Building Applications with React 17 and ASP.NET Core 6
With ASP.NET Core we can develop Web APIs using C#. With React you can create modern, fast and flexible web applications. In this course we will use both tools to create a project. We will make an application with its database, user system, back-end and UI, where you will put into practice the concepts learned in the course. At the end we are going to publish our React application and our ASP.NET Core application.
Read more about the course
Some of the topics that we will see:
Web API development with ASP.NET Core
Database in SQL Server using Entity Framework Core
User system with Json Web Tokens (JWT)
Developing a Single Page Application (SPA) with React
Use React Hooks to create modern functional components
Creating forms using formik and yup
Make HTTP requests from React to ASP.NET Core using Axios
Create reusable components in React
Using React Router for browsing
Using maps with leaflet
Save spatial data to a database with NetTopologySuite
Allow users to upload images to be saved to Azure Storage or locally
We will configure CORS to allow our React application to communicate with our Web API
We will use environment variables, both in ASP.NET Core and React, so as not to hardcode the development and production URLs in our applications.
Upon completion of this course, you will have sufficient knowledge to face development challenges involving ASP.NET Core and React applications.
Watch Online Building Applications with React 17 and ASP.NET Core 6
# | Title | Duration |
---|---|---|
1 | Introduction | 00:58 |
2 | What is React? | 04:28 |
3 | What is ASP.NET Core? | 03:55 |
4 | Installing Node | 01:32 |
5 | Adding React and Using Create-React-App | 03:01 |
6 | Installing Visual Studio | 02:59 |
7 | Installing Visual Studio Code | 02:17 |
8 | Installing the EF Core CLI Tool | 01:22 |
9 | Front-End and Back-End | 02:23 |
10 | Creating the React App | 06:17 |
11 | Creating the Web API with Visual Studio | 02:18 |
12 | Creating the Web API with Visual Studio Code and the dotnet CLI | 03:12 |
13 | Reverting Back to the Old Model | 05:56 |
14 | Creating the Combined Project | 05:20 |
15 | Configuring the tsconfig.json | 00:45 |
16 | Summary | 01:17 |
17 | Introduction | 00:53 |
18 | Preparing the Development Environment | 02:39 |
19 | Let and Const | 08:58 |
20 | Arrow Functions and this | 11:35 |
21 | Template Strings | 05:32 |
22 | Ternary Operator | 03:04 |
23 | Enhanced Object Literals | 06:41 |
24 | Destructuring | 06:46 |
25 | Spread Operator - Arrays | 06:29 |
26 | Spread Operator - Objects | 05:25 |
27 | Classes | 08:16 |
28 | Map Function | 05:07 |
29 | Promises and Async Programming | 11:59 |
30 | Modules | 07:56 |
31 | Summary | 02:07 |
32 | Introduction | 01:23 |
33 | The Problem with HTML | 04:03 |
34 | A Solution is React | 08:08 |
35 | Component and Application Structure | 05:01 |
36 | Functional vs Class Components | 05:55 |
37 | Making a Simple Component | 02:59 |
38 | What is JSX? | 05:53 |
39 | Fragments | 02:07 |
40 | Expressions in JSX | 05:25 |
41 | Conditionals - Ternary Operator | 03:05 |
42 | Events - Reacting to User Interaction | 08:01 |
43 | React Hooks and useState | 04:42 |
44 | Using the useState React Hook | 05:12 |
45 | Conditionals - if-else | 07:46 |
46 | Dynamically Generating UI from an Array | 07:02 |
47 | Reusing Functionality with Components - Parameters | 07:56 |
48 | Child to Parent Component Communication | 04:53 |
49 | Default Parameters | 02:33 |
50 | Projecting Content | 09:49 |
51 | CSS in React | 10:54 |
52 | Introduction to useEffect | 02:12 |
53 | Using useEffect | 09:12 |
54 | useContext React Hook for Message Broadcasting | 10:40 |
55 | Custom UI for Errors with ErrorBoundary | 12:37 |
56 | Introduction to prop-types | 02:42 |
57 | Summary | 02:15 |
58 | Introduction | 01:17 |
59 | Cleanup | 02:45 |
60 | Individual Movie Component | 07:29 |
61 | Movies List Component | 06:22 |
62 | Making the MoviesList Component More Robust | 09:45 |
63 | Generic List Component | 04:56 |
64 | Installing Bootstrap | 05:01 |
65 | Summary | 00:25 |
66 | Introduction | 01:03 |
67 | Building the Menu Component | 04:51 |
68 | Configuring Routes with React Router | 07:39 |
69 | Route Configuration in an External File | 06:28 |
70 | Building the Pages of our Project | 13:12 |
71 | Using JavaScript for Navigation | 03:24 |
72 | Route Parameters | 03:21 |
73 | Handling Routes that do not exist | 03:17 |
74 | Summary | 00:47 |
75 | Introduction | 01:08 |
76 | Types of Forms | 04:11 |
77 | Building Our First Form | 07:22 |
78 | Validation Rules with Yup | 07:01 |
79 | Custom Validation Rules | 06:34 |
80 | Disabling Button When Submitting the Form | 03:51 |
81 | Creating the Edit Genres Form | 08:31 |
82 | Making the Filtering Movies Form | 10:52 |
83 | Creating the Actors Form | 07:20 |
84 | Working with Dates | 07:22 |
85 | Selecting the Picture of the Actor | 14:19 |
