JavaScript Algorithms and Data Structures Masterclass
This course crams months of computer science and interview prep material into 20 hours of video. The content is based directly on last semester of my in-person coding bootcamps, where my students go on to land 6-figure developer jobs. I cover the exact same computer science content that has helped my students ace interviews at huge companies like Google, Tesla, Amazon, and Facebook. Nothing is watered down for an online audience; this is the real deal :) We start with the basics and then eventually cover “advanced topics” that similar courses shy away from like Heaps, Graphs, and Dijkstra’s Shortest Path Algorithm.
Read more about the course
I start by teaching you how to analyze your code’s time and space complexity using Big O notation. We cover the ins and outs of Recursion. We learn a 5-step approach to solving any difficult coding problem. We cover common programming patterns. We implement popular searching algorithms. We write 6 different sorting algorithms: Bubble, Selection, Insertion, Quick, Merge, and Radix Sort. Then, we switch gears and implement our own data structures from scratch, including linked lists, trees, heaps, hash tables, and graphs. We learn to traverse trees and graphs, and cover Dijkstra's Shortest Path Algorithm. The course also includes an entire section devoted to Dynamic Programming.
Here's why this course is worth your time:
It's interactive - I give you a chance to try every problem before I show you my solution.
Every single problem has a complete solution walkthrough video as well as accompanying solution file.
I cover helpful "tips and tricks" to solve common problems, but we also focus on building an approach to ANY problem.
It's full of animations and beautiful diagrams!
Watch Online JavaScript Algorithms and Data Structures Masterclass
# | Title | Duration |
---|---|---|
1 | Curriculum Walkthrough | 07:44 |
2 | What Order Should You Watch In? | 02:53 |
3 | How I'm Running My Code | 03:22 |
4 | Intro to Big O | 07:42 |
5 | Timing Our Code | 10:20 |
6 | Counting Operations | 04:37 |
7 | Visualizing Time Complexities | 04:26 |
8 | Official Intro to Big O | 09:59 |
9 | Simplifying Big O Expressions | 09:33 |
10 | Space Complexity | 06:27 |
11 | Logs and Section Recap | 08:47 |
12 | Section Introduction | 01:43 |
13 | The BIG O of Objects | 05:32 |
14 | When are Arrays Slow? | 06:26 |
15 | Big O of Array Methods | 05:57 |
16 | Introduction to Problem Solving | 07:09 |
17 | Step 1: Understand The Problem | 08:00 |
18 | Step 2: Concrete Examples | 06:20 |
19 | Step 3: Break It Down | 07:45 |
20 | Step 4: Solve Or Simplify | 10:33 |
21 | Step 5: Look Back and Refactor | 16:58 |
22 | Recap and Interview Strategies | 04:13 |
23 | Intro to Problem Solving Patterns | 02:56 |
24 | Frequency Counter Pattern | 15:12 |
25 | Frequency Counter: Anagram Challenge | 02:34 |
26 | Anagram Challenge Solution | 06:19 |
27 | Multiple Pointers Pattern | 09:43 |
28 | Multiple Pointers: Count Unique Values Challenge | 04:30 |
29 | Count Unique Values Solution | 06:31 |
30 | Sliding Window Pattern | 13:15 |
31 | Divide And Conquer Pattern | 07:03 |
32 | Story Time: Martin & The Dragon | 07:07 |
33 | Why Use Recursion? | 05:54 |
34 | The Call Stack | 07:08 |
35 | Our First Recursive Function | 05:12 |
36 | Our Second Recursive Function | 07:55 |
37 | Writing Factorial Iteratively | 02:20 |
38 | Writing Factorial Recursively | 03:16 |
39 | Common Recursion Pitfalls | 05:07 |
40 | Helper Method Recursion | 06:24 |
41 | Pure Recursion | 07:46 |
42 | Intro to Searching | 04:05 |
43 | Intro to Linear Search | 04:48 |
44 | Linear Search Solution | 05:19 |
45 | Linear Search BIG O | 01:56 |
46 | Intro to Binary Search | 05:48 |
47 | Binary Search PseudoCode | 02:41 |
