Microservices Architecture and Implementation on .NET 5
You will learn how to build Microservices on .Net platforms which used Asp.Net Web API, Docker, RabbitMQ, MassTransit, Grpc, Ocelot API Gateway, MongoDB, Redis, PostgreSQL, SqlServer, Dapper, Entity Framework Core, CQRS and Clean Architecture implementation with 24.5 hours fully upgraded videos.
Read more about the course
You will develop e-commerce modules over Product, Basket and Ordering microservices with NoSQL (MongoDB, Redis) and Relational databases (PostgreSQL, Sql Server) with communicating over RabbitMQ Event Driven Communication and using Ocelot API Gateway. You can find Microservices Architecture and Step by Step Implementation on .NET which step by step developing this course with extensive explanations and details.
Along with this you’ll develop following microservices and items:
Catalog microservice which includes;
ASP.NET Core Web API application
REST API principles, CRUD operations
MongoDB database connection and containerization
Repository Pattern Implementation
Swagger Open API implementation
Basket microservice which includes;
ASP.NET Web API application
REST API principles, CRUD operations
Redis database connection and containerization
Consume Discount Grpc Service for inter-service sync communication to calculate product final price
Publish BasketCheckout Queue with using MassTransit and RabbitMQ
Discount microservice which includes;
ASP.NET Grpc Server application
Build a Highly Performant inter-service gRPC Communication with Basket Microservice
Exposing Grpc Services with creating Protobuf messages
Using Dapper for micro-orm implementation to simplify data access and ensure high performance
PostgreSQL database connection and containerization
Microservices Communication
Sync inter-service gRPC Communication
Async Microservices Communication with RabbitMQ Message-Broker Service
Using RabbitMQ Publish/Subscribe Topic Exchange Model
Using MassTransit for abstraction over RabbitMQ Message-Broker system
Publishing BasketCheckout event queue from Basket microservices and Subscribing this event from Ordering microservices
Create RabbitMQ EventBus.Messages library and add references Microservices
Ordering Microservice
Implementing DDD, CQRS, and Clean Architecture with using Best Practices
Developing CQRS with using MediatR, FluentValidation and AutoMapper packages
Consuming RabbitMQ BasketCheckout event queue with using MassTransit-RabbitMQ Configuration
SqlServer database connection and containerization
Using Entity Framework Core ORM and auto migrate to SqlServer when application startup
API Gateway Ocelot Microservice
Implement API Gateways with Ocelot
Sample microservices/containers to reroute through the API Gateways
Run multiple different API Gateway/BFF container types
The Gateway aggregation pattern in Shopping.Aggregator
WebUI ShoppingApp Microservice
ASP.NET Core Web Application with Bootstrap 4 and Razor template
Call Ocelot APIs with HttpClientFactory
ASPNET Core Razor Tools — View Components, partial Views, Tag Helpers, Model Bindings and Validations, Razor Sections etc.
Ancillary Containers
Use Portainer for Container lightweight management UI which allows you to easily manage your different Docker environments
pgAdmin PostgreSQL Tools feature rich Open Source administration and development platform for PostgreSQL
Docker Compose establishment with all microservices on docker;
Containerization of microservices
Containerization of databases
Override Environment variables
On top of all these, you'll learn how to write quality code, not just how to build microservices. In this course you will see the demonstrating a layered application architecture with DDD best practices. Implements NLayer Hexagonal architecture (Core, Application, Infrastructure and Presentation Layers) and Domain Driven Design (Entities, Repositories, Domain/Application Services, DTO's...) and aimed to be a Clean Architecture, with applying SOLID principles in order to use for a project template. Also implements best practices like loosely-coupled, dependency-inverted architecture and using design patterns such as Dependency Injection, logging, validation, exception handling and so on.
