Testing React Apps with React Testing Library
6h 48m 20s
English
Paid
March 20, 2024
Tired of piecing together disconnected tutorials or dealing with rambling, confusing instructors? This course is for you! It's perfectly structured into a series of bite-sized, easy-to-follow videos that cover both theory and practice.
More
- Master the fundamentals of testing React applications with React Testing Library.
- Write maintainable, robust, and trustworthy tests that consistently deliver value.
- Efficiently mock API responses with Mock Service Worker (MSW).
- Mock data using @mswjs/data and @faker-js.
- Simulate user events in a test environment.
- Dive into advanced testing techniques involving authentication, state management, routing, etc.
- Master refactoring techniques that pros use to make their code more readable and maintainable.
- Learn from real-world examples and exercises that prepare you for the job.
- Use ESLint to catch code quality issues early.
Watch Online Testing React Apps with React Testing Library
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Introduction | 01:23 |
2 | Prerequisites | 01:41 |
3 | Course Structure | 01:47 |
4 | How to Take this Course | 01:53 |
5 | Setting Up the Development Environment | 01:01 |
6 | Setting Up the Starter Project | 05:57 |
7 | Setting Up Vitest | 03:49 |
8 | Setting Up React Testing Library | 02:59 |
9 | Introduction | 00:59 |
10 | What to Test | 03:17 |
11 | Testing Rendering | 08:42 |
12 | Simplifying Test Setup | 03:04 |
13 | Exercise- Testing UserAccount | 05:37 |
14 | Testing Lists | 06:06 |
15 | Exercise- Testing ProductImageGallery | 04:22 |
16 | Testing User Interactions | 08:57 |
17 | Exercise- Testing ExpandableText | 11:13 |
18 | Simplifying Tests | 03:02 |
19 | Exercise- Testing SearchBox | 08:36 |
20 | Testing Asynchronous Code | 04:17 |
21 | Exercise- Testing ToastDemo | 03:50 |
22 | Working with Component Libraries | 09:48 |
23 | Exercise- Simplifying Code | 03:51 |
24 | Exercise- Testing OrderStatusSelector | 09:35 |
25 | Is Unit Testing Worth It | 01:48 |
26 | Catching Common Issues with ESLint | 02:25 |
27 | Introduction | 00:39 |
28 | Setting Up Mock Service Worker | 06:54 |
29 | Testing Data Fetching | 06:25 |
30 | Exercise- Testing Data Fetching | 12:29 |
31 | Generating Fake Data | 02:37 |
32 | Mocking Data | 10:05 |
33 | Exercise- Mocking Data | 03:04 |
34 | Testing Errors | 01:58 |
35 | Exercise- Testing Errors | 01:33 |
36 | Testing the Loading State | 05:44 |
37 | Exercise- Testing the Loading State | 00:25 |
38 | Refactoring- Using React Query | 08:36 |
39 | Wrapping Components for Testing | 03:21 |
40 | Exercise- Using React Query | 04:31 |
41 | Exercise- Testing BrowseProductsPage | 02:12 |
42 | Exercise- Testing Loading Skeletons | 10:16 |
43 | Exercise- Testing Error Handling | 05:47 |
44 | Exercise- Testing Data Rendering | 13:28 |
45 | Exercise- Refactoring Tests | 08:37 |
46 | Exercise- Testing Filtering | 07:35 |
47 | Exercise- Refactoring Tests | 10:25 |
48 | Code Coverage | 02:33 |
49 | Exercise- Refactoring with React Query | 08:20 |
50 | Exercise- Extracting CategorySelect | 06:40 |
51 | Exercise- Extracting ProductTable | 03:19 |
52 | Introduction | 00:35 |
53 | What to Test | 01:39 |
54 | Testing Rendering | 08:39 |
55 | Exercise- Testing Initial Data | 03:51 |
56 | Exercise- Refactoring Tests | 06:27 |
57 | Exercise- Testing Focus | 03:17 |
58 | Testing Validation Rules | 03:30 |
59 | Parameterizing Tests | 03:47 |
60 | Exercise- Testing Validation Rules | 03:55 |
61 | Exercise- Extracting a Function for Filling Forms | 10:47 |
62 | Resolving the Act Warning | 03:57 |
63 | Exercise- Testing Form Submission | 07:30 |
64 | Testing Form Feedback | 03:26 |
65 | Introduction | 00:36 |
66 | What to Test | 00:39 |
67 | Exercise- Testing Components that Use Context | 10:50 |
68 | Exercise- Testing Quantity Selector | 17:34 |
69 | Exercise- Extracting Utility Functions | 06:06 |
70 | Exercise- Testing Components that Use Redux | 03:30 |
71 | Exercise- Replacing Redux with React Query | 04:50 |
72 | Introduction | 00:23 |
73 | What to Test | 01:23 |
74 | Mocking the Authentication Status | 05:14 |
75 | Exercise- Testing AuthStatus | 05:06 |
76 | Introduction | 00:19 |
77 | What to Test | 00:28 |
78 | Testing Routes | 07:42 |
79 | Extracting navigateTo | 02:56 |
80 | Exercise- Testing Routes with Parameters | 03:00 |
81 | Exercise- Testing Invalid Routes | 00:51 |
82 | Testing Protected Routes | 03:01 |
83 | Exercise- Testing ProductDetailPage | 04:34 |
84 | Course Wrap Up | 00:26 |
Read Book Testing React Apps with React Testing Library
# | Title |
---|---|
1 | 2- Testing Components |
2 | 3- Mocking APIs |
3 | 4- Testing Forms |
4 | Forms- Additional Exercises |
5 | Routing- Additional Exercises |
Similar courses to Testing React Apps with React Testing Library
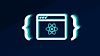
The React practice course, learn by building projects.udemy
Duration 43 hours 45 minutes 48 seconds
Course
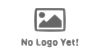
React - The Complete GuideudemyAcademind Pro
Duration 47 hours 42 minutes 41 seconds
Course
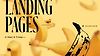
Build fancy landing pages with React and ThreejsPaul Henschel (@0xca0a)
Duration 38 minutes 9 seconds
Course
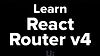
React Router v4ui.dev (ex. Tyler McGinnis)
Duration 6 hours 44 minutes 1 second
Course
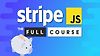
Stripe Payments JavaScript Coursefireship.io
Duration 1 hour 29 minutes 26 seconds
Course
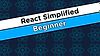
React Simplified - Beginnerwebdevsimplified.com
Duration 10 hours 58 minutes 46 seconds
Course
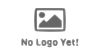
Next.js 14 & React - The Complete GuideudemyAcademind Pro
Duration 36 hours 13 minutes 15 seconds
Course
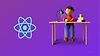
Advanced React For Enterprise: React for senior engineersudemy
Duration 6 hours 4 minutes 5 seconds
Course
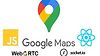
React and WebRTC 2023 & Sharing Location App with Video Chatudemy
Duration 8 hours 11 minutes 54 seconds
Course
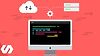
React and Typescript: Build a Portfolio ProjectudemyStephen Grider
Duration 29 hours 21 minutes 48 seconds
Course