React Front To Back 2022
This course is for anyone that wants to learn React and also for React developers looking to build some projects and sharpen their skills. The first project (Feedback App) is structured in a way so I can explain the fundamentals such as components, hooks, props, state, etc in a way that beginners can understand. The second project (Github Finder) will show you how to work with 3rd party APIs and the third project (House Marketplace) is a larger app that uses Firebase 9 and includes authentication, Firestore queries, file storage and more.
More
Here are some of the things you will learn in this course:
React fundamentals including components, props, hooks, state, etc
React hooks such as useState, useEffect, useContext, useReducer, useRef, etc
Creating custom hooks
React Router v6 (latest version)
How to handle global state with context, reducers and hooks
Firebase 9 authentication, queries, storage
Deploying React apps to Vercel & Netlify
Basic Animation with Framer Motion
Implement Leaflet maps and Swiper sliders
Much More...
This course has been completely re-done with new projects and concepts from the old course. I have a few React courses and this is the one that I always suggest people start with before moving on to the MERN courses
I am also working on a 4th project for the course, which will be a full-stack MERN ticketing system that uses Redux for state management.
Watch Online React Front To Back 2022
# | Title | Duration |
---|---|---|
1 | Welcome To The Course! | 01:55 |
2 | What Is React? | 08:32 |
3 | Environment Setup | 05:51 |
4 | Code Repos | 02:00 |
5 | Feedback Project Intro | 02:50 |
6 | Create React App | 07:02 |
7 | Initializing React | 07:13 |
8 | Intro To JSX | 07:06 |
9 | Dynamic Values & LIsts in JSX | 05:24 |
10 | Conditionals in JSX | 05:09 |
11 | Creating Your First Component & Props | 08:01 |
12 | Adding Styles To A Component | 05:35 |
13 | State & useState Hook | 08:22 |
14 | Managing Global State | 08:29 |
15 | Card Component & Conditional Styles | 10:16 |
16 | Events & Prop Drilling | 10:19 |
17 | FeedbackStats Component & Reactivity | 07:32 |
18 | Form Input & State | 06:03 |
19 | Custom Button Component | 05:28 |
20 | Real-Time Validation | 04:32 |
21 | Rating Select Component | 07:08 |
22 | Add Feedback | 07:21 |
23 | Fade Animation With Framer Motion | 03:52 |
24 | Creating Routes (React Router 5) | 08:22 |
25 | Upgrading To React Router 6 | 02:33 |
26 | Creating Links (v5 & v6) | 05:13 |
27 | NavLink & useParams | 06:40 |
28 | Navigate & Nested Routes | 06:52 |
29 | Create a Context & Provider | 06:35 |
30 | Get Global State With The useContext Hook | 04:42 |
31 | Moving Functions To Context | 07:25 |
32 | Edit Feedback Event | 05:47 |
33 | Side Effects With useEffect | 07:33 |
34 | Update Feedback Item | 05:14 |
35 | Deploy To Netlify | 05:23 |
36 | APIs & Requests Explained | 06:26 |
37 | Setting Up JSON-Server Mock Backend | 07:47 |
38 | Run Client & Server With Concurrently | 02:37 |
39 | Fetch Data From JSON-Server Backend | 04:56 |
40 | Spinner Component | 05:34 |
41 | Add Feedback & Setting a Proxy | 05:26 |
42 | Update & Delete From JSON-Server | 04:17 |
43 | GitHub Finder Project Intro | 02:35 |
44 | Setup Tailwind & Daisy UI | 08:26 |
45 | Navbar Component | 09:06 |
46 | Footer Component | 03:33 |
47 | Pages & Routes | 07:39 |
48 | Github API & Getting Token | 07:07 |
49 | UserList Component | 10:16 |
50 | Loading Spinner | 03:08 |
51 | Display Users | 06:49 |
52 | Setup Github Context | 09:18 |
53 | Reducers & useReducer Hook | 09:17 |
54 | Clean Up Fetch Users | 03:43 |
55 | User Search Component | 10:27 |
56 | Search Users | 04:32 |
57 | Clear Users | 03:27 |
58 | Alert Context & Reducer | 08:09 |
59 | Alert Component | 07:43 |
60 | Get Single User | 09:39 |
61 | User Profile Top | 11:32 |
62 | User Profile Stats | 08:44 |
63 | Get User Repos | 10:06 |
64 | Repo Items | 08:25 |
65 | Move SearchUsers To Actions File | 06:20 |
