The Ultimate Redux Course
Redux is the most popular state management library for JavaScript apps. It's mostly used with React but it's also becoming popular in Angular, Ember and Vue communities. Despite being a small library with a small footprint, a lot of developers find Redux confusing and complicated. They also complain that Redux introduces a lot of boilerplate in the code and makes trivial things overly complicated.
Read more about the course
This course gives you a solid foundation on Redux and teaches you how to write clean and concise modern Redux code.
You'll learn everything about Redux including the whats, whys, hows, and industry best practices.
A short and sweet course that you can watch in an afternoon - free of fluff and nonsense.
Simply put, this is the Redux course that I wish I had when I started learning Redux.
By the end of this course…
You’ll be able to:
- Build fast and scalable apps with Redux
- Write clean, concise Redux code free of clutter and boilerplate
- Apply fundamental functional programming techniques
- Maintain existing Redux-based apps
- Prepare for technical interviews
What You'l Learn...
- What Redux is and when to use it
- Essential functional programming principles
- Use Redux Toolkit to kickstart Redux apps
- Debug apps using Redux DevTools
- Design a Redux store
- Write clean, concise, maintainable Redux code
- Properly structure Redux code
- Handle asynchronous events with redux-thunk
- Use existing middleware or write your own
- Test Redux code, the right way
- Connect React and Redux using react-redux
- The popular tools in Redux ecosystem
- Industry best practices and recommended patterns
- Common mistakes that many Redux devs make
- And much, much, more…
Who is this course for?
- Developers curious about Redux, the benefits it provides and how to use it properly
- Developers who want to add Redux to their skill set to get a raise or apply for a better job
Prerequisites
To take this course, you need to have at least 3 months of experience programming in JavaScript. You don't any familiarity with Redux. I'll teach you everything from the ground up.
Watch Online The Ultimate Redux Course
# | Title | Duration |
---|---|---|
1 | 2- What is Redux | 02:18 |
2 | 3- Pros and Cons of Redux | 05:30 |
3 | 4- Is Redux for You | 02:30 |
4 | 5- Setting Up the Development Environment | 01:49 |
5 | 1- Introduction | 00:29 |
6 | 2- What is Functional Programming | 01:26 |
7 | 3- Functions as First-class Citizens | 03:08 |
8 | 4- Higher-order Functions | 01:34 |
9 | 5- Function Composition | 03:39 |
10 | 6- Composing and Piping | 02:23 |
11 | 7- Currying | 05:45 |
12 | 8- Pure Functions | 02:51 |
13 | 9- Immutability | 04:15 |
14 | 10- Updating Objects | 04:34 |
15 | 11- Updating Arrays | 03:56 |
16 | 12- Enforcing Immutability | 01:06 |
17 | 13- Immutable.js | 03:40 |
18 | 14- Immer | 02:55 |
19 | 1- Introduction | 00:26 |
20 | 2- Redux Architecture | 05:04 |
21 | 3- Your First Redux App | 01:45 |
22 | 4- Designing the Store | 01:28 |
23 | 5- Defining the Actions | 03:27 |
24 | 6- Creating a Reducer | 06:14 |
25 | 7- Creating the Store | 01:27 |
26 | 8- Dispatching Actions | 02:46 |
27 | 9- Subscribing to the Store | 02:08 |
28 | 10- Action Types | 03:54 |
29 | 11- Action Creators | 02:59 |
30 | 12- Exercise | 00:18 |
31 | 13- Solution | 04:58 |
32 | 1- Introduction | 00:19 |
33 | 2- Redux Store | 01:11 |
34 | 3- Private Properties | 04:19 |
35 | 4- Dispatching Actions | 03:05 |
36 | 5- Subscribing to the Store | 02:37 |
