Vue JS 3: Composition API (with Pinia, Firebase 9 & Vite)
If you’re already familiar with Vue 2 & The Options API, then this course will teach you everything you need to know to switch over to (and get started with) Vue 3 & the amazing new Composition API. My name’s Danny, I’m an Indie App Developer & Creator of Fudget, the highest rated personal finance app for iOS, Android, Mac & Windows. And I’ve spent the last 12 months creating Fudget 2 - which is built on Vue 3 & The Composition API.
More
In this course you’ll start by learning the key differences between the Options API & Composition API by creating a simple Options API app & converting it to the Composition API.
You’ll then master all of the basics including:
Reactive data with Refs & Reactive Objects
Methods, Computed Properties & Watchers
Lifecycle Hooks
Directives
Vue Router
Child Components - including the new ways of handling props, emits & modelValue
Dynamic Components
Composables - how to create them from scratch & how to import them from the VueUse library
And you’ll learn State Management using Pinia, the incredible successor to Vuex
After learning the basics, you’re gonna create a real world app called Noteballs from scratch - which has full CRUD capabilities, uses Pinia for State Management and demonstrates real-world use of all the basics you learned earlier.
After this course, you’ll be able to create your own Vue 3 apps based entirely on the Composition API - from scratch.
This course requires a basic understanding of Vue 2 & The Options API, HTML, CSS & JavaScript.
Watch Online Vue JS 3: Composition API (with Pinia, Firebase 9 & Vite)
# | Title | Duration |
---|---|---|
1 | Introduction | 08:03 |
2 | What is the Composition API? | 05:37 |
3 | Editor & Software Setup | 07:45 |
4 | Vue Devtools | 02:08 |
5 | Vue 3 Docs & Install Node.js | 01:06 |
6 | Create a Vue Project | 02:39 |
7 | Project Setup | 03:44 |
8 | Options API - Design | 01:35 |
9 | Options API - Data & Methods | 02:00 |
10 | Convert it to Composition API | 01:28 |
11 | Composition API - Data (refs) & Methods | 03:21 |
12 | Script Setup - An Easier Way! | 02:52 |
13 | Refs | 01:28 |
14 | Two-Way Data Binding | 01:32 |
15 | Reactive Objects | 03:00 |
16 | Non-Reactive Data | 01:21 |
17 | Methods | 03:41 |
18 | Computed Properties | 04:15 |
19 | A Note on Filters | 01:33 |
20 | Watch | 03:42 |
21 | Mounted Hooks | 04:19 |
22 | Activated Hooks | 02:01 |
23 | Updated Hooks | 01:32 |
24 | Multiple Hooks! | 02:13 |
25 | Local Custom Directives | 03:49 |
26 | Global Custom Directives | 02:51 |
27 | $route - Part 1 | 03:50 |
28 | $route - Part 2 | 03:22 |
29 | useRoute | 02:41 |
30 | useRouter | 03:30 |
31 | Lists (v-for) | 02:55 |
32 | Template Refs | 03:05 |
33 | nextTick | 01:52 |
34 | Teleport - Part 1 | 04:24 |
35 | Teleport - Part 2 | 03:22 |
36 | Child Components | 02:52 |
37 | Fix Lazy-Loading Views | 01:36 |
38 | Slots | 03:35 |
39 | Props | 03:52 |
40 | Emits | 03:53 |
41 | modelValue | 02:25 |
42 | update:modelValue | 02:39 |
43 | Dynamic Components - Part 1 | 02:47 |
44 | Dynamic Components - Part 2 | 02:20 |
45 | Provide / Inject - Part 1 | 04:11 |
46 | Provide / Inject - Part 2 | 03:14 |
47 | What is a Composable? | 04:55 |
48 | Create a Composable | 02:40 |
49 | Use Our Composable | 03:27 |
50 | Reuse our Composable | 04:16 |
51 | Add Composable from VueUse | 03:28 |
52 | What is State Management? | 05:36 |
53 | Composable State vs Vuex vs Pinia | 05:03 |
54 | State - Part 1 | 02:42 |
55 | State - Part 2 | 05:42 |
56 | Actions | 03:41 |
57 | Getters | 02:43 |
58 | Use our Store Anywhere | 02:23 |
59 | Introduction & Noteballs App | 02:07 |
60 | Create Project | 02:15 |
61 | Router - Install & Setup | 03:03 |
62 | Router - Add Some Routes | 04:01 |
63 | Add RouterView & Navigation | 01:51 |
64 | Router - Tidying Up | 02:20 |
