Testing Next.js Apps with Jest, Testing Library and Cypress
More
Comprehensive Next.js App Testing
This course teaches how to test all aspects of a Next.js application, including:
UI unit tests
API unit tests
Next.js routes
Cached pages
Data updates (both to the cache and via SWR refresh interval)
Authentication
End-to-End tests for a complete user flow
The course uses a cross-section of testing technologies, featuring:
Jest
React Testing Library
Mock Service Worker
Cypress
Cypress Testing Library
You'll also learn a wide array of testing techniques, such as:
Using a test database
Environment variables
Jest module mocking
Testing definitions and tradeoffs (not necessarily a technique, but helpful in making decisions about what to test!)
Practice what you've learned
The course includes "code quizzes" -- challenges to write code based on the concepts you've just learned. For more concept-heavy sections (such as testing definitions and guidelines) the course provides multiple-choice quizzes.
Test a pre-written app
A Next.js app has already been written for the course, so the course content can focus on testing. The course tests a popular concert venue app called... Popular Concert Venue (the owners were apparently running low on creativity when they named the place). The app features shows from bands such as The Joyous Nun Riot and Avalanche of Cheese. Randomized, mad-libs style band descriptions and images add to the fun.
Watch Online Testing Next.js Apps with Jest, Testing Library and Cypress
# | Title | Duration |
---|---|---|
1 | Introduction | 03:11 |
2 | Course Technologies and Prerequisites | 03:09 |
3 | create-next-app Using with-jest Example | 03:21 |
4 | First Next.js Test | 05:43 |
5 | Course Features: Code Quizzes and Side Notes | 02:39 |
6 | Guide to the Rest of the Course | 05:15 |
7 | Introduction to Testing | 03:14 |
8 | Types of Tests | 04:34 |
9 | What to Test | 04:05 |
10 | Test Redundancy | 04:58 |
11 | Test Granularity Guidelines | 03:22 |
12 | My View on Snapshot Tests (hint: not my thing) | 03:06 |
13 | Summary: Testing Definitions and Philosophy | 02:28 |
14 | Introduction to Course App and Next.js Data Fetching | 01:14 |
15 | Demo of Course App | 03:19 |
16 | Next.js Data Fetching Strategies | 10:49 |
17 | Installing the Course App | 04:39 |
18 | Course App Code Notes | 05:28 |
19 | Introduction and Technologies | 03:46 |
20 | Testing a Static Page | 07:19 |
21 | Testing SSG Props | 07:56 |
22 | Code Quiz! Band Component Error | 02:59 |
23 | Decisions and Guidelines for the Tests So Far | 04:31 |
24 | Introduction to Mock Service Worker | 02:59 |
25 | Setting up MSW with Next.js | 05:13 |
26 | Adding a MSW Handler | 07:38 |
27 | Using MSW for a Test: Reservation Component | 06:13 |
28 | Code Quiz! User Reservations | 06:32 |
29 | Different MSW Responses per Test | 10:20 |
30 | Code Quiz! Different MSW Responses per Test | 03:19 |
31 | Summary: UI Testing | 02:40 |
32 | Introduction to Test Databases | 03:40 |
33 | Creating a Test Database | 03:29 |
34 | Environment Variables | 07:17 |
35 | Creating the Test DB and Environment Variables | 07:59 |
36 | Create a Method to Reset the Test DB | 08:54 |
37 | Summary: Setting up a Test Database | 01:28 |
38 | Introducing testing Next.js Routes and Cypress | 02:57 |
39 | OPTIONAL: Introduction to Cypress | 02:07 |
40 | Setting up Next.js for Cypress | 05:47 |
41 | Setting up Cypress | 09:05 |
42 | First Cypress Test: Static Route | 06:39 |
43 | Code Quiz! Static Route | 03:03 |
44 | Testing Dynamic Routes | 02:21 |
45 | Resetting the Database in Cypress | 07:10 |
46 | Test Dynamic Route that was Present at Build Time | 03:36 |
47 | Code Quiz! Test route that does not exist | 02:44 |
48 | Test Route Created after Build | 07:09 |
49 | Run all Cypress and Jest Tests | 05:44 |
50 | Summary: Testing Next.js Routes and Cypress | 02:33 |
51 | Introduction to Testing ISR and Data Updates | 02:43 |
52 | Testing Data Comes from ISR Cache | 04:20 |
53 | First ISR Cache Test | 05:14 |
54 | Code Quiz! ISR Bands Page | 04:03 |
55 | Updating the ISR Cache on Demand | 05:28 |
56 | Writing a Cypress Plug-In for Environment Variable | 03:44 |
57 | ISR Revalidation Test | 09:55 |
58 | Clearing the ISR Cache | 11:38 |
59 | Adding ISR Cache Clearing to Test | 04:50 |
60 | Code Quiz! Revalidate ISR Cache | 04:45 |
61 | SWR Revalidate on Interval | 04:17 |
62 | Testing Revalidate on Interval | 11:18 |
63 | Code Quiz! Revalidate on Interval | 04:12 |
64 | Summary: Testing ISR and Data Updates | 02:13 |
65 | Introduction to Testing Authentication | 03:23 |
66 | Auth Wrapper in Course App | 06:23 |
67 | Adding Sign-In Details to Cypress | 04:13 |
68 | Testing Success Flow with Auth Wrapper | 11:34 |
69 | Code Quiz! Authentication Failure followed by Success | 03:56 |
70 | Parametrizing Protected Page Tests | 05:22 |
71 | Authenticating Programmatically | 09:10 |
72 | Code Quiz! Authenticating Programmatically | 05:40 |
73 | Ticket Purchase End-to-End Test | 03:22 |
74 | Summary: Testing Authentication | 01:49 |
75 | Introduction to API Tests | 05:30 |
76 | First API Test | 08:33 |
77 | Fixing Test Errors: Polyfill, resetDB, ignore DB directory in watchlist | 08:15 |
78 | Testing a Route with a URL Param | 06:58 |
79 | Testing a POST Route | 06:55 |
80 | Mocking utils Module for Authentication | 06:58 |
81 | Write Test using Mocked Module | 05:31 |
82 | Code Quiz! User with No Reservations | 03:06 |
83 | Code Quiz! Post a Reservation | 06:10 |
84 | Fixing Issues with Parallel Tests using Shared Database | 05:45 |
85 | Updating Mock Function Return Value: Testing Unauthorized Request | 05:02 |
86 | Code Quiz! Updating Mock Function Return Value | 02:15 |
87 | Testing Routes with Query String Params | 05:10 |
88 | Code Quiz! Query String Params | 01:59 |
89 | Summary: Testing Next.js APIs | 03:00 |
90 | Congratulations and Thank You! | 01:04 |
Similar courses to Testing Next.js Apps with Jest, Testing Library and Cypress
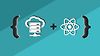
Server side rendering with Next + Reactudemy
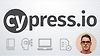
Cypress V6- UI Automation Testing + API Testing + Frameworksudemy
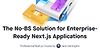
The No-BS Solution for Enterprise-Ready Next.js ApplicationsJack Herrington
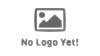
Mastering Next.js - 50+ Lesson Video Course on React and Nextmasteringnuxt.com
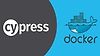
Automated Software Testing with Cypressudemy
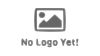
Ecommerce on the Jamstack with Snipcart, Next.js, & WordPressleveluptutorials
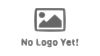
Mastering Next.js 13 with TypeScriptcodewithmosh (Mosh Hamedani)
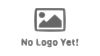
Complete Next.js Developer in 2023: Zero to Masteryzerotomastery.io
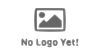