Vue.js Master Class 2024 Edition
11h 55m 11s
English
Paid
Learn by building a real-world application from scratch! This comprehensive course by Vue School teaches you Vue.js, best practices, modern JavaScript, and exciting technologies by developing a forum. Topics include Vue CLI, Vue Router, Vuex, state management, third-party authentication, REST API consumption, SEO, and more. The course is suitable for existing Vue developers, other developers, students, and developer teams. Requirements include familiarity with JavaScript and basic ES6. Taught by experienced instructors like Alex Kyriakidis and Daniel Kelly.
Watch Online Vue.js Master Class 2024 Edition
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Introduction and Project Over-Vue | 03:00 |
2 | Learning Environment Intro | 03:15 |
3 | Prerequisites and Initial Environment | 05:21 |
4 | Scaffolding a Lightning-Fast Vue.js 3 App with Vite | 03:31 |
5 | Powering Up VS Code P1: Vue.js 3 Official Extension | 05:16 |
6 | Powering Up VS Code P2: Eslint for Vue.js 3 | 11:09 |
7 | Powering Up VS Code P3: Prettier for Vue.js 3 | 05:39 |
8 | Git Basics for Every Developer | 07:12 |
9 | Navigating the Project Directories and Boilerplate Cleanup | 07:36 |
10 | Setting Up Vue Router and Navigation with Router Link | 07:31 |
11 | Lazy Load Routes with Vite’s Dynamic Imports in Vue Router | 02:18 |
12 | Create Dynamic Routes with Wildcards in Vue Router | 04:19 |
13 | Catch All Undefined Routes and Create 404 Not Found Page in Vue Router | 05:09 |
14 | Set Up Auto Routes in Vue Router with TypeScript Support | 05:58 |
15 | Refactor Vue.js Codebase for File-Based Routing | 05:40 |
16 | Supabase: The Perfect Backend for Vue.js Frontend | 02:37 |
17 | Integrate Supabase and Connect with Vue.js | 06:06 |
18 | Secure Secret App Data with Vite Environment Variables | 07:31 |
19 | Use Supabase UI to Create Tables and Rows | 05:08 |
20 | Use Supabase SQL Editor to Create Tables and Rows | 05:43 |
21 | Use Supabase CLI in Vue.js and Connect to the Remote Project | 05:09 |
22 | Create Supabase Database Migration Files in Vue.js | 04:43 |
23 | Getting Started with Fakerjs | 04:59 |
24 | Set Up Supabase JavaScript Client in Node Environment | 05:24 |
25 | Seeding the Supabase Remote Database with Fakerjs | 06:05 |
26 | Insert Bulk Entries Into Supabase Database | 02:58 |
27 | Query Supabase from Vue.js Script Setup | 05:21 |
28 | Use Immediately Invoked Function Expression in Script Setup | 03:09 |
29 | Introduction to Vue.js Reactivity System and Using Refs | 05:23 |
30 | Provide TypeScript Type Definitions for Refs in Vue.js Script Setup | 03:48 |
31 | Add TypeScript Support to Supabase in Vue.js | 06:38 |
32 | Create a New Page for Tasks with Database Migration, Seed and Types | 07:54 |
33 | Getting Started with Shadcn UI and Vue.js | 06:38 |
34 | Prepare the Vue App Layout with TailwindCSS | 05:59 |
35 | Utilize Shadcn Input and Dropdown Components | 05:51 |
36 | Use Lucide Icons with Iconify and Vue.js | 03:50 |
37 | Iconify Icon Web Component in Vue.js | 06:19 |
38 | Create a Sidebar and Organize Code with Vue Components | 04:54 |
39 | Extract a Reusable Vue Component for Sidebar Links | 06:58 |
40 | Configure Vue Router Active Links with TailwindCSS | 03:39 |
41 | Create Layout Vue.js Component | 04:50 |
42 | Build a Vue.js Data Table Component with Shadcn and TanStack | 06:24 |
43 | Customize the Data Table Implementation for Our Vue.js App | 06:14 |
44 | Create a Data Table for the Projects Page | 04:04 |
45 | Make the Data Table Cells Clickable with RouterLink | 06:58 |
46 | Exploring Alternative Ways to Integrate Shadcn and TanStack DataTable | 09:17 |
47 | Integrate unplugin-auto-import with Vue and Vite | 07:14 |
48 | Configure unplugin-auto-import for unplugin-vue-router | 05:07 |
49 | Implement Components Auto Importing Feature in Vue.js 3 | 06:40 |
50 | Use Vue.js Suspense Component to Handle Async Dependencies | 06:24 |
51 | Enhance Vue Router with Suspense for Async Components | 06:54 |
52 | Dynamic Page Titles with Pinia | 09:11 |
53 | Retrieve Project Details for Tasks: Querying Nested Database Tables | 04:13 |
54 | Handle Complex Supabase Queries | 06:08 |
55 | Cleaning Time P1: Separating Supabase Queries and Types | 05:14 |
56 | Cleaning Time P2: Separating Column Definitions | 03:14 |
57 | Fetch the Data for the Individual Project Page | 07:15 |
58 | Use the Vue Watch API to Update Pinia Store with the Project Name | 03:51 |
59 | Make the Project Page Template Dynamic | 05:23 |
60 | Exercise: Create Dynamic Indvidual Task Page | 07:05 |
61 | Intro: Why Error Handling Matters for Developers and Users | 05:29 |
62 | Create Global Error Handler in Vue.js with Pinia and Vue Router | 05:34 |
63 | Adjust the Error Page for Custom Errors | 07:44 |
