React: Intermediate Topics
6h 5m 3s
English
Paid
March 6, 2024
If you know the basics of building web apps with React and TypeScript and are looking for a more advanced course to take your skills to the next level, you're in the right place! This is part 2 of my Ultimate React series where we'll explore state management and routing.
More
You'll learn:
- Fetching & updating data with React Query
- All about reducers, context, and providers
- Global state management with Zustand
- Routing with React Router
Learn the latest techniques and best practices to build complex apps with React.
By the end of this course, you'll be able to…
- Confidently build front-end apps with React and TypeScript
- Apply the latest techniques and best practices
- Troubleshoot errors with ease
- Write clean code like a pro
What You'll Learn...
This course is the second part of a series. The first part covered fundamentals of building web apps with React and TypeScript. In this part, you'll learn how to:
- Fetch and update data using React Query
- Boost your application's performance with caching
- Implement infinite scrolling
- Use reducers to consolidate state management logic
- Use context to share state
- Manage application state using Zustand
- Implement routing with React Router
- Structure your React projects for maintainability
- Write clean code like a pro
- Apply best practices
Who is this course for?
- React developers who want to fill in the gaps in their knowledge.
- Anyone who wants to become a confident and proficient React developer
Watch Online React: Intermediate Topics
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | 1- Introduction | 01:03 |
2 | 2- Prerequisites | 00:55 |
3 | 3- What You'll Learn | 01:36 |
4 | 4- How to Take This Course | 02:00 |
5 | 5- Source Code | 02:22 |
6 | 1- Introduction | 01:15 |
7 | 2- What is React Query | 04:37 |
8 | 3- Setting Up React Query | 01:24 |
9 | 4- Fetching Data | 05:18 |
10 | 5- Handling Errors | 02:27 |
11 | 6- Showing a Loading Indicator | 01:05 |
12 | 7- Creating a Custom Query Hook | 02:33 |
13 | 8- Using React Query DevTools | 03:09 |
14 | 9- Customizing Query Settings | 05:19 |
15 | 10- Exercise- Fetching Data | 03:32 |
16 | 11- Parameterized Queries | 06:19 |
17 | 12- Paginated Queries | 05:38 |
18 | 13- Infinite Queries | 07:08 |
19 | 14- Part Two- Mutating Data | 00:40 |
20 | 15- Mutating Data | 09:41 |
21 | 16- Handling Mutation Errors | 02:15 |
22 | 17- Showing Mutation Progress | 01:28 |
23 | 18- Optimistic Updates | 07:49 |
24 | 19- Creating a Custom Mutation Hook | 07:05 |
25 | 20- Creating a Reusable API Client | 10:01 |
26 | 21- Creating a Reusable HTTP Service | 02:35 |
27 | 22- Understanding the Application Layers | 01:11 |
28 | 23- Part Three- Project Work | 00:32 |
29 | 24- Exercise- Fetching Genres | 10:56 |
30 | 25- Exercise- Fetching Platforms | 03:31 |
31 | 26- Exercise- Fetching Games | 08:47 |
32 | 27- Exercise- Removing Duplicate Interfaces | 02:58 |
33 | 28- Exercise- Creating a Reusable API Client | 05:45 |
34 | 29- Exercise- Implementing Infinite Queries | 07:40 |
35 | 30- Exercise- Implementing Infinite Scroll | 05:39 |
36 | 31- Exercise- Simplifying Query Objects | 10:25 |
37 | 32- Exercise- Creating Lookup Hooks | 07:00 |
38 | 33- Exercise- Simplifying Time Calculations | 03:32 |
39 | 1- Introduction | 01:11 |
40 | 2- Consolidating State Logic with a Reducer | 07:13 |
