Blazor Bootcamp - .NET 6 (WASM and Server)
This course will provide you a complete real world scenario with Blazor which will make you face many challenges and solve those issues as we proceed with the course. There are other courses on Udemy, but this is the ultimate course, it covers everything there is to Blazor from Blazor Server to Blazor WASM applications which consumes .NET 6 API. This course will help developers transition from building basic sample apps to implementing more real world concepts, design patterns, and features.
Read more about the course
We will be using the latest .NET 6 for this course along with Entity Framework Core and Stripe for payment processing.
For years JavaScript frameworks have dominated the front end/client side development! But things are about to change with Blazor!
Blazor is an exciting new part of .NET Core (.NET 6) designed for building rich web user interfaces in C#. This course will help developers transition from building basic sample apps to implementing more real world concepts, design patterns, and features.
With that there are many questions:
What is Blazor? How do I get started with fundamentals of Blazor?
How do you scale an existing application?
How do you architect a mid-large scale project?
How to correctly process payments?
How to efficiently understand Blazor and use it in real world projects?
Watch Online Blazor Bootcamp - .NET 6 (WASM and Server)
# | Title | Duration |
---|---|---|
1 | Welcome | 03:08 |
2 | Project Demo | 08:19 |
3 | 5 What is Blazor | 04:23 |
4 | Blazor client vs Blazor server | 03:46 |
5 | What this course is not about | 02:18 |
6 | Create Project | 02:26 |
7 | Run the application | 03:47 |
8 | Source Control | 01:03 |
9 | Blazor Files Overview | 10:19 |
10 | Blazor Server Project Flow | 15:03 |
11 | Blazor Web Assembly Project Brief Overview | 04:38 |
12 | Understand the Default Home Page | 02:17 |
13 | Creating Razor Component | 04:51 |
14 | One way Data Binding | 04:11 |
15 | 3 Two Way Data Binding | 05:35 |
16 | 4 Assignment 1 - Data Binding | 01:06 |
17 | 5 Assignment 1 Solution - Data Binding | 03:34 |
18 | 6 Dropdowns and Data Binding | 06:25 |
19 | 7 Organizing Individual Product Playground | 04:57 |
20 | 8 Product List | 03:30 |
21 | 9 Assignment 2 - Bind Property | 01:24 |
22 | 10 Assignment 2 Solution - Bind Property | 05:19 |
23 | Assignment 3 - Razor Component | 01:30 |
24 | 12 Assignment 3 Solution - New Razor Component | 06:03 |
25 | 1 Create Shared Component | 04:55 |
26 | 2 Passing Props to Shared Component | 02:29 |
27 | 3 Assignment 4 - Shared Components | 00:58 |
28 | 4 Assignment 4 Solution - Shared Components | 01:28 |
29 | 5 Favourites CheckBox | 03:13 |
30 | 6 Event Callback | 07:09 |
31 | 7 Assignment 5 - Event Callback | 01:29 |
32 | 8 Assignment 5 Solution - Event Callback | 05:16 |
33 | 2. Render Fragment | 06:04 |
34 | 3 Another way for Basic Event Call Back | 03:29 |
35 | 4 Multiple Render Fragment | 03:13 |
36 | 5 Assignment 6 - Render Fragment | 00:42 |
37 | 6 Assignment 6 Solution - Render Fragment | 01:24 |
38 | 7 why we need attribute splatting | 05:21 |
39 | 8 Attribute Splatting | 02:42 |
40 | 9 - Capture all values with splatting | 02:34 |
41 | 10 Passing Parameters at multiple level | 03:36 |
42 | 11 Cascading Parameter | 03:12 |
43 | 12 Cascading Parameter with Name | 03:37 |
44 | 13 - Routing Basics | 05:16 |
45 | 14 Routing - Query Parameters | 05:34 |
46 | 15 Routing - Navigation Manager | 03:10 |
47 | 1 Confirm Box in Blazor | 05:14 |
48 | 2 Toastr JS | 07:23 |
49 | 3 JsRuntime Extensions | 05:20 |
