JavaScript: The Advanced Concepts
After many years of working with the language, Andrei has taken decades of experience, combining best practices from some of the top developers in the world, to get you to become one of the top performing Javascript developers. You will go from understanding the basics of JavaScript, to learning it to the point that you can teach it to others, impress coworkers, and wow future employers. You will be in the top 10% of JavaScript Programmers by the end of this course.
More
- Advanced JavaScript Practices
- Object Oriented Programming
- Functional Programming
- Scope and Execution Context
- Inheritance + Prototype Chain
- Latest features: ES6, ES7, ES8, ES9, ES10
- Closures
- Asynchronous JavaScript + Event Loop
- JavaScript Modules
- `this` keyword
- JavaScript Engine and Runtime
- Error Handling
- Stack Overflow
- Memory Leaks
- Composition vs Inheritance
- Type Coersion
- Pass By Reference vs Pass by Value
- Higher Order Functions
- IIFE
- .call(), .apply(), .bind()
- Interpreter/ Compiler/ JIT Compiler
- Garbage Collection
- Hoisting
- JavaScript best practices
Recently updated with ES10 for 2020! This course is unlike any JavaScript course you will find online. Join a live online community of over 200,000+ developers and a course taught by an industry expert that has actually worked both in Silicon Valley and Toronto as a senior developer. Graduates of this course are now working at Google, Tesla, Amazon, Apple, IBM, JP Morgan, Facebook + other top tech companies.
This course is the accumulation of years in the field, and combining the best resources, tools, and tutorials out there to create the ultimate JavaScript course that will teach you everything you need to know to be considered a Senior Javascript Developer.
Instead of spending years learning advanced Javascript concepts, you can fast track and get the knowledge that senior javascript developers have in just 30 days.
With this course you are going to learn beyond just the basics like most online courses. You won't just learn patterns, techniques and best practices. You are going to understand the "why" of the toughest part of the language, to the point that when you get asked any question about Javascript in an interview or in a meeting, you will be able to explain concepts that would truly make people see that you are a senior javascript programmer.
Most importantly, you will become a top 10% javascript developer by going beyond the superficial basics that a lot of courses cover. We are going to dive deep and come out the other end a confident advanced javascript developer. I guarantee it. Whether you are a web developer, a React, Angular, Vue.js developer (frontend developer), or a Node.js backend developer, you will benefit from this course because Javascript is at the core of these professions.
Some of the topics covered in this course are:
Javascript Engine
Javascript Runtime
Interpreter, Compiler, JIT Compiler
Writing Optimized Code
Call Stack + Memory Heap
Stack Overflow + Memory Leaks
Garbage Collection
Node.js
ES6, ES7, ES8, ES9 features
Single Threaded Model
Execution Context
Lexical Environment
Scope Chain
Hoisting
Function Invocation
Function Scope vs Block Scope
Dynamic vs Lexical Scope
this - call(), apply(), bind()
IIFEs
Context vs Scope
Static vs Dynamically Typed
Primitive Types
Pass by Reference vs Pass by Value
Type Coercion
Arrays, Functions, Objects
Closures
Prototypal Inheritance
Class Inheritance
Memoization
Higher Order Functions
Functions vs Objects
Scheme + Java in JavaScript
OOP (Object Oriented Programming)
Private vs Public properties
Functional Programming
Immutability
Imperative vs Declarative code
Composition vs Inheritance
Currying
Partial Application
Pure Functions
Referential Transparency
Compose
Pipe
Error Handling
Asynchronous JavaScript
Callbacks, Promises, Async/Await
Event Loop + Callback Queue
Task Queue + Microtask Queue
Concurrency + Parallelism
Modules in Javascript
Who this course is for:
