The Modern Angular Bootcamp [2020]
Angular has been under development by Google for nearly a full decade. It has one purpose: scale up to gracefully handle the largest and most complex web apps around. If you want to build a big app, Angular is the way to go. Every other course online around Angular shows you how to use the basic syntax and features of Angular, but only this course will show you how to build large, professional projects using Angular.
Read more about the course
Mastering Angular by reading the documentation alone is challenging. The official documentation always offers multiple ways to achieve any simple task, but never clarifies what the best way to do something is. In this course, you will gain a fundamental understanding of the best way to achieve any goal with Angular, along with an explanation of the pros and cons of each possible approach.
Top companies are hungry for Angular developers. You've probably heard of the crazy salaries being offered for front-end developers. The average starting salary for an Angular developer is $115k USD. That is not an exaggeration, it is not a joke - this is how badly employers are looking for Angular developers. You can be the latest hire at a new tech startup, a fantastic engineer with amazing skills in Angular! All you have to do to earn this salary is understand Angular, and this course will help you do that.
Learning Angular will make you a better software engineer. Here's the little secret of Angular that nobody tells you about: Angular is really three different topics in one! You've got Angular itself, Typescript, and RxJs. Learning Angular isn't just about Angular, its about Typescript and RxJs as well! Typescript's goal is to help you catch errors quickly during development, rather than when you are running your app, while RxJs has a goal of helping you manage data transparently throughout your application. Understanding Typescript and RxJS will undoubtedly help you become a more knowledgeable, productive, and successful engineer. Note that you do not need any prior experience with Typescript or RxJs to take this course - I will teach you everything you need to know!
Planning on building your own apps? This course will give you a countless number of reusable code snippets that you can utilize on your own personal projects, saving you valuable time. Angular is all about code reusability, and this course will get you started on the right track. Not only will you get incredibly reusable code, but you'll also learn to integrate multiple different CSS frameworks with Angular, so you can get some custom styling that suits the needs of your app.
Learn from someone who has worked with Angular since its 1.0 release. I have worked with Angular since version 1.0, released many years ago. I've seen an incredible number of design patterns and approaches, and I've narrowed down that list to the critical topics that you need to understand. Rather than showing you every last tiny feature of Angular, you'll learn only the best way to achieve any given task.
But don't just take my word for it - check out the reviews for this course! You'll see that other engineers - just like yourself - have had great success and acquired a new understanding of how to build large web apps using Angular.
What You'll Learn
This is a long course, with just about every fact about Angular you could ever hope to know. Here's a brief subset of the topics you'll cover:
Master the fundamentals of Angular, including components, services, pipes, and directives
Create reusable components that you can plug-and-play to create dynamic, complex apps
Understand how to design and architect large apps, with a careful eye on code maintainability
Build modular apps that can easily be updated and changed
Get a handle on performance by leveraging lazy loading, supercharging the speed of your apps
Take a look at Typescript, which will help you write perfect code the first time, rather than encountering errors in the browser
Use RxJs to declaratively manage data throughout your app, leveraging built-in functions to transform your data
Test your code using Angular's excellent testing framework
Connect to backend databases like MySQL, Postgres, or MS SQL Server to add data to your app
Understand how to handle security in your Angular code
...And so much more!
