Event-Driven Microservices Spring Boot, Kafka and Elastic
Hi there! My name is Ali Gelenler. I'm here to help you learn event-driven microservices architecture by applying best practices for real-life challenges. In this course, you will focus on the development of microservices. With the help of microservices you can independently develop and deploy your application components . You can also easily scale services according to each service's own resource needs, for example you can scale better and create more instances of a service that requires more requests.
Read more about the course
You can always use the latest versions for spring boot, spring cloud and other dependencies in this course. Please just follow the last section's lectures to see the required code and configuration changes for updated versions.
When moving from a monolith application to microservices architecture, some challenges will arise as a result of having a distributed application and system. In this course you will learn how to deal with these challenges using event-driven architecture (EDA) architecture with Apache Kafka.
With an event-driven architecture;
You will truly decouple the services and create resilient services because a service has no direct communication with other services
You will use asynchronous/non-blocking communication between services
You will use an event/state store (Kafka), and remove the state from the services for better scalability
You will develop a microservice architecture from scratch using the most recent software platforms, technologies, libraries and tools, following best practices, applying microservices patterns and using Java, Spring boot, Spring cloud, Spring Security, Kafka and Elasticsearch. We will also cover Event sourcing and Event-driven services using Kafka as the event store.
The microservices patterns that you will be implementing are:
Externalized configuration with Spring Cloud Config
CQRS with Kafka and Elastic search
Api versioning for versioning of Rest APIs
Service Registration and Discovery with Spring Cloud and Netflix Eureka
Api Gateway with Spring Cloud Gateway
Circuit breaker with Spring Cloud Gateway and Resilience4j
Rate limiting with Spring Cloud Gateway and Redis to use Redis as the Rate limiter
Distributed tracing with SLF4J MDC, Spring Cloud Sleuth and Zipkin
Log aggregation with ELK stack (Elasticsearch, Logstash and Kibana)
Client side load balancing with Spring Cloud Load Balancer
Database per Service
Messaging between microservices using Kafka
Watch Online Event-Driven Microservices Spring Boot, Kafka and Elastic
# | Title | Duration |
---|---|---|
1 | Introduction to microservices architecture | 14:00 |
2 | Project overview & Introduction to Event-driven architecture | 13:27 |
3 | Implementation details for each microservice | 10:43 |
4 | Setting up the environment | 04:24 |
5 | Introduction to Spring boot | 04:29 |
6 | Creating the base Spring boot project | 10:41 |
7 | The very first microservice | 15:55 |
8 | Streaming tweets with Twitter4j: The command component in CQRS & Event sourcing | 13:41 |
9 | Streaming tweets with Twitter Api V2 | 17:29 |
10 | Adding mock twitter stream as an alternative | 16:00 |
11 | Introducing Apache Kafka:Event sourcing, topics, partitions, producer & consumer | 15:50 |
12 | Adding common config module | 06:23 |
13 | Running Apache Kafka cluster with docker: Kafka, Zookeeper and Schema Registry | 09:44 |
14 | Creating kafka-model module | 03:06 |
15 | Creating kafka-admin module - Part 1: Configuration and dependencies | 10:40 |
16 | Creating kafka-admin module - Part 2: Creating Kafka topics programmatically | 13:57 |
17 | Creating kafka-producer module: Configuration of Kafka producer | 05:44 |
18 | Creating kafka-producer module: Produce events to store in Kafka event store | 07:03 |
19 | Integrate Kafka modules with Microservice: Use Kafka as event store for service | 08:54 |
20 | Containerization of microservice with docker image: Run all with docker compose | 07:56 |
21 | Creating Config Server Repository | 03:01 |
22 | Creating Spring Cloud Config Server as a Microservice | 06:56 |
23 | Using a common logback file for all microservices | 03:41 |
24 | Changing twitter-to-kafka-service to work with config server | 03:54 |
25 | Using remote GitHub repository | 05:30 |
26 | Adding security to config server and encrypt passwords | 02:31 |
27 | Using Jasypt to encrypt sensitive data | 08:05 |
28 | Using JCE to encrypt sensitive data | 06:13 |
29 | JCE vs Jasypt | 09:11 |
30 | Containerization of config server by creating the docker image | 09:28 |
31 | Introduction to Kafka Consumer: Reading data events from Kafka using messaging | 05:41 |
32 | Adding kafka-consumer module | 10:03 |
33 | Creating the microservice: kafka-to-elastic-service | 07:51 |
34 | Adding initialization check | 01:58 |