48 | Binary Search Solution | 16:42 |
49 | Binary Search BIG O | 06:10 |
50 | Naive String Search | 04:39 |
51 | Naive String Search Implementation | 12:30 |
52 | Introduction to Sorting Algorithms | 08:36 |
53 | Built-In JavaScript Sorting | 04:41 |
54 | Bubble Sort: Overview | 07:22 |
55 | Bubble Sort: Implementation | 09:59 |
56 | Bubble Sort: Optimization | 04:23 |
57 | Bubble Sort: BIG O Complexity | 01:29 |
58 | Selection Sort: Introduction | 06:19 |
59 | Selection Sort: Implementation | 11:15 |
60 | Selection Sort: Big O Complexity | 01:41 |
61 | Insertion Sort: Introduction | 03:18 |
62 | Insertion Sort: Implementation | 10:43 |
63 | Insertion Sort: BIG O Complexity | 02:25 |
64 | Comparing Bubble, Selection, and Insertion Sort | 05:34 |
65 | Intro to the "Crazier" Sorts | 06:06 |
66 | Merge Sort: Introduction | 05:26 |
67 | Merging Arrays Intro | 05:12 |
68 | Merging Arrays: Implementation | 06:56 |
69 | Writing Merge Sort Part 1 | 02:22 |
70 | Writing Merge Sort Part 2 | 12:38 |
71 | Merge Sort BIG O Complexity | 06:23 |
72 | Introduction to Quick Sort | 09:01 |
73 | Pivot Helper Introduction | 08:07 |
74 | Pivot Helper Implementation | 08:09 |
75 | Quick Sort Implementation | 08:47 |
76 | Quick Sort Call Stack Walkthrough | 04:16 |
77 | Quick Sort Big O Complexity | 04:07 |
78 | Radix Sort: Introduction | 09:23 |
79 | Radix Sort: Helper Methods | 11:10 |
80 | Radix Sort: Pseudocode | 04:19 |
81 | Radix Sort: Implementation | 10:25 |
82 | Radix Sort: BIG O Complexity | 03:52 |
83 | Which Data Structure Is The Best? | 12:39 |
84 | ES2015 Class Syntax Overview | 05:15 |
85 | Data Structures: The Class Keyword | 06:37 |
86 | Data Structures: Adding Instance Methods | 09:50 |
87 | Data Structures: Adding Class Methods | 07:12 |
88 | Intro to Singly Linked Lists | 07:47 |
89 | Starter Code and Push Intro | 07:23 |
90 | Singly Linked List: Push Solution | 04:25 |
91 | Singly Linked List: Pop Intro | 06:15 |
92 | Singly Linked List: Pop Solution | 07:36 |
93 | Singly Linked List: Shift Intro | 01:32 |
94 | Singly Linked List: Shift Solution | 03:23 |
95 | Singly Linked List: Unshift Intro | 01:35 |
96 | Singly Linked List: Unshift Solution | 05:59 |
97 | Singly Linked List: Get Intro | 02:33 |
98 | Singly Linked List: Get Solution | 03:33 |
99 | Singly Linked List: Set Intro | 01:27 |
100 | Singly Linked List: Set Solution | 02:11 |
101 | Singly Linked List: Insert Intro | 04:28 |
102 | Singly Linked List: Insert Solution | 07:50 |
103 | Singly Linked List: Remove Intro | 01:57 |
104 | Singly Linked List: Remove Solution | 03:16 |
105 | Singly Linked List: Reverse Intro | 04:47 |
106 | Singly Linked List: Reverse Solution | 08:59 |
107 | Singly Linked List: BIG O Complexity | 05:42 |
108 | Doubly Linked Lists Introduction | 04:44 |
109 | Setting Up Our Node Class | 03:01 |
110 | Push | 02:11 |
111 | Push Solution | 04:05 |
112 | Pop | 03:21 |
113 | Pop Solution | 06:24 |
114 | Shift | 02:45 |
115 | Shift Solution | 04:13 |
116 | Unshift | 01:37 |
117 | Unshift Solution | 02:20 |
118 | Get | 04:03 |
119 | Get Solution | 07:05 |
120 | Set | 01:19 |
121 | Set Solution | 02:09 |
122 | Insert | 02:51 |
123 | Insert Solution | 06:49 |
124 | Remove | 02:19 |
125 | Remove Solution | 06:29 |
126 | Comparing Singly and Doubly Linked Lists | 04:33 |
127 | Intro to Stacks | 06:20 |
128 | Creating a Stack with an Array | 07:06 |
129 | Writing Our Own Stack From Scratch | 11:34 |
130 | BIG O of Stacks | 02:15 |
131 | Intro to Queues | 04:15 |
132 | Creating Queues Using Arrays | 03:26 |
133 | Writing Our Own Queue From Scratch | 10:25 |
134 | BIG O of Queues | 02:31 |
135 | Introduction to Trees | 06:46 |
136 | Uses For Trees | 06:33 |
137 | Intro to Binary Trees | 05:55 |
138 | POP QUIZ! | 01:14 |
