The Vue 3 Bootcamp - The Complete Developer Guide
17h 31m 14s
English
Paid
In this course we will take you from a Vue 3 novice to a job ready engineer. This course is loaded with practical projects and examples so that you can truly understand and utilize Vue 3 and the composition API in great depth.
We will be building five projects, each one getting more and more complex. We will end this course by building an Instagram clone with features like file upload and user authentication. By the end of this course, you should have multiple practical example to show off your knowledge!
Read more about the course
Here are a list of thing you will learn in this course:
- The difference between pure Vanilla JS and Vue3
- How to utilize the composition API - this course is 100% composition API, no options API
- Utilizing all the important Vue directives for things like conditional rendering or rendering of a list
- Fetching data from an external API and handling the success, loading and error states
- Handling user authentication
- Building a Postgres database to handle complex relations
- Utilizing TypeScript for bug free code
- All the important ways to handle state (pinia, composables, inject/eject, ref, reactive)
- Animating and transitioning a UI application
- Storing and retrieving images from a bucket
- Scroll based pagination with the Observer Intersection API
Watch Online The Vue 3 Bootcamp - The Complete Developer Guide
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Introduction | 03:06 |
2 | A Little Bit of Setup | 03:42 |
3 | What is VueJS | 02:41 |
4 | Building the HTML Template | 04:19 |
5 | Styling Our App With CSS | 03:27 |
6 | Making it Functional With JavaScript | 09:12 |
7 | Creating a Vue App | 04:03 |
8 | Rebuilding the App in Vue | 08:08 |
9 | Vue vs Vanilla JS | 04:21 |
10 | Installing Needed Dependencies | 09:05 |
11 | Spinning Up a Vue Development Server | 04:52 |
12 | Some Important Terminologies | 05:07 |
13 | Creating HTML With Vue Templates | 06:11 |
14 | Styling Our App | 05:07 |
15 | Accessing Variables Within Our HTML | 04:16 |
16 | Adding Event Handlers | 05:48 |
17 | Defining Our Variable as State | 06:27 |
18 | Composition API vs Options API | 08:32 |
19 | App Overview | 02:34 |
20 | [OPTIONAL] Building the HTML and CSS | 19:12 |
21 | Conditional Rendering With v-if | 08:21 |
22 | Two Way Binding With v-model | 06:26 |
23 | Event Handling By Creating a New Note | 10:44 |
24 | Iteratively Rendering DOM Elements With v-for | 08:53 |
25 | The Importances of a Key | 04:16 |
26 | Adding Error Handling | 09:33 |
27 | App Overview | 03:26 |
28 | [OPTIONAL] The HTML and CSS | 10:59 |
29 | List Card Rendering | 05:10 |
30 | Watching For State Changes | 06:09 |
31 | Separating Our Code Into Components | 09:49 |
32 | Passing Data to Components via Props | 06:02 |
33 | Introduction to Routing | 04:25 |
34 | Creating a New App | 02:35 |
35 | Creating Two View Components | 03:26 |
36 | Adding Routing to Our App | 10:17 |
37 | RouterLink to Navigate Within the App | 07:52 |
38 | Styling the Active RouterLink | 03:30 |
39 | Pages With Dynamic Paths | 13:05 |
40 | Extracting the Path Params | 05:14 |
41 | The Powers of Nested Routes | 11:24 |
42 | Programmatically Adjust the Route | 05:57 |
43 | Adding 404 Not Found Pages | 09:08 |
44 | Redirect Routes | 02:16 |
45 | Routing Our Quiz App | 12:24 |
46 | Quiz Page HTML and CSS | 11:35 |
47 | Separating Code Into Components | 05:08 |
48 | Passing Props to the Question Component | 08:57 |
49 | Listening to Changes With Watch | 07:16 |
50 | Computing Values With State | 03:35 |
51 | More Computing... | 04:03 |
52 | Passing Data From the Child to the Parent | 09:10 |
53 | Emitting an Event From the Child Component | 09:22 |
54 | Completing the Quiz | 11:44 |
55 | Introduction to Vue Animations | 04:12 |
56 | The Transition Component | 09:31 |
57 | Animating When a Component Leaves | 04:16 |
58 | Conditional Rendering Animations | 04:55 |
59 | Building a Small App | 11:51 |
60 | TransitionGroup to Animate Multiple Elements | 05:17 |
61 | Animating the Other Cards Into Place | 03:48 |
62 | Routing Animations | 08:17 |
63 | Animating Upon Rendering | 06:17 |
64 | Lifecycle Hook of the Transition | 06:09 |
65 | Animation Styling With JavaScript | 08:02 |
66 | App Overview | 01:46 |
67 | The Process of Fetching Data From an API | 05:25 |
68 | Creating the App | 02:25 |
69 | Where to Fetch the Data | 04:44 |
70 | Making the HTTP Requests | 09:34 |
71 | The Suspense Component | 07:35 |
72 | Request Offsets and Limits | 05:59 |
73 | Implementing Paginations | 07:00 |
74 | Building the UI | 18:03 |
75 | A Little Fix | 02:59 |
76 | Component Lifecycle Hooks | 10:47 |
77 | Fetching Data With onMounted | 07:31 |
