Master the Coding Interview: Big Tech (FAANG) Interviews
Want to land a job at a great tech company like Google, Microsoft, Facebook, Netflix, Amazon, or other companies but you are intimidated by the interview process and the coding questions? Do you find yourself feeling like you get "stuck" every time you get asked a coding question? This course is your answer. Using the strategies, lessons, and exercises in this course, you will learn how to land offers from all sorts of companies. This is the ultimate resource to prepare you for coding interviews. Everything you need in one place!
Read more about the course
The goal of the course isn't to tell you: "Do 100 interview questions and hope you memorize their answers." NO! Our goal is to use the hand selected common interview questions to give you a framework to answer ANY interview question that these companies may throw at you. Instead of trying to memorize common questions, we teach you the principles and fundamentals that you can use to notice certain common patterns in questions so that any question that you get, you have a framework to answer and be confident in your programming interview.
You will also get access to our private online community with thousands of developers online to help you get through the course and the interview!
Here is what you will learn to use in this course while answering the interview questions step by step with us:
----Technical----
1. Big O Notation
2. Data Structures Used:
* Arrays
* Hash Tables
* Singly linked lists
* Doubly linked lists
* Stacks
* Queues
* Binary Trees
* Binary Search Trees
* Tries
* N-ary Trees
* Min/Max Heaps
* Priority Queues
* 2-D Arrays/ Matrices
* Graphs
* Adjacency List
* Adjacency Matrix
* Interface Design
3. Algorithmic Paradigms Used:
* Recursion
* Sorting
* Searching
* Tree Traversals
* Graph Traversals
* Breadth First Search
* Depth First Search
* Divide and Conquer
* Greedy Method
* Dynamic Programming
* Backtracking
4. Specific Algorithms Used:
* Hoare's Quickselect Algorithm
* Floyd's Tortoise and Hare Cycle Detection Algorithm
* Bellman-Ford Algorithm
* Dijkstra's Algorithm
* Topological Sort
Unlike most instructors out there, We are not marketers or salespeople. We are senior engineers and programmers who have worked and managed teams of engineers and have been in these interviews both as an interviewee as well as the interviewer.
Our job as instructors will be successful if we are able to help you get your dream job at a big company. This one skill of mastering the coding interview can really change the course of your career and life and we hope you sign up today to see what it can do for your career!
Watch Online Master the Coding Interview: Big Tech (FAANG) Interviews
# | Title | Duration |
---|---|---|
1 | Master The Coding Interview: Big Tech (FAANG) Interview | 02:35 |
2 | Course Breakdown | 11:05 |
3 | Interview Question #1 Two Sum | 07:15 |
4 | How To Approach Our Problem | 11:46 |
5 | Writing Our Brute Force Solution | 06:41 |
6 | Testing Our Solution With Our Test Cases | 09:24 |
7 | Submitting To Leetcode | 01:50 |
8 | Analyzing Space and Time Complexity | 10:38 |
9 | Optimizing Our Solution | 08:07 |
10 | Coding Our Optimal Solution | 05:22 |
11 | Testing Our Optimal Solution With Our Test Cases | 08:03 |
