Building a Virtual Machine for Programming Language
How programming languages work under the hood? What’s the difference between compiler and interpreter? What is a virtual machine, and JIT-compiler? And what about the difference between functional and imperative programming? There are so many questions when it comes to implementing a programming language! The problem with “compiler classes” in school is such classes are usually presented as some “hardcore rocket science” which is only for advanced engineers.
Read more about the course
Moreover, classic compiler books start from the least significant topic, such as Lexical analysis, going straight down to the theoretical aspects of formal grammars. And by the time of implementing the first Tokenizer module, students simply lose an interest to the topic, not having a chance to actually start implementing a programing language itself. And all this is spread to a whole semester of messing with tokenizers and BNF grammars, without understanding an actual semantics of programming languages.
I believe we should be able to build and understand a full programming language semantics, end-to-end, in 4-6 hours — with a content going straight to the point, showed in live coding sessions as pair-programming and described in a comprehensible way.
In the Building a Virtual Machine class we focus specifically on runtime semantics, and build a stack-based VM for a programming language very similar to JavaScript or Python. Working closely with the bytecode level you will understand how lower-level interpretation works in production VMs today.
Implementing a programing language would also make your practical level in other programming languages more professional.
Prerequisites
There are two prerequisites for this class.
The Building a Virtual Machine course is a natural extension for the previous class — Building an Interpreter from scratch (aka Essentials of Interpretation), where we build also a full programming language, but at a higher, AST-level. Unless you already have understanding of how programming languages work at this level, i.e. what eval, a closure, a scope chain, environments, and other constructs are — you have to take the interpreters class as a prerequisite.
Also, going to lower (bytecode) level where production VMs live, we need to have basic C++ experience. This class however is not about C++, so we use just very basic (and transferrable) to other languages constructs.
Watch the introduction video for the details.
Who this class is for?
This class is for any curious engineer, who would like to gain skills of building complex systems (and building a programming language is an advanced engineering task!), and obtain a transferable knowledge for building such systems.
If you are interested specifically in compilers, bytecode interpreters, virtual machines, and source code transformation, then this class is also for you.
What is used for implementation?
Since lower-level VMs are about performance, they are usually implemented in a low-level language such as C or C++. This is exactly what we use as well, however mainly basic features from C++, not distracting to C++ specifics. The code should be easily convertible and portable to any other language, e.g. to Rust or even higher-level languages such as JavaScript — leveraging typed arrays to mimic memory concept. Using C++ also makes it easier implementing further JIT-compiler.
Note: we want our students to actually follow, understand and implement every detail of the VM themselves, instead of just copy-pasting from final solution. Even though the full source code for the language is presented in the video lectures, the code repository for the project contains /* Implement here */ assignments, which students have to solve.
Watch Online Building a Virtual Machine for Programming Language
# | Title | Duration |
---|---|---|
1 | Introduction to Virtual Machines | 19:23 |
2 | Stack-based vs. Register-based VMs | 09:37 |
3 | Logger implementation | 04:01 |
4 | Numbers | Introduction to Stack | 08:01 |
5 | Math binary operations | 07:08 |
6 | Strings | Introduction to Heap and Objects | 06:40 |
7 | Syntax | Parser implementation | 09:21 |
8 | Compiler | Bytecode | 09:14 |
9 | Complex expressions | 05:27 |
10 | Comparison | Booleans | 05:19 |
11 | Control flow | Branch instruction | 08:19 |
12 | Disassembler | 08:46 |
13 | Global variables | 11:08 |
14 | Blocks | Local variables | 14:41 |
15 | Control flow | While-loops | 03:35 |
16 | Native functions | 07:53 |
17 | User-defined functions | 12:08 |
18 | Call stack | Return address | 06:38 |
19 | Lambda functions | 05:28 |
20 | Bytecode optimizations | 05:32 |
21 | Closures | Scope analysis | 17:56 |
22 | Closures | Compilation | 09:32 |
23 | Closures | Runtime | 12:25 |
24 | Tracing heap | Object header | 11:01 |
25 | Mark-Sweep GC | 15:04 |
26 | Class objects | Methods storage | 13:24 |
27 | Instance objects | Property access | 10:58 |
28 | Super classes | Inheritance | 02:14 |
29 | Final VM executable Final VM executable | 06:15 |
Similar courses to Building a Virtual Machine for Programming Language
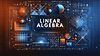
Fundamentals to Linear AlgebraLunarTech
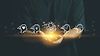
Team Dynamics and Soft Skills for Developers | Don’t ImitateAnthony Alicea
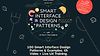
Smart Interface Design Patterns Vitaly Friedmansmashingmagazine.com
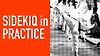
Sidekiq in PracticeNate Berkopec
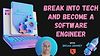
Break Into Tech And Become A Software EngineerBrian Jenney
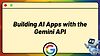
Building AI Apps with the Gemini APIzerotomastery.io
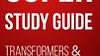