Hibernate and Spring Data JPA: Beginner to Guru
Hibernate is the default JPA implementation used by Spring Data JPA.
NOTE: Java 17 and Spring Boot 3 are required for this course.
JPA stands for Java Persistence API. This is a common Java API used to work with Relational Databases.
Spring Data JPA is an abstraction built on top of the JPA API specification.
Being an abstraction, Spring Data JPA makes working with database entities very efficient.
Spring Data JPA eliminates a lot of the boilerplate / cerimonial code, and allows developers to focus on developing business logic.
Read more about the course
The downside of the efficient abstraction is that accessing the database can become a mystery. Developers who just understand how to use Spring Data JPA do not understand the complexities of JDBC and Hibernate.
You will start this course with a basic demonstration of Spring Data JPA. In this section you will learn how to work with a H2 in-memory database.
You'll see how easy it is to work with Spring Data JPA. You will also begin to understand how the Hibernate interaction is being abstracted away.
Since JPA is the Java API for working with Relational Databases, the course takes a closer look at Relational Databases and MySQL specifically.
MySQL is the most popular open source relational database in the world. You will learn how to configure Spring Boot to test with a H2 in-memory database and to run integration tests against a MySQL database. This is a common real-world example leveraging the power of Spring and Hibernate to give you a very flexible environment.
Once we've established a persistent database, we can explore using database migration tools.
Liquibase and Flyway are two very popular database migration tools. Spring Boot supports both options. And you will learn about both options and database security best practices.
By establishing a MySQL database, Spring Boot Integration Tests, and automated database migrations we can use Test Driven Development to explore the features of JDBC and Hibernate.
In the course you will learn:
What is the DAO pattern, and how to implement it using JDBC, Spring's JDBCTemplate, and Hibernate
Relational Database Principles
Schema Creation in MySQL
Schema Generation using Hibernate
Database Migrations using Liquibase
Database Migrations using Flyway
Database Integration Testing using Spring Boot and JUnit 5
Defining Primary Key's with Hibernate
Hibernate Criteria Queries
Named JPA Queries
Spring Data JPA query methods
Spring Data JPA @Query Annotation
Entity Relationships - One to One, One to Many, Many to One, Many to Many
Embedded Types
Natural Keys
Composite Keys
Spring Data JPA Query Methods
Paging and Sorting
Database Transaction Management
Database Fetch Operations
Data Validation
JPA Inheritance
Hibernate Interceptors and Listeners
JPA Callbacks
Legacy Database Mapping
Using Multiple Data Sources
Spring Data REST
Watch Online Hibernate and Spring Data JPA: Beginner to Guru
# | Title | Duration |
---|---|---|
1 | Introduction | 02:13 |
2 | Getting the Most out of this Course | 05:16 |
3 | Setting up your Development Environment | 05:09 |
4 | GitHub Work Flow | 12:21 |
5 | Is your IDE Free Like A Puppy? | 02:46 |
6 | Introduction | 02:04 |
7 | Introduction to Spring Data JPA | 14:33 |
8 | Use Spring Initializr to Create Project | 06:16 |
9 | JPA Entities | 06:05 |
10 | Equality in Hibernate | 03:53 |
11 | Spring Data Repositories | 06:44 |
12 | Initializing Data with Spring | 08:05 |
13 | SQL Logging | 05:17 |
14 | H2 Database Console | 03:47 |
15 | Introduction | 01:23 |
16 | What is a Database? | 04:22 |
17 | Relational Database Principles | 12:09 |
18 | What is a Relational Database Management System | 08:37 |
19 | History of MySQL | 05:09 |
20 | RDBMS Deployment Architectures | 06:43 |
21 | Data Mapping SQL to Java | 06:36 |
22 | Create Schema and User for Spring Boot | 12:08 |
23 | Introduction | 01:32 |
24 | Spring Boot Test | 04:46 |
25 | Spring Boot JPA Test Splice | 05:22 |
26 | Test Transactions | 08:06 |
27 | Bootstrapping Data | 04:05 |
28 | Introduction | 01:19 |
29 | Hibernate DDL Update Modes | 07:44 |
30 | MySQL Spring Boot Configuration | 08:12 |
31 | Integration Test for MySQL | 09:39 |
32 | H2 MySQL Compatibility Mode | 07:43 |
33 | Schema Initialization with Hibernate | 06:08 |