12 | Checking Performance On LeetCode | 03:25 |
13 | Interview Question #2 - Container With Most Water | 04:47 |
14 | Coming Up With Test Cases | 04:20 |
15 | Thinking Through A Logical Brute Force Solution | 12:50 |
16 | Coding Out Our Brute Force Solution | 07:21 |
17 | Stepping Through Our Code | 04:45 |
18 | Thinking About Our Optimal Solution | 13:53 |
19 | Coding Our Optimal Solution And Testing On LeetCode | 09:51 |
20 | Interview Question #3 - Trapping Rainwater | 07:20 |
21 | Thinking About A Logical Solution | 12:46 |
22 | Coding Our Brute Force Solution | 08:53 |
23 | Figuring Out Our Optimization Strategy | 24:27 |
24 | Coding Our Optimal Solution | 13:27 |
25 | Optimal Code And Testing On LeetCode | 02:10 |
26 | Interview Question #4 -Typed Out Strings | 07:09 |
27 | Logic Of Our Brute Force Solution | 08:03 |
28 | Coding Our Brute Force Solution | 09:38 |
29 | Space And Time Complexity | 07:23 |
30 | Coming Up With Optimal Solution | 11:53 |
31 | Coding Our Optimal Solution | 12:36 |
32 | Submitting To LeetCode | 05:52 |
33 | Interview Question #5 - Longest Substring Without Repeating Characters | 06:40 |
34 | Coming Up With A Brute Force Approach | 06:35 |
35 | Coding Our Brute Force Solution | 09:58 |
36 | Space And Time Complexity | 03:17 |
37 | Sliding Window Technique | 05:19 |
38 | Hints For Optimizing Our Solution | 02:16 |
39 | Thinking About Optimal Solution | 09:10 |
40 | Coding Our Optimal Solution | 08:31 |
41 | Optimal Code And Testing On LeetCode | 03:19 |
42 | Intro To Palindromes | 09:42 |
43 | Interview Question #6 - Valid Palindrome | 08:40 |
44 | Almost A Palindrome | 10:16 |
45 | Figuring Out Our Solution | 06:51 |
46 | Coding Our Solution | 09:47 |
47 | Linked List Introduction | 03:59 |
48 | Basic Algorithm: Reverse a Linked List | 08:22 |
49 | Thinking About Our Solution | 10:56 |
50 | Coding Reverse A Linked List Solution | 07:08 |
51 | Interview Question #7 - M, N Reversals | 06:51 |
52 | Coming Up With A Logical Solution | 16:34 |
53 | Coding Our Solution | 15:39 |
54 | Interview Question #8 - Merge Multi-Level Doubly Linked List | 08:53 |
55 | Figuring Out Our Test Cases | 08:35 |
56 | Thinking About Our Approach | 16:48 |
57 | Coding Out Our Solution | 14:38 |
58 | Interview Question #9 - Cycle Detection | 11:40 |
59 | Floyd's Tortoise And Hare | 03:05 |
60 | Coding Floyd's Algorithm | 08:55 |
61 | Optional: Proof Of How Floyd's Algorithm Works | 25:52 |
62 | Intro to Stacks and Queues | 03:12 |
63 | Interview Question #10 - Valid Parentheses | 09:24 |
64 | Walking Through Our Problem - Identifying The Need For A Stack | 08:16 |
65 | Coding Our Solution With A Stack | 09:19 |
66 | Interview Question #11 - Minimum Brackets To Remove | 07:39 |
67 | Thinking About Our Solution | 12:46 |
68 | Coding Our Solution | 10:54 |
69 | Question #12 - Implement Queue With Stack | 04:11 |
70 | Figuring Out Our Solution | 10:20 |
71 | Coding Our Solution | 08:28 |
72 | Introducing Recursion | 03:08 |
73 | Optional: Tail Recursion | 19:55 |
74 | Sorting | 02:24 |
75 | Interview Question #13 - Kth Largest Element | 06:51 |
76 | Insights From Quick Sort | 12:37 |
77 | Understanding Divide And Conquer | 08:18 |
78 | Coding Quicksort Into Our Solution | 14:54 |
