Laravel Livewire
Building modern web apps is hard. Tools like Vue and React are extremely powerful, but the complexity they add to a full-stack developer's workflow is insane.
More
How the he*k does this work?
- Livewire renders the initial component output with the page (like a Blade include), this way it's SEO friendly.
- When an interaction occurs, Livewire makes an AJAX request to the server with the updated data.
- The server re-renders the component and responds with the new HTML.
- Livewire then intelligently mutates DOM according to the things that changed.
Some questions you might have...
Does this use websockets?
No, Livewire relies solely on AJAX requests to do all its server communication. This means it's as reliable and scalable as your current setup.
Is this a Vue-replacement?
In some ways yes, but mostly for cases where your Vue components are already sending `axios` or `fetch` requests. (Think searching, filtering, forms)
If it doesn't replace Vue, what do I do when I need JavaScript, like a drop-down, modal, or datepicker?
Livewire works beautifully with the AlpineJS framework (It was built for this need). For third-party library integration (something like Select2, Pickaday, or Dropzone.js), Livewire provides APIs to add support for these. Livewire also has a plugin to support using VueJs components inside of your Livewire components.
Watch Online Laravel Livewire
# | Title | Duration |
---|---|---|
1 | Installation | 03:35 |
2 | Actions | 05:54 |
3 | Properties | 07:41 |
4 | Lifecycle Hooks | 04:05 |
5 | Page Components | 07:49 |
6 | Basic Table | 09:59 |
7 | Basic Form | 06:35 |
8 | Alpine | 07:05 |
9 | Testing | 08:14 |
10 | Nesting | 10:52 |
11 | Navigatetegrating With Filepond | 03:42 |
12 | Setup | 05:08 |
13 | Showing a success message | 10:58 |
14 | Adding validation | 10:15 |
15 | Extracting a Form Object | 06:26 |
16 | Accessibility | 11:45 |
17 | Radio buttons | 13:18 |
18 | Checkboxes | 06:53 |
19 | Select dropdowns | 06:57 |
20 | Enums | 11:58 |
21 | Building a modal | 10:40 |
22 | Extracting a Blade component | 13:08 |
23 | Confirmation dialogs | 14:22 |
24 | Forms inside modals | 12:24 |
25 | Extracting a nested Livewire component | 12:36 |
26 | Creating row components | 07:27 |
27 | Adding an edit modal | 09:36 |
28 | Adding a dropdown menu | 10:27 |
29 | Teleporting modals | 07:38 |
30 | Styling for mobile devices | 06:57 |
31 | Adding "swipe to close" | 17:45 |
32 | Teaser | 03:46 |
33 | Getting started | 06:31 |
34 | Building a static table | 12:25 |
35 | Page authorization | 01:57 |
36 | Pagination | 08:02 |
37 | Loading indicators | 06:35 |
38 | Text searching | 07:18 |
39 | Column sorting | 19:23 |
40 | Row dropdown actions | 11:38 |
41 | Exporting to CSV | 09:40 |
42 | Bulk actions | 10:11 |
43 | Select-all checkboxes | 11:24 |
44 | Indeterminate checkbox states | 10:28 |
45 | Lazy loading | 05:28 |
46 | Refactoring to traits | 08:01 |
47 | Extracting a nested component | 03:35 |
48 | Chart scaffolding | 09:06 |
49 | Charting real data | 10:43 |
50 | Making the chart reactive | 11:36 |
51 | Product filtering | 18:47 |
52 | Tracking products in the URL | 04:48 |
53 | Date range filtering | 12:06 |
54 | Charting different date ranges | 03:54 |
55 | Custom date range | 26:08 |
56 | Order status filtering | 12:21 |
57 | Using radio groups for filtering | 09:05 |
58 | Designing for mobile | 06:17 |
59 | Component Basics | 06:55 |
60 | Forwarding attributes | 10:55 |
61 | Slots | 02:47 |
62 | Advanced Slots | 09:33 |
63 | Nesting components | 06:22 |
64 | Using @aware | 08:59 |
65 | Conditional attributes | 05:25 |
66 | Using with `wire:model` | 05:34 |
67 | Dynamic components | 03:26 |
68 | Teaser | 01:06 |
69 | Building a Todo List | 06:35 |
70 | Storing positions in the database | 07:57 |
71 | Using Alpine's Sort Plugin | 07:55 |
72 | Sorting items in the database | 13:52 |
73 | Handling removals | 03:05 |
74 | Adding drag handles | 06:36 |
75 | Extracting a Blade Component | 06:27 |
76 | Extracting an Eloquent Trait | 15:05 |
77 | Re-arranging Items | 06:12 |
78 | Sorting between groups | 11:20 |
Similar courses to Laravel Livewire
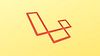
Master Laravel for Beginners Intermediate
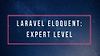
Laravel Eloquent: Expert Level
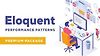
Eloquent Performance Patterns
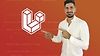
Mastering Laravel 10 Query Builder, Eloquent & Relationships
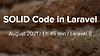
SOLID Code in Laravel
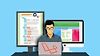
Ultimate Laravel Course 2018 (PayPal, Webshop, RESTful API)
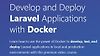
Develop and Deploy Laravel Applications with Docker
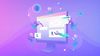
Laravel and Vue.js - Fullstack Web Development (2019)
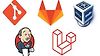
DevOps Project: CICD with Git GitLab Jenkins and Laravel
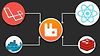