78 | Slots For Dynamic HTML | 13:34 |
79 | More on Slots | 09:09 |
80 | Adding a Better Loading State | 06:26 |
81 | Creating the Header Component | 10:04 |
82 | Maintaining State With KeepAlive | 05:48 |
83 | Introduction to State Management | 03:18 |
84 | Building a Small Project | 05:50 |
85 | Declaring State With Reactive | 03:44 |
86 | Ref vs Reactive | 06:52 |
87 | A Lot of Nested Components | 06:18 |
88 | Prop Drilling | 07:37 |
89 | Provide/Inject | 09:02 |
90 | Store Reusable Logic With Composables | 07:06 |
91 | Global State With Pinia | 13:39 |
92 | An Introduction to TypeScript | 09:51 |
93 | An Optional TypeScript Lesson | 18:47 |
94 | Building the HTML App | 05:17 |
95 | Types With State | 05:10 |
96 | Types With Functions | 07:56 |
97 | Types With Props | 08:38 |
98 | Types With Computed | 08:10 |
99 | Reusing Our Types Throughout Multiple Components | 03:48 |
100 | Project Overview | 03:05 |
101 | Your Two Options | 01:37 |
102 | Setting Up the Vue App | 07:07 |
103 | Building the NavBar | 14:58 |
104 | Building the Modal | 13:23 |
105 | Building the Timeline | 12:37 |
106 | Adding a Profile View | 07:13 |
107 | Adding the User Bar | 08:59 |
108 | Adding an Image Gallary | 05:17 |
109 | An Introduction to Authentication | 01:58 |
110 | Supabase - A Backend as a Service | 03:38 |
111 | Connecting Our Vue App to Supabase | 04:12 |
112 | Creating a Pinia User Store | 04:39 |
113 | Adding Input Validation | 14:45 |
114 | Client vs Backend Validation | 03:21 |
115 | Signing Up the User | 11:42 |
116 | Validating if the User is Already Registered | 08:10 |
117 | Improving Error Handing | 03:15 |
118 | Adding a Loading State For the Modal | 05:31 |
119 | Handling the Success Case | 07:34 |
120 | Adding the Login Logic | 13:01 |
121 | Persisting the Login State | 11:52 |
122 | A Loading State for Retrieving the User | 05:38 |
123 | Handling Logout | 07:13 |
124 | Navigating to the User's Profile | 03:17 |
125 | Conditionally Rendering the Upload Photo Modal | 09:54 |
126 | Adding the Input Elements | 06:43 |
127 | Creating a Bucket For File Storage | 02:52 |
128 | Programmatically Uploading a Photo | 06:05 |
129 | Updating the Image Policy | 02:50 |
130 | Creating the Posts Table | 05:59 |
131 | Adding a Post to Our DB | 06:12 |
132 | Adding a Loading State | 04:37 |
133 | Handling the Success State | 08:10 |
134 | Fetching Posts Upon Render | 09:37 |
135 | Adding a Loading State | 02:35 |
136 | Building a Following Follower Table | 04:50 |
137 | Conditionally Rendering a Follow Button | 03:06 |
138 | Making the Profile Component Reactive | 01:33 |
139 | Implementing the Follow User Functionality | 05:19 |
140 | Determining Follower Info on Render | 11:00 |
141 | Implementing the Unfollow Functionality | 02:32 |
142 | Toggling the Follow/Following Buttons | 02:29 |
143 | Performing Aggregate Queries | 07:53 |
144 | Check Auth Status in Timeline Page | 06:40 |
145 | Separating HTML into Components | 03:54 |
146 | Fetching the Followers | 05:28 |
147 | Fetching the Followers' Posts | 05:14 |
148 | Rendering the Posts | 02:32 |
149 | Sorting By Most Recent | 01:43 |
150 | Let's Do Some Math | 04:01 |
151 | The Intersection Observer API | 08:41 |
152 | Emitting an Event on Intersect | 03:42 |
153 | Implementing the Pagination | 06:23 |
154 | Stopping Unnecessary HTTP Requests | 04:19 |
155 | Saving Data in Environment Variables | 05:31 |
Similar courses to The Vue 3 Bootcamp - The Complete Developer Guide
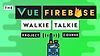
The Vue Firebase Project Coursefireship.io
Category: Vue
Duration 46 minutes 28 seconds
Course
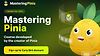
The complete guide to Mastering Pinia (Complete)vueschool.ioEduardo San Martin Morote
Category: Vue
Duration 10 hours 18 minutes 32 seconds
Course
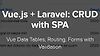
Vue.js + Laravel: CRUD with SPAlaraveldaily.com
Category: Vue, Laravel
Duration 1 hour 50 minutes 29 seconds
Course
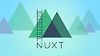
Nuxt.js - Vue.js on Steroidsudemy
Category: Vue, Nuxt
Duration 6 hours 27 minutes 50 seconds
Course
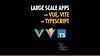
Course: Large Scale Apps with Vue, Vite and TypeScriptDamiano Fusco
Category: TypeScript, Vue, Vite
Duration
Course
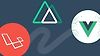
Vue 3, Nuxt.js and Laravel: A Practical Guideudemy
Category: Vue, Nuxt, Laravel
Duration 10 hours 17 minutes 9 seconds
Course
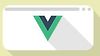
Using Vue 2 to Create Beautiful SEO-Ready Websitescoursetro
Category: Vue, Adobe XD
Duration 1 hour 48 minutes 11 seconds
Course
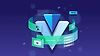
Material UI with Vuetify and Vue.jsvueschool.io
Category: Vue
Duration 1 hour 40 minutes 10 seconds
Course