35 | Introducing Elasticsearch | 02:55 |
36 | Running elastic search with docker | 05:35 |
37 | Creating elastic-model module | 05:05 |
38 | Creating elastic-config module | 03:09 |
39 | Creating elastic-index-client module | 05:56 |
40 | Using Elasticsearch repositories for indexing | 06:16 |
41 | Integrating elastic modules with microservice | 07:49 |
42 | Containerization of microservice with docker image: Run all with docker compose | 06:20 |
43 | Introduction to Elastic Query Api | 10:19 |
44 | Creating elastic-query-client module | 12:05 |
45 | Using spring data elasticsearch repository for querying | 07:51 |
46 | Elastic query microservice: The query component in CQRS & Event sourcing | 06:18 |
47 | Creating web controller | 18:53 |
48 | Creating business layer to return elastic data in controller | 06:23 |
49 | Creating validation and controller advice | 07:08 |
50 | Introduction to Hateoas (Hypermedia as the Engine of Application State) | 02:26 |
51 | Adding Hateoas capability to Rest API with Spring Hateoas | 05:55 |
52 | Introduction to Api versioning of Rest API | 06:08 |
53 | Implementing Api versioning for elastic query service Rest API | 08:58 |
54 | Enabling Open Api v3 for documentation of RESTful API with Swagger v3 | 08:05 |
55 | Containerization of microservice by creating the docker image | 02:08 |
56 | Introduction to Web clients | 01:29 |
57 | Starting to implement elastic-query-web-client | 06:21 |
58 | Creating Thymeleaf templates with Bootstrap | 04:51 |
59 | Creating Rest API controller | 08:31 |
60 | Creating configurations for web client and security | 07:20 |
61 | Creating Webclient implementation | 09:09 |
62 | Adding client side load balancer with Spring Cloud Load Balancer | 09:03 |
63 | Containerization of microservice by creating the docker image | 02:25 |
64 | Creating common service and web client modules to re-use them with DRY principle | 06:25 |
65 | Creating reactive elastic query service: Reactive Spring and Flux reactive type | 12:33 |
66 | Creating reactive elastic web client: Reactive Spring, WebFlux and WebClient | 09:37 |
67 | Introduction to Oauth and Keycloak authorization server | 07:23 |
68 | Run and configure Keycloak authorization server with OIDC for authentication | 11:27 |
69 | Understanding Spring security with Spring Security OAuth 2.0 resource server | 02:37 |
70 | Configuring query service with Spring security Oauth 2.0, OpenId Connect and JWT | 12:40 |
71 | Adding user permissions with Spring security by reading from database | 14:16 |
72 | Configuring web client security with Spring boot security Oauth2, OIDC and JWT | 08:02 |
73 | Implementing SSO with Keycloak | 07:43 |
74 | Introduction to Kafka streams | 01:50 |
75 | Kafka streams microservice base project | 04:32 |
76 | Completing the Kafka streams microservice | 09:03 |
77 | Creating an endpoint for Kafka state store | 03:23 |
78 | Calling the Kafka State Store from Query Service | 10:21 |
79 | Analytics microservice configuration with Spring Boot Data JPA and PostgreSQL | 04:02 |
80 | Completing the Analytics microservice with Spring Data JPA and PostgreSQL | 06:02 |
81 | Creating an Rest API endpoint for analytics data | 01:53 |
82 | Calling the analytics Rest API from query service | 04:45 |
83 | Introduction to service registry and service discovery with Spring Eureka | 01:28 |
84 | Integrating Spring eureka discovery service with microservices | 06:32 |
85 | Introduction to Api Gateway: Resilience4J Circuit Breaker and Redis Rate Limiter | 05:15 |
86 | Implementing Api Gateway with Springcloud gateway, Redis and Resilience4J | 08:53 |
87 | Integrate Spring Cloud Api Gateway Circuit Breaker & Rate limiting with services | 06:22 |
88 | Configure microservices with Spring boot actuator, Prometheues and Micrometer | 01:47 |
89 | Adding monitoring and creating dashboards with Grafana | 05:00 |
90 | Configuring microservices to work with Logstash | 04:58 |
91 | Adding Logstash and Kibana into application: Complete ELK stack | 02:51 |
92 | Making gateway and config server high available and improve log file naming | 03:22 |
93 | Introducing Spring Cloud Sleuth and Zipkin | 04:30 |
94 | Integrating Spring Cloud Sleuth and Zipkin | 11:31 |
95 | Update to spring boot 2.5.0 | 15:51 |
96 | Update to spring boot 2.5.3 & Update other dependencies and docker images | 20:32 |
97 | Update to spring boot 2.5.6 | 07:12 |
98 | Update to spring boot 2.6.3 | 09:28 |
99 | Update to spring boot 2.7.0 | 10:56 |
100 | Update to spring boot 2.7.5 | 07:17 |
Similar courses to Event-Driven Microservices Spring Boot, Kafka and Elastic
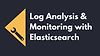
Log Analysis with ElasticsearchAndreas Kretz
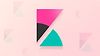
Data Visualization with Kibanaudemy
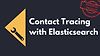