79 | Understanding Hoare's Quickselect Algorithm | 06:56 |
80 | Coding Our Solution With Quickselect | 11:48 |
81 | Understanding Binary Search | 09:33 |
82 | How To Code Binary Search | 06:23 |
83 | Question #14 - Start And End Of Target In A Sorted Array | 05:06 |
84 | Walking Through Our Solution | 10:49 |
85 | Coding Our Solution | 12:56 |
86 | Intro to Binary Trees | 01:53 |
87 | Question #15 - Maximum Depth of Binary Tree | 06:55 |
88 | Learning The Process For Solving Binary Tree Problems | 19:13 |
89 | Coding Our Solution | 06:12 |
90 | Question #16 - Level Order Of Binary Tree | 05:29 |
91 | Walking Through Our Solution | 17:28 |
92 | Coding Out Level Order Traversal | 11:39 |
93 | Question #17 - Right Side View of Tree | 06:44 |
94 | Understanding The Breadth First Search Approach | 08:27 |
95 | Understanding The Depth First Search Approach | 09:23 |
96 | Thinking About Pre-Order, In-Order, and Post-Order Traversals For Our Solution | 12:24 |
97 | Completing Our DFS Solution | 07:42 |
98 | Coding Our Final DFS Solution | 11:56 |
99 | Question #18 - Number Of Nodes In Complete Tree | 08:32 |
100 | Thinking Deeply About A Full Binary Tree | 10:27 |
101 | Figuring Out Number Of Nodes At Last Level | 15:29 |
102 | Coding Out Our Full Solution | 20:40 |
103 | Question #19 - Validate Binary Search Tree | 08:48 |
104 | Thinking About Our Logical Solution | 15:05 |
105 | Figuring Out Our Boundaries | 06:54 |
106 | Coding Our Our Full Solution | 07:59 |
107 | Introducing Heaps | 08:46 |
108 | Insertion In Heaps - Understanding Sift Up | 07:36 |
109 | Deletion In Heaps - Understanding Sift Down | 10:49 |
110 | Starting To Code Our Priority Queue Class | 10:49 |
111 | Coding Our Insertion And Sift Up Methods | 05:50 |
112 | Coding Our Deletion And Sift Down Methods | 13:01 |
113 | Introducing 2D Arrays - What Are They? | 07:24 |
114 | Depth First Search In 2D-Arrays | 07:25 |
115 | Coding DFS - Setting Up For DFS | 09:44 |
116 | Coding DFS - Implementing Recursive DFS | 08:41 |
117 | Breadth First Search In 2D-Arrays | 07:49 |
118 | Coding BFS | 10:06 |
119 | A General Approach To Thinking About Most Graph Questions | 09:10 |
120 | Question #20 Number Of Islands | 07:51 |
121 | Approaching Our Problem - Thinking Deeply About The Values | 16:12 |
122 | Approaching Our Problem - Thinking About Traversals | 08:56 |
123 | Coding Our Solution | 19:09 |
124 | Thinking About Space And Time Complexity | 14:39 |
125 | Question #21 Rotting Oranges | 06:58 |
126 | Figuring Out Our Initial Logic | 11:05 |
127 | Figuring Out The Rest Of Our Solution | 14:28 |
128 | Coding Out Our Solution | 14:48 |
129 | Question #22 - Walls And Gates | 06:20 |
130 | Figuring Out Our Logical Solution | 12:17 |
131 | Coding Out Our Solution | 11:46 |
132 | Introduction To The Types Of Graphs | 11:07 |
133 | Representing Our Graphs - Adjacency List & Adjacency Matrix | 07:20 |
134 | Breadth First Search In Graphs | 04:40 |
135 | Coding BFS | 07:54 |
136 | Depth First Search In Graphs | 03:04 |
137 | Coding DFS | 07:04 |
138 | Question #23 - Time Needed to Inform All Employees | 12:52 |
139 | Verifying Our Constraints And Thinking About Test Cases | 08:13 |
140 | How To Represent Our Graph As An Adjacency List | 09:38 |