Watch Online Microservices Architecture and Implementation on .NET 5
# | Title | Duration |
---|---|---|
1 | Introduction | 06:35 |
2 | Prerequisites and Source Code | 03:10 |
3 | Run Final Application | 13:49 |
4 | What are Microservices ? | 01:39 |
5 | Monolithic Architecture Pros-Cons | 03:02 |
6 | Microservices Architecture Pros-Cons | 04:37 |
7 | Monolithic vs Microservices Architecture Comparison | 02:53 |
8 | What is Containers and Docker ? | 01:16 |
9 | Docker Containers, Images, and Registries | 01:55 |
10 | Introduction | 01:30 |
11 | Create New Github Repository For Our Microservice Project | 01:49 |
12 | Clone Github Repository and Create New Solution with Visual Studio | 04:55 |
13 | Create Asp.Net Web Api Project for Catalog.API Microservice | 05:35 |
14 | MongoDb in Catalog Microservices | 01:57 |
15 | Setup Mongo Docker Database for Catalog.API Microservices | 08:01 |
16 | MongoDb CLI Commands using Interactive Terminal For MongoDb Connection | 06:49 |
17 | Analysis and Architecting of Catalog Microservices | 06:33 |
18 | Repository Design Pattern | 02:56 |
19 | Developing Catalog.API Microservices Creating Entities and MongoDB.Driver Nuget | 07:38 |
20 | Developing Data Layer - Connect Mongo Docker Container from Catalog.API | 16:07 |
21 | Developing Business Layer - Repository Pattern on Catalog.API Microservice | 13:11 |
22 | Developing Presentation Layer - Create CatalogController Class for Catalog.API | 22:30 |
23 | Test and Run Catalog Microservice | 07:23 |
24 | Containerize Catalog Microservices with MongoDB using Docker Compose | 10:27 |
25 | Adding MongoDb image into Docker-Compose File for Multi-Container Docker Environ | 11:27 |
26 | Test on Docker environment - Catalog.API and MongoDb into Docker-Compose File | 07:31 |
27 | Debugging Docker-Compose on Visual Studio for Catalog.API with MongoDb | 16:11 |
28 | Mongo GUI Options for MongoDb Docker Image | 04:42 |
29 | Introduction | 01:32 |
30 | Create Asp.Net Web Api Project for Basket.API Microservice | 05:32 |
31 | Redis in Basket Microservices | 02:07 |
32 | Setup Redis Cache Docker Database for Basket.API Microservices | 05:19 |
33 | Redis CLI Commands using Interactive Terminal For Redis Connection | 03:37 |
34 | Analysis and Architecting of Basket Microservices | 07:21 |
35 | Developing Basket.API Microservices Creating Entities | 06:09 |
36 | Connect Redis Docker Container from Basket.API Microservice w/ AddStackExchange | 07:31 |
37 | Developing Repository Pattern on Basket.API Microservice | 12:57 |
38 | Create BasketController Class for Basket.API Microservice | 12:23 |
39 | Test and Run Basket Microservice | 07:18 |
40 | Containerize Basket Microservices with Redis using Docker Compose | 10:29 |
41 | Adding Redis image into Docker-Compose File for Multi-Container Docker Env | 05:47 |
42 | Test on Docker environment - Basket.API and Redis into Docker-Compose File | 07:19 |
43 | Container management with Portainer | 12:21 |
44 | Introduction | 01:47 |
45 | Create Asp.Net Web Api Project for Discount.API Microservice | 05:25 |
46 | PostgreSQL in Discount Microservices | 01:54 |
47 | Setup PostgreSQL Docker Database for Discount.API Microservices | 05:21 |
48 | Setup pgAdmin Management Portal for PostgreSQL Database for Discount.API Microse | 13:08 |
49 | Create Coupon Table in the DiscountDb of PostgreSQL Database with pgAdmin Manage | 06:37 |
50 | Analysis and Architecting of Discount Microservices | 05:43 |
51 | Developing Discount.API Microservices Creating Entities | 01:43 |