34 | Schema Initialization with MySQL | 03:09 |
35 | Use H2 for Spring Boot Application | 04:50 |
36 | Introduction | 01:18 |
37 | Overview of Liquibase | 11:09 |
38 | Liquibase Maven Plugin | 07:41 |
39 | Generate Changeset from Database | 05:29 |
40 | Organizing Change Logs | 05:51 |
41 | Spring Boot Configuration | 07:46 |
42 | Initializing Data with Spring | 06:43 |
43 | Alter Table with Liquibase | 08:47 |
44 | Introduction | 00:55 |
45 | Overview of Flyway | 10:06 |
46 | Spring Boot Configuration | 10:17 |
47 | Alter Table with Flyway | 05:25 |
48 | Clean and Rebuild with Flyway | 04:47 |
49 | Introduction | 01:53 |
50 | Hibernate Primary Keys Overview | 06:53 |
51 | Auto Incremented Primary Key | 06:28 |
52 | Vendor Specific Flyway Migrations | 06:43 |
53 | UUID Primary Key | 07:40 |
54 | UUID RFC 4122 Primary Key | 07:14 |
55 | H2 Workaround | 05:48 |
56 | Natural Primary Key | 05:34 |
57 | Composite Primary Key | 06:38 |
58 | Embedded Composite Primary Key | 07:13 |
59 | Introduction | 02:14 |
60 | Introduction to DAO Pattern | 05:12 |
61 | Create Author DAO | 08:47 |
62 | Implement Get Author By Id | 07:55 |
63 | Release Database Resources | 05:45 |
64 | IntelliJ Database Configuration | 03:50 |
65 | Using Prepared Statements | 06:33 |
66 | Refactoring Duplicate Code | 05:28 |
67 | Save New Author | 08:30 |
68 | Update Author | 05:45 |
69 | Delete Author | 06:27 |
70 | Refactor Author id to Author | 10:08 |
71 | Introduction | 01:25 |
72 | Introduction to Spring JDBC Template | 02:40 |
73 | Create Row Mapper | 03:37 |
74 | Implement Get Author By Id | 04:54 |
75 | Implement Find Author By Name | 02:25 |
76 | Save New Author | 06:32 |
77 | Update Author | 03:42 |
78 | Delete Author | 04:00 |
79 | Implement Author with List of Books | 16:12 |
80 | Introduction | 01:02 |
81 | Introduction to Hibernate | 08:53 |
82 | Project Code Review | 04:07 |
83 | Implement Get Author By Id | 05:19 |
84 | Implement Find Author By Name | 07:05 |
85 | Save New Author | 06:59 |
86 | Update Author | 04:46 |
87 | Delete Author | 03:55 |
88 | Introduction | 00:52 |
89 | Query | 04:25 |
90 | Typed Query | 04:08 |
91 | Named Query | 04:41 |
92 | Named Query with Parameters | 05:01 |
93 | Criteria Query | 09:17 |
94 | Native SQL Queries | 07:13 |
95 | Introduction | 01:16 |
96 | Spring Data JPA Query Methods | 03:33 |
97 | Project Code Review | 03:14 |
98 | Author CRUD Operations | 06:59 |
99 | Query Methods | 03:23 |
100 | Optional Return Type | 03:37 |
101 | Null Handling | 05:54 |
102 | Stream Query Results | 04:37 |
103 | Asynchronous Query Results | 04:31 |
104 | Declaring Queries Using @Query | 03:21 |
105 | Named Parameters with @Query | 03:10 |
106 | Native SQL Queries | 03:05 |
107 | JPA Named Queries | 03:44 |
108 | Introduction | 01:06 |
109 | Overview of Paging and Sorting | 04:23 |
110 | JDBCTemplate Code Review | 02:43 |
111 | Find All Books with JDBCTemplate | 04:19 |
112 | Find All Books with Paging | 05:27 |
113 | Find All Books Using Pagable | 06:27 |
114 | Find All Books Order By Title | 10:04 |
115 | Hibernate Code Review | 02:13 |
116 | Paging with Hibernate | 05:14 |
117 | Sorting with Hibernate | 04:04 |
118 | Paging with Spring Data JPA | 06:30 |
119 | Sorting with Spring Data JPA | 05:03 |
120 | Query, Paging, and Sorting with Spring Data JPA | 05:23 |
121 | Introduction | 01:28 |
122 | JPA Inheritance | 10:23 |
123 | JPA Mapped Super Class | 06:07 |
124 | Embedded Types | 11:36 |
125 | Java Enumerated Types | 05:19 |
126 | Hibernate Created Date | 03:50 |
127 | Hibernate Update Date | 03:32 |
128 | Introduction | 01:17 |
129 | Overview of Database Relationships | 10:43 |
130 | One to Many | 08:46 |
131 | Cascade on Persist | 08:07 |
132 | Many to One Unidirectional | 06:50 |
133 | Association Helper Methods | 04:17 |
134 | Many to Many | 12:31 |
135 | JPA Buddy Plugin | 06:49 |
136 | One to One | 05:20 |
137 | Cascade Delete | 06:12 |
138 | Orphan Removal | 06:00 |
139 | One to One Bi-Directional | 06:36 |
140 | Hibernate Cascade Types | 03:17 |
141 | Introduction | 01:04 |
142 | Load Test Data | 18:10 |
143 | Load Test Data Fix | 05:40 |
144 | Lazy vs Eager Fetch | 08:54 |
145 | Hibernate N + 1 Problem | 13:19 |
146 | Introduction | 01:12 |
147 | Overview of Database Transactions | 18:48 |
148 | Database Locking Demo | 07:44 |
