React - The Complete Guide 2024
Learn React or dive deeper into it. Learn the theory, solve assignments, practice in demo projects and build one big application which is improved throughout the course: The Burger Builder!
More
More details please!
JavaScript is the major driver of modern web applications since it's the only programming language which runs in the browser and hence allows you to provide highly reactive apps. You'll be able to achieve mobile-app like user experiences in the web.
But using JavaScript can be challenging - it quickly becomes overwhelming to create a nice web app with vanilla JavaScript and jQuery only.
React to the rescue!
React is all about components - basically custom HTML elements - with which you can quickly build amazing and powerful web apps. Just build a component once, configure it to your needs, dynamically pass data into it (or listen to your own events!) and re-use it as often as needed.
Need to display a list of users in your app? It's as simple as creating a "User" component and outputting it as often as needed.
This course will start at the very basics and explain what exactly React is and how you may use it (and for which kind of apps). Thereafter, we'll go all the way from basic to advanced. We'll not just scratch the surface but dive deeply into React as well as popular libraries like react-router and Redux.
By the end of the course, you can build amazing React (single page) applications!
A detailed list with the course content can be found below.
Who's teaching you in this course?
My name is Maximilian Schwarzmüller, I'm a freelance web developer and worked with React in many projects. I'm also a 5-star rated instructor here on Udemy. I cover React's most popular alternatives - Vue and Angular - as well as many other topics. I know what I'm talking about and I know where the pain points can be found.
It's my goal to get you started with React as quick as possible and ensure your success. But I don't just focus on students getting started. I want everyone to benefit from my courses, that's why we'll dive deeply into React and why I made sure to also share knowledge that's helpful to advanced React developers.
Is this course for you?
This course is for you if ...
- ...you're just getting started with frontend/ JavaScript development and only got the JS basics set (no prior React or other framework experience is required!)
- ...you're experienced with Angular or Vue but want to dive into React
- ...know the React basics but want to refresh them and/ or dive deeper
- ...already worked quite a bit with React but want to dive deeper and see it all come together in a bigger app
What should you bring to succeed in that course?
- HTML + CSS + JavaScript knowledge is required. You don't need to be an expert but the basics need to be set
- NO advanced JavaScript knowledge is required, though you'll be able to move even quicker through the course if you know next-gen JavaScript features like ES6 Arrow functions. A short refresher about the most important next-gen features is provided in the course though.
What's inside the course?
- The "What", "Why" and "How"
- React Basics (Base features, syntax and concepts)
- Managing state with class-based components and React Hooks
- How to output lists and conditional content
- Styling of React components
- A deep dive into the internals of React and advanced component features
- How to access Http content from within React apps (AJAX)
- Redux, Redux, Redux ... from basics to advanced!
- Forms and form validation in React apps
- Authentication
- An introduction to unit testing
- An introduction to Next.js
- React app deployment instructions
- ...and much more!
Who this course is for:
- Students who want to learn how to build reactive and fast web apps
- Anyone who's interested in learning an extremely popular technology used by leading tech companies like Netflix
- Students who want to take their web development skills to the next level and learn a future-proof technology
What you'll learn:
- Build powerful, fast, user-friendly and reactive web apps