- Developers who want to be considered in the top 10% of JavaScript programmers
- Bootcamp or online tutorial graduates that want to go beyond the basics
- Junior JavaScript Developers
- Junior Web Developers
- Programmers who don't want to waste time on basics and want to dive deep
Watch Online JavaScript: The Advanced Concepts
# | Title | Duration |
---|---|---|
1 | How To Succeed In This Course | 05:38 |
2 | Section Overview | 02:28 |
3 | Javascript Engine | 05:29 |
4 | Exercise: Javascript Engine | 00:57 |
5 | Inside the Engine | 04:06 |
6 | Exercise: JS Engine For All | 02:34 |
7 | Interpreters and Compilers | 06:22 |
8 | Inside the V8 Engine | 08:57 |
9 | Comparing Other Languages | 04:16 |
10 | Writing Optimized Code | 08:54 |
11 | WebAssembly | 03:16 |
12 | Call Stack and Memory Heap | 14:38 |
13 | Stack Overflow | 03:22 |
14 | Garbage Collection | 04:31 |
15 | Memory Leaks | 06:35 |
16 | Single Threaded | 01:59 |
17 | Exercise: Issue With Single Thread | 02:41 |
18 | Javascript Runtime | 14:08 |
19 | Node.js | 06:28 |
20 | Section Review | 05:03 |
21 | Section Overview | 01:07 |
22 | Execution Context | 08:36 |
23 | Lexical Environment | 05:40 |
24 | Hoisting | 10:48 |
25 | Exercise: Hoisting | 03:59 |
26 | Exercise: Hoisting 2 | 07:07 |
27 | Function Invocation | 07:01 |
28 | arguments Keyword | 04:23 |
29 | Variable Environment | 06:42 |
30 | Scope Chain | 12:21 |
31 | [[scope]] | 02:04 |
32 | Exercise: JS is Weird | 04:59 |
33 | Function Scope vs Block Scope | 03:36 |
34 | Exercise: Block Scope | 03:46 |
35 | Global Variables | 04:11 |
36 | IIFE | 13:39 |
37 | this Keyword | 17:00 |
38 | Exercise: Dynamic Scope vs Lexical Scope | 12:09 |
39 | call(), apply(), bind() | 11:18 |
40 | bind() and currying | 03:43 |
41 | Exercise: this Keyword | 03:10 |
42 | Context vs Scope | 01:09 |
43 | Section Review | 02:48 |
44 | Section Overview | 01:23 |
45 | Javascript Types | 13:41 |
46 | Array.isArray() | 02:18 |
47 | Pass By Value vs Pass By Reference | 17:07 |
48 | Type Coercion | 09:03 |
49 | JTS: Dynamic vs Static Typing | 11:51 |
50 | JTS: Weakly vs Strongly Typed | 03:19 |
51 | JTS: Static Typing In JavaScript | 09:46 |
52 | Section Overview | 01:55 |
53 | Functions are Objects | 09:19 |
54 | First Class Citizens | 04:05 |
55 | Extra Bits: Functions | 03:26 |
56 | Higher Order Functions | 17:15 |
57 | Exercise: Higher Order Functions | 03:46 |
58 | Closures | 15:12 |
59 | Exercise: Closures | 03:19 |
60 | Closures and Memory | 07:53 |
61 | Closures and Encapsulation | 07:42 |
62 | Exercise: Closures 2 | 01:32 |
63 | Solution: Closures 2 | 02:35 |
64 | Exercise: Closures 3 | 01:29 |
65 | Solution: Closures 3 | 03:48 |
66 | Closures Review | 01:35 |
67 | Prototypal Inheritance | 06:48 |
68 | Prototypal Inheritance 2 | 09:08 |
69 | Prototypal Inheritance 3 | 07:47 |
70 | Prototypal Inheritance 4 | 10:36 |
71 | Prototypal Inheritance 5 | 02:44 |
72 | Prototypal Inheritance 6 | 09:17 |
73 | Exercise: Prototypal Inheritance | 02:57 |
74 | Solution: Prototypal Inheritance | 08:22 |
75 | Section Review | 03:58 |
76 | Section Overview | 06:25 |
77 | OOP and FP | 03:51 |
78 | OOP Introduction | 03:22 |
79 | OOP1: Factory Functions | 08:21 |
80 | OOP2: Object.create() | 07:39 |
81 | OOP3: Constructor Functions | 13:02 |
82 | More Constructor Functions | 08:39 |
83 | Funny Thing About JS... | 02:53 |
84 | OOP4: ES6 Classes | 08:45 |
85 | Object.create() vs Class | 01:49 |
86 | this - 4 Ways | 04:45 |
87 | Inheritance | 14:42 |
88 | Inheritance 2 | 05:26 |
89 | Public vs Private | 04:22 |
90 | OOP in React.js | 03:06 |
91 | 4 Pillars of OOP | 08:18 |
92 | Reviewing OOP | 02:07 |
93 | Section Overview | 02:30 |
94 | Functional Programming Introduction | 03:32 |
95 | Exercise: Amazon | 03:38 |
96 | Pure Functions | 04:36 |
97 | Pure Functions 2 | 07:48 |
98 | Can Everything Be Pure? | 04:34 |
99 | Idempotent | 04:09 |
100 | Imperative vs Declarative | 06:29 |
101 | Immutability | 05:29 |
102 | Higher Order Functions and Closures | 07:50 |