52 | Developing Repository Pattern Connect PostgreSQL use Dapper on Discount.API | 19:04 |
53 | Create DiscountController Class for Discount.API Microservice | 08:16 |
54 | Test and Run Discount Microservice | 09:22 |
55 | Containerize Discount Microservices with PostgreSQL using Docker Compose | 05:51 |
56 | Adding PostgreSQL image into Docker-Compose File for Multi-Container Docker Env | 02:38 |
57 | Migrate PostreSQL Database When Discount Microservices Startup | 22:31 |
58 | Test on Docker environment - Discount.API and PostgreSQL into Docker-Compose | 09:51 |
59 | Introduction | 01:53 |
60 | gRPC usage of Microservices Communication | 02:29 |
61 | Using gRPC in Microservices Communication with .Net - Example Repository | 01:50 |
62 | Create Discount Grpc Microservices Project in Microservices Solution | 13:57 |
63 | Managing PostreSQL Database Operations in Discount Grpc | 09:00 |
64 | Developing discount.proto ProtoBuf file for Exposing Crud Services Discount Grpc | 09:58 |
65 | Generate Proto Service Class from Discount proto File in Discount Grpc | 05:02 |
66 | Developing DiscountService class to Implement Grpc Proto Service Methods | 09:48 |
67 | Implementing AutoMapper into DiscountService Class of Discount Grpc Microservice | 05:21 |
68 | Developing CRUD in DiscountService class to Implement CRUD Grpc Proto Service | 05:36 |
69 | Introduction | 01:46 |
70 | Consuming Discount Grpc Service From Basket Microservice When Adding Cart Item 1 | 08:50 |
71 | Consuming Discount Grpc Service From Basket Microservice When Adding Cart Item 2 | 11:58 |
72 | Register Discount Grpc Client and Discount Grpc Service into the Basket.API | 07:55 |
73 | Test and Run Discount Grpc and Basket Microservice | 09:06 |
74 | Containerize Discount Grpc Microservices with PostgreSQL using Docker Compose | 06:28 |
75 | Adding Grpc DiscountUrl Configuration in Basket.API image configuration on DC | 04:25 |
76 | Test on Docker environment - Basket.API integrate Discount.Grpc into DC | 20:47 |
77 | Introduction | 01:58 |
78 | Analysis and Architecting of Ordering Microservices | 06:36 |
79 | Design Principles - SOLID | 03:09 |
80 | Design Principles - Dependency Inversion Principles (DIP) | 02:41 |
81 | Design Principles - Separation of Concerns (SoC) | 01:53 |
82 | Domain Driven Design - DDD | 04:31 |
83 | Clean Architecture with Domain Driven Design(DDD) | 09:45 |
84 | CQRS (Command Query Responsibility Segregation) Design Pattern | 02:20 |
85 | Eventual Consisteny and Event Sourcing with CQRS Design Pattern | 05:46 |
86 | Code Structure on CQRS and DDD Implementation in Ordering Microservices | 06:08 |
87 | Create Asp.Net Web Api Project for Ordering.API Microservice | 04:22 |
88 | Create Clean Architecture Layers that Ordering Domain - Application and Infra | 04:22 |
89 | Adding Project References Between Clean Architecture Layers | 04:31 |
90 | Developing Ordering.Domain Layer in Clean Architecture | 06:21 |
91 | Developing Ordering.Application Layer with CQRS Pattern Implementation in Clean | 03:41 |
92 | Developing Ordering.Application Layer - Application Contracts | 15:53 |
93 | CQRS Implementation with Mediator Design Pattern | 03:16 |
94 | Developing Ordering.Application Layer- Application Features - GetOrdersListQuery | 19:01 |
95 | Developing Ordering.Application Layer - Application Command Features - Checkout | 25:02 |
96 | Developing Ordering.Application Layer- Application Command Features- UpdateOrder | 14:53 |