- Provide amazing user experiences by leveraging the power of JavaScript with ease
- Apply for high-paid jobs or work as a freelancer in one the most-demanded sectors you can find in web dev right now
- Learn React Hooks & Class-based
Watch Online React - The Complete Guide 2024
# | Title | Duration |
---|---|---|
1 | Welcome to the Course | 01:09 |
2 | What Is React? And Why Would You Use It? | 02:59 |
3 | ReactJS vs Vanilla JavaScript | 10:58 |
4 | Editing Our First React App | 04:23 |
5 | About the Course | 02:56 |
6 | One Course, Two Paths | 03:09 |
7 | How To Get The Most Out Of This Course | 05:25 |
8 | How To Create New React Projects | 07:11 |
9 | Why Do We Need A Special Project Setup? | 02:52 |
10 | Module Introduction | 01:50 |
11 | Starting Project | 01:01 |
12 | Adding JavaScript To A Page & How React Projects Differ | 06:58 |
13 | React Projects Use a Build Process | 08:05 |
14 | "import" & "export" | 12:05 |
15 | Revisiting Variables & Values | 07:02 |
16 | Revisiting Operators | 02:34 |
17 | Revisiting Functions & Parameters | 08:15 |
18 | Arrow Functions | 02:12 |
19 | Revisiting Objects & Classes | 06:08 |
20 | Arrays & Array Methods like map() | 11:11 |
21 | Destructuring | 05:17 |
22 | The Spread Operator | 03:14 |
23 | Revisiting Control Structures | 05:29 |
24 | Manipulating the DOM - Not With React! | 00:53 |
25 | Using Functions as Values | 07:23 |
26 | Defining Functions Inside Of Functions | 01:56 |
27 | Reference vs Primitive Values | 04:45 |
28 | Module Introduction | 01:49 |
29 | It's All About Components! [Core Concept] | 06:08 |
30 | Setting Up The Starting Project ( | 03:07 |
31 | JSX & React Components [Core Concept] | 04:43 |
32 | Creating & Using a First Custom Component | 05:53 |
33 | How React Handles Components & How It Builds A "Component Tree" [Core Concept] | 08:22 |
34 | Using & Outputting Dynamic Values [Core Concept] | 06:02 |
35 | Setting HTML Attributes Dynamically & Loading Image Files | 04:43 |
36 | Making Components Reusable with Props [Core Concept] | 09:07 |
37 | Alternative Props Syntaxes | 06:45 |
38 | Best Practice: Storing Components in Files & Using a Good Project Structure | 06:33 |
39 | Storing Component Style Files Next To Components | 06:16 |
40 | Component Composition: The special "children" Prop [Core Concept] | 08:07 |
41 | Reacting to Events [Core Concept] | 06:46 |
42 | Passing Functions as Values to Props | 06:44 |
43 | Passing Custom Arguments to Event Functions | 05:37 |
44 | How NOT to Update the UI - A Look Behind The Scenes of React [Core Concept] | 05:31 |
45 | Managing State & Using Hooks [Core Concept] | 10:29 |
46 | Deriving & Outputting Data Based on State | 04:51 |
47 | Rendering Content Conditionally | 08:04 |
48 | CSS Styling & Dynamic Styling | 05:13 |
49 | Outputting List Data Dynamically | 07:41 |
50 | Module Summary | 06:06 |
51 | Module Introduction | 01:27 |
52 | You Don't Have To Use JSX! | 04:38 |
53 | Working with Fragments | 05:41 |
54 | When Should You Split Components? | 03:15 |
55 | Splitting Components By Feature & State | 06:55 |
56 | Problem: Props Are Not Forwarded To Inner Elements | 06:45 |
57 | Forwarding Props To Wrapped Elements | 03:47 |
58 | Working with Multiple JSX Slots | 08:43 |
59 | Setting Component Types Dynamically | 08:45 |
60 | Setting Default Prop Values | 02:10 |
61 | Onwards To The Next Project & Advanced Concepts | 02:18 |
62 | Not All Content Must Go Into Components | 05:11 |
63 | New Project: First Steps Towards Our Tic-Tac-Toe Game | 03:59 |
64 | Concept Repetition: Splitting Components & Building Reusable Components | 04:14 |
65 | Concept Repetition: Working with State | 07:58 |
66 | Component Instances Work In Isolation! | 02:04 |
67 | Conditional Content & A Suboptimal Way Of Updating State | 04:55 |
68 | Best Practice: Updating State Based On Old State Correctly | 07:05 |
69 | User Input & Two-Way-Binding | 07:43 |
70 | Rendering Multi-Dimensional Lists | 07:41 |
71 | Best Practice: Updating Object State Immutably | 08:45 |
72 | Lifting State Up [Core Concept] | 09:40 |
73 | Avoid Intersecting States! | 05:23 |
74 | Prefer Computed Values & Avoid Unnecessary State Management | 06:18 |
