#100DaysOfCode with Python course
#100DaysOfCode in Python is your perfect companion to take the 100 days of code challenge and be successful. This course is 1-part video lesson, 2-parts guided projects. You will be amazed at how many Python technologies and libraries you learn on this journey. Join the course and get started.
Read more about the course
Source code and course GitHub repository
github.com/talkpython/100daysofcode-with-python-course
What's this course about and how is it different?
100 days of code is not just about the commitment. The true power and effectiveness is in having a guide and pursuing the "right-sized" projects. That’s why we have 33 deeply practical projects. Each paired with 20-40 minute lessons at the beginning of the project.
Just a small sampling of the projects you’ll work on include:
- Understating basic Python data structures
- Searching large text corpuses with regular expressions
- Consume HTTP services including the Twitter and GitHub APIs among others
- Visual data with graphs using plotly
- Convert your Python CLI (command line interface) app to a GUI application
- Program against Excel in Python to automate your spreadsheet data
- Build a text-based game and learn object-oriented programming
- Automate multi-step web processes using selenium
- Test your code with pytest and unit testing
- Create a basic web app with Flask
- Create a JSON-based online game service using Flask too
- And 22 more projects!
Who is this course for?
This course is for anyone who wants to immerse themselves in Python for 100 days worth of learning and hands-on projects.
We don’t start from absolute zero in terms of programming but if you are new to Python we have a language appendix and we start somewhat slow. By the end of the course, we get into intermediate-level Python projects.
Watch Online #100DaysOfCode with Python course
# | Title | Duration |
---|---|---|
1 | Welcome to the course | 02:54 |
2 | The rules of #100DaysOfCode | 01:06 |
3 | Topics covered | 03:07 |
4 | Why Python for #100DaysOfCode | 02:06 |
5 | Course flow | 01:34 |
6 | Meet your instructors | 01:46 |
7 | Need a Python primer? | 00:40 |
8 | Get the source code | 02:09 |
9 | Three devs are better than one | 01:43 |
10 | Julian's setup | 02:44 |
11 | Bob's setup | 03:55 |
12 | Michael's tool's and setup | 02:47 |
13 | PyBites code challenge platform | 03:11 |
14 | Video player: A quick feature tour | 02:05 |
15 | Lesson introduction | 00:41 |
16 | Your 3 day overview | 01:33 |
17 | Learning datetime and date | 08:13 |
18 | Datetime timedelta usage | 06:08 |
19 | Concepts: what did we learn | 04:03 |
20 | Lesson introduction | 00:41 |
21 | Namedtuples: more readable code | 02:06 |
22 | Defaultdicts: factory for data structures | 03:01 |
23 | Counter: don't reinvent the wheel | 01:21 |
24 | Deque: when lists become slow | 03:28 |
25 | Concepts: what did we learn | 00:55 |
26 | Second day: use collections on movie data | 04:39 |
27 | Third day: get more practice | 01:03 |
28 | Introduction to Data Structures | 00:26 |
29 | Your 3 day overview | 02:47 |
30 | Manipulating Lists | 07:35 |
31 | Immutability and Tuples | 03:08 |
32 | Creating and Parsing Dictionaries | 07:21 |
33 | Concepts: what did we learn | 03:26 |
34 | Lesson introduction | 00:48 |
35 | The importance of testing | 02:08 |
36 | Setup and a guessing game to test | 03:21 |
37 | Hello test world - unittest vs pytest | 03:18 |
38 | Mocking randomness / pytest-cov | 05:30 |
39 | Mocking user input and exceptions | 05:07 |
40 | Testing a program's stdout with capfd | 04:51 |
41 | Testing (simulating) the game end-to-end | 07:23 |
42 | A TDD primer writing Fizz buzz | 05:06 |
43 | Concepts: what did we learn | 02:28 |
44 | Second day: use pytest on your code | 02:20 |
45 | Third day: writing pytest fixtures | 01:37 |
46 | Introduction to the chapter | 01:33 |