141 | Solving Our Problem Logically Using DFS Traversal | 07:49 |
142 | Coding Our DFS Solution | 10:02 |
143 | Question #24 - Course Scheduler | 10:25 |
144 | Thinking About Our Initial Solution - BFS | 07:57 |
145 | Coding Out Our Initial BFS Solution | 15:29 |
146 | What is Topological Sort? | 07:56 |
147 | Thinking About A Solution With Topological Sort | 04:50 |
148 | Coding Our Final Solution | 15:48 |
149 | Question #25 - Network Time Delay | 12:40 |
150 | Thinking About How To Approach The Problem | 08:38 |
151 | Greedy Method & What Is Dijkstra's Algorithm? | 15:31 |
152 | Thinking About A Solution With Dijkstra's Algorithm | 08:56 |
153 | Coding Our Solution With Dijkstra | 17:33 |
154 | Time And Space Complexity Of Our Solution | 13:09 |
155 | Thinking About Negative Weights | 07:42 |
156 | What is The Bellman-Ford Algorithm? - Conceptualizing Dynamic Programming | 16:23 |
157 | What is The Bellman-Ford Algorithm? - The Algorithm Itself | 16:18 |
158 | Coding Our Solution With Bellman-Ford | 09:20 |
159 | Question #26 - Minimum Cost Of Climbing Stairs & How To Approach DP | 09:39 |
160 | Understanding & Identifying Recurrence Relation | 15:49 |
161 | First Step - Recursive Solution From Recurrence Relation | 05:35 |
162 | Second Step - Memoizing Our Redundant Recursive Calls | 07:50 |
163 | Coding Our Memoization Optimization | 07:51 |
164 | Understanding The Bottom Up Approach (Tabulation) | 06:30 |
165 | Third Step - Bottom Up Tabulation | 05:25 |
166 | Fourth Step - Bottom Up Optimization | 06:53 |
167 | Question #27 - Knight Probability In Chessboard | 10:10 |
168 | Thinking About Test Cases To Help Us | 03:52 |
169 | Identifying The Recurrence Relation | 15:30 |
170 | First Step - Recursive Solution From Recurrence Relation | 05:11 |
171 | Second Step - Memoizing Our Redundant Recursive Calls | 14:14 |
172 | Figuring Out The Logic For Our Bottom Up Solution | 14:00 |
173 | Third Step - Bottom Up Tabulation | 08:32 |
174 | Fourth Step - Bottom Up Optimization | 06:56 |
175 | Understanding The Basics Of Backtracking | 07:07 |
176 | Question #28 - Sudoku Solver | 04:20 |
177 | Learning The Backtracking Template | 11:19 |
178 | Applying Our Backtracking Template To Sudoku Solver Logic | 06:43 |
179 | Coding How To Get Box ID | 07:50 |
180 | Setting Up Our Solution Code | 07:06 |
181 | Coding The Recursive Backtracking Portion Of Our Solution | 19:19 |
182 | Thinking About The Space And Time Complexity | 06:59 |
183 | Understanding Interface Design & Question #29 - Monarchy | 10:53 |
184 | Figuring Out Our Test Cases | 05:58 |
185 | Thinking About The Logical Of The Monarchy | 09:42 |
186 | Coding Our Monarchy Solution | 14:58 |
187 | Introducing Tries | 01:08 |
188 | Question #30 - Implement Prefix Trie | 09:50 |
189 | Understanding The Logic For Our Methods | 03:55 |
190 | Implementing Our Prefix Trie Data Structure Solution | 15:16 |
191 | Section Overview | 02:25 |
192 | What Is Good Code? | 06:58 |
193 | Big O and Scalability | 11:09 |
194 | O(n) | 05:40 |
195 | O(1) | 06:11 |
196 | Solution: Big O Calculation | 05:55 |
197 | Solution: Big O Calculation 2 | 02:30 |
198 | Simplifying Big O | 01:51 |
199 | Big O Rule 1 | 04:29 |
200 | Big O Rule 2 | 06:37 |
201 | Big O Rule 3 | 03:14 |
202 | O(n^2) | 07:14 |
203 | Big O Rule 4 | 06:48 |