64 | Adjust the Error Page for Supabase Errors | 10:09 |
65 | Adjust the Error Page for Native JavaScript Errors | 03:27 |
66 | Handle Uncaught JavaScript Errors in Vue.js with onErrorCaptured Hook | 04:25 |
67 | Use Props and Vue.js Deep Pseudo-class to Create a Dev Error Component | 05:11 |
68 | Create an Error Page for the Production Server | 05:28 |
69 | Use defineAsyncComponent to Conditionally Render the Appropriate Error Page | 04:19 |
70 | Set Up Vue.js and Supabase for Seamless Auth Integration | 05:33 |
71 | Use v-model to Collect Form Data Values | 04:30 |
72 | Register new Users with Supabase Auth and Vue.js | 05:32 |
73 | Automatically Generate User Profiles on Registration | 03:34 |
74 | Login Users with Supabase Auth and Vue.js | 04:39 |
75 | Quick Cleanup for the Login and Register Pages | 06:08 |
76 | Set Up Auth Store and Integrate It with Utility Functions | 05:43 |
77 | Fetch the User Profile and Update the Auth Store | 06:14 |
78 | Retrieve the Auth Session using Supabase Client | 05:08 |
79 | Create a Vue Router Guard to Validate the Supabase Auth Session | 02:53 |
80 | Manage v-for loops with v-if in Vue.js Using Filters | 08:21 |
81 | Use the Vue.js Template Special Element with v-for Loops | 03:57 |
82 | Emit Custom Events from Child to Parent in Vue.js | 07:23 |
83 | Overcome Challenges of Using Pinia Stores in External Files | 07:09 |
84 | Watch for Supabase Auth changes and Update Auth Store | 05:26 |
85 | Protect Routes with Navigation Guards | 05:52 |
86 | Wait for Pinia Store Updates Before Navigating | 04:26 |
87 | Changes to Default Email Provider in Supabase | 05:42 |
88 | Handle Supabase Server Auth Errors | 05:45 |
89 | Create a Composable for Handling Form Errors | 05:21 |
90 | Implement Realtime Form Validation | 09:51 |
91 | Use watchDebounced from VueUse | 05:07 |
92 | TypeScript Mapping and Generics | 05:31 |
93 | Create Dynamic User Profiles | 07:02 |
94 | Using Pinia for Efficient Data Loading and Caching | 06:26 |
95 | Use useMemoize from VueUse to Optimize Pinia Loader Functions | 03:21 |
96 | Implement Stale While Revalidate with Pinia and useMemoize | 06:07 |
97 | Update Stale Data with Fresh Data | 03:17 |
98 | Set Up Eslint 9 with Flat Config in Vue.js | 08:21 |
99 | Create a Vue.js Composable for Projects Collaborators | 07:21 |
100 | Fetch and Collect Collaborators Across All Projects | 09:27 |
101 | Use Vue.js Render Functions to Render Collaborators | 04:47 |
102 | Load the Collaborators Without Impacting Page Loading Speed | 05:04 |
103 | Reuse the Pinia Loader to Load Single Project | 06:14 |
104 | Make the Pinia Loader Cache Invalidation Logic Reusable | 06:59 |
105 | Fix a Little Bug with the Project Title Watcher | 02:39 |
106 | Create Text Field Component with defineModel | 04:09 |
107 | Emit Custom Events on Input Blur | 03:15 |
108 | Update Project Title in the Database | 06:20 |
109 | Create a Toggle Component for the Project Status | 06:00 |
110 | Update the Project Status in the Database | 05:39 |
111 | Use Vue.js Props Destructure to Assign Default Values for Props | 04:04 |
112 | Reuse the useCollab Composable in the Project Page | 07:10 |
113 | Use defineModel with Textarea and Adjust the Database Schema | 04:08 |
114 | It's Showtime: Apply Your Skills to Tasks! | 02:43 |
115 | Add Tasks and Projects From Anywhere in the App | 07:11 |
116 | How to Install and Use FormKit in Vue.js 3 | 05:23 |
117 | Create a Form for Tasks using FormKit | 05:07 |
118 | Fetch the Select Fields Options from the Database | 06:06 |
119 | Validate and Create Tasks | 05:52 |
120 | Delete Tasks | 08:46 |
121 | Render the Appropriate Layout | 03:26 |
122 | Implement Global State with Composables | 09:48 |
123 | Use Vue.js Provide and Inject with TypeScript | 05:18 |
124 | Combine RouterView and Suspense with the Vue Transition Component | 06:54 |
125 | Add Dark Mode Toggle in Vue With useDark from VueUse | 05:55 |
126 | Use Vue Meta to Set Dynamic Title and Meta Data | 04:08 |
127 | Finished? Not Really—We’re Just Warming Up! | 01:55 |
Similar courses to Vue.js Master Class 2024 Edition
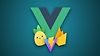
Vue JS 3: Composition API (with Pinia, Firebase 9 & Vite)udemy
Category: Vue
Duration 9 hours 10 minutes 13 seconds
Course
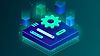
Testing Vue.js Componentsvueschool.io
Category: Vue
Duration 1 hour 6 minutes 20 seconds
Course
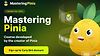
The complete guide to Mastering Pinia (Complete)vueschool.ioEduardo San Martin Morote
Category: Vue
Duration 10 hours 18 minutes 32 seconds
Course
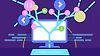
100 Days Of Code: The Complete Web Development Bootcamp 2024Academind Pro
Category: JavaScript, Sql, HTML, CSS, Node.js, Vue
Duration 78 hours 51 minutes 55 seconds
Course