Understanding React | Don’t Imitate Understand
Even if you've been using React for years, your mental model is likely inaccurate. That's because the best mental model is understanding how something really works.
React is wildly popular and well-established in the job market for web developers. However, to use and debug it well, you must understand how it actually works.
In this course, designed for both React beginners and experienced React devs you will come to deeply understand how React works under-the-hood by diving into React internals: the React source code itself.
Read more about the course
Most courses teach you how to use React. In this course you will fully understand how React works, which will enable you to use and debug React well.
You will gain truly under-the-hood knowledge on topics such as the React Element Tree, the Fiber Tree, JSX, Rendering, Reconciliation, State, Hooks, Effects, Suspense, React Server Components, and more.
That knowledge will give you a clarity that will serve you well every day you use React, or React-based frameworks like Next.js or Remix.
Watch Online Understanding React | Don’t Imitate Understand
# | Title | Duration |
---|---|---|
1 | Introduction | 01:49 |
2 | Setup | 01:54 |
3 | A Basic React App | 02:08 |
4 | The React Source Code | 02:07 |
5 | The DOM and Declarative Programming | 00:21 |
6 | HTML and Trees | 02:01 |
7 | HTML, The Browser, and the DOM | 04:03 |
8 | DOM Manipulation | 06:33 |
9 | Conceptual Aside: Imperative vs. Declarative Programming | 03:01 |
10 | The DOM and Imperative Programming | 03:13 |
11 | The DOM and Declarative Programming | 04:09 |
12 | React Elements | 00:27 |
13 | Conceptual Aside: Recursion | 04:18 |
14 | Conceptual Aside: POJOs | 12:53 |
15 | Creating React Elements | 11:37 |
16 | React Element Trees | 05:26 |
17 | Conceptual Aside: DOM Element References | 01:32 |
18 | React Elements and DOM Elements | 06:04 |
19 | React DOM Updates | 12:12 |
20 | Components | 06:02 |
21 | Components and Reusability | 11:08 |
22 | Conceptual Aside: Pure Functions | 05:19 |
23 | Props | 12:25 |
24 | Template Logic | 14:12 |
25 | Element Properties | 07:19 |
26 | JSX | 00:22 |
27 | Conceptual Aside: Markup and Tree Creation Shorthand | 01:57 |
28 | Conceptual Aside: Transformation | 01:49 |
29 | React Elements and JSX | 19:41 |
30 | JSX and Thinking In Elements | 12:01 |
31 | Conceptual Aside: HTML Authoring | 02:48 |
32 | Fragment and HTML Authoring | 10:04 |
33 | Fiber and Reconciliation | 00:42 |
34 | Root Creation and render | 08:58 |
35 | Conceptual Aside: Trees and Linked Lists | 04:23 |
36 | Fiber, Fiber Nodes, and Fiber Trees | 06:58 |
37 | Conceptual Aside: Tree Reconciliation and the Tree Edit Distance Problem | 03:45 |
38 | Work-In-Progress Nodes | 09:29 |
39 | Reconciliation and Work | 05:49 |
40 | Execution Contexts | 00:22 |
41 | Conceptual Aside: Execution Contexts and the Event Loop | 03:43 |
42 | Fiber and Custom Execution Contexts | 04:15 |
43 | Units of Work and The Work Loop | 03:01 |
44 | Conceptual Aside: Equality | 03:35 |
45 | Beginning, Completing, Bailing Out and Pausing Work | 07:02 |
46 | Lanes and Priority | 02:36 |
47 | React DOM and Rendering | 00:15 |
48 | Committing Work and The Renderer | 02:56 |
49 | Mounting, Updating, and Unmounting | 04:49 |
50 | Events | 00:24 |
51 | DOM Events | 11:37 |
52 | React Event Objects | 07:53 |
53 | Synthetic Event Properties and Methods | 05:44 |
54 | State | 00:17 |
55 | Conceptual Aside: State Machines | 06:37 |
56 | Conceptual Aside: Pure Functions (again) | 02:42 |
57 | UI: A Function of State | 02:47 |
58 | Conceptual Aside: Reducers | 06:32 |
59 | Actions and State | 08:57 |
60 | Hooks and State | 00:25 |
61 | Fibers, Hooks, and State | 04:05 |
62 | Conceptual Aside: Queues | 03:55 |
63 | Update Queues | 02:47 |
64 | State and Re-renders | 02:39 |
65 | useReducer (Part 1) | 07:49 |
66 | useReducer (Part 2) | 06:05 |