50 | 4 Assignment 7 - SweetAlert | 01:13 |
51 | 5 Assignment 7 Solution - SweetAlert | 03:48 |
52 | 6 Referencing Components | 03:11 |
53 | 7 Remove Default Components | 02:57 |
54 | 2 OnInitialized Lifecycle | 07:21 |
55 | 3 OnParameterSet Lifecycle | 04:05 |
56 | 4 OnAfterRender | 05:52 |
57 | 5 Should Render and StateHasChanged | 05:59 |
58 | 2 Add Projects to Solution | 01:53 |
59 | 3 Setup ApplicationDbContext | 04:56 |
60 | 4 Add DbContext to Container | 05:51 |
61 | 5 Push Category to Database | 06:59 |
62 | 6 Category DTO | 03:19 |
63 | 8 ICategory Repository | 03:30 |
64 | 9 Category Repository Setup | 04:16 |
65 | 10 AutoMapper | 05:38 |
66 | 11 Implement Category Repository | 09:36 |
67 | 2 Create Category List Component | 04:09 |
68 | 3 Forms in Blazor Part 1 | 05:50 |
69 | 4 Forms in Blazor Part 2 | 01:47 |
70 | 5 Validations in Blazor Form | 06:30 |
71 | 6 Create Category | 02:45 |
72 | 7 List Category | 04:19 |
73 | 8 Loading Spinner | 04:50 |
74 | 9 Load Category on Edit | 04:23 |
75 | 2 Delete Confirmation Component | 06:23 |
76 | 3 Delete Method and Assignment 8 | 02:51 |
77 | 4 Assignment 8 - Delete Confirmation EventCallBack | 02:46 |
78 | Processing on Delete Confirmation | 03:32 |
79 | Cleaning Up Task | 04:41 |
80 | Assignment 9 - OnAfterRenderAsync | 00:34 |
81 | Assignment 9 Solution - OnAfterRenderAsync | 01:22 |
82 | Async Repository | 03:42 |
83 | 1 Section Introduction | 03:03 |
84 | 2 Create Product Table | 03:29 |
85 | 3 Product DTO | 01:36 |
86 | 4 Assignment 10 - Product Rpeository | 00:49 |
87 | 5 Assignment 10 Solution - Product Repository | 03:00 |
88 | 6 Product List Component | 05:01 |
89 | 7 Product Upsert Component | 06:17 |
90 | 8 Error Solving in Blazor | 02:57 |
91 | 9 Category Dropdown | 04:26 |
92 | 10 FileUpload Service | 07:19 |
93 | Handle File Upload | 04:29 |
94 | Create Product | 02:39 |
95 | Update Product | 02:14 |
96 | 14 Delete Product | 05:29 |
97 | 15 Syncfusion Components | 01:47 |
98 | 16 Syncfusion RichTextEditor in Action | 10:20 |
99 | 17 Create Products | 00:56 |
100 | What we will cover? | 01:12 |
101 | Product Price Model and DTO | 04:34 |
102 | Assignment 11 - Product Price Repository | 00:48 |
103 | Assignment 11 Solution - Product Price Repository | 02:43 |
104 | Load Product Details and Product Price | 06:23 |
105 | Show Product Summary | 03:51 |
106 | Add Syncfusion DataGrid | 04:03 |
107 | Syncfusion DataGrid Settings Part 1 | 05:38 |
108 | Syncfusion DataGrid Settings Part 2 | 04:56 |
109 | Syncfusion DataGrid Settings Part 3 | 03:27 |
110 | Syncfusion DataGrid Settings Part 4 | 07:06 |
111 | Create Tangy API | 02:14 |
112 | Add Connection String to API Project | 03:19 |
113 | Success Error DTO | 03:40 |
114 | Product Controller API Endpoint | 06:16 |
115 | Return Product Prices from Product API | 03:45 |
116 | Add AppSettings to WASM Project | 03:19 |
117 | Changing default navigation | 04:39 |
118 | Basic Settings like Server Project | 03:24 |
119 | Product Service Interface | 04:38 |
120 | Product Service Calls | 05:52 |
121 | Home Page UI | 04:29 |
122 | Retrieve Products from API | 06:27 |
123 | Home Page In Action | 05:54 |
124 | Details UI | 08:08 |
125 | Details VM | 03:51 |
126 | Selecting Product Price | 03:28 |
127 | Details UI - Add to Cart Toggle | 03:43 |
128 | Add Blazored Local Storage | 03:34 |
129 | Cart Service | 02:29 |
130 | Implement Cart Service | 08:48 |
131 | Cart Service Add to Cart in Action | 04:22 |
132 | Shopping Cart UI | 05:08 |
133 | Shopping Cart Load Data | 06:55 |
134 | Increment and Decrement Cart | 05:57 |
