Building .NET REST APIs
10h 42m 54s
English
Paid
"Building .NET REST APIs" is a comprehensive course that guides you through the process of creating a production-ready, .NET-based REST API from the ground up. The course is led by Julio Casal, a seasoned software engineer, who brings extensive experience in building various .NET applications.
Read more about the course
Key Features of the Course:
- Complete REST API Development: The course focuses on building a complete REST API using the latest technologies and best practices from the .NET platform.
- Real-World Application: You will not only learn to develop the REST API for local environments but also how to deploy it fully on the Azure cloud, ensuring real-world applicability.
- Structured Learning Path: The course is structured to be step-by-step, making it suitable for learners of different levels, especially those looking to deepen their .NET skills.
- Focus on Latest Technologies: Emphasis is placed on using the most current and effective technologies and practices, ensuring the REST API you build is modern and efficient.
- Practical and Hands-on: Expect practical lessons and exercises that will help you not just understand but also apply the concepts in real-world scenarios.
Watch Online Building .NET REST APIs
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Course introduction | 00:39 |
2 | What this course covers | 03:55 |
3 | What you are going to build | 08:25 |
4 | Is this course for you? | 00:28 |
5 | Software prerequisites | 00:52 |
6 | Introduction to ASP.NET Core | 05:20 |
7 | Development environment setup | 04:26 |
8 | Introduction | 00:34 |
9 | ASP.NET Core Web API Essentials | 06:22 |
10 | Creating a Web API project | 09:44 |
11 | Building and debugging a Web API project | 05:37 |
12 | Adding the Game Entity | 11:21 |
13 | Implementing a GET ALL endpoint | 03:29 |
14 | Using Postman | 04:10 |
15 | Implementing a GET BY ID endpoint | 05:48 |
16 | Implementing the POST endpoint | 08:27 |
17 | Implementing the PUT endpoint | 07:24 |
18 | Implementing the DELETE endpoint | 04:12 |
19 | Introduction | 00:26 |
20 | Using Route Groups | 03:28 |
21 | Adding Server-Side Validation | 04:11 |
22 | Introduction to NuGet | 02:11 |
23 | Using NuGet packages | 04:37 |
24 | Refactoring the endpoints | 07:23 |
25 | Introduction | 00:33 |
26 | Introduction to the repository pattern | 01:43 |
27 | Adding the Games Repository | 10:41 |
28 | Understanding Dependency Injection | 04:57 |
29 | Understanding Service Lifetimes | 03:59 |
30 | Using Dependency Injection | 11:45 |
31 | Understanding Data Transfer Objects | 02:24 |
32 | Using Data Transfer Objects | 12:06 |
33 | Introduction | 00:28 |
34 | Introduction to Docker | 01:41 |
35 | Running SQL Server as a Docker container | 13:46 |
36 | Reading configuration from appsettings.json | 06:07 |
37 | Storing secrets for local development | 02:34 |
38 | Using Secret Manager to store a connection string | 05:34 |
39 | Introduction | 00:31 |
40 | Introduction to Entity Framework Core | 03:55 |
41 | Creating the DBContext | 07:56 |
42 | Generating database migrations | 07:01 |
43 | Configuring entities for database migration | 06:20 |
44 | Applying a database migration | 03:21 |
45 | Applying migrations on startup | 07:04 |
46 | Implementing an Entity Framework repository | 06:49 |
47 | Using the Entity Framework repository | 08:51 |
48 | Understanding the Asynchronous Programming Model | 03:25 |
49 | Using the asynchronous programming model | 12:08 |
50 | Introduction | 00:29 |
51 | Using Token Based Authentication | 02:34 |
52 | Securing an ASP.NET Core Web API | 04:37 |
53 | Creating Access Tokens | 04:03 |
54 | Understanding JSON Web Tokens | 02:42 |
55 | Using Access Tokens In Postman | 04:52 |
56 | Using Role Based Authorization | 03:54 |
57 | Using Claims Based Authorization | 11:35 |
58 | Introduction | 00:32 |
59 | Introduction to Logging | 02:03 |
