpenAI API and real-time streaming responses.
- Database Integration: Storing chat data in the Vercel Postgres database.
Styling Next.js Applications
The development of styling methods for web applications continues to advance, but with the advent of App Router and React Server Components, new challenges have emerged. This module will immerse you in popular methods for creating responsive and theme-ready interfaces in Next.js. Specifically:
- CSS Modules: A classic but sometimes cumbersome way to style components.
- Tailwind CSS: An approach using utility classes that requires some configuration steps to maintain readability.
- StyleX: A library from Meta for detailed management of components, design tokens, and themes.
- Pigment CSS: A styling library from the Material UI team optimized for React Server Components.
- Emotion: Although it's not the most modern approach, many applications still use Emotion for styling. Learn how to configure the library to support App Router.
Project Infrastructure
For modern Next.js applications, there are many tools, frameworks, and processes - especially when working in a team. This course module offers best practices for building a reliable project infrastructure. Here's what you will learn:
- Code Style and Consistency: Using ESLint and Prettier to maintain code style.
- Component Organization: Weighing the pros and cons of various project organization strategies.
- Creating a Component Library: Working with Storybook to create and document components.
- Testing: Writing tests using Jest, Vitest, Cypress, and Playwright.
- Monorepo Management: Turborepo for code sharing and dependency management.
- Client and Server Components: In-depth study of client components and RSC, including context handling and data exchange strategies.
Client and Server Interaction
After deploying a Next.js application, there are many factors affecting performance and user experience. This module covers advanced client-server interaction topics:
- Caching Strategies: Various data caching and revalidation options, including API route support and cache management with tags.
- File Uploads: Using server actions and API routes to handle local and cloud file uploads.
- System Architecture: Exploring different architectural patterns for client-server interaction, including Backend-for-Frontend, server actions, and token handling.
- Advanced Server Actions: Building a CDN simulator, intercepting client requests, and creating data streaming solutions.
- Granular Suspense: Building a stock data application using Suspense to improve responsiveness.
From project setup to deployment, styling, and infrastructure management, Pro NextJS covers everything. You will learn through practical examples and assignments to boost your confidence in working with Next.js.
Start now!
Watch Online The No-BS Solution for Enterprise-Ready Next.js Applications
# | Title | Duration |
---|---|---|
1 | Welcome to Pro Next.js | 05:55 |
2 | Why You Should Choose Next.js | 04:38 |
3 | When Not to Use Next.js | 02:46 |
4 | The React, Next.js, and Vercel Controversy | 04:17 |
5 | Create and Deploy a Next.js Application | 07:57 |
6 | An Overview of the First Application | 01:50 |
7 | Create Your First Route | 01:41 |
8 | Add a Header Using Layout | 03:33 |
9 | Choosing a SSR Framework or a SPA System | 05:00 |
10 | Client vs. Server Components in Next.js | 12:45 |
11 | Add Authentication with NextAuth.js | 14:27 |
12 | NextAuth v5 Update | 03:03 |
13 | Composition in Next.js: Understanding Client and Server Components | 07:39 |
14 | Intro to Server Actions | 15:01 |
15 | Add Interactivity with Next.js Server Actions | 08:58 |
16 | Store Chat Data in a Database | 11:05 |
17 | File-Based Routing with App Router | 12:46 |
18 | Adding Parameterized Routes to a Next.js App | 09:47 |
19 | Access Data with React Server Components | 08:21 |
20 | Implement Parallel Routes for a Chat Menu Sidebar | 06:47 |
21 | Implement Streaming AI Responses | 07:30 |
22 | What You've Learned So Far | 00:39 |