97 | Developing Ordering.Application Layer- Application Command Features- DeleteOrder | 13:43 |
98 | Developing Ordering.Application Layer - Application Behaviours | 17:34 |
99 | Developing Ordering.Application Layer - Application Service Registrations | 14:45 |
100 | Developing Ordering.API Presentation Layer in Clean Architecture | 17:25 |
101 | Developing Ordering.Infrastructure Layer in Clean Architecture - Persistence | 12:44 |
102 | Developing Ordering.Infrastructure Layer in Clean Architecture - Repositories | 22:51 |
103 | Developing Ordering.Infrastructure Layer - Infrastructure Service Registrations | 10:49 |
104 | Register Application and Infrastructure Layer Dependencies into Ordering.API | 07:43 |
105 | Adding EF Core Migrations for Code-First Approach in Ordering Microservices | 06:22 |
106 | Applying EF.Core Migrations to Sql Server Automatically When Ordering.API | 18:37 |
107 | Adding SqlServer image into Docker-Compose File for Multi-Container Docker Env | 09:08 |
108 | Test and Run Ordering Microservice | 07:23 |
109 | Test Ordering Microservices CQRS and Clean Architecture Flows | 19:37 |
110 | Containerize Ordering Microservices with SqlServer using Docker Compose | 10:50 |
111 | Test on Docker environment - Ordering.API and SqlServer into Docker-Compose File | 13:02 |
112 | Introduction | 04:00 |
113 | Microservices Communication Types Request-Driven or Event-Driven Architecture | 03:32 |
114 | What is RabbitMQ, Main Components of RabbitMQ | 03:50 |
115 | RabbitMQ Exchange Types | 04:19 |
116 | Adding RabbitMQ image into Docker-Compose File for Multi-Container Docker Env | 09:21 |
117 | Analysis & Design BuildingBlocks EventBus.Messages Class Library Project | 04:21 |
118 | Developing BuildingBlocks EventBus.Messages Class Library | 10:57 |
119 | Produce RabbitMQ Event From Basket Microservice Publisher of BasketCheckoutEvent | 17:42 |
120 | Publish BasketCheckout Queue Message Event in Basket.API Controller Class | 20:59 |
121 | Publish BasketCheckout Queue Message Event in Basket.API Controller Class Part 2 | 07:43 |
122 | Test BasketCheckout Event in Basket.API Microservices | 11:52 |
123 | Consume RabbitMQ Event From Ordering Microservice Subscriber of BasketCheckout | 21:49 |
124 | Subscribe BasketCheckout Queue Message Event in Ordering BasketCheckoutConsumer | 18:10 |
125 | Test BasketCheckout Event in Basket.API and Ordering.API Microservices | 17:18 |
126 | Test MassTransit Retry Mecanism of RabbitMQ Connection Problems | 07:58 |
127 | Containerize Basket and Ordering Microservices w/ RabbitMQ using Docker Compose | 18:17 |
128 | Test on Docker environment - Basket and Ordering with RabbitMQ in Docker-Compose | 13:05 |
129 | Introduction | 01:40 |
130 | Gateway Routing pattern | 01:27 |
131 | API Gateway Pattern | 02:35 |
132 | BFF Backend for Frontend Pattern | 02:33 |
133 | Main features in the API Gateway pattern | 02:02 |
134 | Ocelot API Gateway | 02:33 |
135 | Authentication and authorization in Ocelot API Gateway | 02:14 |
136 | Analysis & Design of API Gateway Microservices | 02:27 |
137 | Developing Ocelot Api Gateway Microservices with Adapting Ocelot Nuget Package | 12:02 |
138 | Adding ocelot.json Configuration File For Routing Microservices in Ocelot Api Gw | 33:01 |
139 | Test Ocelot Api Gateway With Routing Internal Microservices | 15:41 |
140 | Rate Limiting in Ocelot Api Gateway with Configuring Ocelot.json File | 09:55 |
141 | Response Caching in Ocelot Api Gateway with Configuring Ocelot.json File | 06:36 |