Watch Online The Modern Angular Bootcamp [2020]
# | Title | Duration |
---|---|---|
1 | How to Get Help | 01:06 |
2 | Intro - Our First App | 08:50 |
3 | A Few Questions Answered | 07:24 |
4 | Project Overview | 03:25 |
5 | Starting and Stopping an Angular Project | 02:33 |
6 | Updating a Component Template | 06:08 |
7 | Event Binding Syntax | 07:03 |
8 | Property Binding Syntax | 06:55 |
9 | Interpolation Syntax | 04:16 |
10 | Angular vs JavaScript Design Patterns | 04:14 |
11 | Tracking Input State | 04:39 |
12 | Tracking Additional Properties | 04:48 |
13 | Handling Text Input | 08:23 |
14 | Generating a Random Password | 06:36 |
15 | Review on Property Binding | 04:31 |
16 | Adding Third Party CSS | 03:19 |
17 | CSS Import Statements | 03:13 |
18 | Adding Some Styling | 02:25 |
19 | Structural Directives | 05:42 |
20 | Deploying Angular Apps | 03:54 |
21 | Terminology Review | 02:25 |
22 | App Overview | 02:17 |
23 | Project Setup | 03:38 |
24 | A Quick HTML Dump | 05:29 |
25 | Adding Static Files to Angular | 03:51 |
26 | Scoped CSS Files | 06:18 |
27 | Components in Angular | 07:00 |
28 | Creating Components | 05:23 |
29 | How Angular Creates Components | 07:02 |
30 | More on CSS Scoping | 04:14 |
31 | Tying Data to a Component | 05:02 |
32 | Accepting Data in a Child Component | 09:09 |
33 | Communicating from Parent to Child | 05:14 |
34 | Fixing Template References | 04:28 |
35 | Building Lists with NgFor | 04:31 |
36 | Two Notes on NgFor | 03:48 |
37 | Host Element Selectors | 06:42 |
38 | Deployment and Review | 04:32 |
39 | App Overview | 03:55 |
40 | Initial Steps | 01:36 |
41 | Adding CSS | 04:37 |
42 | Displaying Content | 08:51 |
43 | Randomly Generating Text | 03:33 |
44 | Handling User Input | 02:48 |
45 | Conditional Display | 08:55 |
46 | Character by Character Comparison | 06:21 |
47 | Styling by Comparison | 08:31 |
48 | Pipes Overview | 03:13 |
49 | Adding an Input | 03:44 |
50 | Pipes in Action | 02:27 |
51 | A Touch of Styling | 04:31 |
52 | Adding a Date Picker | 03:17 |
53 | Formatting Dates | 07:16 |
54 | Displaying Currency | 03:26 |
55 | Formatting the Currency | 03:04 |
56 | Formatting Numbers | 05:05 |
57 | Displaying JSON | 02:53 |
58 | Creating Custom Pipes | 06:58 |
59 | Custom Arguments | 05:04 |
60 | Two Neat Things with Pipes | 05:11 |
61 | App Overview | 02:03 |
62 | App Setup | 02:46 |
63 | Getting Some Data | 02:41 |
64 | Review on NgFor | 04:47 |
65 | The NgClass Directive | 03:40 |
66 | More on NgClass | 04:37 |
67 | Conditionally Disabling Buttons | 04:04 |
68 | Changes Pages | 02:57 |
69 | Displaying Images | 01:43 |
70 | Reminder on NgIf | 03:48 |
71 | Multiple Directives with Ng-Container | 08:20 |
72 | NgSwitch | 04:34 |
73 | Generating Custom Directives | 03:10 |
74 | Accessing Elements from a Custom Directive | 03:27 |
75 | Communicating Properties to Directives | 06:42 |
76 | Intercepting a Property Assignment | 06:13 |
77 | Input Aliasing | 02:59 |
78 | Replacing NgClass | 04:07 |
79 | Custom Structural Directives | 08:55 |
80 | Context in Structural Directives | 04:41 |
81 | App Overview | 04:47 |
82 | Tackling Some Challenges | 04:42 |
83 | Modules Overview | 05:04 |
84 | Generating Modules | 05:18 |
85 | Importing and Exporting Modules | 06:41 |
86 | Modules Exercise | 02:10 |
87 | Modules Exercise Solution | 03:29 |
88 | Module Property Definitions | 02:25 |
89 | Adding Basic Routing | 05:27 |
