Test-Driven Laravel
21h 48m 56s
English
Paid
The biggest objective of this course is to teach you how to TDD something real; not just another cookie-cutter to-do app.
Read more about the course
We cover fundamentals like:
- What test should you write first
- Organizing your test suite
- Feature tests vs. unit tests
- Testing validation rules
- Testing events and background jobs
- Working with test databases
- Speeding up your tests with test doubles
...as well as hard topics, like:
- Testing code that interacts with third-party services
- Writing your own test doubles from scratch
- Testing automated payouts with Stripe Connect
- Testing the sending of mass emails
- How to test race conditions
- Testing file uploads and server side image processing
Test-Driven Laravel teaches you how to design a solid test suite for a real-world, marketable product that you could actually charge money for.
Watch Online Test-Driven Laravel
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | What Do We Build First? | 11:30 |
2 | Sketching out Our First Test | 09:06 |
3 | Getting to Green | 16:42 |
4 | Unit Testing Presentation Logic | 11:03 |
5 | Refactoring for Speed | 07:16 |
6 | Hiding Unpublished Concerts | 06:56 |
7 | Testing Query Scopes | 05:50 |
8 | Factory States | 04:10 |
9 | Introducing the Next Feature | 01:02 |
10 | Browser Testing vs Endpoint Testing | 09:47 |
11 | Outlining the First Purchasing Test | 06:23 |
12 | Faking the Payment Gateway | 12:23 |
13 | Adding Tickets to Orders | 05:32 |
14 | Encapsulating Relationship Logic in the Model | 05:16 |
15 | Getting Started with Validation Testing | 06:56 |
16 | Reducing Duplication with Custom Assertions | 05:30 |
17 | Handling Failed Charges | 07:00 |
18 | Preventing Ticket Sales to Unpublished Concerts | 06:40 |
19 | Outlining the First Test Case | 03:46 |
20 | Adding Tickets to Concerts | 08:20 |
21 | Refusing Orders When There Are No More Tickets | 09:29 |
22 | Finishing the Feature Test | 04:25 |
23 | Cancelling Failed Orders | 05:59 |
24 | Refactoring and Redundant Test Coverage | 07:49 |
25 | Cleaning Up Our Tests | 11:57 |
26 | Asserting Against JSON Responses | 07:32 |
27 | Returning Order Details | 06:22 |
28 | This Design Sucks | 04:39 |
29 | Persisting the Order Amount | 05:05 |
30 | Removing the Need to Cancel Orders | 06:01 |
31 | Preparing for Extraction | 07:06 |
32 | Extracting a Named Constructor | 04:46 |
33 | Precomputing the Order Amount | 05:23 |
34 | Uncovering a New Domain Object | 05:11 |
35 | You Might Not Need a Mocking Framework | 03:48 |
36 | Uh Oh, a Race Condition! | 01:43 |
37 | Requestception | 04:17 |
38 | Hooking into Charges | 05:38 |
39 | Uh Oh, a Segfault! | 03:01 |
40 | Replicating the Failure at the Unit Level | 05:04 |
41 | Reserving Individual Tickets | 07:00 |
42 | Reserved Means Reserved! | 03:58 |
43 | That Guy Stole My Tickets! | 05:59 |
44 | Cancelling Reservations | 05:46 |
45 | Refactoring Mocks to Spies | 06:47 |
46 | A Change in Behavior | 05:53 |
47 | Deleting Stale Tests | 05:38 |
48 | Cleaning up a Loose Variable | 06:48 |
49 | Moving the Email to the Reservation | 07:28 |
50 | Refactoring "Long Parameter List" Using "Preserve Whole Object" | 09:50 |
51 | Green with Feature Envy | 06:12 |
52 | Avoiding Service Classes with Method Injection | 09:04 |
53 | Generating a Valid Payment Token | 10:01 |
54 | Retrieving the Last Charge | 04:05 |