149 | Spring Data JPA Transactions | 18:50 |
150 | Create Bootstrap Class | 03:30 |
151 | Lazy Initialize Error | 05:27 |
152 | Transactional Proxy Mode | 05:25 |
153 | Adding Version Property | 04:49 |
154 | Optimistic Locking Demo | 06:55 |
155 | Version Property Cannot Be Null | 05:15 |
156 | Pessimistic Locking | 09:27 |
157 | Introduction | 01:05 |
158 | Java Bean Validation Overview | 11:02 |
159 | Java Bean Validation Maven Dependencies | 02:36 |
160 | Adding Validation | 08:40 |
161 | When and Where to Use Validation? | 05:41 |
162 | Introduction | 01:18 |
163 | Overview of JPA Inheritance | 03:23 |
164 | Project Code Review | 02:00 |
165 | Mapped Super Class | 04:29 |
166 | Table Per Class | 06:41 |
167 | Single Table | 05:43 |
168 | Joined Table | 07:43 |
169 | Introduction | 02:18 |
170 | Overview | 03:04 |
171 | Initial Project Creation | 04:04 |
172 | Database Setup | 02:04 |
173 | Spring Configuration | 04:16 |
174 | Flyway Initialization Script | 04:39 |
175 | Table and Column Naming | 05:51 |
176 | LOB Columns | 02:46 |
177 | Basic Annotation | 02:51 |
178 | Column Properties | 05:11 |
179 | Database Indexes | 03:27 |
180 | Refactor for Bi-Directional Association | 04:14 |
181 | Summary | 03:10 |
182 | Introduction | 01:31 |
183 | Overview of Listeners and Interceptors | 04:24 |
184 | Project Code Review | 05:05 |
185 | Initial Persistence Test | 05:36 |
186 | Encryption Service | 05:19 |
187 | Verify Data at Rest | 04:16 |
188 | Create Hibernate Interceptor | 06:19 |
189 | Custom Encryption Annotation | 01:46 |
190 | Implement Encryption Interceptor | 08:23 |
191 | Introduction | 01:17 |
192 | Remove Listener Example | 02:15 |
193 | Create Hibernate Listeners | 02:20 |
194 | Register Hibernate Listeners | 08:12 |
195 | Implement Hibernate Encryption Listeners | 05:57 |
196 | Introduction | 02:07 |
197 | Disable Hibernate Event Listeners | 01:36 |
198 | JPA Callback on Entity Methods | 02:56 |
199 | JPA Callback Listener | 03:33 |
200 | Spring Context Helper | 03:16 |
201 | Implement Callback Encryption | 03:09 |
202 | JPA Converters | 04:56 |
203 | Introduction | 01:52 |
204 | Project Code Review | 02:51 |
205 | Adding Project Lombok | 06:28 |
206 | My SQL Configuration | 02:51 |
207 | Data Source Properties | 04:23 |
208 | Create Data Source Connections | 03:11 |
209 | Create Entity Managers | 04:42 |
210 | Create Transaction Managers | 02:54 |
211 | Spring Data JPA Repository Configuration | 04:24 |
212 | Create Flyway Migration Scripts | 04:31 |
213 | Flyway Datasource Configuration | 03:27 |
214 | Custom Flyway Migrations | 03:43 |
215 | Flyway Migration Testing | 04:09 |
216 | Hikari Database Pooling Properties | 04:34 |
217 | Configure Hibernate Schema Validation | 03:39 |
218 | Configure Hibernate Naming Conventions | 06:20 |
219 | Hibernate Transient Properties | 02:45 |
220 | Create Credit Card Id References | 04:47 |
221 | Save New Credit Card | 05:45 |
222 | Get Credit Card by Id | 05:00 |
223 | Summary | 02:49 |
224 | Introduction | 02:15 |
225 | Project Code Review | 04:58 |
226 | Add Spring Data REST Dependency | 02:39 |
227 | List All With Spring Data REST | 04:26 |
228 | Set Base Path | 01:54 |
229 | Customize URL Path | 04:57 |
230 | Version Property - ETag Header | 02:47 |
231 | API Profile | 03:46 |
232 | Create with Spring Data REST | 02:40 |
233 | Update Data with Spring Data REST | 02:14 |
234 | Delete Data with Spring Data REST | 01:52 |
235 | Use Repository Methods | 02:47 |
236 | GitHub Workflow | 12:21 |
237 | Restful Web Services with Spring MVC | 49:48 |
238 | Dependency Injection Best Practices | 18:51 |
239 | Introduction to Testing with Spring | 17:29 |
240 | Introduction to MockMVC | 04:37 |
241 | Building a Spring Boot Application | 30:33 |
242 | Kube By Example - Building a Spring Boot Docker Images | 56:34 |
Similar courses to Hibernate and Spring Data JPA: Beginner to Guru
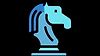
Master Spring 6 Spring Boot 3 REST JPA Hibernateudemy

Master Spring Boot 3 & Spring Framework 6 with Javaudemy
![[NEW] Spring Boot 3, Spring 6 & Hibernate for Beginners](https://cdn.courseflix.net/courses/100x56/new-spring-boot-3-spring-6-hibernate-for-beginners.jpg?d=1743664567230)
[NEW] Spring Boot 3, Spring 6 & Hibernate for Beginnersudemy
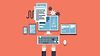