90 | Routing Exercise | 01:37 |
91 | Exercise Solution | 02:11 |
92 | The RouterOutlet Element | 04:04 |
93 | Navigating with RouterLink | 04:18 |
94 | A Touch of Styling | 04:25 |
95 | Styling an Active Link | 01:41 |
96 | Adding Home and NotFound Routes | 05:59 |
97 | Reordering Routing Rules | 04:38 |
98 | Landing and NotFound Components | 01:43 |
99 | Lazy vs Eager Loading | 04:15 |
100 | Implementing Lazy Loading | 09:14 |
101 | Lazy Loading Exercise | 02:54 |
102 | Exercise Solution | 03:44 |
103 | Creating a Placeholder Component | 05:15 |
104 | Customizing Components | 04:57 |
105 | Reminder on Structural Directives | 05:06 |
106 | Widget Modules | 04:39 |
107 | Implementing a Titled Divider | 05:00 |
108 | Grabbing Content with NgContent | 05:03 |
109 | Creating a Segment Component | 03:43 |
110 | NgContent with Selects | 09:37 |
111 | Hiding Empty Elements | 03:35 |
112 | Building a Reusable Table | 04:11 |
113 | Generating and Displaying the Table | 02:48 |
114 | Communicating Down Table Data | 04:31 |
115 | Assembling the Table | 07:28 |
116 | Passing Down Class Names | 04:08 |
117 | Tab Based Navigation | 03:05 |
118 | Adding Child Navigation Routes | 04:21 |
119 | Understanding Child Component Routing | 05:25 |
120 | RouterLink Configuration | 05:46 |
121 | Relative RouterLink References | 09:02 |
122 | Alternate RouterLink Syntax | 03:18 |
123 | Matching Exact Paths | 02:22 |
124 | A Reusable Tabs Component | 03:48 |
125 | Views Module Exercise | 01:55 |
126 | Exercise Solution | 04:37 |
127 | Displaying a List of Statistics | 07:01 |
128 | Displaying a List of Items | 09:03 |
129 | The Mods Module | 02:20 |
130 | Modal Window Setup | 05:16 |
131 | More Basic Modal Setup | 04:14 |
132 | Natural Issues with Modal Windows | 06:30 |
133 | Solving the Modal Issue | 05:51 |
134 | Lifecycle Hooks | 07:55 |
135 | Hiding the Modal with NgOnDestroy | 04:36 |
136 | Opening the Modal | 04:45 |
137 | Closing the Modal | 04:06 |
138 | Stopping Event Bubbling | 02:48 |
139 | Making the Modal Reusable | 11:16 |
140 | Building an Accordion | 02:13 |
141 | Listing Accordion Elements | 07:30 |
142 | Expanding the Active Element | 05:20 |
143 | Intro to TypeScript | 04:22 |
144 | Basic Types | 06:55 |
145 | Type Inference | 05:07 |
146 | Why TypeScript at All? | 04:42 |
147 | TS with Functions | 07:01 |
148 | Difficulties with Objects | 05:03 |
149 | Introducing Interfaces | 08:27 |
150 | Classes and Properties | 07:21 |
151 | Public and Private | 06:50 |
152 | Property Assignment Shortcut | 02:26 |
153 | Enabling Decorator Support | 05:39 |
154 | Decorators | 08:26 |
155 | The Module System | 02:15 |
156 | Strict Mode | 06:04 |
157 | Combining Interfaces and Classes | 06:20 |
158 | Class Generics | 07:23 |
159 | Function Generics | 08:25 |
160 | App Overview | 03:34 |
161 | App Architecture | 06:27 |
162 | Generating Services | 02:50 |
163 | Component Design Methodology | 07:18 |
164 | Handling Form Submission | 04:21 |
165 | Child to Parent Communication | 05:37 |
166 | The Wikipedia API | 06:20 |
167 | Notes on Services | 02:58 |
168 | Accessing Services | 05:00 |
169 | Really Weird Behavior | 13:53 |
170 | Where'd That Come From | 08:31 |
171 | Ok, But Why? | 06:49 |
172 | Why Dependency Injection is Useful | 12:19 |
173 | Making HTTP Requests | 05:46 |
174 | Seeing the Request's Response | 05:06 |
175 | More Parent to Child Communication | 06:52 |