75 | Deriving State From Props | 07:23 |
76 | Sharing State Across Components | 04:13 |
77 | Reducing State Management & Identifying Unnecessary State | 05:53 |
78 | Disabling Buttons Conditionally | 02:38 |
79 | Outsourcing Data Into A Separate File | 04:29 |
80 | Lifting Computed Values Up | 05:20 |
81 | Deriving Computed Values From Other Computed Values | 05:30 |
82 | Tic-Tac-Toe Game: The "Game Over" Screen & Checking for a Draw | 05:41 |
83 | Why Immutability Matters - Always! | 05:29 |
84 | When NOT To Lift State Up | 05:21 |
85 | An Alternative To Lifting State Up | 03:17 |
86 | Final Polishing & Improving Components | 05:16 |
87 | Module Introduction & A Challenge For You! | 05:10 |
88 | Adding a Header Component | 04:49 |
89 | Getting Started with a User Input Component | 05:08 |
90 | Handling Events & Using Two-Way-Binding | 10:29 |
91 | Lifting State Up | 09:00 |
92 | Computing Values & Properly Handling Number Values | 05:42 |
93 | Outputting Results in a List & Deriving More Values | 09:03 |
94 | Outputting Content Conditionally | 04:25 |
95 | Module Introduction & Starting Project | 03:58 |
96 | Splitting CSS Code Across Multiple Files | 03:23 |
97 | Styling React Apps with Vanilla CSS - Pros & Cons | 02:33 |
98 | Vanilla CSS Styles Are NOT Scoped To Components! | 03:32 |
99 | Styling React Apps with Inline Styles | 06:25 |
100 | Dynamic & Conditional Inline Styles | 03:28 |
101 | Dynamic & Conditional Styling with CSS Files & CSS Classes | 05:42 |
102 | Scoping CSS Rules with CSS Modules | 09:52 |
103 | Introducing "Styled Components" (Third-party Package) | 08:04 |
104 | Creating Flexible Components with Styled Components | 04:48 |
105 | Dynamic & Conditional Styling with Styled Components | 11:08 |
106 | Styled Components: Pseudo Selectors, Nested Rules & Media Queries | 07:16 |
107 | Creating Reusable Components & Component Combinations | 09:54 |
108 | Introducing Tailwind CSS For React App Styling | 11:27 |
109 | Adding & Using Tailwind CSS In A React Project | 03:40 |
110 | Tailwind: Media Queries & Pseudo Selectors | 05:23 |
111 | Dynamic & Conditional Styling with Tailwind | 05:51 |
112 | Migrating The Demo App to Tailwind CSS | 04:16 |
113 | Tailwind CSS: Pros & Cons | 04:27 |
114 | Module Introduction | 01:27 |
115 | The Starting Project | 01:17 |
116 | Understanding React Error Messages | 08:32 |
117 | Using the Browser Debugger & Breakpoints | 07:24 |
118 | Understanding React's "Strict Mode" | 06:20 |
119 | Using the React DevTools (Browser Extension) | 03:58 |
120 | Module Introduction & Starting Project | 03:20 |
121 | Repetition: Managing User Input with State (Two-Way-Binding) | 05:18 |
122 | Introducing Refs: Connecting & Accessing HTML Elements via Refs | 06:05 |
123 | Manipulating the DOM via Refs | 02:15 |
124 | Refs vs State Values | 04:46 |
125 | Adding Challenges to the Demo Project | 04:50 |
126 | Setting Timers & Managing State | 04:57 |
127 | Using Refs for More Than "DOM Element Connections" | 08:31 |
128 | Adding a Modal Component | 06:41 |
129 | Forwarding Refs to Custom Components | 06:08 |
130 | Exposing Component APIs via the useImperativeHandle Hook | 07:15 |
131 | More Examples: When To Use Refs & State | 08:51 |
132 | Sharing State Across Components | 04:59 |
133 | Enhancing the Demo App "Result Modal" | 02:13 |
134 | Introducing & Understanding "Portals" | 06:56 |
135 | Module Introduction & Starting Project | 03:12 |
136 | Adding a "Projects Sidebar" Component | 02:30 |
137 | Styling the Sidebar & Button with Tailwind CSS | 05:01 |
138 | Adding the "New Project" Component & A Reusable "Input" Component | 05:59 |
139 | Styling Buttons & Inputs with Tailwind CSS | 07:04 |
140 | Splitting Components to Split JSX & Tailwind Styles (for Higher Reusability) | 06:50 |
141 | Managing State to Switch Between Components | 08:20 |
142 | Collecting User Input with Refs & Forwarded Refs | 13:23 |
143 | Handling Project Creation & Updating the UI | 06:01 |
144 | Validating User Input & Showing an Error Modal via useImperativeHandle | 10:56 |
145 | Styling the Modal via Tailwind CSS | 05:47 |