60 | Sending logs via the WebApplication logger | 03:51 |
61 | Using ILoggerFactory | 02:36 |
62 | Using ILogger | 04:21 |
63 | Using a Json Logger | 03:37 |
64 | Using structured logging | 05:06 |
65 | Configuring log levels | 06:32 |
66 | Use HTTP Logging | 04:24 |
67 | Introduction | 00:27 |
68 | Catching and logging errors | 07:47 |
69 | Returning problem details | 07:48 |
70 | Introduction to Middleware | 01:46 |
71 | Adding a middleware via a request delegate | 08:02 |
72 | Creating a middleware class | 05:31 |
73 | Using the Built-In Exception Handler | 13:21 |
74 | Introduction | 00:27 |
75 | The importance of versioning | 01:56 |
76 | Using version specific DTOs | 03:16 |
77 | Adding URL path-based versioning support | 04:31 |
78 | Using the ASP.NET API Versioning package | 05:09 |
79 | Using Query String versioning | 02:18 |
80 | Specifying a default API version | 02:35 |
81 | Introduction | 00:21 |
82 | Running the Game Store Portal | 04:11 |
83 | Understanding CORS | 04:16 |
84 | Configuring CORS | 06:22 |
85 | Refactoring the CORS configuration | 03:33 |
86 | Introduction | 00:22 |
87 | Introduction to OAuth 2.0 | 03:56 |
88 | Introduction to OpenID Connect | 04:32 |
89 | Setting up Auth0 | 03:04 |
90 | Defining an Auth0 API | 03:11 |
91 | Defining Auth0 Roles and Users | 07:08 |
92 | Defining the Auth0 application | 03:15 |
93 | Introduction | 00:28 |
94 | Configuring the Auth0 scheme in the Web API | 06:06 |
95 | Getting and using access tokens in Postman | 10:29 |
96 | Adding support for multiple scopes | 10:12 |
97 | Getting and exploring ID tokens | 04:21 |
98 | Enable authentication in the Game Store client | 06:49 |
99 | Introduction | 00:22 |
100 | Adding pagination support to repositories | 06:54 |
101 | Add pagination support to the GetGames request | 03:54 |
102 | Adding pagination support to responses | 06:55 |
103 | Trying out pagination | 03:16 |
104 | Introduction | 00:20 |
105 | Add search support to repositories | 06:00 |
106 | Add search support to the request | 02:04 |
107 | Trying out search | 03:43 |
108 | Introduction | 00:23 |
109 | Adding OpenAPI support | 12:22 |
110 | Enable the Swagger UI experience | 07:55 |
111 | Describing response types | 07:01 |
112 | Adding summaries, tags and descriptions | 06:09 |
113 | Introduction | 00:25 |
114 | What Is Microsoft Azure? | 02:50 |
115 | Getting Your Azure Account | 02:15 |
116 | Quick tour of the Azure Portal | 06:54 |
117 | Creating a resource group | 05:47 |
118 | Introduction | 00:24 |
119 | File upload options | 02:56 |
120 | What Is Azure Storage? | 02:58 |
121 | Creating an Azure storage account | 02:32 |
122 | Implement the image uploader | 06:49 |
123 | Registering the Image uploader in the service container | 05:24 |
124 | Implementing the image upload endpoint | 07:31 |
125 | Trying out image upload | 06:50 |
126 | Introduction | 00:25 |
127 | Creating an Azure SQL database | 06:52 |
128 | Creating an Azure App Service | 08:32 |
129 | Enabling Logging to Azure App Service | 01:42 |
130 | Deploying the Web API | 10:12 |
131 | Introduction | 00:30 |
132 | Publishing the client to GitHub | 05:03 |
133 | Creating a Static Web App | 05:51 |
134 | Updating the Web API CORS policy | 03:13 |
135 | Adding Auth0 support to deployed client | 05:15 |
Read Book Building .NET REST APIs
# | Title |
---|---|
1 | Roadmap |
2 | Handouts |
3 | Net8Upgrade |
Similar courses to Building .NET REST APIs
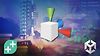
Complete C# Unity Game Developer 3Dudemy
Category: Unity, C Sharp (C#)
Duration 30 hours 34 minutes 50 seconds
Course
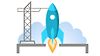
Automate All The Things With DevOpsJulio Casal
Category: C Sharp (C#)
Duration 3 hours 9 minutes 37 seconds
Course
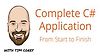
C# Application from Start to Finishiamtimcorey.com (Tim Corey)
Category: C Sharp (C#)
Duration 25 hours 24 minutes 50 seconds
Course