Building Microservices With .NET
18h 10m 56s
English
Paid
This course, "Building Microservices with .NET," is instructed by Julio Casal. It is designed to guide learners through the development of backend services for a client-based video game using the .NET platform. The game, requiring players to acquire items like potions and weapons using an in-game currency named "Jill", sets the context for the services being built.
Read more about the course
Key aspects of the course include:
- High-Level Architecture: Understanding the architecture involving client applications and backend services. The course doesn’t cover client-side app development but focuses on backend services essential for the game's functioning.
- Microservices-Based System: Learners will explore a system named the "Play Economy System," comprising four distinct microservices - Catalog, Inventory, Identity, and Trading, each with its database and inter-service communication via a message broker.
- Technologies and Frameworks: The course encompasses various technologies like .NET, ASP.NET Core, MongoDB, RabbitMQ, Mass Transit, Identity Server, React, Docker, and others for different components of the system.
- Authentication and Authorization: Using OpenID Connect for secure and reliable user authentication and authorization across services.
- API Gateway: Introduction to managing communications in cloud environments and handling cross-cutting concerns through an API gateway.
- Infrastructure Components: Emphasis on logging, distributed tracing, and monitoring using tools like Seq, OpenTelemetry, Jaeger, Prometheus, and Grafana to ensure system health and efficient troubleshooting.
- Practical Application: Access to source code and guidance on building and running a frontend portal and microservices on a local development environment.
- Hands-On Experience: The course includes practical exercises for setting up and using the necessary tools and technologies, ensuring a comprehensive learning experience.
Watch Online Building Microservices With .NET
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Course introduction | 04:07 |
2 | Development environment setup | 07:09 |
3 | Customizing VS Code for C# Development | 04:59 |
4 | What's wrong with the monolith? | 07:55 |
5 | What are microservices? | 09:47 |
6 | introduction | 00:54 |
7 | Creating a microservice via the .NET CLI | 10:18 |
8 | Preparing the initial project files | 16:03 |
9 | Introduction to the REST API and DTOs | 01:50 |
10 | Adding the DTOs | 03:18 |
11 | Adding the REST API operations | 25:12 |
12 | Handling invalid inputs | 11:18 |
13 | Introduction | 01:05 |
14 | Introduction to the repository pattern and MongoDB | 02:22 |
15 | Implementing a MongoDB repository | 14:06 |
16 | Using the repository in the controller | 10:54 |
17 | Introduction to Docker | 01:47 |
18 | Trying out the REST API with a MongoDB container | 12:54 |
19 | Introduction to Dependency Injection and Configuration | 04:57 |
20 | Implementing dependency injection and configuration | 16:53 |
21 | Introduction | 00:58 |
22 | Using Postman | 15:24 |
23 | Reusing common code via NuGet | 04:12 |
24 | Refactoring into a generic MongoDB repository | 10:51 |
25 | Refactoring MongoDB registration into extension methods | 07:05 |
26 | Moving generic code into a reusable NuGet package | 17:12 |
27 | Introduction to Docker Compose | 02:21 |
28 | Moving MongoDB to docker compose | 10:55 |
29 | Introduction | 01:24 |
30 | Creating the Inventory microservice | 26:23 |
31 | Introduction to synchronous communication | 03:43 |
32 | Implementing synchronous communication via IHttpClientFactory | 11:47 |
33 | Understanding timeouts and retries with exponential backoff | 03:30 |
34 | Implementing a timeout policy via Polly | 09:57 |
35 | Implementing retries with exponential backoff | 11:13 |
36 | Understanding the circuit breaker pattern | 03:06 |
37 | Implementing the circuit breaker pattern | 06:38 |
38 | Introduction | 01:34 |
39 | Introduction to asynchronous communication | 09:53 |
40 | Defining the message contracts | 05:25 |
41 | Publishing messages via MassTransit | 11:12 |
42 | Standing up a RabbitMQ docker container | 07:44 |
43 | Refactoring MassTransit configuration into the reusable NuGet package | 11:07 |
44 | Consuming messages for eventual data consistency | 20:44 |
45 | Removing the inter-service synchronous communication | 14:49 |
46 | Introduction | 01:15 |
47 | Installing Node.js | 03:17 |
48 | Getting started with the frontend | 14:43 |
49 | Understanding CORS | 05:56 |
50 | Adding the CORS middleware | 06:27 |
51 | Exploring the frontend to microservices communication | 17:42 |