41 | 3- Creating Complex Actions | 06:47 |
42 | 4- Exercise- Working with Reducers | 04:38 |
43 | 5- Sharing State using React Context | 09:43 |
44 | 6- Exercise- Working with Context | 04:25 |
45 | 7- Debugging with React DevTools | 01:08 |
46 | 8- Creating a Custom Provider | 02:59 |
47 | 9- Creating a Hook to Access Context | 01:25 |
48 | 10- Exercise- Creating a Provider | 02:52 |
49 | 11- Organizing Code for Scalability and Maintainability | 09:38 |
50 | 12- Exercise- Organizing Code | 03:10 |
51 | 13- Splitting Contexts for Efficiency | 02:47 |
52 | 14- When to Use Context | 03:15 |
53 | 15- Context vs Redux | 04:58 |
54 | 16- Managing Application State with Zustand | 07:25 |
55 | 17- Exercise- Working with Zustand | 04:16 |
56 | 18- Preventing Unnecessary Renders with Selectors | 02:43 |
57 | 19- Inspecting Stores with Zustand DevTools | 02:36 |
58 | 20- Part Two- Project Work | 00:26 |
59 | 21- Exercise- Picking the Right State Management Solution | 03:20 |
60 | 22- Exercise- Setting Up a Zustand Store | 06:45 |
61 | 23- Exercise- Removing Props | 12:04 |
62 | 24- Discussion- Building Reusable Components | 01:25 |
63 | 1- Introduction | 00:46 |
64 | 2- Setting Up Routing | 03:10 |
65 | 3- Navigation | 02:44 |
66 | 4- Passing Data with Route Parameters | 02:03 |
67 | 5- Getting Data about the Current Route | 02:58 |
68 | 6- Nested Routes | 03:24 |
69 | 7- Exercise- Working with Nested Routes | 03:10 |
70 | 8- Styling the Active Link | 02:21 |
71 | 9- Handling Errors | 03:23 |
72 | 10- Private Routes | 03:00 |
73 | 11- Layout Routes | 02:09 |
74 | 12- Part Two- Project Work | 00:27 |
75 | 13- Exercise- Setting Up Routing | 06:33 |
76 | 14- Exercise- Handling Errors | 03:23 |
77 | 15- Exercise- Fetching a Game | 11:36 |
78 | 16- Exercise- Refactoring Entities | 02:39 |
79 | 17- Exercise- Building Expandable Text | 05:33 |
80 | 18- Exercise- Building Game Attributes | 09:54 |
81 | 19- Exercise- Building Game Trailer | 08:53 |
82 | 20- Exercise- Building Game Screenshots | 05:51 |
83 | 21- Exercise- Improving the Layout | 01:44 |
84 | 22- Exercise- Fixing the NavBar | 02:33 |
85 | 23- Exercise- Refactoring Entities | 02:30 |
Similar courses to React: Intermediate Topics
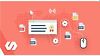
Docker and Kubernetes: The Complete Guide
Duration 21 hours 32 minutes 42 seconds
Course
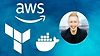
DevOps Deployment Automation with Terraform, AWS and Docker
Duration 10 hours 59 minutes 9 seconds
Course
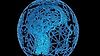
Master Microservices with Java, Spring, Docker, Kubernetes
Duration 23 hours 57 minutes 28 seconds
Course
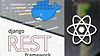
React and Django: A Practical Guide with Docker
Duration 6 hours 50 minutes 20 seconds
Course
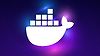
The Ultimate Docker Course
Duration 4 hours 25 minutes 17 seconds
Course
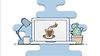
The complete guide to running Java in Docker and Kubernetes
Duration 4 hours 39 minutes 16 seconds
Course
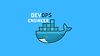
Docker for DevOps Engineers
Duration 4 hours 41 minutes 11 seconds
Course
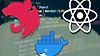
React and NestJS: A Practical Guide with Docker
Duration 6 hours 54 minutes 20 seconds
Course
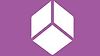
Containers Under the Hood
Duration 11 hours 25 minutes 14 seconds
Course