37 | 1- Introduction | 00:16 |
38 | 2- Installing Redux DevTools | 02:56 |
39 | 3- The Basics | 02:26 |
40 | 4- Inspector Monitor | 03:53 |
41 | 5- Tracing | 04:27 |
42 | 6- Exporting and Importing | 01:36 |
43 | 1- Introduction | 00:16 |
44 | 2- Structuring Files and Folders | 02:37 |
45 | 3- Ducks Pattern | 05:47 |
46 | 4- Redux Toolkit | 01:36 |
47 | 5- Creating the Store | 02:46 |
48 | 6- Creating Actions | 05:28 |
49 | 7- Creating Reducers | 06:57 |
50 | 8- Creating Slices | 05:07 |
51 | 9- Exercise | 00:56 |
52 | 10- Solution | 03:23 |
53 | 1- Introduction | 00:20 |
54 | 2- Redux State vs Local State | 03:15 |
55 | 3- Structuring a Redux Store | 03:24 |
56 | 4- Combining Reducers | 04:10 |
57 | 5- Normalization | 01:47 |
58 | 6- Selectors | 03:38 |
59 | 7- Memoizing Selectors with Reselect | 04:48 |
60 | 8- Exercise | 00:22 |
61 | 9- Solution | 08:20 |
62 | 1- Introduction | 00:21 |
63 | 2- What is Middleware | 01:12 |
64 | 3- Creating Middleware | 06:09 |
65 | 4- Parameterizing Middleware | 01:45 |
66 | 5- Dispatching Functions | 08:15 |
67 | 6- Exercise | 00:49 |
68 | 7- Solution | 02:04 |
69 | 1- Introduction | 00:25 |
70 | 2- Setting Up the Backend | 01:16 |
71 | 3- The Approach | 03:36 |
72 | 4- API Middleware | 08:59 |
73 | 5- Actions | 05:51 |
74 | 6- Restructuring the Store | 02:22 |
75 | 7- Getting Data from the Server | 06:35 |
76 | 8- Loading Indicators | 05:04 |
77 | 9- Caching | 06:18 |
78 | 10- Saving Data to the Server | 03:41 |
79 | 11- Exercise | 00:19 |
80 | 12- Solution- Resolving Bugs | 04:05 |
81 | 13- Solution- Assigning a Bug to a User | 02:30 |
82 | 14- Reducing Coupling | 04:56 |
83 | 15- Cohesion | 01:49 |
84 | 1- Introduction | 00:40 |
85 | 2- What is Automated Testing | 03:11 |
86 | 3- Setting Up the Testing Environment | 04:44 |
87 | 4- Your First Unit Test | 05:31 |
88 | 5- Unit Testing Redux Applications | 05:11 |
89 | 6- Solitary Tests | 09:05 |
90 | 7- Social Tests | 09:21 |
91 | 8- Mocking HTTP Calls | 03:37 |
92 | 9- Writing Clean Tests | 05:40 |
93 | 10- Test Coverage | 01:44 |
94 | 11- Exercises | 00:24 |
95 | 12- Solution- getUnresolvedBugs | 06:00 |
96 | 13- Solution- resolveBug | 05:46 |
97 | 14- Solution- loadingBugs | 12:10 |
98 | 1- Creating a React App | 01:35 |
99 | 2- Installing Redux | 01:07 |
100 | 3- Providing the Store | 05:30 |
101 | 4- Subscribing and Dispatching | 05:11 |
102 | 5- Connecting Components Using react-redux | 08:33 |
103 | 6- Hooks | 05:59 |
104 | 8- Exercise | 00:25 |
105 | 9- Solution | 03:20 |
Similar courses to The Ultimate Redux Course
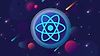
Complete React Developer in 2023 (w/ Redux, Hooks, GraphQL)udemyzerotomastery.io
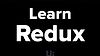
Reduxui.dev (ex. Tyler McGinnis)
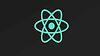
Starting with React & Redux: Build modern apps (2nd edition)udemy
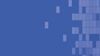
Advanced Projects in Electron 4, React, and Reduxudemy
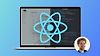
The Ultimate React Course 2024: React, Redux & Moreudemy
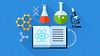
Server Side Rendering with React and ReduxudemyStephen Grider
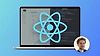
The Ultimate React Course 2024: React, Redux & Moreudemy
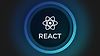
The Creative React and Redux Coursedevelopedbyed.com
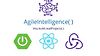
Full Stack HATEOAS: Spring Boot 2.1, ReactJS, Reduxudemy
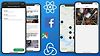