52 | Introduction | 00:59 |
53 | Introduction to ASP.NET Core Identity | 06:31 |
54 | Creating the Identity microservice | 10:07 |
55 | Integrating ASP.NET Core Identity with MongoDB | 24:01 |
56 | Adding the Users REST API | 21:01 |
57 | Introduction | 00:57 |
58 | Microservices authentication | 06:55 |
59 | Introduction to OAuth 2.0 | 08:15 |
60 | Introduction to OpenID Connect | 06:24 |
61 | Introduction to IdentityServer | 04:32 |
62 | Introduction | 00:58 |
63 | Setting up IdentityServer | 15:24 |
64 | Requesting tokens via Postman | 23:07 |
65 | Understanding JSON Web Tokens | 05:37 |
66 | Securing the Catalog microservice | 18:43 |
67 | Generalizing how to add authentication to microservices | 14:20 |
68 | Securing the Inventory microservice | 12:59 |
69 | Securing the Identity microservice | 05:24 |
70 | Introduction | 00:45 |
71 | Understanding Authorization in ASP.NET Core | 04:56 |
72 | Seeding users and roles | 22:42 |
73 | Adding users to the Player role | 08:25 |
74 | Implementing role-based authorization | 22:56 |
75 | Implementing claims-based authorization | 00:00 |
76 | Introduction | 01:28 |
77 | Configuring CORS in the Identity microservice | 07:28 |
78 | Adding the frontend client to the IdentityServer configuration | 09:51 |
79 | Fixing the logout experience | 12:41 |
80 | Using ID tokens in the frontend client | 10:32 |
81 | Using access tokens in the frontend client | 12:11 |
82 | Fixing the user registration experience | 03:49 |
83 | Introduction | 00:47 |
84 | Database Transactions | 04:07 |
85 | Distributed Transactions | 04:48 |
86 | Introduction to Sagas | 03:26 |
87 | Choreographed VS Orchestrated Sagas | 06:48 |
88 | Introduction | 00:48 |
89 | Purchase Saga Overview | 02:49 |
90 | Publishing Catalog prices | 03:09 |
91 | Allowing services to customize the retry configuration | 07:37 |
92 | Granting and subtracting items via asynchronous messages | 20:18 |
93 | Debiting gil via asynchronous messages | 16:54 |
94 | Introduction | 01:07 |
95 | Creating a MassTransit state machine | 12:17 |
96 | Initializing the state machine | 07:19 |
97 | Adding the Purchase controller | 09:01 |
98 | Configuring the Trading microservice | 11:47 |
99 | Trying out the state machine | 09:56 |
100 | Querying the state machine via a controller action | 16:04 |
101 | Consuming Catalog prices in the Trading microservice | 06:34 |
102 | Adding a custom state machine activity | 18:48 |
103 | Sending and consuming Inventory messages from the state machine | 14:48 |
104 | Using the MassTransit In-Memory Outbox | 05:04 |
105 | Sending and consuming Identity messages from the state machine | 11:50 |
106 | Introduction | 00:42 |
107 | Compensating actions in the state machine | 16:47 |
108 | Idempotency in microservices | 05:39 |
109 | Adding idempotency to a controller action | 12:56 |
110 | Handling out of order messages in the state machine | 05:27 |
111 | Handling message duplication | 04:01 |
112 | Implementing idempotent consumers | 15:44 |
113 | Introduction | 00:39 |
114 | Store experience overview | 01:58 |
115 | Publishing inventory updated events | 08:03 |
116 | Publishing user updated events | 08:28 |
117 | Consuming Inventory and Identity events in the Trading microservice | 16:00 |
118 | Implementing the Store controller | 19:27 |
119 | Trying out the updated Frontend portal | 15:19 |
120 | Real-time updates via SignalR | 02:51 |
121 | Configuring SignalR authentication | 06:25 |
122 | Sending real-time updates from the state machine via SignalR | 04:11 |
Read Book Building Microservices With .NET
# | Title |
---|---|
1 | Roadmap_NetMicroservices |
2 | Handouts_NetMicroservices |
3 | Net8Upgrade |
Similar courses to Building Microservices With .NET
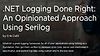
.NET Logging Done Right: An Opinionated Approach Using Serilogpluralsight
Category: C Sharp (C#)
Duration 5 hours 2 minutes 40 seconds
Course
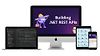
Building .NET REST APIsJulio Casal
Category: C Sharp (C#)
Duration 10 hours 42 minutes 54 seconds
Course
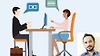
.NET/ C# Interview Masterclass - Top 500 Questions & Answersudemy
Category: Preparing for an interview, C Sharp (C#)
Duration 8 hours 31 minutes 35 seconds
Course
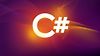
C# Advanced Topics: Take Your C# Skills to the Next Leveludemy
Category: C Sharp (C#)
Duration 3 hours 7 minutes 18 seconds
Course
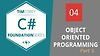
Foundation in C#: Object Oriented Programmingiamtimcorey.com (Tim Corey)
Category: C Sharp (C#)
Duration 3 hours 24 minutes 9 seconds
Course