139 | Searching A Binary Search Tree | 02:56 |
140 | Our Tree Classes | 02:45 |
141 | BST: Insert | 03:51 |
142 | BST: Insert Solution | 11:54 |
143 | BST: Find | 04:43 |
144 | BST: Find Solution | 05:37 |
145 | Big O of Binary Search Trees | 05:59 |
146 | Intro To Tree Traversal | 04:51 |
147 | Breadth First Search Intro | 05:52 |
148 | Breadth First Search Solution | 06:21 |
149 | Depth First PreOrder Intro | 05:38 |
150 | Depth First PreOrder Solution | 06:51 |
151 | Depth First PostOrder Intro | 04:03 |
152 | Depth First PostOrder Solution | 02:39 |
153 | Depth First InOrder Intro | 02:08 |
154 | Depth First InOrder Solution | 02:33 |
155 | When to Use BFS and DFS | 07:38 |
156 | Intro to Heaps | 07:31 |
157 | Storing Heaps | 07:06 |
158 | Heap: Insert Intro | 09:15 |
159 | Heap: Insert Solution | 10:52 |
160 | Heap: ExtractMax Intro | 08:29 |
161 | Heap: ExtractMax Solution | 17:57 |
162 | Priority Queue Intro | 09:00 |
163 | Priority Queue Pseudocode | 03:44 |
164 | Priority Queue Solution | 09:22 |
165 | BIG O of Binary Heaps | 08:55 |
166 | Intro to Hash Tables | 05:51 |
167 | More About Hash Tables | 04:33 |
168 | Intro to Hash Functions | 06:12 |
169 | Writing Our First Hash Function | 08:28 |
170 | Improving Our Hash Function | 07:11 |
171 | Handling Collisions | 04:00 |
172 | Hash Table Set and Get | 04:03 |
173 | Hash Table Set Solution | 05:15 |
174 | Hash Table Get Solution | 06:44 |
175 | Hash Table Keys and Values | 01:42 |
176 | Hash Table Keys and Values Solution | 08:44 |
177 | Hash Table Big O Complexity | 05:42 |
178 | Intro to Graphs | 03:51 |
179 | Uses for Graphs | 07:58 |
180 | Types of Graphs | 08:49 |
181 | Storing Graphs: Adjacency Matrix | 03:58 |
182 | Storing Graphs: Adjacency List | 02:30 |
183 | Adjacency Matrix Vs. List BIG O | 05:52 |
184 | Add Vertex Intro | 02:11 |
185 | Add Vertex Solution | 02:55 |
186 | Add Edge Intro | 02:33 |
187 | Add Edge Solution | 02:12 |
188 | Remove Edge Intro | 01:36 |
189 | Remove Edge Solution | 02:42 |
190 | Remove Vertex Intro | 02:36 |
191 | Remove Vertex Solution | 04:35 |
192 | Intro to Graph Traversal | 08:39 |
193 | Depth First Graph Traversal | 08:31 |
194 | DFS Recursive Intro | 07:28 |
195 | DFS Recursive Solution | 12:46 |
196 | DFS Iterative Intro | 03:38 |
197 | DFS Iterative Solution | 08:45 |
198 | Breadth First Graph Traversal | 03:00 |
199 | BFS Intro | 02:28 |
200 | BFS Solution | 08:10 |
201 | Intro to Dijkstra's and Prerequisites | 02:42 |
202 | Who was Dijkstra and what is his Algorithm? | 09:01 |
203 | Writing a Weighted Graph | 05:21 |
204 | Walking through the Algorithm | 16:27 |
205 | Introducing Our Simple Priority Queue | 03:49 |
206 | Dijkstra's Pseudo-Code | 04:29 |
207 | Implementing Dijkstra's Algorithm | 21:19 |
208 | Upgrading the Priority Queue | 01:53 |
209 | Intro to Dynamic Programming | 05:04 |
210 | Overlapping Subproblems | 06:00 |
211 | Optimal Substructure | 06:29 |
212 | Writing A Recursive Solution | 06:44 |
213 | Time Complexity of Our Solution | 04:12 |
214 | The Problem With Our Solution | 03:40 |
215 | Enter Memoization! | 09:01 |
216 | Time Complexity of Memoized Solution | 03:28 |
217 | Tabulation: A Bottom Up Approach | 07:00 |
Similar courses to JavaScript Algorithms and Data Structures Masterclass
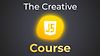
The Creative Javascript Coursedevelopedbyed.com
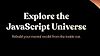
Explore the JavaScript Universe (Explore the JavaScript Universe)Dan Abramov
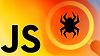
Mastering JavaScript Unit Testingcodewithmosh (Mosh Hamedani)
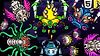
Remake Retro Games with JavaScriptudemy
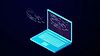
Full Stack Isomorphic JavaScript with Vue.js & Node.jsudemy
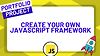