Ultimate Service 3.0
This updated course teaches you how to build production-level services in Go, leveraging the power of Kubernetes.
Read more about the course
From the beginning of the course, you will pair-program with your instructor Bill Kennedy as he walks you through the design philosophies, architectural decisions, and best practices as they apply to engineering a production-ready Go service.
With each new feature that is added to the service, you will learn how to deploy and manage the Kubernetes environment used to run the service. Throughout the class, the code being worked on is pushed to a repository for personal access and review.
This class teaches how to build production-level services in Go with a focus on macro-level engineering decisions. From the beginning you will pair program with the instructor, walking through the design philosophies and guidelines used to engineer the code.
Watch Online Ultimate Service 3.0
# | Title | Duration |
---|---|---|
1 | 1.0 Intro | 00:31 |
2 | 1.1: Design Philosophy, Guidelines, What to Expect | 02:36 |
3 | 1.2: Tooling to Install | 04:54 |
4 | 2.0 Intro | 00:49 |
5 | 2.1: Adding Dependencies | 23:28 |
6 | 2.2: Module Mirrors | 19:23 |
7 | 2.3: Checksum Database | 05:18 |
8 | 2.4: Vendoring | 05:28 |
9 | 2.5: MVS Algorithm | 18:15 |
10 | 3.0 Intro | 00:51 |
11 | 3.1: Tooling Installation | 05:15 |
12 | 3.2: Understanding Clusters, Nodes and Pods | 10:07 |
13 | 3.3: Write Basic Service for Testing | 07:57 |
14 | 3.4.1: Docker Images | 10:34 |
15 | 3.4.2: Kind Configuration | 05:47 |
16 | 3.4.3: Core K8s Configuration | 16:37 |
17 | 3.4.4: K8s Quotas / Patching | 18:33 |
18 | 4.0 Intro | 00:55 |
19 | 4.1: Project Layers, Policies, and Guidelines | 18:14 |
20 | 4.2: Prepare Project | 19:08 |
21 | 4.3: Logging Support | 17:04 |
22 | 4.4: Configuration Support | 21:33 |
23 | 4.5: Debugging / Metrics Support | 22:28 |
24 | 4.6: Shutdown Signaling and Load Shedding | 11:01 |
25 | 5.0 Intro | 00:36 |
26 | 5.1: Basic Structure of an HTTP Router | 11:26 |
27 | 5.2: Add a Readiness, Liveness and Test Handler | 23:59 |
28 | 6.0 Intro | 00:48 |
29 | 6.1: Custom Router | 17:59 |
30 | 6.2: Custom Handler Function | 12:31 |
31 | 6.3: Middleware Support | 09:02 |
32 | 6.4: Sending Responses | 05:11 |
33 | 7.0 Intro | 01:01 |
34 | 7.1: Logging | 13:56 |
35 | 7.2: Request Context | 15:44 |
36 | 7.3.1: Understanding what Error Handling Means | 04:54 |
37 | 7.3.2: Declaring Custom Error Types | 16:48 |
38 | 7.3.3: Consistent Handling and Response | 13:08 |
39 | 7.4: Panic Handling | 09:06 |
40 | 7.5: Metrics | 18:21 |
41 | 8.0 Intro | 00:39 |
42 | 8.1: Understanding JWT | 09:02 |
43 | 8.2: Private/Public Key Generation | 12:16 |
44 | 8.3: Token Generation | 21:21 |
45 | 8.4: Token Signature Validation | 07:26 |
46 | 9.0 Intro | 00:45 |
47 | 9.1: Auth Package | 11:02 |
48 | 9.2: Implementation of an In-Memory Key Store | 08:33 |
49 | 9.3: Middleware | 13:52 |
50 | 9.4: Auth Unit Test | 19:31 |
51 | 10.0 Intro | 00:46 |
52 | 10.1: Kubernetes Support for Postgres | 10:14 |
53 | 10.2: Using Sqlx | 20:58 |
54 | 10.3: Update Readiness Handler to Perform DB Checks | 05:49 |
55 | 11.0 Intro | 00:46 |
56 | 11.1: Maintaining Database Schemas | 16:03 |
57 | 11.2: Seeding Data | 05:18 |
58 | 11.3: Init Containers | 09:53 |
59 | 12.0 Intro | 00:58 |
60 | 12.1: Design Philosophies, Policies, and Guidelines | 11:46 |
61 | 12.2: User Data Models and API Precision | 18:16 |
62 | 12.3: Data Model Validation | 07:49 |
63 | 12.4: User CRUD Data Business Package APIs | 26:34 |
64 | 12.5: User Core Business Package APIs | 07:40 |
65 | 13.0 Intro | 00:45 |
66 | 13.1: Support for Starting and Stopping Containers | 13:56 |
67 | 13.2: Support for Starting and Stopping a Unit Test | 10:36 |
68 | 13.3: Write User CRUD Data Unit Tests | 11:50 |
69 | 14.0 Intro | 00:44 |
70 | 14.1: Writing User Web Handlers | 16:08 |
71 | 14.2: Support for Starting and Stopping an Integration Test | 04:07 |
72 | 14.3: Write Integration Tests for Users | 10:40 |
73 | 15.0 Intro | 00:45 |
74 | 15.1: Integrate OTEL Web Handler into the Framework | 11:43 |
75 | 15.2: Integrate OTEL into Service Startup | 09:08 |
76 | 15.3: Add Zipkin into POD | 10:51 |
77 | 15.4: Add Tracing Calls Inside Functions to Trace | 08:30 |
78 | 16.0 Intro | 00:37 |
79 | 16.1: Check For Dependcy Upgrades | 07:00 |
80 | 16.2: Rebuild and Run the Project | 07:12 |
Similar courses to Ultimate Service 3.0
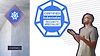
Kubernetes CKS 2023 Complete Course + Simulatorudemy
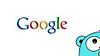
Golang (Google go) udemy
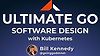
Ultimate Go: Software Design with Kubernetesardanlabs.com
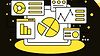
Build a Google Analytics in GoDominic St-Pierre
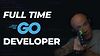
Full Time Go Devfulltimegodev
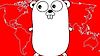
Web Development with Google’s Go (golang) Programming Languagegreatercommons.com
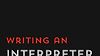
Writing An Interpreter In GoThorsten Ball
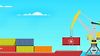
Kubernetes Hands-On - Deploy Microservices to the AWS Cloududemy
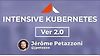
Intensive Kubernetes: 2.0ardanlabs.com
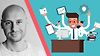