47 | Modeling concepts: Inheritance, classes, and objects | 03:00 |
48 | Demo: Initial project structure | 02:43 |
49 | Demo: Building our classes | 09:02 |
50 | Demo: Implementing the game play | 05:17 |
51 | Concept: Classes | 00:56 |
52 | Your turn: Day 1 | 01:37 |
53 | Your turn: Day 2 | 01:55 |
54 | Your turn: Day 3 | 01:08 |
55 | Lesson introduction | 01:19 |
56 | Writing a simple list comprehension | 03:26 |
57 | Cleaning data with list comprehensions | 03:33 |
58 | Generators - the basics | 01:56 |
59 | Use generators to build a sequence | 01:30 |
60 | List vs generator performance | 01:38 |
61 | Concepts: what did we learn | 01:32 |
62 | Second day: a practical exercise | 00:59 |
63 | Third day: solution and islice | 05:05 |
64 | Third day: more code challenges | 01:18 |
65 | Introduction to the lesson | 00:30 |
66 | Your 3 day overview | 02:52 |
67 | Iteration Refresher | 04:53 |
68 | Itertools - Cycle | 05:34 |
69 | Itertools - Product | 04:46 |
70 | Combinations and Permutations | 03:55 |
71 | Traffic Lights Project | 08:29 |
72 | Concepts: what did we learn? | 04:48 |
73 | Lesson introduction | 00:40 |
74 | Quick primer on decorators | 02:37 |
75 | Function argument types: args and kwargs | 03:54 |
76 | Write a timeit decorator (wraps) | 02:09 |
77 | More abstraction: stacking decorators | 01:56 |
78 | Examples of real life decorators | 01:29 |
79 | Concepts: what did we learn | 02:01 |
80 | Second day: a practical exercise | 00:46 |
81 | Third day: write your own decorator | 01:35 |
82 | Introducing Python's error handling | 01:01 |
83 | Demo: The starter app skeleton | 02:36 |
84 | Demo: try-except blocks | 02:31 |
85 | Demo: Error types | 04:05 |
86 | Concepts: Error handling and exceptions | 01:42 |
87 | Your turn: Day 1 | 01:19 |
88 | Your turn: Day 2 | 01:01 |
89 | Your turn: Day 3 | 00:52 |
90 | Lesson introduction | 00:35 |
91 | When not to use regexes | 01:55 |
92 | Comparing re.search and re.match | 01:50 |
93 | String capturing parenthesis | 02:16 |
94 | findall is your friend | 03:20 |
95 | Compiling regexes with re.VERBOSE | 05:23 |
96 | Using re.sub for string replacing | 02:54 |
97 | Concepts: what did we learn | 01:58 |
98 | Second day: write your own regexes | 02:08 |
99 | Third day: more regex exercises | 01:54 |
100 | Introduction to logging | 03:25 |
101 | Logging with Logbook | 01:18 |
102 | Demo: Introducing our app | 02:13 |
103 | Demo: Configuring logging | 04:32 |
104 | Demo: Writing the log messages | 04:45 |
105 | Demo: Logging a the API level | 02:27 |
106 | Demo: File logging | 02:31 |
107 | Concepts: Logging | 02:30 |
108 | Your turn: Day 1 | 00:38 |
109 | Your turn: Day 2 | 00:31 |
110 | Your turn: Day 3 | 01:21 |
111 | Lesson introduction | 01:21 |
112 | Refactoring 1: if-elif-else horror | 03:01 |
113 | Refactoring 2: loop counting == enumerate | 01:30 |
114 | Refactoring 3: with statement (context managers) | 02:25 |
115 | Refactoring 4: use built-ins / standard library | 03:15 |
116 | Refactoring 5: tuple unpacking and namedtuples | 03:34 |
117 | Refactoring 6: list comprehensions and generators | 02:54 |
118 | Refactoring 7: string formatting and concatenation | 03:12 |
119 | Refactoring 8: PEP8 and Zen of Python | 00:48 |
120 | Refactoring 9: be explicit in your exceptions | 02:56 |
121 | Refactoring 10: quality code best practices | 02:35 |
122 | Refactoring / code quality resources | 02:13 |
123 | Concepts: what did we learn | 05:12 |
124 | Your turn: Day 2 and 3 | 01:32 |
125 | Introduction to CSV programming | 00:36 |
126 | Some amazing data sets | 02:18 |
127 | Our data | 01:03 |
128 | Demo: Getting started with CSV processing | 03:39 |
129 | Demo: Reading the CSV file contents | 04:20 |
130 | Demo: Parsing the CSV file | 02:28 |
131 | Demo: Converting our CSV data to a usable form | 07:00 |
132 | Demo: Answer the questions | 06:36 |