142 | Configure Ocelot Json For Docker Development Environment in Ocelot Api Gateway | 10:59 |
143 | Containerize Ocelot Api Gateway Microservices using Docker Compose | 06:03 |
144 | Test on Docker environment - Ocelot API Gateway into Docker-Compose File | 12:52 |
145 | Introduction | 01:52 |
146 | Gateway Aggregation pattern | 03:19 |
147 | Analysis & Design of Shopping.Aggregator Microservices - Gateway Aggregation | 03:23 |
148 | Developing Shopping.Aggregator Microservices | 05:02 |
149 | Developing Dto Model Class for Api Aggreation Operations | 11:14 |
150 | Developing Service Classes for Consuming Internal Microservices in Shopping.Aggr | 21:32 |
151 | Developing Service Classes Consuming Internal Microservices in Shopping.Aggr-2 | 30:03 |
152 | Test Shopping.Aggreation Microservices with Docker Internal Microservices | 15:29 |
153 | Containerize Shopping.Aggregator Microservices using Docker Compose | 07:15 |
154 | Test on Docker environment - Shopping.Aggregator into Docker-Compose File | 06:30 |
155 | Introduction | 02:05 |
156 | Introduction 2 | 01:51 |
157 | Background of Project | 02:21 |
158 | Analysis & Design Shopping Web Application Microservice | 03:08 |
159 | Developing AspnetBasics Shopping Web Application Microservices | 08:29 |
160 | Overview of AspnetBasics Shopping Web Application Microservices | 12:55 |
161 | Refactoring of AspnetBasics Shopping Web Application Microservices | 11:12 |
162 | Register Http Client Factory for Consuming Api Gateway in AspnetBasics Shopping | 06:42 |
163 | Developing Service Implementations for Consuming Api Gateway in AspnetBasics | 10:32 |
164 | Developing Index Page in AspnetBasics Shopping Web Application Microservices | 06:21 |
165 | Developing Product Page in AspnetBasics Shopping Web Application Microservices | 07:04 |
166 | Developing Product Detail Page in AspnetBasics Shopping Web Application | 02:37 |
167 | Developing Cart and Order Page in AspnetBasics Shopping Web Application | 08:37 |
168 | Developing CheckOut Page in AspnetBasics Shopping Web Application Microservices | 07:17 |
169 | Refactoring Developments in AspnetBasics Shopping Web Application Microservices | 04:38 |
170 | Test AspnetBasics Shopping Web Application Microservices Over Ocelot Api Gateway | 16:54 |
171 | Containerize AspnetBasics Shopping Web Application Microservices using DC | 06:40 |
172 | Test on Docker environment - AspnetBasics Shopping Web Microservices into Docker | 09:03 |
173 | Developing Blazor Single Page Application with Custom Api Gateway for CRUD | 02:07 |
174 | Microservices Observability with Distributed Logging, Health Monitoring, Resilie | 02:18 |
175 | Deploying Microservices to Kubernetes, Automating with Azure DevOps into AKS | 02:16 |
176 | Bonus Lecture | 05:42 |
Similar courses to Microservices Architecture and Implementation on .NET 5
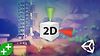
Complete C# Unity Developer 2D: Learn to Code Making Gamesudemygamedev.tv
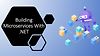
Building Microservices With .NETJulio Casal
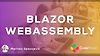
Blazor WebAssemblycode-maze.com
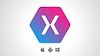
Xamarin Forms: Build Native Mobile Apps with C#codewithmosh (Mosh Hamedani)
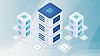
Entity Framework Core - The Complete Guide (.NET Core 5)udemy
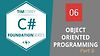
Foundation in C#: Object Oriented Programming Part 2iamtimcorey.com (Tim Corey)
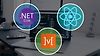