86 | Formatted Text with Markdown | 06:51 |
87 | Creating the Movie Theaters Form | 05:58 |
88 | Integrating Leaflet in React | 12:42 |
89 | Integrating Formik and Leaflet | 07:49 |
90 | Creating the Movies Form Component | 09:48 |
91 | Building a Multiple Selector Component | 09:09 |
92 | Relating Movies with Genres and Movie Theaters | 14:49 |
93 | Creating the Typeahead | 08:47 |
94 | Listing the Selected Actors | 13:38 |
95 | Reordering Elements in a List | 09:29 |
96 | Making a Footer | 01:18 |
97 | Summary | 00:50 |
98 | Introduction | 00:57 |
99 | Installing Postman | 02:01 |
100 | Creating the Repository | 06:11 |
101 | Controllers and Actions | 09:50 |
102 | Routing Rules | 09:07 |
103 | ActionReturn | 04:30 |
104 | Asynchronous Programming | 05:08 |
105 | Model Binding | 07:51 |
106 | Model Validation | 06:27 |
107 | Custom Validation | 08:29 |
108 | Dependency Injection | 06:06 |
109 | Services | 09:52 |
110 | Loggers | 11:21 |
111 | Middleware | 09:39 |
112 | Filters | 08:03 |
113 | Custom and Global Filters | 07:41 |
114 | Summary | 01:17 |
115 | Introduction | 00:43 |
116 | Cleanup | 04:00 |
117 | Axios, Environment Variables and CORS | 09:42 |
118 | Installing and Configuring Entity Framework Core | 07:38 |
119 | Creating Genres - Web API | 04:13 |
120 | Creating Genres - React | 05:48 |
121 | Transform Errors into Arrays | 08:05 |
122 | Display Web API Errors in React | 04:05 |
123 | DTOs | 07:37 |
124 | Index Genres | 04:19 |
125 | Pagination - Web API | 08:07 |
126 | Building the Pagination Component | 10:36 |
127 | Paginating Genres | 10:09 |
128 | Editing Genres | 08:29 |
129 | Deleting Genres | 07:49 |
130 | Edit Entity | 12:07 |
131 | Index Entities | 12:12 |
132 | Actor Entity | 07:11 |
133 | Posting the Actor | 09:10 |
134 | Saving Images into Azure Storage | 11:29 |
135 | Saving Images Locally - Optional | 06:51 |
136 | Actors Index | 04:16 |
137 | Editing Actors | 09:20 |
138 | Movie Theater Entity | 14:07 |
139 | Creating Movie Theaters | 03:16 |
140 | Index Movie Theaters | 03:22 |
141 | Editing and Deleting Movie Theaters | 02:15 |
142 | Movie Entity | 07:35 |
143 | Movie Creation Backend | 14:47 |
144 | Loading the Data on the Movie Creation Page | 08:06 |
145 | Selecting Actors from the Database | 09:53 |
146 | Creating a Movie | 09:08 |
147 | Movie Details - Web API | 09:17 |
148 | Movie Details - React - Part 1 | 13:11 |
149 | Movie Details - React - Part 2 | 09:59 |
150 | Landing Page | 06:21 |
151 | Movie Edit - Backend | 07:46 |
152 | Movie Edit - React | 10:07 |
153 | Deleting a Movie | 08:11 |
154 | Movie Filter - Web API | 05:41 |
155 | Movie Filter - React - Part 1 | 10:19 |
156 | Movie Filter - React - Part 2 | 09:51 |
157 | Summary | 00:52 |
158 | Introduction | 01:01 |
159 | Authentication and Authorization | 03:47 |
160 | Hiding Content If The User is Not Logged In | 04:12 |
161 | Using Claims in our React App | 13:03 |
162 | Protecting Routes From Unauthorized Users | 04:30 |
163 | Configuring Identity | 06:46 |
164 | Building the Register and Login Functionality | 10:27 |
165 | Adding The New Kind of Errors to our ParseBadRequest Filter | 02:01 |
166 | Building the Register Component | 12:16 |
167 | Building the Login Component | 07:42 |
168 | Extracting the Claims from the JWT | 13:07 |
169 | Building the Rating Functionality - Web API | 08:56 |
170 | Getting the Rating of the Movie | 06:06 |
171 | Displaying Icons in the React App | 06:44 |
172 | Building the Ratings Component | 07:05 |
173 | Sending the JWT using Interceptors | 07:20 |
174 | Configuring Claim-Based Authorization | 06:29 |
175 | Assign Admin Claim - Web API | 04:55 |
176 | Assign Admin Claim - React | 10:28 |
177 | Summary | 00:59 |
178 | Introduction | 00:58 |
179 | Deploying the Web API to Azure App Service | 07:26 |
180 | Debugging Errors in Production using Application Insights | 07:18 |
181 | Deploying the React App to Firebase | 06:24 |
182 | Summary | 00:28 |
Similar courses to Building Applications with React 17 and ASP.NET Core 6
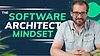
The Software Architect Mindset (COMPLETE)ArjanCodes
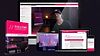
Full Time Game DevThomas Brush
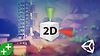
Complete C# Unity Developer 2D: Learn to Code Making Gamesudemygamedev.tv
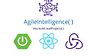
Full Stack HATEOAS: Spring Boot 2.1, ReactJS, Reduxudemy
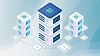
Entity Framework Core - The Complete Guide (.NET Core 5)udemy
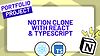
Build a Notion Clone with React and TypeScriptzerotomastery.io
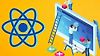
Mastering React With Interview Questions,eStore Project-2024udemy
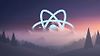
The Modern React 18 Bootcamp - A Complete Developer Guideudemy
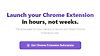
React Chrome Extension boilerplate | ShippedLuca Restagno (shipped.club)
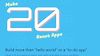