135 | Displaying Cart Counter | 03:47 |
136 | Update NavBar On Cart Update | 06:14 |
137 | Create Order Header and Detail | 07:23 |
138 | Create Order Model and DTO | 04:08 |
139 | Order Repository | 03:01 |
140 | Order Create Method | 05:52 |
141 | Order Repository Implementation | 09:18 |
142 | API - Create order controller | 02:21 |
143 | Order Service | 03:00 |
144 | Order Summary Part 1 | 03:24 |
145 | Order Summary Part 2 | 05:37 |
146 | Order Summary UI | 06:17 |
147 | Scaffold Identity in Blazor Server | 07:29 |
148 | Add Identity Tables | 05:14 |
149 | Add Columns to ASP Net Users Table | 03:23 |
150 | Register First User | 05:21 |
151 | Login and Logout | 07:03 |
152 | Show Email of Logged in User | 01:27 |
153 | Authorize Attribute | 03:12 |
154 | Authentication State in OnInitialized | 05:10 |
155 | Create Admin User | 07:17 |
156 | Roles in Action | 03:06 |
157 | DbInitializer | 06:53 |
158 | Seed New Database | 04:11 |
159 | Authorization In NavMenu | 02:32 |
160 | Add Identity to API | 01:59 |
161 | Account Controller | 02:04 |
162 | SignIn SignUp DTO | 04:58 |
163 | SignUp API Endpoint | 04:22 |
164 | Demo - SignUp API | 02:49 |
165 | SignIn API Endpoint Part 1 | 02:52 |
166 | API Settings Section | 05:02 |
167 | SignIn Helper Methods | 05:34 |
168 | Sign In Endpoint Part 2 | 06:27 |
169 | Add Authentication to API | 03:42 |
170 | Add Bearer to Swagger | 04:24 |
171 | JwtParser | 03:32 |
172 | Add AuthenticationState Provider | 05:23 |
173 | Custom Authentication State Demo | 05:37 |
174 | IAuthenticationSerivce | 01:49 |
175 | Login Service | 05:14 |
176 | Register and Logout Service | 01:51 |
177 | Register and Login UI | 02:00 |
178 | Register Component | 05:55 |
179 | Registration in Action | 03:40 |
180 | Splitting code files | 03:19 |
181 | Login in Action | 04:14 |
182 | Toggle Navbar on Authorization | 01:28 |
183 | Login and Logout in Action | 02:30 |
184 | Avoid force load on auth state change | 05:22 |
185 | Redirect to Login | 08:52 |
186 | Load User Details | 02:05 |
187 | Call Create Order From Summary | 06:45 |
188 | Create Order Header and Details | 03:52 |
189 | Create Stripe Account | 01:48 |
190 | Add Stripe to API Project | 02:43 |
191 | Payment Service in Blazor WASM | 03:52 |
192 | Stripe Payment Controller Method | 11:10 |
193 | Call Stripe | 07:11 |
194 | Issue with Order Total | 03:04 |
195 | Order Confirmation | 04:46 |
196 | Stripe Session Status and Payment Successful | 06:32 |
197 | Summary Processor | 00:55 |
198 | Create Order List Component | 05:36 |
199 | Radzen Component | 08:59 |
200 | Order Details Component | 04:58 |
201 | Order Details UI | 06:46 |
202 | Update Order Details | 04:27 |
203 | Ship Order | 02:23 |
204 | Payment Intent ID | 05:08 |
205 | Refund Order | 08:22 |
206 | Send Email using MailKit and MimeKit | 08:07 |
207 | Send Email using SendGrid | 05:13 |
208 | Blazor WebAssembly Package Issues | 02:13 |
209 | Azure SQL Database | 06:17 |
210 | Blazor Server Deployment | 05:59 |
211 | API Deployment | 03:31 |
212 | Blazor Client Deployment | 05:49 |
Similar courses to Blazor Bootcamp - .NET 6 (WASM and Server)
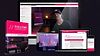
Full Time Game DevThomas Brush
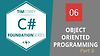
Foundation in C#: Object Oriented Programming Part 2iamtimcorey.com (Tim Corey)
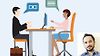
.NET/ C# Interview Masterclass - Top 500 Questions & Answersudemy
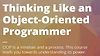
Thinking Like an Object-Oriented ProgrammerBob Tabor
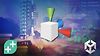