146 | Making Projects Selectable & Viewing Project Details | 15:23 |
147 | Handling Project Deletion | 04:45 |
148 | Adding "Project Tasks" & A Tasks Component | 05:52 |
149 | Managing Tasks & Understanding Prop Drilling | 15:24 |
150 | Clearing Tasks & Fixing Minor Bugs | 06:41 |
151 | Module Introduction | 01:55 |
152 | Understanding Prop Drilling & Project Overview | 05:59 |
153 | Prop Drilling: Component Composition as a Solution | 05:18 |
154 | Introducing the Context API | 02:18 |
155 | Creating & Providing The Context | 07:35 |
156 | Consuming the Context | 05:50 |
157 | Linking the Context to State | 07:28 |
158 | A Different Way Of Consuming Context | 05:02 |
159 | What Happens When Context Values Change? | 01:26 |
160 | Migrating the Entire Demo Project to use the Context API | 06:21 |
161 | Outsourcing Context & State Into a Separate Provider Component | 06:06 |
162 | Introducing the useReducer Hook | 10:07 |
163 | Dispatching Actions & Editing State with useReducer | 10:15 |
164 | Module Introduction & Starting Project | 03:39 |
165 | What's a "Side Effect"? A Thorough Example | 07:24 |
166 | A Potential Problem with Side Effects: An Infinite Loop | 02:49 |
167 | Using useEffect for Handling (Some) Side Effects | 05:32 |
168 | Not All Side Effects Need useEffect | 07:59 |
169 | useEffect Not Needed: Another Example | 08:31 |
170 | Preparing Another Use-Case For useEffect | 04:11 |
171 | Using useEffect for Syncing With Browser APIs | 04:10 |
172 | Understanding Effect Dependencies | 03:01 |
173 | Preparing Another Problem That Can Be Fixed with useEffect | 04:55 |
174 | Introducing useEffect's Cleanup Function | 04:50 |
175 | The Problem with Object & Function Dependencies | 08:10 |
176 | The useCallback Hook | 03:49 |
177 | useEffect's Cleanup Function: Another Example | 07:32 |
178 | Optimizing State Updates | 03:45 |
179 | Module Introduction & Starting Project | 02:22 |
180 | A First Component & Some State | 07:04 |
181 | Deriving Values, Outputting Questions & Registering Answers | 12:54 |
182 | Shuffling Answers & Adding Quiz Logic | 06:58 |
183 | Adding Question Timers | 11:41 |
184 | Working with Effect Dependencies & useCallback | 07:15 |
185 | Using Effect Cleanup Functions & Using Keys for Resetting Components | 07:54 |
186 | Highlighting Selected Answers & Managing More State | 11:35 |
187 | Splitting Components Up To Solve Problems | 17:44 |
188 | Moving Logic To Components That Actually Need It ("Moving State Down") | 12:47 |
189 | Setting Different Timers Based On The Selected Answer | 08:08 |
190 | Outputting Quiz Results | 15:10 |
191 | Module Introduction | 01:17 |
192 | React Builds A Component Tree / How React Works Behind The Scenes | 08:42 |
193 | Analyzing Component Function Executions via React's DevTools Profiler | 05:58 |
194 | Avoiding Component Function Executions with memo() | 08:05 |
195 | Avoiding Component Function Executions with Clever Structuring | 06:07 |
196 | Understanding the useCallback() Hook | 07:06 |
197 | Understanding the useMemo() Hook | 05:59 |
198 | React Uses A Virtual DOM - Time To Explore It! | 07:16 |
199 | Why Keys Matter When Managing State! | 11:57 |
200 | More Reasons For Why Keys Matter | 02:55 |
201 | Using Keys For Resetting Components | 05:23 |
202 | State Scheduling & Batching | 05:50 |
203 | Optimizing React with MillionJS | 04:46 |
204 | Module Introduction | 02:11 |
205 | What & Why | 04:54 |
206 | Adding a First Class-based Component | 06:55 |
207 | Working with State & Events | 11:39 |
208 | The Component Lifecycle (Class-based Components Only!) | 05:21 |
209 | Lifecycle Methods In Action | 11:47 |
210 | Class-based Components & Context | 04:54 |
211 | Class-based vs Functional Components: A Summary | 02:43 |
212 | Introducing Error Boundaries | 09:53 |
213 | Module Introduction | 02:54 |
214 | How (Not) To Connect To A Database | 06:21 |
215 | Starting Project & Dummy Backend API | 03:26 |
216 | Preparing the App For Data Fetching | 05:08 |
217 | How NOT To Send HTTP Requests (And Why It's Wrong) | 06:25 |
218 | Sending HTTP Requests (GET Request) via useEffect | 04:00 |