176 | Building the Table | 05:16 |
177 | Escaping HTML Characters | 07:20 |
178 | XSS Attacks | 09:28 |
179 | More on XSS Attacks | 08:20 |
180 | Adding Title Links | 05:23 |
181 | Another CSS Gotcha | 04:10 |
182 | Last Bit of Styling | 04:43 |
183 | Notes on RxJs | 06:18 |
184 | A Quick JS Example | 07:35 |
185 | Adding RxJs Terminology | 06:06 |
186 | Creating an Observable | 07:50 |
187 | Implementing the Processing Pipeline | 09:07 |
188 | More Processing! | 09:30 |
189 | Adding an Observer | 07:10 |
190 | Operator Groups | 04:46 |
191 | Specific Operators | 05:25 |
192 | Low Level Observables | 11:39 |
193 | Alternative Observer Syntax | 03:21 |
194 | Unicast Observables | 04:32 |
195 | More on Unicast Observables | 07:04 |
196 | Multicast Observables | 03:06 |
197 | Multicast in Action | 05:25 |
198 | Hot vs Cold Observables | 04:48 |
199 | RxJs in an Angular World | 03:34 |
200 | Applying TypeScript to RxJs | 08:00 |
201 | Generics with RxJs | 06:54 |
202 | Using TypeScript to Catch Errors | 05:47 |
203 | TypeScript is Smart | 07:40 |
204 | Summary | 02:31 |
205 | App Overview | 02:03 |
206 | App Architecture Design | 02:56 |
207 | API Signup | 05:33 |
208 | HTTP Module Hookup | 03:35 |
209 | HTTP Dependency Injection | 06:22 |
210 | Making the Request | 04:12 |
211 | Displaying the Component | 02:34 |
212 | Making the Request | 04:02 |
213 | Using a Generic Type | 03:14 |
214 | Displaying the Image | 02:51 |
215 | Refetching Data | 04:23 |
216 | App Overview | 04:27 |
217 | Reactive Forms vs Template Forms | 05:51 |
218 | Creating a Form Instance | 07:13 |
219 | Binding a FormGroup to a Form | 05:38 |
220 | Validating Fields | 03:53 |
221 | Finding Validation Errors | 03:55 |
222 | Nasty Error Handling | 07:02 |
223 | Showing and Hiding Validation Messages | 07:42 |
224 | Making a Reusable Input | 06:21 |
225 | Adding Message Cases | 06:22 |
226 | Changing Styling on Validation Errors | 05:39 |
227 | Adding Additional Inputs | 03:40 |
228 | Handling Form Submission | 04:10 |
229 | Additional Validations | 06:59 |
230 | Input Masking | 04:09 |
231 | Hijacking Form Control Values | 08:54 |
232 | Inserting Extra Characters | 05:35 |
233 | Reformatting the Input | 06:58 |
234 | Using a Library for Masking | 09:49 |
235 | Resetting a Form | 06:11 |
236 | A Touch of Styling | 03:59 |
237 | Adding a Credit Card | 06:46 |
238 | App Overview | 01:14 |
239 | Basic Template Form Setup | 05:37 |
240 | Two Way Binding Syntax | 03:24 |
241 | Differences Between Template and Reactive Forms | 09:12 |
242 | Validation Around Template Forms | 03:52 |
243 | Conditional Validation | 04:25 |
244 | Handling Form Submission | 02:17 |
245 | Adding Styling and Wrapup | 04:08 |
246 | App Overview | 03:06 |
247 | App Setup | 02:05 |
248 | Possible Design Approaches | 05:30 |
249 | Displaying Form Values | 06:33 |
250 | Adding Custom Validation | 08:34 |
251 | Extracting Custom Validation Logic | 06:19 |
252 | Making Validators Reusable | 05:22 |
253 | RxJs with Reactive Forms | 03:06 |
254 | Handling Correct Answers | 05:19 |
255 | The Delay Operator | 03:46 |
256 | Adding a Statistic | 04:39 |
257 | RxJs Solution | 06:29 |
258 | A Touch of Styling | 03:09 |
259 | Helping the User Along | 03:05 |
260 | Accessing FormGroups from Custom Directives | 11:02 |
261 | Detecting Changes | 07:17 |
262 | Applying a Class Name | 03:15 |
263 | App Overview | 04:31 |