133 | Concepts: CSV programming | 01:44 |
134 | Your turn: Day 1 | 01:44 |
135 | Your turn: Day 2 | 00:16 |
136 | Your turn: Day 3 | 00:30 |
137 | Introduction to JSON | 01:12 |
138 | Your 3 day overview | 01:59 |
139 | Inspecting JSON schema | 02:09 |
140 | Request JSON data from an API | 05:36 |
141 | Parsing nested dicts in JSON | 08:59 |
142 | Concepts: what did we learn | 05:44 |
143 | Introduction to HTTP APIs | 01:12 |
144 | Exploring the service | 01:46 |
145 | Introducing the Postman app | 01:20 |
146 | The requests package | 00:55 |
147 | Demo: Building the program structure | 04:03 |
148 | Demo: Downloading search results | 02:20 |
149 | Demo: Data version one: dicts | 02:31 |
150 | Demo: Data version two: Better results | 05:04 |
151 | Concepts | 01:24 |
152 | Your turn: Day 1 | 00:45 |
153 | Your turn: Day 2 | 01:44 |
154 | Your turn: Day 3 | 00:40 |
155 | Introduction to BeautifulSoup4 | 00:42 |
156 | Your 3 day overview | 02:47 |
157 | Setting up the environment | 01:09 |
158 | A quick BS4 overview | 02:08 |
159 | Building your first BS4 scraper | 09:40 |
160 | Requests best practice | 01:50 |
161 | Detailed BS4 scraping and searching | 10:20 |
162 | Concepts: what did we learn | 05:31 |
163 | Introduction to profiling | 00:50 |
164 | Intuition fail | 01:11 |
165 | Demo: Getting started | 04:14 |
166 | Demo: Focus on our code | 01:52 |
167 | Demo: Fine-tuning collection with the API | 03:23 |
168 | Demo: Even more focused collection | 03:54 |
169 | Demo: Faster with less data processed | 06:20 |
170 | PyCharm's profiling | 03:04 |
171 | Concepts: Profiling | 01:38 |
172 | A quantum warning | 01:59 |
173 | Your turn: Day 1 | 00:46 |
174 | Your turn: Day 2 | 00:59 |
175 | Your turn: Day 3 | 00:58 |
176 | Lesson introduction | 00:35 |
177 | Your 3 day overview | 01:48 |
178 | Setting up our Feedparser environment | 01:17 |
179 | Pulling the feed with Requests | 03:13 |
180 | Parsing XML with Feedparser | 05:24 |
181 | Feedparser Sanity Check | 02:45 |
182 | Concepts: what did we learn | 03:01 |
183 | Introducing uplink | 01:09 |
184 | A glimpse at an API | 02:08 |
185 | Use the official API if available | 01:02 |
186 | Demo: Getting started | 03:09 |
187 | Demo: Exploring the service | 01:41 |
188 | Demo: Creating the client | 04:22 |
189 | Demo: Getting a individual post | 03:21 |
190 | Demo: Only success responses | 02:51 |
191 | Demo: Writing a new post | 04:43 |
192 | Demo: Better wrappers and helpers | 03:21 |
193 | Concepts: uplink | 01:56 |
194 | Your turn: Day 1 | 01:14 |
195 | Your turn: Day 2 | 00:58 |
196 | Your turn: Day 3 | 00:38 |
197 | Lesson introduction | 00:56 |
198 | Create a Twitter app | 00:43 |
199 | Virtual environment and env variables | 02:40 |
200 | Get all tweets with tweepy.Cursor | 02:59 |
201 | Identify the most popular tweets | 02:47 |
202 | Most common hashtags and mentions | 02:13 |
203 | Build a Twitter wordcloud | 03:50 |
204 | Concepts: what did we learn | 02:11 |
205 | Second + third day: practice projects | 03:37 |
206 | Lesson introduction | 00:53 |
207 | Setup and creating a Github user object | 03:30 |
208 | Quick detour: getting help in Python | 02:17 |
209 | Ranking user's repos by popularity | 04:14 |
210 | Creating a gist with the Github API | 04:44 |
211 | Inspecting Github objects with pdb | 03:50 |
212 | Concepts: what did we learn | 02:03 |
213 | Second day: examples / get practice | 02:01 |
214 | Third day: more practice / requests-cache | 01:31 |
215 | Introduction to sending Emails | 00:48 |
216 | Your 3 day overview | 01:45 |
217 | Obtaining your Gmail App ID | 02:57 |
218 | Email Project Setup | 00:56 |
219 | Sending an Email with smtplib | 06:56 |
220 | Getting into MIME | 08:27 |
221 | Emailing with BCC | 03:33 |
222 | Concepts: what did we learn | 04:32 |
223 | Lesson introduction | 00:50 |
224 | Your 3 day overview | 01:45 |