219 | Using async / await | 02:27 |
220 | Handling Loading States | 05:28 |
221 | Handling HTTP Errors | 09:17 |
222 | Transforming Fetched Data | 05:32 |
223 | Extracting Code & Improving Code Structure | 02:54 |
224 | Sending Data with POST Requests | 12:01 |
225 | Using Optimistic Updating | 07:34 |
226 | Deleting Data (via DELETE HTTP Requests) | 03:44 |
227 | Practice: Fetching Data | 07:14 |
228 | Module Introduction & Starting Project | 02:08 |
229 | Revisiting the "Rules of Hooks" & Why To Use Hooks | 06:12 |
230 | Creating a Custom Hook | 05:33 |
231 | Custom Hook: Managing State & Returning State Values | 10:52 |
232 | Exposing Nested Functions From The Custom Hook | 06:04 |
233 | Using A Custom Hook in Multiple Components | 04:26 |
234 | Creating Flexible Custom Hooks | 05:00 |
235 | Module Introduction & Starting Project | 02:05 |
236 | What Are Forms & What's Tricky About Them? | 04:00 |
237 | Handling Form Submission | 10:27 |
238 | Managing & Getting User Input via State & Generic Handlers | 11:13 |
239 | Getting User Input via Refs | 04:36 |
240 | Getting Values via FormData & Native Browser APIs | 09:04 |
241 | Resetting Forms | 04:39 |
242 | Validating Input on Every Keystroke via State | 06:59 |
243 | Validating Input Upon Lost Focus (Blur) | 07:54 |
244 | Validating Input Upon Form Submission | 07:55 |
245 | Validating Input via Built-in Validation Props | 05:00 |
246 | Mixing Custom & Built-in Validation Logic | 04:29 |
247 | Building & Using a Reusable Input Component | 10:06 |
248 | Outsourcing Validation Logic | 03:23 |
249 | Creating a Custom useInput Hook | 15:12 |
250 | Using Third-Party Form Libraries | 01:32 |
251 | Module Introduction & Starting Project | 04:15 |
252 | Planning the App & Adding a First Component | 06:41 |
253 | Fetching Meals Data (GET HTTP Request) | 12:01 |
254 | Adding a "MealItem" Component | 07:40 |
255 | Formatting & Outputting Numbers as Currency | 02:52 |
256 | Creating a Configurable & Flexible Custom Button Component | 08:03 |
257 | Getting Started with Cart Context & Reducer | 20:10 |
258 | Finishing & Using the Cart Context & Reducer | 17:22 |
259 | Adding a Reusable Modal Component with useEffect | 07:27 |
260 | Opening the Cart in the Modal via a New Context | 19:33 |
261 | Working on the Cart Items | 08:02 |
262 | Adding a Custom Input Component & Managing Modal Visibility | 19:32 |
263 | Handling Form Submission & Validation | 06:04 |
264 | Sending a POST Request with Order Data | 07:36 |
265 | Adding a Custom HTTP Hook & Avoiding Common Errors | 26:24 |
266 | Handling HTTP Loading & Error States | 03:13 |
267 | Finishing Touches | 14:08 |
268 | Module Introduction | 01:06 |
269 | Another Look At State In React Apps | 05:15 |
270 | Redux vs React Context | 06:20 |
271 | How Redux Works | 05:49 |
272 | Exploring The Core Redux Concepts | 15:15 |
273 | More Redux Basics | 03:05 |
274 | Preparing a new Project | 02:00 |
275 | Creating a Redux Store for React | 04:55 |
276 | Providing the Store | 03:14 |
277 | Using Redux Data in React Components | 05:09 |
278 | Dispatching Actions From Inside Components | 03:34 |
279 | Redux with Class-based Components | 10:21 |
280 | Attaching Payloads to Actions | 04:16 |
281 | Working with Multiple State Properties | 06:20 |
282 | How To Work With Redux State Correctly | 05:08 |
283 | Redux Challenges & Introducing Redux Toolkit | 05:28 |
284 | Adding State Slices | 08:12 |
285 | Connecting Redux Toolkit State | 04:48 |
286 | Migrating Everything To Redux Toolkit | 06:20 |
287 | Working with Multiple Slices | 11:51 |
288 | Reading & Dispatching From A New Slice | 06:57 |
289 | Splitting Our Code | 05:04 |
290 | Summary | 03:54 |
291 | Module Introduction | 00:40 |
292 | Redux & Side Effects (and Asynchronous Code) | 03:28 |
293 | Refresher / Practice: Part 1/2 | 20:13 |
294 | Refresher / Practice: Part 2/2 | 18:01 |
295 | Redux & Async Code | 04:10 |
296 | Frontend Code vs Backend Code | 05:41 |
297 | Where To Put Our Logic | 09:00 |
298 | Using useEffect with Redux | 06:01 |
299 | Handling Http States & Feedback with Redux | 12:50 |