264 | The API Server | 01:56 |
265 | Contacting the Backend API | 08:47 |
266 | Cookie Based Authentication | 05:27 |
267 | File Generation | 03:59 |
268 | Navigation Reminder | 06:18 |
269 | Adding a Signup Form | 05:26 |
270 | Adding Basic Styling | 03:45 |
271 | Username and Password Validation | 09:06 |
272 | Writing Custom Validators | 09:40 |
273 | Connecting Custom Validators | 06:06 |
274 | Implementing Async Validators | 09:53 |
275 | Nasty Async Validators | 07:42 |
276 | Understanding Async Validators | 11:44 |
277 | Handling Errors from Async Validation | 10:38 |
278 | Building an Auth Service | 07:37 |
279 | Another Reusable Input | 06:10 |
280 | Robust Error Handling | 08:34 |
281 | Customizing Input Type | 05:00 |
282 | Fixing a Few Odds and Ends | 07:12 |
283 | Signup Process Overview | 04:10 |
284 | Making the Signup Request | 09:35 |
285 | Cleaning up the Auth Service | 06:31 |
286 | Handling Signup Errors | 08:00 |
287 | Generic Error Handling | 02:51 |
288 | Adding a Navigation Header | 04:54 |
289 | Maintaining Authentication State | 07:00 |
290 | Oh No, More RxJs | 12:17 |
291 | Using BehaviorSubjects | 08:23 |
292 | The Async Pipe | 04:37 |
293 | Exact Active Links | 01:53 |
294 | Checking Auth Status | 06:47 |
295 | A Gotcha Around the HttpClient | 05:37 |
296 | HTTP Interceptors | 09:00 |
297 | Wiring up an Interceptor | 06:36 |
298 | Modifying Outgoing Requests | 04:09 |
299 | Other Uses of Interceptors | 08:08 |
300 | A Little Record Keeping | 04:52 |
301 | Adding Sign Out | 06:32 |
302 | Automated Signouts | 06:40 |
303 | Programmatic Navigation | 03:20 |
304 | Building the Sign In Flow | 05:51 |
305 | Sign In Authentication | 08:14 |
306 | Showing Authentication Errors | 07:33 |
307 | Inbox Module Design | 08:15 |
308 | Navigation on Authentication | 03:52 |
309 | Restricting Routing with Guards | 09:16 |
310 | Issues With Guards | 10:30 |
311 | A Solution to the Guard with RxJs | 09:52 |
312 | Implementing Our RxJs Solution | 04:59 |
313 | Navigation on Failing a Guard | 03:22 |
314 | Generating Inbox Components | 04:42 |
315 | Retrieving Emails | 06:33 |
316 | Adding Email Fetching to the Service | 05:38 |
317 | Connecting the Service | 04:34 |
318 | Rendering a List of Emails | 04:56 |
319 | Child Route Navigation | 07:49 |
320 | Relative Links with Router Link | 04:06 |
321 | Styling the Selected Email | 04:35 |
322 | Placeholder Markup | 01:02 |
323 | Extracting URL Params | 06:27 |
324 | Accessing Route Information | 07:27 |
325 | Snapshot vs Observable Route Params | 04:22 |
326 | Issues with Nested Subscribes | 07:00 |
327 | Canceling Previous Email Requests | 04:50 |
328 | Handling Undefined Data | 07:27 |
329 | Reusable Data Fetching with Resolvers | 04:27 |
330 | Using a Resolver | 07:13 |
331 | Communicating Data Out of a Resolver | 08:34 |
332 | Error Handling with Resolvers | 08:15 |
333 | Showing Email HTML | 06:36 |
334 | Component Reuse in Email Creation | 03:09 |
335 | A Reusable Modal | 04:53 |
336 | Some More Modal Implementation | 08:53 |
337 | Where to Place the Modal? | 06:09 |
338 | Toggling Modal Visibility | 05:13 |
339 | Providing a Form Default Values | 08:13 |
340 | Displaying Email Inputs | 05:30 |
341 | Adding Form Validation | 05:29 |
342 | Displaying Textarea instead of Text Input | 03:42 |
343 | Capturing the Username | 06:47 |
344 | Submitting a New Email | 07:17 |