225 | Setup: Install Pyperclip and your env | 00:53 |
226 | Pyperclip Usage | 02:34 |
227 | Demo: Affiliate script | 05:26 |
228 | Demo: Pyperclip text replacer | 06:15 |
229 | Concepts: what did we learn | 03:05 |
230 | Lesson introduction | 00:39 |
231 | Your 3 day overview | 01:59 |
232 | Setup: install openpyxl and your env | 00:58 |
233 | Understanding workbooks and worksheets in openpyxl | 04:05 |
234 | Working with cell values | 06:43 |
235 | Using maxrow | 04:02 |
236 | Inserting data into a worksheet | 08:10 |
237 | Concepts: what did we learn | 06:58 |
238 | Lesson introduction | 01:08 |
239 | Setup: install Selenium and ChromeDriver | 02:04 |
240 | Hello world Selenium: search python.org | 01:12 |
241 | Demo 1: access my Packt ebook collection | 06:49 |
242 | Demo 2: automating PyBites banner creation | 06:59 |
243 | Concepts: what did we learn | 01:55 |
244 | Your turn: Day 2 | 01:16 |
245 | Your turn: Day 3 | 01:07 |
246 | Flask introduction | 00:43 |
247 | Your 3 day overview | 01:57 |
248 | Setting up the environment | 01:55 |
249 | Creating your first Flask app! | 06:31 |
250 | Dict data in Flask | 09:47 |
251 | Concepts: what did we learn | 02:44 |
252 | A brief intro to SQLite3 Databases | 01:04 |
253 | Your 3 day overview | 02:08 |
254 | Installing SQLite DB Browser | 01:13 |
255 | Creating a simple SQLite3 address book | 07:13 |
256 | Analysing the DB with SQLite DB Browser | 00:48 |
257 | Demo: Script to Generate a DB | 04:28 |
258 | Inserting data into the address book | 02:48 |
259 | Demo: Script to populate the address book | 06:22 |
260 | Pulling data with SELECT | 02:26 |
261 | Concepts: what did we learn | 03:44 |
262 | Lesson introduction | 00:59 |
263 | Installing feedparser and plotly | 02:18 |
264 | Prep 1: parse PyBites RSS feed data | 05:54 |
265 | Prep 2: useful data structures for plotting | 04:29 |
266 | Prep 3: transpose data and init Plotly | 02:23 |
267 | Creating bar and pie charts with Plotly | 03:11 |
268 | Other data visualization libraries | 01:54 |
269 | Concepts: what did we learn | 03:30 |
270 | Second day: build your own graphs | 01:57 |
271 | Third day: extra inspiration / keep coding | 01:02 |
272 | Fullstack web introduction | 00:46 |
273 | What is fullstack development? | 02:21 |
274 | What app will we build? | 01:01 |
275 | Introducing Anvil | 00:54 |
276 | Anvil building blocks | 02:42 |
277 | Creating a new project in Anvil | 02:09 |
278 | Adding navigation | 02:28 |
279 | Subforms | 02:07 |
280 | Linking the forms | 04:20 |
281 | Building the Add new document form | 02:09 |
282 | Processing add new document | 04:45 |
283 | Data tables | 02:32 |
284 | Anvil server code | 03:27 |
285 | Creating the document | 02:54 |
286 | Add document finale | 04:52 |
287 | All docs | 06:55 |
288 | A refactoring | 01:32 |
289 | Adding filtering | 03:05 |
290 | Document details form | 02:48 |
291 | Publishing our web app | 01:56 |
292 | Anvil concepts | 02:56 |
293 | Your turn: Day 1 | 01:50 |
294 | Your turn: Day 2 | 01:07 |
295 | Your turn: Day 3 | 01:07 |
296 | Lesson Introduction | 00:48 |
297 | Your 3 day overview | 02:37 |
298 | Writing and working the main menu | 06:07 |
299 | SQLite3 database usage | 04:38 |
300 | Scrub function - SQLite3 workaround | 05:46 |
301 | Home Inventory app run through | 05:02 |
302 | Bug and functionality fixes | 05:40 |
303 | Your Turn! - Fix the app | 01:06 |
304 | Introducing SQLAlchemy | 01:19 |
305 | Demo: Introducing our app | 02:38 |
306 | Demo: The app skeleton | 02:18 |
307 | Demo: Defining database classes | 03:02 |
308 | Demo: Defining columns (via classes) | 04:57 |
309 | Demo: Connecting to the database | 06:24 |
310 | Demo: Using the data access layer (DAL) | 07:34 |
311 | Demo: The final game | 00:56 |
312 | Demo: Seeing the database | 01:25 |
313 | Concepts: SQLAlchemy | 03:18 |
314 | Your turn: Day 1 | 00:56 |