300 | Using an Action Creator Thunk | 12:08 |
301 | Getting Started with Fetching Data | 08:40 |
302 | Finalizing the Fetching Logic | 05:17 |
303 | Exploring the Redux DevTools | 05:38 |
304 | Summary | 01:54 |
305 | Module Introduction | 03:22 |
306 | Routing: Multiple Pages in Single-Page Applications | 03:16 |
307 | Project Setup & Installing React Router | 03:07 |
308 | Defining Routes | 07:43 |
309 | Adding a Second Route | 02:08 |
310 | Exploring an Alternative Way of Defining Routes | 03:02 |
311 | Navigating between Pages with Links | 04:37 |
312 | Layouts & Nested Routes | 08:25 |
313 | Showing Error Pages with errorElement | 03:59 |
314 | Working with Navigation Links (NavLink) | 06:38 |
315 | Navigating Programmatically | 02:42 |
316 | Defining & Using Dynamic Routes | 07:45 |
317 | Adding Links for Dynamic Routes | 03:23 |
318 | Understanding Relative & Absolute Paths | 10:39 |
319 | Working with Index Routes | 01:57 |
320 | Onwards to a new Project Setup | 03:17 |
321 | Time to Practice: Problem | 01:26 |
322 | Time to Practice: Solution | 23:17 |
323 | Data Fetching with a loader() | 07:36 |
324 | Using Data From A Loader In The Route Component | 02:52 |
325 | More loader() Data Usage | 03:18 |
326 | Where Should loader() Code Be Stored? | 02:19 |
327 | When Are loader() Functions Executed? | 02:49 |
328 | Reflecting The Current Navigation State in the UI | 02:54 |
329 | Returning Responses in loader()s | 04:02 |
330 | Which Kind Of Code Goes Into loader()s? | 01:15 |
331 | Error Handling with Custom Errors | 04:57 |
332 | Extracting Error Data & Throwing Responses | 06:25 |
333 | The json() Utility Function | 02:08 |
334 | Dynamic Routes & loader()s | 07:33 |
335 | The useRouteLoaderData() Hook & Accessing Data From Other Routes | 07:41 |
336 | Planning Data Submission | 02:23 |
337 | Working with action() Functions | 09:09 |
338 | Submitting Data Programmatically | 09:07 |
339 | Updating the UI State Based on the Submission Status | 04:03 |
340 | Validating User Input & Outputting Validation Errors | 06:58 |
341 | Reusing Actions via Request Methods | 07:56 |
342 | Behind-the-Scenes Work with useFetcher() | 09:12 |
343 | Deferring Data Fetching with defer() | 09:08 |
344 | Controlling Which Data Should Be Deferred | 07:23 |
345 | Module Summary | 02:58 |
346 | Module Introduction | 01:11 |
347 | How Authentication Works | 09:09 |
348 | Project Setup & Route Setup | 03:47 |
349 | Working with Query Parameters | 07:36 |
350 | Implementing the Auth Action | 11:41 |
351 | Validating User Input & Outputting Validation Errors | 04:19 |
352 | Adding User Login | 01:56 |
353 | Attaching Auth Tokens to Outgoing Requests | 06:33 |
354 | Adding User Logout | 04:22 |
355 | Updating the UI Based on Auth Status | 06:06 |
356 | Adding Route Protection | 02:47 |
357 | Adding Automatic Logout | 05:11 |
358 | Managing the Token Expiratoin | 07:29 |
359 | Module Introduction | 01:41 |
360 | Deployment Steps | 03:36 |
361 | Understanding Lazy Loading | 04:48 |
362 | Adding Lazy Loading | 09:12 |
363 | Building the Code For Production | 02:23 |
364 | Deployment Example | 06:45 |
365 | Server-side Routing & Required Configuration | 04:07 |
366 | Module Introduction | 01:47 |
367 | Project Setup & Overview | 04:09 |
368 | React Query: What & Why? | 06:00 |
369 | Installing & Using Tanstack Query - And Seeing Why It's Great! | 16:33 |
370 | Understanding & Configuring Query Behaviors - Cache & Stale Data | 07:44 |
371 | Dynamic Query Functions & Query Keys | 13:06 |
372 | The Query Configuration Object & Aborting Requests | 05:28 |
373 | Enabled & Disabled Queries | 06:56 |
374 | Changing Data with Mutations | 11:30 |
375 | Fetching More Data & Testing the Mutation | 06:40 |
376 | Acting on Mutation Success & Invalidating Queries | 08:51 |
377 | A Challenge! The Problem | 02:15 |
378 | A Challenge! The Solution | 16:38 |
379 | Disabling Automatic Refetching After Invalidations | 02:43 |
380 | Enhancing the Demo App & Repeating Mutation Concepts | 09:19 |
381 | React Query Advantages In Action | 08:58 |
382 | Updating Data with Mutations | 04:50 |