103 | Currying | 04:25 |
104 | Partial Application | 04:25 |
105 | MCI: Memoization 1 | 07:48 |
106 | MCI: Memoization 2 | 03:57 |
107 | Compose and Pipe | 12:34 |
108 | Arity | 01:47 |
109 | Is FP The Answer To Everything? | 02:55 |
110 | Solution: Amazon | 21:49 |
111 | Reviewing FP | 05:15 |
112 | Composition vs Inheritance | 16:21 |
113 | OOP vs FP | 04:30 |
114 | OOP vs FP 2 | 05:20 |
115 | Section Overview | 04:52 |
116 | How JavaScript Works | 24:13 |
117 | Promises | 22:27 |
118 | ES8 - Async Await | 15:23 |
119 | ES9 (ES2018) | 05:22 |
120 | ES9 (ES2018) - Async | 11:12 |
121 | Job Queue | 06:56 |
122 | Parallel, Sequence and Race | 10:01 |
123 | ES2020: allSettled() | 04:23 |
124 | Threads, Concurrency and Parallelism | 11:28 |
125 | Section Overview | 02:45 |
126 | What Is A Module? | 11:06 |
127 | Module Pattern | 13:07 |
128 | Module Pattern Pros and Cons | 04:54 |
129 | CommonJS, AMD, UMD | 10:06 |
130 | ES6 Modules | 08:54 |
131 | Section Review | 02:15 |
132 | Section Overview | 01:08 |
133 | Errors In JavaScript | 08:53 |
134 | Try Catch | 09:54 |
135 | Async Error Handling | 12:57 |
136 | Async Error Handling 2 | 03:31 |
137 | Extending Errors | 05:09 |
138 | Section Review | 01:19 |
139 | Thank You | 00:40 |
140 | Section Overview | 01:59 |
141 | What Is A Data Structure? | 05:54 |
142 | How Computers Store Data | 12:35 |
143 | Data Structures In Different Languages | 03:29 |
144 | Operations On Data Structures | 03:06 |
145 | Array Introduction | 13:52 |
146 | Static vs Dynamic Arrays | 06:41 |
147 | Implementing An Array | 17:20 |
148 | Strings and Arrays | 01:05 |
149 | Exercise: Reverse A String | 01:36 |
150 | Solution Reverse A String | 10:32 |
151 | Exercise: Merge Sorted Arrays | 00:45 |
152 | Solution: Merge Sorted Arrays | 14:13 |
153 | Arrays Review | 03:29 |
154 | Hash Tables Introduction | 04:11 |
155 | Hash Function | 05:57 |
156 | Hash Collisions | 09:44 |
157 | Hash Tables In Different Languages | 03:31 |
158 | Exercise: Implement A Hash Table | 03:52 |
159 | Solution: Implement A Hash Table | 17:26 |
160 | keys() | 06:12 |
161 | Hash Tables vs Arrays | 02:02 |
162 | Exercise: First Recurring Character | 01:19 |
163 | Solution: First Recurring Character | 16:12 |
164 | Hash Tables Review | 06:10 |
165 | What is JavaScript? | 05:34 |
166 | Your First JavaScript | 11:42 |
167 | Variables | 15:10 |
168 | Control Flow | 16:37 |
169 | JavaScript On Our Webpage | 09:06 |
170 | Functions | 23:54 |
171 | Data Structures: Arrays | 13:07 |
172 | Data Structures: Objects | 15:26 |
173 | Exercise: Build Facebook | 11:17 |
174 | JavaScript Terminology | 03:44 |
175 | Loops | 22:23 |
176 | Exercise: Build Facebook 2 | 08:04 |
177 | JavaScript Keywords | 03:03 |
178 | Scope | 10:27 |
179 | Advanced Control Flow | 11:43 |
180 | ES5 and ES6 | 26:42 |
181 | Advanced Functions | 20:05 |
182 | Advanced Arrays | 16:41 |
183 | Advanced Objects | 24:53 |
184 | ES7 | 03:07 |
185 | ES8 | 09:20 |
186 | ES10 (ES2019) | 16:50 |
187 | Advanced Loops | 12:05 |
188 | ES2020 Part 1 | 10:08 |
189 | ES2020 Part 2 | 03:30 |
190 | ES2020 Part 3 | 04:12 |
191 | ES2020: globalThis | 03:15 |
192 | ES2021 | 03:09 |
193 | Debugging | 08:24 |
194 | Modules | 13:20 |
195 | AMA - 100,000 Students!! | 38:31 |
Similar courses to JavaScript: The Advanced Concepts
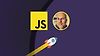
Animating with the JavaScript Web Animations API
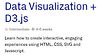
Data Visualization + D3.js
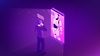
Build Incredible Chatbots
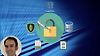
Web security: Injection Attacks with Java & Spring Boot
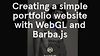
Creating a simple portfolio website with WebGL and Barba.js
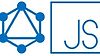
GraphQL for beginners with JavaScript
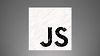
JavaScript: Understanding the Weird Parts
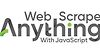
Web Scrape Anything With JavaScript
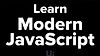