55 | Making a Successful Charge | 04:37 |
56 | Dealing with Lingering State | 12:18 |
57 | Don't Mock What You Don't Own | 09:41 |
58 | Using Groups to Skip Integration Tests | 02:19 |
59 | Handling Invalid Payment Tokens | 03:59 |
60 | The Moment of Truth | 04:28 |
61 | When Interfaces Aren't Enough | 04:29 |
62 | Refactoring Towards Duplication | 12:38 |
63 | Capturing Charges with Callbacks | 08:10 |
64 | Making the Tests Identical | 07:42 |
65 | Extracting a Contract Test | 07:42 |
66 | Extracting the Failure Case | 08:24 |
67 | Upgrading to Laravel 5.4 | 04:49 |
68 | Removing the BrowserKit Dependency | 10:26 |
69 | Sketching Out Order Confirmations | 07:52 |
70 | Driving out the Endpoint | 05:21 |
71 | Asserting Against View Data | 05:46 |
72 | Extracting a Finder Method | 06:51 |
73 | Making Static Data Real | 08:49 |
74 | Deciding What to Test in a View | 08:24 |
75 | Decoupling Data from Presentation | 05:11 |
76 | Fixing the Test Suite | 06:28 |
77 | Stubbing the Interface | 06:28 |
78 | Updating Our Unit Tests | 05:16 |
79 | Confirmation Number Characteristics | 04:48 |
80 | Testing the Confirmation Number Format | 07:29 |
81 | Ensuring Uniqueness | 08:44 |
82 | Refactoring to a Facade | 05:59 |
83 | Promoting Charges to Objects | 17:49 |
84 | Leveraging Our Contract Tests | 11:02 |
85 | Storing Charge Details with Orders | 08:01 |
86 | Deleting More Stale Code | 10:27 |
87 | Feature Test and JSON Updates | 08:27 |
88 | Claiming Tickets When Creating Orders | 09:12 |
89 | Assigning Codes When Claiming Tickets | 10:54 |
90 | The Birthday Problem | 11:55 |
91 | Integrating Hashids | 11:44 |
92 | Dealing with Out of Sync Mocks | 04:21 |
93 | Wiring It All Together | 05:19 |
94 | Ready to Demo | 02:52 |
95 | Using a Fake to Intercept Email | 10:35 |
96 | Testing Mailable Contents | 10:39 |
97 | Cleanup and Demo | 02:40 |
98 | Testing the Login Endpoint | 11:26 |
99 | Should You TDD Simple Templates? | 04:42 |
100 | Namespacing Our Test Suite | 06:31 |
101 | Getting Started with Laravel Dusk | 05:22 |
102 | QA Testing the Login Flow | 06:26 |
103 | Preventing Guests from Adding Concerts | 06:24 |
104 | Adding a Valid Concert | 14:20 |
105 | Validation and Redirects | 09:49 |
106 | Converting Empty Strings to Null | 04:21 |
107 | Reducing Noise with Form Factories | 09:13 |
108 | Connecting Promoters and Concerts | 06:49 |
109 | Autopublishing New Concerts | 05:46 |
110 | Asserting Against View Objects | 12:09 |
111 | Avoiding Sort-Sensitive Tests | 05:47 |
112 | Refactoring Assertions with Macros | 11:39 |
113 | Viewing the Update Form | 05:29 |
114 | The First Update Test | 09:27 |
115 | Driving Out Basic Concert Updates | 09:14 |
116 | Restricting Updates to Unpublished Concerts | 11:31 |
117 | Storing the Intended Ticket Quantity | 06:26 |
118 | Updating the Other Tests | 05:25 |
119 | Refactoring Away Some Test Duplication | 07:52 |
120 | Creating Tickets at Time of Publish | 08:28 |
121 | Custom Factory Classes | 07:44 |
122 | Discovering a New Resource | 07:35 |
123 | Creating Published Concerts | 11:12 |
124 | Adding Concerts without Publishing | 04:03 |
125 | Pushing Logic Out of the View | 08:47 |
126 | More Custom Assertion Fun | 06:20 |
127 | Calculating Tickets Sold | 08:22 |
128 | Making the Progress Bar Work | 08:58 |
129 | Total Revenue and a Relationship Bug | 08:40 |