65 | Install Bulma | 03:10 |
66 | Nav Bar - Design | 02:47 |
67 | Nav Bar - Navigation & Logo | 02:55 |
68 | Nav Bar - Responsive Design & Menu | 05:06 |
69 | Pages (Design) | 02:05 |
70 | Notes (Design) | 01:52 |
71 | Add Note Form (Design) | 02:31 |
72 | Notes Array (Ref) | 02:38 |
73 | Add Note Method | 09:01 |
74 | Child Component - Note | 02:33 |
75 | Props (Note) | 01:35 |
76 | Computed (Note Length) | 05:46 |
77 | Delete Note (Emit) | 06:25 |
78 | Pinia - Setup & State | 04:21 |
79 | Use Our Store | 03:41 |
80 | Action - Add Note | 03:48 |
81 | Action (with Parameters) - Add Note | 02:39 |
82 | Action - Delete Note | 05:16 |
83 | Edit Note Page & Route | 04:39 |
84 | Reusable Component - AddEditNote | 03:58 |
85 | Hook up with modelValue | 05:33 |
86 | Fix the Focus | 05:05 |
87 | Custom Color, Placeholder & Label Props | 10:33 |
88 | Getter - Get Note Content (useRoute) | 05:36 |
89 | Getter (with Parameters) - Get Note Content | 03:10 |
90 | Action - Update Note | 06:25 |
91 | useRouter - Redirect to Notes Page | 01:21 |
92 | More Getters & Stats Page | 07:34 |
93 | Directive - Autofocus | 02:08 |
94 | Global Directive - Autofocus | 02:58 |
95 | Watch the Number of Characters (Watch) | 02:11 |
96 | Composable - useWatchCharacters | 04:25 |
97 | Composable - Multiple Parameters | 02:49 |
98 | Click Outside Composable (VueUse, Template Refs) | 06:11 |
99 | Delete Modal Design (Reactive Objects) | 07:20 |
100 | Hide the Delete Modal (modelValue & update:modelValue) | 05:22 |
101 | Delete Modal - Click Outside to Close | 03:35 |
102 | Delete Modal - Keyboard Control (Lifecycle Hooks) | 06:19 |
103 | Delete Modal - Delete The Note | 04:58 |
104 | Introduction to Firebase | 03:30 |
105 | Create a Firebase Project | 01:47 |
106 | Create App & Install Firebase | 02:04 |
107 | Setup Firestore Database | 02:36 |
108 | Connect to Database | 02:24 |
109 | Display Notes from Firestore | 07:18 |
110 | Get Notes in Real Time | 07:50 |
111 | Add Note | 06:22 |
112 | Delete Note | 02:37 |
113 | Update Note | 05:06 |
114 | Order Notes by Date (ID) | 05:33 |
115 | Improve Document Structure & Auto ID’s | 05:27 |
116 | Display Date on Note | 09:38 |
117 | Add a Progress Bar | 04:55 |
118 | Show Placeholder when No Notes | 02:09 |
119 | Login & Register Page - Tabs | 07:30 |
120 | Login & Register Page - Form | 08:32 |
121 | Firestore Authentication & Auth Store | 03:41 |
122 | Register User | 07:06 |
123 | Logout User | 02:25 |
124 | Login User | 03:26 |
125 | Listen for Auth Changes & Store User Data | 07:51 |
126 | Improve Logout Button | 04:04 |
127 | Redirect User on Auth Change | 05:24 |
128 | Restructure Database for Multiple Users | 05:43 |
129 | Setup Refs for Multiple Users | 07:19 |
130 | Clear Notes array in State when user logs out | 04:13 |
131 | Unsubscribe from the Get Notes Listener | 08:54 |
132 | Navigation Guards - Part 1 | 03:49 |
133 | Navigation Guards - Part 2 | 04:47 |
134 | Firestore Security Rules - Part 1 | 03:31 |
135 | Firebase Security Rules - Part 2 | 05:20 |
136 | Hosting - Part 1 | 06:19 |
137 | Hosting - Part 2 | 04:08 |
138 | Bonus Lecture | 02:23 |
Similar courses to Vue JS 3: Composition API (with Pinia, Firebase 9 & Vite)
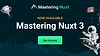
Mastering Nuxt 3masteringnuxt.comvueschool.io
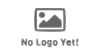
Vue.js + Laravel: CRUD with SPAlaraveldaily.com
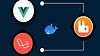
Vue 3 and Laravel: Breaking a Monolith to Microservicesudemy
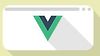
Using Vue 2 to Create Beautiful SEO-Ready Websitescoursetro
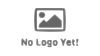
Interactive Vue.js Resume Builderfullstack.io
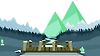
The Nuxt 3 Bootcamp The Complete Developer Guideudemy
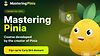