23 | Styling Next.js App Router Applications | 03:18 |
24 | Create a Layout with CSS Modules | 04:18 |
25 | Refine the Layout with CSS Modules | 08:52 |
26 | Getting Started with Tailwind CSS | 03:47 |
27 | Configure Container Queries with Tailwind CSS | 03:04 |
28 | Combine CSS Modules with Tailwind CSS | 02:33 |
29 | Intro to Meta's StyleX | 06:25 |
30 | Finish Styling with StyleX | 03:03 |
31 | Styling with Material UI's Pigment CSS | 04:36 |
32 | Finish Styling with Pigment CSS | 04:41 |
33 | Styling with Emotion | 01:41 |
34 | Comparing Emotion with Tailwind and Pigment | 03:16 |
35 | Introduction to Component Libraries | 02:21 |
36 | shadcn/ui - Blog App Overview | 11:06 |
37 | Park UI | 07:11 |
38 | Bootstrap | 05:30 |
39 | Ant Design | 06:07 |
40 | Material UI | 06:51 |
41 | Chakra UI | 05:33 |
42 | Wedges | 04:57 |
43 | NextUI | 06:58 |
44 | Mantine | 05:45 |
45 | Building a Strong Foundation | 01:35 |
46 | Setting up ESLint and Prettier | 05:50 |
47 | Organizing Component Files | 05:54 |
48 | Organizing Components into Directories | 01:55 |
49 | Importing Component Files | 02:47 |
50 | Component Locations | 07:09 |
51 | Setting up Storybook with Next.js | 04:50 |
52 | Testing with Jest | 07:33 |
53 | Testing Async RSCs with Jest | 03:42 |
54 | Testing with Vitest | 07:26 |
55 | Testing Async RSCs with Vitest | 02:11 |
56 | End-to-End Testing with Cypress | 05:29 |
57 | E2E Testing with Playwright | 04:56 |
58 | Check Bundle Size with GitHub Actions and Husky | 14:55 |
59 | Should You Use a Monorepo? | 04:04 |
60 | Creating a Next.js App in a Turborepo Monorepo | 06:17 |
61 | Storybook in a Turborepo Monorepo | 12:44 |
62 | Naming and Organizing Server and Client Components | 04:26 |
63 | Lego Components | 08:00 |
64 | Embrace the JS, Next.js, and React Ecosystem | 00:42 |
65 | Caching with the Next.js App Router | 01:00 |
66 | The Full Route Cache | 03:34 |
67 | Dynamic Routes in Next.js | 02:03 |
68 | Automatic and Manual Revalidation | 03:13 |
69 | Next.js API Route Caching | 02:28 |
70 | Data Caching and Revalidation with React Server Components | 03:52 |
71 | Cache-busting with Tags | 05:18 |
72 | The Next.js Router Cache | 09:03 |
73 | Understanding the Example Monorepo Structure | 03:32 |
74 | Intro to Systems Architecture | 05:42 |
75 | The API Route Variant of Local Systems | 03:02 |
76 | Building with Local Server Actions in Next.js | 02:30 |
77 | BFF Architecture with GraphQL | 05:15 |
78 | Backend-for-Frontend (BFF) Architecture with Server Actions | 03:20 |
79 | The API Variant of Backend-for-Frontend Architecture | 03:57 |
80 | BFF Pattern with gRPC (TwirpScript) | 04:14 |
81 | BFF Architecture with tRPC | 05:02 |
82 | Server Architecture with an External API Domain | 05:26 |
83 | Proxying External Systems with Next.js | 04:06 |
84 | Token Variation of the External Systems Architecture | 06:36 |
85 | Token Variation of the External Systems Architecture | 03:13 |
86 | Adding Suspense to the Application | 01:15 |
87 | Granular Suspense in React | 03:11 |
88 | DIY Streaming with Server Actions | 05:16 |
89 | Cached Server Actions in Next.js | 16:09 |
90 | File Uploads in Next.js App Router Apps | 07:31 |
Similar courses to The No-BS Solution for Enterprise-Ready Next.js Applications
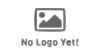
Next.js From Scratch 2024Brad Traversy
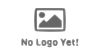
Real-Time Collaborative Apps with Next.js and Supabasefullstack.io
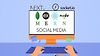
MERN Stack React, Socket io, Next.js Express,MongoDb, Nodejsudemy
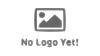
Next.JS with Sanity CMS - Serverless Blog App (w/ Vercel)udemy
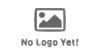
Testing Next.js Apps with Jest, Testing Library and Cypressudemy
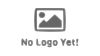
React Simplified - Next.jswebdevsimplified.com
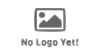
Next.js & React with ChatGPT - Development Guide (2023)udemy
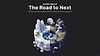