345 | Sending Original Email | 05:19 |
346 | Replying to Emails | 05:05 |
347 | Formatting Reply Emails | 09:57 |
348 | Sending Replies | 04:06 |
349 | Quick Bug Fix! | 01:15 |
350 | Type Annotations and Inference | 02:04 |
351 | Annotations and Variables | 04:54 |
352 | Object Literal Annotations | 06:54 |
353 | Annotations Around Functions | 05:56 |
354 | Understanding Inference | 03:52 |
355 | The Any Type | 07:48 |
356 | Fixing the "Any" Type | 01:50 |
357 | Delayed Initialization | 03:06 |
358 | When Inference Doesn't Work | 04:38 |
359 | Annotations Around Functions | 04:57 |
360 | Inference Around Functions | 06:09 |
361 | Annotations for Anonymous Functions | 01:43 |
362 | Void and Never | 02:50 |
363 | Destructuring with Annotations | 03:36 |
364 | Annotations and Objects | 07:06 |
365 | Arrays in TypeScript | 05:06 |
366 | Why Typed Arrays? | 04:31 |
367 | Multiple Typees in Arrays | 02:58 |
368 | When to Use Typed Arrays | 00:55 |
369 | Tuples in TypeScript | 04:05 |
370 | Tuples in Action | 05:29 |
371 | Why Tuples? | 03:21 |
372 | Interfaces | 01:27 |
373 | Long Type Annotations | 04:43 |
374 | Fixing Annotations With Interfaces | 04:37 |
375 | Syntax Around Interfaces | 03:32 |
376 | Functions in Interfaces | 04:47 |
377 | Code Reuse with Interfaces | 04:16 |
378 | General Plan with Interfaces | 03:13 |
379 | Classes | 03:48 |
380 | Basic Inheritance | 03:04 |
381 | Class Method Modifiers | 06:42 |
382 | Fields in Classes | 06:19 |
383 | Fields with Inheritance | 04:19 |
384 | Where to Use Classes | 01:10 |
385 | App Overview | 02:46 |
386 | Parcel in Action | 04:56 |
387 | Project Structure | 03:20 |
388 | Generating Random Data | 05:30 |
389 | Type Definition Files | 05:18 |
390 | Using Type Definition Files | 06:21 |
391 | Export Statements in TypeScript | 05:07 |
392 | Defining a Company | 04:44 |
393 | Adding Google Maps Support | 07:39 |
394 | Google Maps Integration with TypeScript | 04:07 |
395 | Exploring Type Definition Files | 12:47 |
396 | Hiding Functionality | 06:29 |
397 | Why Use Private Modifiers? Here's Why | 08:26 |
398 | Adding Markers | 09:19 |
399 | Duplicate Code | 02:46 |
400 | One Possible Solution | 06:39 |
401 | Restricting Access with Interfaces | 05:36 |
402 | Implicit Type Checks | 03:27 |
403 | Showing Popup Windows | 06:48 |
404 | Updating Interface Definitions | 07:12 |
405 | Optional Implements Clauses | 06:07 |
406 | App Wrapup | 08:09 |
407 | App Overview | 01:35 |
408 | Configuring the TS Compiler | 07:41 |
409 | Concurrently Compilation and Execution | 05:06 |
410 | A Simple Sorting Algorithm | 04:48 |
411 | Sorter Scaffolding | 03:11 |
412 | Sorting Implementation | 05:18 |
413 | Two Huge Issues | 07:38 |
414 | TypeScript is Really Smart | 09:35 |
415 | Type Guards | 09:14 |
416 | Why Is This Bad? | 02:23 |
417 | Extracting Key Logic | 07:30 |
418 | Seperating Swapping and Comparison | 13:59 |
419 | The Big Reveal | 04:39 |
420 | Interface Definition | 04:49 |
421 | Sorting Abritrary Collections | 11:09 |
422 | Linked List Implementation | 24:16 |
423 | Just... One... More... Fix... | 04:04 |
424 | Integrating the Sort Method | 02:45 |
425 | Issues with Inheritance | 06:55 |
426 | Abstract Classes | 06:26 |
427 | Abstract Classes in Action | 04:31 |
428 | Solving All Our Issues with Abstract Classes | 04:00 |
429 | Interfaces vs Abstract Classes | 03:24 |
430 | App Overview | 05:11 |