383 | Optimistic Updating | 13:07 |
384 | Using the Query Key As Query Function Input | 07:48 |
385 | React Query & React Router | 20:27 |
386 | Module Introduction | 02:09 |
387 | What is NextJS? | 04:46 |
388 | Key Feature 1: Built-in Server-side Rendering (Improved SEO!) | 06:38 |
389 | Key Feature 2: Simplified Routing with File-based Routing | 03:14 |
390 | Key Feature 3: Build Fullstack Apps | 01:51 |
391 | Creating a New Next.js Project & App | 05:40 |
392 | Analyzing the Created Project | 02:53 |
393 | Adding First Pages | 06:06 |
394 | Adding Nested Paths & Pages (Nested Routes) | 03:48 |
395 | Creating Dynamic Pages (with Parameters) | 03:37 |
396 | Extracting Dynamic Parameter Values | 04:08 |
397 | Linking Between Pages | 07:14 |
398 | Onwards to a bigger Project! | 03:33 |
399 | Preparing the Project Pages | 03:43 |
400 | Outputting a List of Meetups | 05:04 |
401 | Adding the New Meetup Form | 03:56 |
402 | The "_app.js" File & Layout Wrapper | 06:18 |
403 | Using Programmatic (Imperative) Navigation | 03:48 |
404 | Adding Custom Components & CSS Modules | 10:01 |
405 | How Pre-rendering Works & Which Problem We Face | 05:53 |
406 | Data Fetching for Static Pages | 08:57 |
407 | More on Static Site Generation (SSG) | 05:45 |
408 | Exploring Server-side Rendering (SSR) with "getServerSideProps" | 06:28 |
409 | Working with Params for SSG Data Fetching | 05:15 |
410 | Preparing Paths with "getStaticPaths" & Working With Fallback Pages | 07:17 |
411 | Introducing API Routes | 06:21 |
412 | Working with MongoDB | 09:33 |
413 | Sending Http Requests To Our API Routes | 06:50 |
414 | Getting Data From The Database | 07:11 |
415 | Getting Meetup Details Data & Preparing Pages | 09:42 |
416 | Adding "head" Metadata | 09:20 |
417 | Deploying Next.js Projects | 12:27 |
418 | Using Fallback Pages & Re-deploying | 04:14 |
419 | Summary | 02:16 |
420 | Module Introduction | 03:06 |
421 | Project Setup & Overview | 01:43 |
422 | Animating with CSS Transitions | 07:42 |
423 | Animating with CSS Animations | 05:39 |
424 | Introducing Framer Motion | 03:49 |
425 | Framer Motion Basics & Fundamentals | 08:30 |
426 | Animating Between Conditional Values | 04:14 |
427 | Adding Entry Animations | 04:29 |
428 | Animating Element Disappearances / Removal | 03:52 |
429 | Making Elements "Pop" With Hover Animations | 04:14 |
430 | Reusing Animation States | 03:29 |
431 | Nested Animations & Variants | 07:47 |
432 | Animating Staggered Lists | 04:30 |
433 | Animating Colors & Working with Keyframes | 04:05 |
434 | Imperative Animations | 07:29 |
435 | Animating Layout Changes | 03:35 |
436 | Orchestrating Multi-Element Animations | 10:06 |
437 | Combining Animations With Layout Animations | 03:56 |
438 | Animating Shared Elements | 04:16 |
439 | Re-triggering Animations via Keys | 04:53 |
440 | Scroll-based Animations | 15:45 |
441 | Module Introduction | 01:24 |
442 | What & Why? | 03:24 |
443 | Understanding Different Kinds Of Tests | 04:05 |
444 | What To Test & How To Test | 01:30 |
445 | Understanding the Technical Setup & Involved Tools | 02:40 |
446 | Running a First Test | 07:17 |
447 | Writing Our First Test | 10:15 |
448 | Grouping Tests Together With Test Suites | 02:15 |
449 | Testing User Interaction & State | 14:01 |
450 | Testing Connected Components | 03:20 |
451 | Testing Asynchronous Code | 09:12 |
452 | Working With Mocks | 08:31 |
453 | Summary & Further Resources | 03:48 |
454 | Module Introduction | 01:27 |
455 | What & Why? | 06:35 |
456 | Installing & Using TypeScript | 06:39 |
457 | Exploring the Base Types | 03:56 |
458 | Working with Array & Object Types | 05:34 |
459 | Understanding Type Inference | 02:48 |
460 | Using Union Types | 02:49 |
461 | Understanding Type Aliases | 02:43 |
462 | Functions & Function Types | 05:20 |
463 | Diving Into Generics | 08:02 |
464 | Creating a React + TypeScript Project | 08:35 |
465 | Working with Components & TypeScript | 05:42 |
466 | Working with Props & TypeScript | 14:21 |
467 | Adding a Data Model | 09:10 |
468 | Time to Practice: Exercise Time! | 07:03 |
469 | Form Submissions In TypeScript Projects | 05:22 |