315 | Your turn: Day 2 | 01:46 |
316 | Your turn: Day 3 | 00:40 |
317 | Introduction to Python UIs | 02:51 |
318 | Demo: Where we are starting | 02:48 |
319 | Demo: Refactoring to isolate user input | 02:16 |
320 | Demo: Adding Gooey to our app | 05:26 |
321 | Demo: Packaging our app for redistribution | 04:48 |
322 | Concepts: Gooey | 01:25 |
323 | Your turn: Day 1 | 01:59 |
324 | Your turn: Day 2 | 01:03 |
325 | Your turn: Day 3 | 01:04 |
326 | Introducing our online game server | 03:41 |
327 | What API operations are required? | 02:45 |
328 | Getting started: Program structures | 03:06 |
329 | Adding the Flask basics | 02:05 |
330 | Defining JSON methods in Flask | 02:58 |
331 | Migrating our SQLAlchemy models | 01:48 |
332 | Ensuring starter data | 04:24 |
333 | Defining the API methods in Flask | 04:56 |
334 | Exercising the API | 01:36 |
335 | Implementing the all-rolls method | 01:26 |
336 | Implementing the create-game method | 01:24 |
337 | Implementing the find-user method | 02:58 |
338 | Implementing the create-user method | 04:07 |
339 | Implementing the game-status method | 02:38 |
340 | Implementing the top-scores method | 01:32 |
341 | Implementing the play-round method | 04:15 |
342 | Refactoring our web code for single responsibility | 04:18 |
343 | Implementing the client | 02:36 |
344 | Making the client self-validating | 01:25 |
345 | Writing the full client | 00:46 |
346 | Implementing the game (client-side) | 03:09 |
347 | Your turn: Day 1 | 01:45 |
348 | Your turn: Day 2 | 00:46 |
349 | Your turn: Day 3 | 01:19 |
350 | The final day | 00:46 |
351 | You've done it! | 00:31 |
352 | What you've learned | 01:22 |
353 | Make sure you have the source | 00:29 |
354 | Stay immersed in Python | 01:08 |
355 | Continue to challenge yourself with PyBites | 01:12 |
356 | Thanks and goodbye | 01:12 |
357 | Concept: The shape of a program | 01:26 |
358 | Concept: Variables | 00:51 |
359 | Concept: Truthiness | 01:47 |
360 | Concept: If else | 01:25 |
361 | Concept: Complex conditionals | 01:32 |
362 | Concept: for-in | 01:41 |
363 | Concept: Calling functions | 01:00 |
364 | Concept: Creating functions | 01:34 |
365 | Concept: File I/O | 01:20 |
366 | Concept: Imports and importing modules | 02:00 |
367 | Concept: Python package index | 01:54 |
368 | Concept: pip | 02:26 |
369 | Concept: Virtual environments | 03:54 |
370 | Concept: Slicing | 02:54 |
371 | Concept: Tuples | 01:44 |
372 | Concept: Named tuples | 01:44 |
373 | Concept: Classes | 02:01 |
374 | Concept: objects vs. classes | 01:45 |
375 | Concept: Inheritance | 01:50 |
376 | Concept: Polymorphism | 00:54 |
377 | Concept: Dictionaries | 02:31 |
378 | Concept: Error handling | 02:39 |
379 | Concept: lambdas | 02:09 |
380 | Concept: list comprehensions | 02:58 |
381 | Concept: Want more foundational Python? | 00:47 |
Similar courses to #100DaysOfCode with Python course
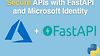
Secure APIs with FastAPI and the Microsoft Identity PlatformTalkpython
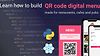
Python/Django + React QR Digital Menu BuilderPythonYoga
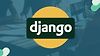
Django Masterclass : Build Web Apps With Python & Djangoudemy
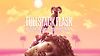
Fullstack Flask: Build a Complete SaaS App with Flaskfullstack.io
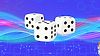
Statistics Bootcamp (with Python): Zero to Masteryzerotomastery.io
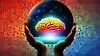
Mastering OpenAI Python APIs: Unleash ChatGPT and GPT4udemy
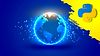
Python for Business Data Analytics & Intelligencezerotomastery.io
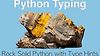
Rock Solid Python with Python Typing CourseTalkpython
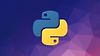
The Complete Python Programming Course for Beginnerscodewithmosh (Mosh Hamedani)
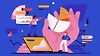