204 | Big O Cheat Sheet | 03:19 |
205 | What Does This All Mean? | 05:33 |
206 | O(n!) | 01:19 |
207 | 3 Pillars Of Programming | 03:33 |
208 | Space Complexity | 02:23 |
209 | Exercise: Space Complexity | 06:25 |
210 | Exercise: Twitter | 07:14 |
211 | Section Summary | 04:44 |
212 | Arrays Introduction | 13:52 |
213 | Static vs Dynamic Arrays | 06:41 |
214 | Optional: Implementing An Array | 17:20 |
215 | Hash Tables Introduction | 04:11 |
216 | Hash Function | 05:57 |
217 | Hash Collisions | 09:44 |
218 | Hash Tables VS Arrays | 02:02 |
219 | Linked Lists Introduction | 02:27 |
220 | What Is A Linked List? | 04:37 |
221 | Exercise: Why Linked Lists? | 02:06 |
222 | Solution: Why Linked Lists? | 05:36 |
223 | What Is A Pointer? | 05:46 |
224 | Doubly Linked Lists | 03:19 |
225 | Singly VS Doubly Linked Lists | 02:41 |
226 | Linked Lists Review | 05:08 |
227 | Stacks + Queues Introduction | 02:59 |
228 | Stacks | 03:29 |
229 | Queues | 03:31 |
230 | Exercise: Stacks VS Queues | 03:07 |
231 | Solution: Stacks VS Queues | 03:40 |
232 | Stacks + Queues Review | 02:20 |
233 | Trees Introduction | 06:24 |
234 | Binary Trees | 05:46 |
235 | O(log n) | 07:01 |
236 | Binary Search Tree | 06:13 |
237 | Balanced VS Unbalanced BST | 03:43 |
238 | BST Pros and Cons | 02:27 |
239 | Trie | 03:17 |
240 | Graph + Tree Traversals | 03:57 |
241 | BFS Introduction | 02:46 |
242 | DFS Introduction | 03:24 |
243 | BFS vs DFS | 03:21 |
244 | Exercise: BFS vs DFS | 00:45 |
245 | Solution: BFS vs DFS | 03:17 |
246 | breadthFirstSearch() | 09:16 |
247 | PreOrder, InOrder, PostOrder | 05:22 |
248 | depthFirstSearch() | 12:04 |
249 | Recursion Introduction | 05:37 |
250 | Stack Overflow | 06:18 |
251 | Anatomy Of Recursion | 10:28 |
252 | Recursive VS Iterative | 04:17 |
253 | When To Use Recursion | 04:01 |
254 | Recursion Review | 02:48 |
255 | Sorting Introduction | 07:02 |
256 | The Issue With sort() | 06:52 |
257 | Sorting Algorithms | 03:39 |
258 | Bubble Sort | 03:46 |
259 | Solution: Bubble Sort | 05:07 |
260 | Selection Sort | 02:40 |
261 | Solution: Selection Sort | 02:24 |
262 | Dancing Algorithms | 01:37 |
263 | Insertion Sort | 02:39 |
264 | Solution: Insertion Sort | 02:07 |
265 | Merge Sort and O(n log n) | 09:00 |
266 | Solution: Merge Sort | 04:45 |
267 | Quick Sort | 07:41 |
Similar courses to Master the Coding Interview: Big Tech (FAANG) Interviews
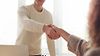
Pass your job interview in English : Get your dream job!udemy
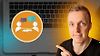
TypeScript Interview Questions - Coding Interview 2023udemy
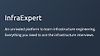
InfraExpertalgoexpert
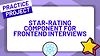
Crack the Frontend Interview with Reactzerotomastery.io
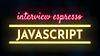
JavaScript Interview Espressointerviewespresso (Aaron Jack)
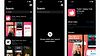
Ultimate SwiftUI Mock Interview AppStoreletsbuildthatapp
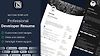
Professional Developer ResumeGeorge Moller
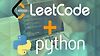
Python & LeetCode | The Ultimate Interview BootCampkaeducation.com
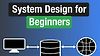
System Design for Beginnersneetcode.io
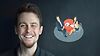