66 | Move getUser To Actions File | 07:48 |
67 | Cleaning Up Our Actions & Axios | 07:52 |
68 | Deploy To Vercel | 02:30 |
69 | Section Intro | 00:46 |
70 | useRef Example 1 - Create DOM Reference | 08:20 |
71 | useRef Example 2 - Get Previous State | 05:16 |
72 | useRef Example 3 - Memory Leak Error Fix | 09:44 |
73 | useMemo Example | 10:44 |
74 | useCallback Example | 07:12 |
75 | Custom Hook 1 - useFetch | 09:12 |
76 | Custom Hook 2 - useLocalStorage | 15:10 |
77 | House Marketplace Project Intro | 04:41 |
78 | App & FIrebase Setup | 06:57 |
79 | Enable Authentication & Create Rules | 07:55 |
80 | Dummy Data & Indexes | 11:49 |
81 | Pages & Routes | 06:48 |
82 | Navbar Component | 10:03 |
83 | Sign In & Sign Up Forms | 14:56 |
84 | Register User | 05:29 |
85 | Save User To Firestore | 04:52 |
86 | User Sign In | 08:26 |
87 | Alerts With React Toastify | 04:55 |
88 | User Logout | 05:38 |
89 | Display & Update User Details | 13:25 |
90 | PrivateRoute Component & useAuthStatus Hook | 14:51 |
91 | Forgot Password Page | 09:16 |
92 | Google OAuth | 14:00 |
93 | Explore Page | 05:00 |
94 | Fetch Listings From Firebase | 15:07 |
95 | Listing Item Component | 11:44 |
96 | Offers Page | 03:30 |
97 | Start Create Listing Page | 12:48 |
98 | Create Listing Form | 17:38 |
99 | Get Coords With Geocoding API | 16:34 |
100 | Uploading Images To FIrebase | 11:52 |
101 | Save Listings To Firestore | 08:03 |
102 | Quick Note & Change | 02:06 |
103 | Fetch Single Listing | 08:04 |
104 | Listing Details | 10:57 |
105 | Contact Landlord Page | 12:30 |
106 | Leaflet Map | 07:30 |
107 | Listings Page Slider | 07:02 |
108 | Explore Slider | 13:29 |
109 | Profile Listings & Delete | 12:43 |
110 | Load More Pagination | 08:28 |
111 | Edit Listing Icon | 05:21 |
112 | Edit Listing | 12:42 |
113 | Clear Up Console Warnings | 03:37 |
114 | Deploy To Vercel | 03:18 |
115 | Project Intro | 02:00 |
116 | What Is The MERN Stack? | 04:30 |
117 | MongoDB Setup | 05:08 |
118 | Server File Stucture | 05:51 |
119 | Basic Express Server Setup | 06:25 |
120 | Add Routes & Controller | 05:58 |
121 | Error & Exception Handling | 11:43 |
122 | Connect To The Database | 06:01 |
123 | Register User | 10:05 |
124 | Login & Create JWT | 09:14 |
125 | Protect Routes & Authentication | 13:24 |
126 | Frontend Folder Setup | 09:02 |
127 | Header & Initial Pages | 08:03 |
128 | Home, Login & Register UI | 15:06 |
129 | Redux Setup & Auth Slice | 08:42 |
130 | Hook Register Form To Redux | 09:40 |
131 | Register User | 21:01 |
132 | Logout User | 08:13 |
133 | User Login | 07:54 |
134 | Ticket Model & Routes | 11:08 |
135 | Get & Create Tickets (Backend) | 06:22 |
136 | Single Ticket, Update & Delete (Backend) | 09:21 |
137 | Route Guard | 10:00 |
138 | New Ticket Form | 08:35 |
139 | Add Tickets To Redux | 05:08 |
140 | Create Ticket Functionality | 16:11 |
141 | Fetch Tickets From Backend | 08:52 |
142 | Listing Tickets In UI | 06:42 |
143 | Single Ticket Display | 15:22 |
144 | Close Ticket Functionality | 09:44 |
145 | Notes Backend | 13:31 |
146 | Fetch Notes Through Redux | 08:58 |
147 | Display Notes | 07:54 |
148 | Note Form Modal | 10:19 |
149 | Submit a Note | 05:31 |
150 | Deploy To Heroku | 12:06 |
Similar courses to React Front To Back 2022
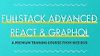
Full Stack Advanced React + GraphQL
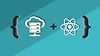
Server side rendering with Next + React
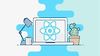
Building Applications with React 17 and ASP.NET Core 6
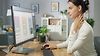
Complete DApp - Solidity & React - Blockchain Development
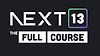
Next.js - The Full Course
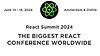
React Summit 2024 - Amsterdam
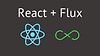
React: Flux Architecture (ES6)
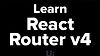
React Router v4
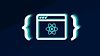