67 | useState (Part 1) | 04:17 |
68 | useState (Part 2) | 10:48 |
69 | Rules of Hooks | 07:44 |
70 | useState (Part 3) | 10:38 |
71 | Conceptual Aside: Shallow Equality and Object.is | 08:11 |
72 | Immutable State | 07:47 |
73 | Adding Your Own Side Effects: useEffect | 00:16 |
74 | Conceptual Aside: Pure Functions and Side Effects | 01:32 |
75 | Adding Your Own Effects | 11:47 |
76 | Dependencies | 08:07 |
77 | A Game of Ping Pong | 02:35 |
78 | Unmounting and Effects | 09:55 |
79 | Fetching Data...or not | 10:27 |
80 | Conceptual Aside: Stale Closures | 04:09 |
81 | useEffect and Stale Closures | 09:52 |
82 | What Not to Do | 10:26 |
83 | useRef and forwardRef | 00:24 |
84 | useRef | 04:27 |
85 | useRef and the DOM | 05:16 |
86 | forwardRef | 04:16 |
87 | Custom Hooks | 00:25 |
88 | Extracting Custom Hooks | 12:31 |
89 | Component Design | 00:22 |
90 | Real World Complexity and Loops | 18:31 |
91 | Lifting State Up | 28:52 |
92 | &&, 0, and Ternary Operators | 12:28 |
93 | Children | 06:02 |
94 | useContext | 00:51 |
95 | Prop Drilling | 04:29 |
96 | Context | 39:23 |
97 | Context with Caution | 02:21 |
98 | useId and Key | 00:31 |
99 | useId | 08:49 |
100 | Key | 15:29 |
101 | memo, useMemo, and useCallback | 00:19 |
102 | Conceptual Aside: Memoization | 07:32 |
103 | memo | 16:01 |
104 | useMemo | 20:16 |
105 | useCallback | 10:33 |
106 | React Forget | 01:38 |
107 | useContext and Reducer | 00:22 |
108 | useContext + Reducer | 27:23 |
109 | 3rd Party State Management | 06:15 |
110 | Toolchains | 00:50 |
111 | Conceptual Aside: Toolchains | 03:50 |
112 | Conceptual Aside: ES Modules | 06:02 |
113 | Create React App | 01:53 |
114 | Vite | 18:46 |
115 | Frameworks | 03:51 |
116 | Strict Mode | 00:33 |
117 | Adding Strict Mode | 03:34 |
118 | Extra Re-render | 10:28 |
119 | Extra Effect Re-run | 09:14 |
120 | Forms | 00:18 |
121 | Reorganizing Our App | 15:05 |
122 | Uncontrolled Inputs | 05:29 |
123 | Controlled Inputs | 13:44 |
124 | form | 08:00 |
125 | textarea, select, and More | 11:17 |
126 | 3rd Party Form Help | 01:58 |
127 | Future Form Features | 02:13 |
128 | React Dev Tools | 00:17 |
129 | Using Dev Tools | 03:22 |
130 | useDebugValue | 01:47 |
131 | CSS and Components | 00:24 |
132 | CSS and React | 03:16 |
133 | Toolchains and CSS | 04:11 |
134 | CSS Modules and more | 04:12 |
135 | Class Project | 00:24 |
136 | Spelling Bee | 01:59 |
137 | HTML Authoring | 03:22 |
138 | Getting the Data | 06:22 |
139 | Header | 08:49 |
140 | Honeycomb | 14:34 |
141 | Shuffle | 09:30 |
142 | Words and Letters | 12:10 |
143 | Word List | 14:50 |
144 | Score | 06:07 |
145 | Highlighted Letter | 06:09 |
146 | Final Thoughts | 03:30 |
147 | Meta-Frameworks | 00:21 |
148 | NextJS | 03:42 |
149 | Remix | 02:26 |
150 | Suspense | 00:31 |
151 | Suspense and Frameworks | 03:45 |
152 | Suspense and Remix | 10:16 |
153 | React Server Components | 00:53 |
154 | Server Components | 11:55 |
155 | Client Components and 'use client' | 05:29 |
156 | RSC Payload | 07:59 |
157 | Composing Client and Server Components | 07:30 |
158 | Conclusion | 01:37 |
Similar courses to Understanding React | Don’t Imitate Understand
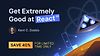
Learn React 19 with Epic React v2Kent C. Dodds
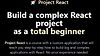
Project React. Build a complex React project as a total beginnerCosden Solutions
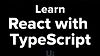
React with TypeScriptui.dev (ex. Tyler McGinnis)
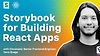
Storybook for building React appsfullstack.io
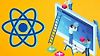
Mastering React With Interview Questions,eStore Project-2024udemy
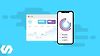
Microfrontends with React: A Complete Developer's GuideudemyStephen Grider
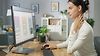
Complete DApp - Solidity & React - Blockchain Developmentudemy
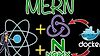