130 | Creating a Custom OrderFactory | 11:28 |
131 | Asserting Against Sort Order | 12:15 |
132 | Splitting Large Tests | 03:41 |
133 | Storing Messages for Attendees | 12:31 |
134 | Confirming That a Job Was Dispatched | 09:27 |
135 | Unit Testing the Job | 18:29 |
136 | Refactoring for Robustness | 11:16 |
137 | Mailable Testing Refresher and Demo | 04:11 |
138 | Upgrading to Laravel 5.5 | 07:41 |
139 | Faking Uploads and File Systems | 10:43 |
140 | Storing Files and Comparing Content | 09:16 |
141 | Validating Poster Images | 07:45 |
142 | Optional Files and the Null Object Pattern | 05:39 |
143 | Testing Events | 10:41 |
144 | Testing the Event Listener | 11:42 |
145 | Resizing the Posted Image | 12:26 |
146 | Optimizing the Image Size | 10:51 |
147 | Upgrading Laravel and Deleting Some Code | 03:40 |
148 | Viewing an Unused Invitation | 10:25 |
149 | Viewing Used or Invalid Invitations | 07:29 |
150 | Registering with a Valid Invitation | 12:46 |
151 | Registering with an Invalid Invitation | 05:20 |
152 | Validating Promoter Registration | 05:54 |
153 | Testing a Console Command | 17:40 |
154 | Sending Promoters an Invitation Email | 05:52 |
155 | Test-Driving the Email Contents | 07:53 |
156 | Getting Cozy with Stripe Connect | 10:55 |
157 | Authorizing with Stripe | 10:05 |
158 | Exchanging Tokens | 17:44 |
159 | Unit Testing Middleware | 13:29 |
160 | Testing Callbacks with Invokables | 09:43 |
161 | Testing That Middleware Is Applied | 08:16 |
162 | Updating Factories and a Speed Trick | 03:30 |
163 | Total Charges for a Specific Account | 10:43 |
164 | Paying Promoters Directly | 07:47 |
165 | Splitting Payments with Stripe | 16:53 |
166 | It's Alive | 04:36 |
Similar courses to Test-Driven Laravel
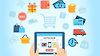
Build an e-commerce platformCodecourse
Category: Laravel
Duration 14 hours 19 minutes 18 seconds
Course
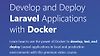
Develop and Deploy Laravel Applications with DockerAndrew Schmelyun
Category: Laravel
Duration 2 hours 29 minutes 56 seconds
Course
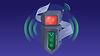
Real-Time Data with Laravel Reverb and Vue.jsvueschool.io
Category: Vue, Laravel
Duration 44 minutes 56 seconds
Course
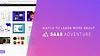
SAAS Adventure - Learn to Create your own SAASsaasadventure.io
Category: JavaScript, Laravel
Duration 8 hours 37 minutes 3 seconds
Course
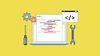
Laravel with React JS - Build Twitter Like Real Time Web Appudemy
Category: React.js, Laravel
Duration 4 hours 56 minutes 37 seconds
Course
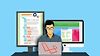
Ultimate Laravel Course 2018 (PayPal, Webshop, RESTful API)udemy
Category: Laravel
Duration 9 hours 9 minutes 37 seconds
Course
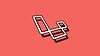
PHP with Laravel for beginners - Become a Master in Laraveludemy
Category: Laravel
Duration 42 hours 35 minutes 23 seconds
Course
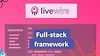
Laravel LivewireCaleb Porziolaravel-livewire.com
Category: Laravel
Duration 11 hours 29 minutes 28 seconds
Course
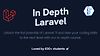
In Depth Laravel Course (2023 Version)Sarthak Shrivastava
Category: Laravel
Duration 35 hours 46 minutes 3 seconds
Course
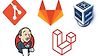
DevOps Project: CICD with Git GitLab Jenkins and Laraveludemy
Category: Laravel
Duration 4 hours 47 minutes 21 seconds
Course