431 | Modules Overview | 06:38 |
432 | Generating Modules | 04:23 |
433 | Module Properties | 06:46 |
434 | Connecting Modules | 07:00 |
435 | Examining the API | 05:32 |
436 | Reading the Users Location | 05:00 |
437 | The Angular Way | 03:44 |
438 | Geolocation in an Observable | 05:27 |
439 | Connecting the Service to a Component | 03:34 |
440 | Transforming Coordinates to Query Params | 09:03 |
441 | SwitchMap vs MergeMap | 11:27 |
442 | But Why SwitchMap? | 05:34 |
443 | Making a Request | 08:04 |
444 | Further Processing | 04:25 |
445 | Generics on HTTP Requests | 08:57 |
446 | Filter, MergeMap, Pluck Operators | 14:19 |
447 | Map and toArray Operators | 02:49 |
448 | Accessing Data in the Template | 03:58 |
449 | Pipes Overview | 07:09 |
450 | Data Pipes | 04:07 |
451 | The Async Pipe | 06:16 |
452 | Adding Bootstrap | 02:30 |
453 | Styling the Forecast Component | 04:21 |
454 | Reminder on the Share Operator | 04:37 |
455 | How to Structure Services | 10:48 |
456 | Generating the Notification Module | 05:44 |
457 | Notifications Service Design | 04:46 |
458 | Introducing Subjects | 11:04 |
459 | Subject Variations | 08:14 |
460 | More Design on Notifications | 09:08 |
461 | Building a Command Structure | 09:19 |
462 | The Scan Operator | 12:57 |
463 | Scan in the Service | 03:03 |
464 | Fixing a Few Errors | 03:29 |
465 | Replay Subject in Action | 07:19 |
466 | A Preferred Solution | 05:17 |
467 | Displaying Messages | 05:50 |
468 | Automatic Timeout | 03:19 |
469 | Notification Styling | 03:33 |
470 | Clearing Messages | 03:52 |
471 | When to Add Notifications | 04:54 |
472 | Showing Success and Errors | 05:38 |
473 | CatchError and ThrowError | 09:38 |
474 | The Retry Operator | 04:48 |
475 | Service Design | 07:44 |
476 | More on API Pagination | 05:34 |
477 | Service Generation | 04:18 |
478 | Subject Declarations | 04:47 |
479 | Building the HTTP Params | 03:58 |
480 | Applying a Type to the Response | 06:25 |
481 | Calculating Pages Available | 04:44 |
482 | Wrapping the Input Subject | 04:24 |
483 | A Better Interface | 03:00 |
484 | The Article List Component | 03:45 |
485 | Accessing the Data | 04:07 |
486 | Rendering Articles | 03:35 |
487 | Fetching a Page | 01:18 |
488 | Fixing Some Styling | 02:17 |
489 | Creating Custom Pipes | 04:09 |
490 | Custom Pipe Arguments | 04:47 |
491 | The Shared Module Pattern | 02:37 |
492 | Connecting the Paginator | 03:42 |
493 | Paginator Strategy | 03:13 |
494 | Paginator Internals | 07:25 |
495 | Styling the Current Page | 03:10 |
496 | Parent to Child Communication | 03:46 |
Similar courses to The Modern Angular Bootcamp [2020]
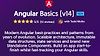
Angular Basics (v15)ultimatecourses.com
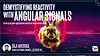
Demystifying Reactivity with Angular Signalsfullstack.io
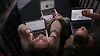
Angular 7 + Spring Boot and Cloud Microservices(Inc. Docker)udemy
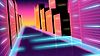
Accelerating Through Angular 2codeschool
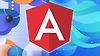
Complete Angular Developer in 2023 Zero to Masteryzerotomastery.io
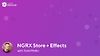
NGRX Store + Effectsultimatecourses.com
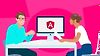
Angular Interview HackingDmytro Mezhenskyi (decodedfrontend.io)
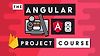
Angular 9 Firebase Project Coursefireship.io
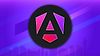