470 | Working with refs & useRef | 10:57 |
471 | Working with "Function Props" | 07:27 |
472 | Managing State & TypeScript | 05:14 |
473 | Adding Styling | 02:20 |
474 | Time to Practice: Removing a Todo | 09:28 |
475 | The Context API & TypeScript | 13:56 |
476 | Summary | 02:19 |
477 | Bonus: Exploring tsconfig.json | 05:47 |
478 | Module Introduction | 02:11 |
479 | What Are React Hooks? | 04:57 |
480 | The Starting Project | 04:52 |
481 | Getting Started with useState() | 09:21 |
482 | More on useState() & State Updating | 11:55 |
483 | Array Destructuring | 02:35 |
484 | Multiple States | 03:48 |
485 | Rules of Hooks | 02:22 |
486 | Passing State Data Across Components | 07:57 |
487 | Time to Practice: Hooks Basics - Problem | 01:04 |
488 | Time to Practice: Hooks Basics - Solution | 02:56 |
489 | Sending Http Requests | 07:17 |
490 | useEffect() & Loading Data | 08:07 |
491 | Understanding useEffect() Dependencies | 02:22 |
492 | More on useEffect() | 09:38 |
493 | What's useCallback()? | 05:29 |
494 | Working with Refs & useRef() | 05:22 |
495 | Cleaning Up with useEffect() | 03:22 |
496 | Deleting Ingredients | 02:30 |
497 | Loading Errors & State Batching | 08:49 |
498 | Understanding useReducer() | 09:44 |
499 | Using useReducer() for the Http State | 10:41 |
500 | Working with useContext() | 08:28 |
501 | Performance Optimizations with useMemo() | 10:31 |
502 | Getting Started with Custom Hooks | 13:46 |
503 | Sharing Data Between Custom Hooks & Components | 14:59 |
504 | Using the Custom Hook | 08:12 |
505 | Wrap Up | 03:06 |
506 | Module Introduction | 01:09 |
507 | What Is React & Why Would You Use It? | 05:38 |
508 | React Projects - Requirements | 02:10 |
509 | Creating React Projects | 03:28 |
510 | Our Starting Project | 03:29 |
511 | Understanding How React Works | 07:47 |
512 | Building A First Custom Component | 11:16 |
513 | Outputting Dynamic Values | 05:04 |
514 | Reusing Components | 06:01 |
515 | Passing Data to Components with Props | 06:16 |
516 | CSS Styling & CSS Modules | 10:08 |
517 | Exercise & Another Component | 06:32 |
518 | Preparing the App For State Management | 03:47 |
519 | Adding Event Listeners | 07:53 |
520 | Working with State | 10:01 |
521 | Lifting State Up | 09:09 |
522 | The Special "children" Prop | 07:22 |
523 | State & Conditional Content | 09:00 |
524 | Adding a Shared Header & More State Management | 07:52 |
525 | Adding Form Buttons | 03:35 |
526 | Handling Form Submission | 06:19 |
527 | Updating State Based On Previous State | 05:31 |
528 | Outputting List Data | 06:40 |
529 | Adding a Backend to the React SPA | 06:11 |
530 | Sending a POST HTTP Request | 04:13 |
531 | Handling Side Effects with useEffect() | 09:07 |
532 | Handle Loading State | 04:24 |
533 | Understanding & Adding Routing | 03:56 |
534 | Adding Routes | 05:37 |
535 | Working with Layout Routes | 04:09 |
536 | Refactoring Route Components & More Nesting | 05:36 |
537 | Linking & Navigating | 08:10 |
538 | Data Fetching via loader()s | 09:08 |
539 | Submitting Data with action()s | 11:09 |
540 | Dynamic Routes | 08:42 |
541 | Module Summary | 01:26 |
542 | Roundup | 01:11 |
Similar courses to React - The Complete Guide 2024
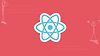
The Complete Guide to Advanced React Patterns (2020)udemy
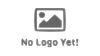
PHP Symfony 4 API Platform + React.js Full Stack Masterclassudemy
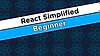
React Simplified - Beginnerwebdevsimplified.com
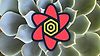
React Query: Server State Management in Reactudemy
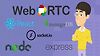
Discord Clone - Learn MERN Stack with WebRTC and SocketIOudemy
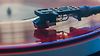
My first Remix appBuild UI
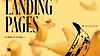
Build fancy landing pages with React and ThreejsPaul Henschel (@0xca0a)
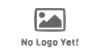
Next.js & React with ChatGPT - Development Guide (2023)udemy
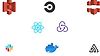
Build a React & Redux App w CircleCI CICD, AWS & Terraformudemy
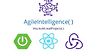